Overcoming Common Pitfalls in Java CI/CD with Azure DevOps
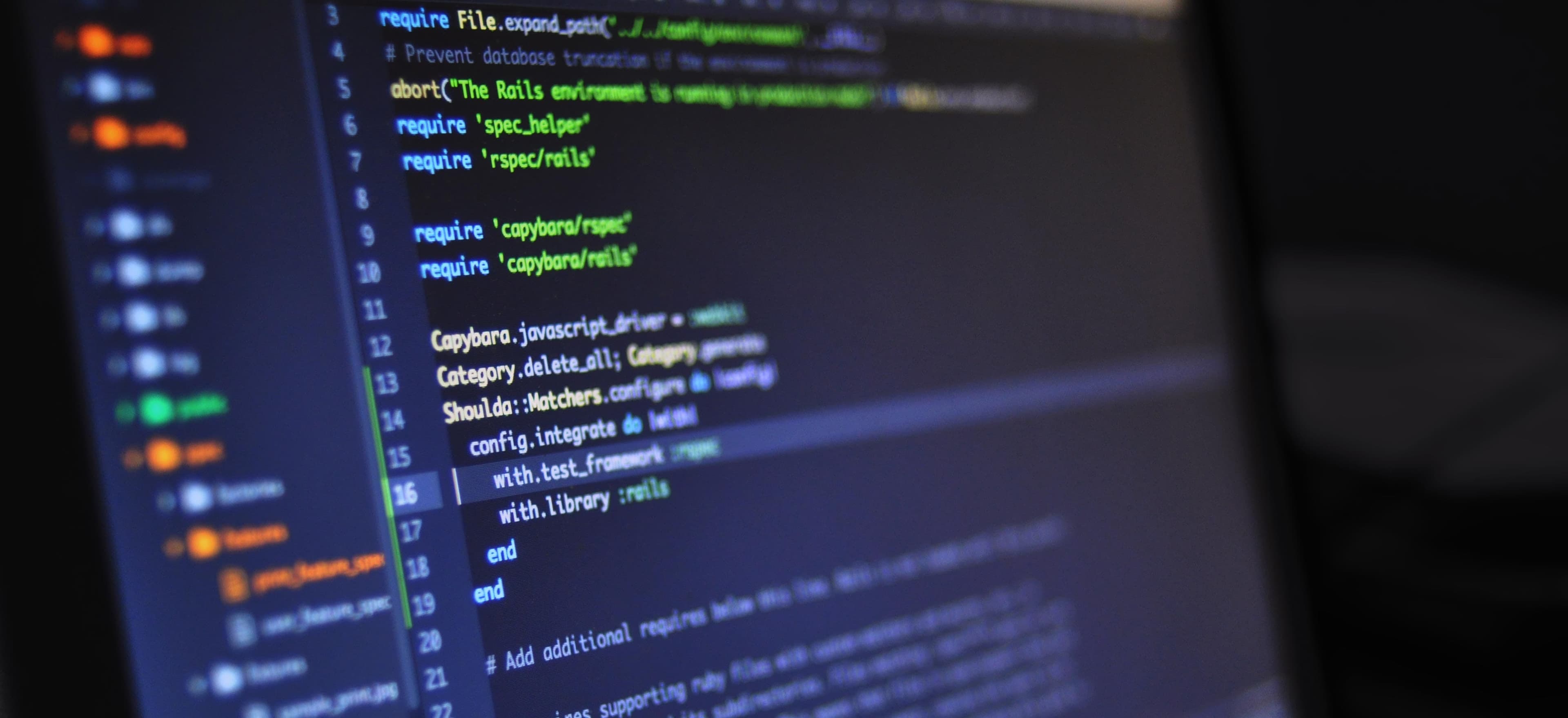
- Published on
Overcoming Common Pitfalls in Java CI/CD with Azure DevOps
As software development continues to evolve rapidly, Continuous Integration (CI) and Continuous Deployment (CD) have become integral methodologies. They enhance collaboration, speed up development cycles, and ultimately lead to a smoother deployment process. However, diving into CI/CD, especially within a Java environment using Azure DevOps, can come with its own set of challenges. In this post, we will discuss common pitfalls encountered in Java CI/CD with Azure DevOps and how to overcome them.
Table of Contents
- Understanding CI/CD Concepts
- Common Pitfalls in Java CI/CD
- Configuration Issues
- Build Failures
- Testing Pitfalls
- Deployment Challenges
- Best Practices to Overcome Pitfalls
- Conclusion: Embracing CI/CD in Your Java Projects
Understanding CI/CD Concepts
What is CI/CD?
Continuous Integration (CI) is the practice of automatically building and testing code changes, ensuring that new code integrates smoothly with the existing codebase. Continuous Deployment (CD) extends this concept by automating the release of this integrated code to production.
Why Use CI/CD?
- It reduces integration issues.
- It accelerates the release process.
- It promotes code quality through automated testing.
Java and Azure DevOps
Java is a versatile and powerful programming language that plays a significant role in enterprise applications. Azure DevOps, on the other hand, offers a range of tools and services to support the entire development lifecycle. Using both in tandem enables teams to streamline their workflows, automate testing, and deliver software more reliably.
Getting Started with Azure DevOps
Before jumping into pitfalls, make sure you have a solid foundation set up in Azure DevOps. Create a project, set up your repository, and explore pipelines for CI/CD.
Common Pitfalls in Java CI/CD
1. Configuration Issues
The Problem: Misalignment in configurations can lead to unexpected behaviors during the build and deployment stages.
Solution:
Ensure your build configurations are stable. Utilize pom.xml
in Maven or build.gradle
in Gradle effectively by consolidating dependencies and plugins.
Here’s an example of a basic pom.xml
snippet highlighting key configuration elements:
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-java-app</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>11</maven.compiler.source>
<maven.compiler.target>11</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Additional dependencies -->
</dependencies>
</project>
Why This Matters? Setting Java version sources ensures that you maintain compatibility and avoid runtime errors.
2. Build Failures
The Problem: Intermittent or consistent build failures can significantly disrupt development flow.
Solution: Monitor your CI pipeline by breaking down the build into smaller steps. Implement linting tools to catch issues early.
Here’s an example of defining a build pipeline in Azure DevOps:
trigger:
branches:
include:
- main
pool:
vmImage: 'ubuntu-latest'
steps:
- task: Maven@3
inputs:
mavenPomFile: 'pom.xml'
goals: 'package'
options: '-DskipTests=true'
Why This Matters?
Using the -DskipTests=true
option can speed up builds. However, ensure that tests are run in subsequent build steps.
3. Testing Pitfalls
The Problem: Not utilizing testing frameworks properly can result in inadequate code verification.
Solution: Leverage JUnit or TestNG for unit tests and set up integration tests. Use code coverage tools like Jacoco to ensure your tests cover the right areas.
JUnit example:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calc = new Calculator();
assertEquals(3, calc.add(1, 2));
}
}
Why This Matters? Writing clear tests and ensuring high code coverage helps catch bugs early, making your application more robust.
4. Deployment Challenges
The Problem: Inconsistent deployment environments lead to "It works on my machine" scenarios.
Solution: Use containerization with Docker or orchestration tools like Kubernetes. This provides consistency across environments.
Dockerfile example:
FROM openjdk:11-jdk
COPY target/my-java-app.jar /usr/app/my-java-app.jar
WORKDIR /usr/app
CMD ["java", "-jar", "my-java-app.jar"]
Why This Matters? Docker ensures that your application runs in the same environment during development, testing, and production, which minimizes discrepancies.
Best Practices to Overcome Pitfalls
-
Automate Everything: Automate your builds and deployments to minimize manual errors.
-
Monitor and Log: Implement logging and monitoring to quickly identify issues in the CI/CD pipeline.
-
Use Branch Policies: Establish branch policies to maintain code quality and approval processes before merging into the main branch.
-
Document Your Processes: Document your CI/CD processes well so that new team members can onboard easily and existing members can reference best practices.
-
Regularly Review and Refactor: Regularly check your CI/CD configurations and codebase for improvement opportunities.
-
Stay Informed: Keep abreast of updates on Java and Azure DevOps to leverage new features and best practices.
The Closing Argument: Embracing CI/CD in Your Java Projects
The benefits of integrating CI/CD into your Java projects using Azure DevOps are numerous, but so are the pitfalls. By understanding these challenges and implementing the best practices discussed, your teams can foster a more efficient, streamlined, and dependable development process. Remember: the journey to a robust CI/CD pipeline is ongoing. It requires commitment, continuous learning, and adaptation.
For further reading, check out the official Azure DevOps documentation which provides more in-depth knowledge on the tools available at your disposal. Embrace the evolution of software development and let CI/CD guide your Java applications to success!
This blog post provides you with valuable insights gleamed from industry experience, actionable solutions, and a pathway to improving your development pipeline. Implement these practices, and you will not only overcome common pitfalls but will also drive your Java projects towards a resilient and agile future.
Checkout our other articles