Missing Ceylon Language Features That Could Improve Java
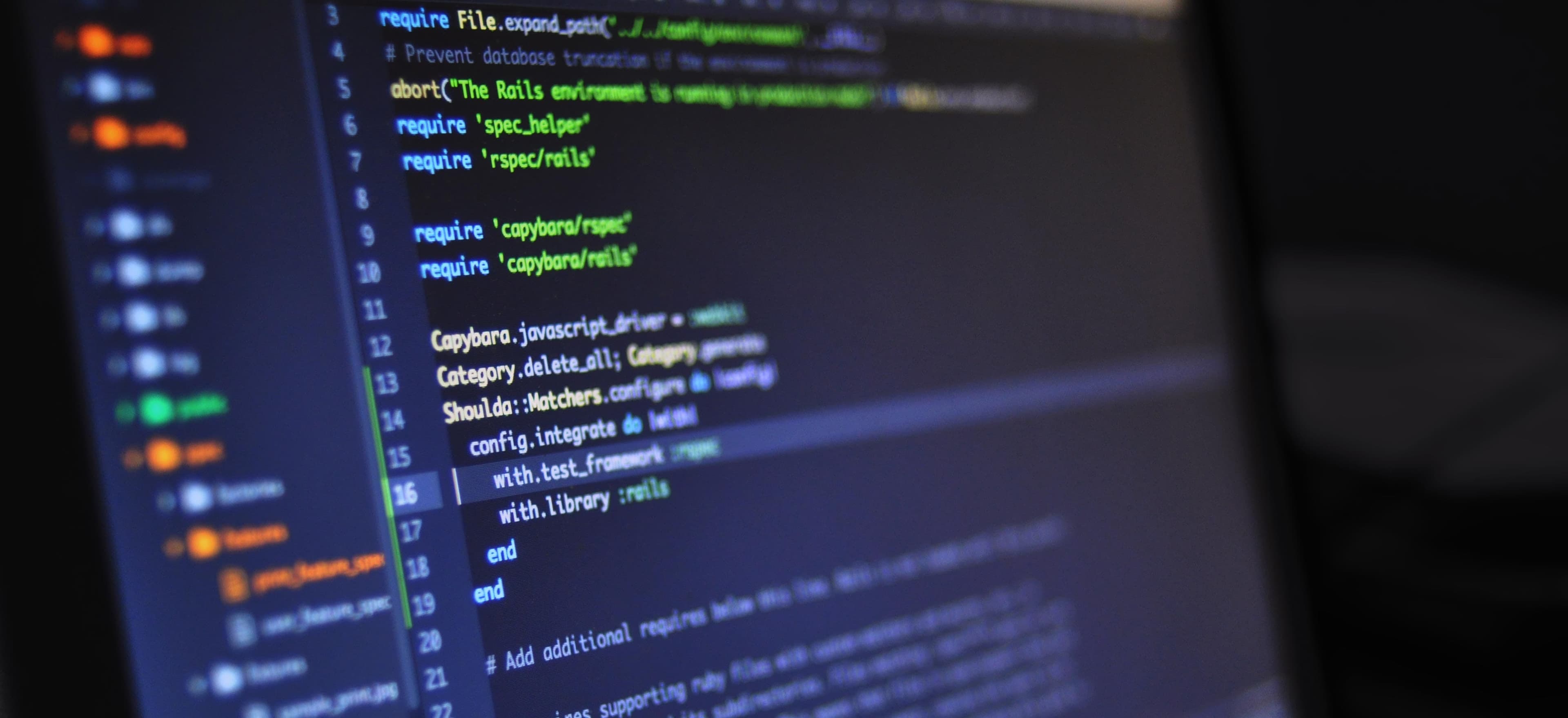
- Published on
Missing Ceylon Language Features That Could Improve Java
Java has long been a prominent player in the programming world, known for its robustness, portability, and security. However, as technologies and designs evolve, so too do our expectations for programming languages. One such language that has garnered interest is Ceylon, a language designed with modern programming concepts in mind. In this post, we’ll take a look at some of the missing features from Ceylon that could greatly enhance the Java programming experience.
1. Type Inference
What Is Type Inference?
Type inference allows the compiler to automatically deduce the type of a variable based on the assigned value, sparing developers from explicitly declaring the type. Ceylon embraces this feature, which results in cleaner, more concise code.
Example of Type Inference in Ceylon
shared void run() {
value myString = "Hello, Ceylon"; // The type is automatically inferred as `String`
print(myString);
}
Why Java Needs Type Inference
Java's verbosity can be a double-edged sword. While explicit type declarations provide clarity, they can also create unnecessary length. With type inference introduced in Java 10 through the var
keyword, the language took a step towards modernity. However, it could be taken further by allowing more scenarios where type inference applies.
Imagine a more succinct way to declare lambda expressions or complex generic types:
var list = new ArrayList<String>(); // Instead of: ArrayList<String> list = new ArrayList<>();
This less cluttered syntax encourages readability without losing type safety.
2. First-Class Functions
The Concept of First-Class Functions
In languages like Ceylon, functions are treated as first-class citizens. This means they can be assigned to variables, passed as arguments, and returned from other functions.
Example of First-Class Functions in Ceylon
shared void run() {
value greet = (String name) => "Hello, " + name;
value message = greet("Ceylon");
print(message);
}
The Impact on Java
While Java has come a long way, especially with the introduction of lambda expressions and functional interfaces in Java 8, it still falls short of true first-class function support. With Java's imperative nature, developers often find themselves resorting to anonymous classes for more complex function management.
Imagine how powerful Java could be with first-class functions. It would allow for cleaner, more expressive code, enabling seamless integration of functional programming concepts. Here’s a hypothetical scenario:
// Hypothetical scenario (Java-like pseudo-code)
Function<String, String> greet = name -> "Hello, " + name;
String message = greet.apply("Java");
System.out.println(message);
This would facilitate a more functional approach to Java development, making it easier to work with higher-order functions.
3. Modular System
Why Modularity Matters
Ceylon introduces a modular system that facilitates better management of dependencies, allowing developers to focus on specific components of their applications without worrying about the larger ecosystem. This becomes particularly relevant in enterprise applications where robustness is key.
Example of Modularity in Ceylon
Ceylon uses modules that make it easier to segregate code. Here’s how you might define a module:
module my.module {
import my.other.module;
}
The Java Challenge
Java 9 introduced the Java Platform Module System (JPMS), yet many developers still find it challenging to navigate। With dependency management often feeling cumbersome, a more streamlined process would benefit the organization and scalability of Java applications.
Implementing true modularity would provide significant advantages:
- Easier code sharing and collaboration.
- Reduced namespace pollution.
- Improved compile-time performance.
4. Nullable Types
Null Safety in Ceylon
Ceylon’s approach towards types includes built-in null safety features. This means that the compiler helps prevent null pointer exceptions, which are a common source of runtime errors in Java.
Example of Nullable Checks in Ceylon
String? name = null; // This is allowed
if (name != null) {
print(name.length);
}
Java’s Null Safety Issue
Java developers face the notorious null pointer exception. While Optional has been introduced, it’s often seen as a workaround rather than a native solution. With nullable types, developers could avoid unnecessary workarounds and focus on more relevant aspects of their logic.
Imagine a more intuitive Java way of handling potential null values:
String name = null; // Declare a nullable type (hypothetical syntax)
if (name != null) {
System.out.println(name.length());
}
Integrating nullable types into the Java language could greatly reduce error rates and improve overall application stability.
5. Augmented Constructors
Constructors in Ceylon
Ceylon allows for more flexibility in constructor design by supporting default parameters, enabling cleaner construction of objects.
Example of Constructors in Ceylon
class Person(String name, Integer age = 30) {
shared void printInfo() {
print("Name: " + name + ", Age: " + age);
}
}
Relevance to Java
In Java, every parameter must be explicitly passed unless utilizing method overloading, which can become cumbersome—especially when many parameters are optional. This can lead to multiple constructors, cluttering your class.
Consider the benefit of a cleaner constructor in Java:
public class Person {
private String name;
private int age;
public Person(String name) {
this(name, 30); // Default age parameter
}
public Person(String name, int age) {
this.name = name;
this.age = age;
}
}
This would significantly reduce redundancy and improve readability for developers.
Closing Remarks
Ceylon introduces a wealth of features that modernize the programming landscape. By borrowing ideas from Ceylon, Java can maintain its strength while also embracing modern best practices that enhance clarity, reduce verbosity, and mitigate common coding pitfalls.
Incorporating features like type inference, first-class functions, a modular system, nullable types, and augmented constructors could place Java on an undeniable upward trajectory. Each of these enhancements wouldn't dilute the essence of Java but rather elevate it to better align with the evolving landscape of software development.
For a deeper dive into both languages, check out the Ceylon Language Documentation and further explore Java through the Official Java Documentation.
Let's advocate for languages that not only have a rich history but also an innovative future!
Checkout our other articles