Mastering Java: Control Class and Interface Visibility
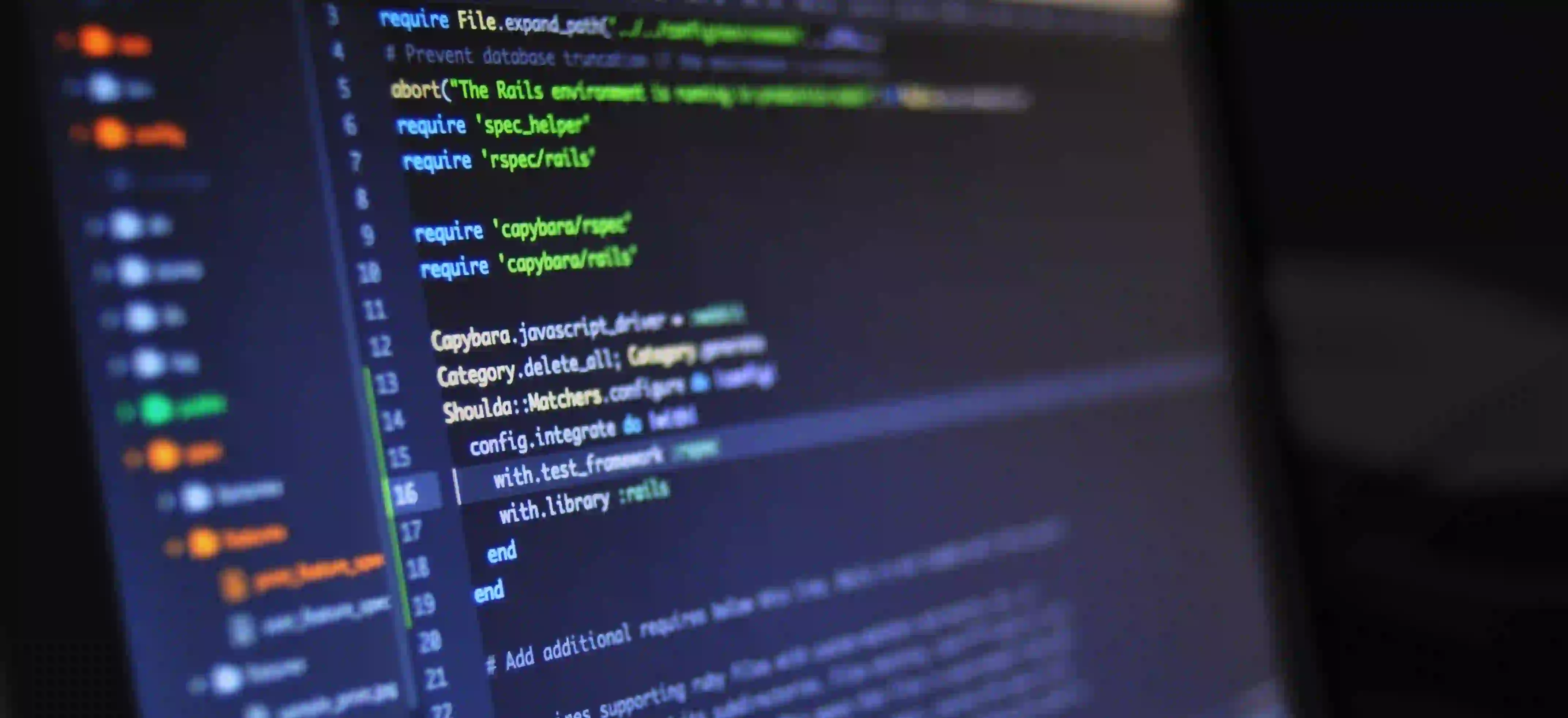
Mastering Java: Control Class and Interface Visibility
Java is a robust programming language that implements a variety of access control mechanisms to enhance encapsulation and manage visibility between classes and interfaces. Understanding these mechanisms is fundamental for developing responsible object-oriented designs. In this blog post, we will explore access modifiers, delve into how they control the visibility of classes and interfaces, and examine practical examples that demonstrate their use.
Understanding Access Modifiers in Java
Before we dive into classes and interfaces, let's touch on the primary access modifiers Java offers:
- Public: The class or member is visible to all classes everywhere, regardless of the package.
- Protected: The class or member is accessible within its own package and by subclasses outside the package.
- Default (package-private): If no modifier is specified, the class or member is accessible only within its own package.
- Private: The class or member is accessible only within the class it is declared.
The choice of access modifier impacts the design of your Java application and its maintainability.
Controlling Class Visibility
Public Classes
You can declare a class as public if it needs to be accessed from outside its package:
// Public class declaration
public class PublicExample {
public void display() {
System.out.println("This is a public class!");
}
}
In the example above, PublicExample
can be instantiated by any other class from any package. This is ideal for utility classes or APIs that need to be accessible globally.
Default Classes
Classes without an access modifier are default level, meaning they're accessible only within the same package:
// Default class
class DefaultExample {
void display() {
System.out.println("This is a default class!");
}
}
You’d typically use default visibility when you want to hide implementation details from other packages while still allowing classes within the same package to interact.
Protected Classes
The protected modifier allows a class to be inherited by subclasses, even if those subclasses exist in different packages:
// Protected base class
protected class ProtectedBase {
protected void show() {
System.out.println("This is a protected method!");
}
}
However, note that ProtectedBase
must reside in a separate .java
file as protected classes can’t be public, and the correct usage of protected is vital for promoting inheritance.
Private Classes
Private classes are confined to their outer class. This is particularly useful in providing a nested structure. Here’s a quick example:
class OuterClass {
private class PrivateInner {
void display() {
System.out.println("This is a private inner class!");
}
}
void invokeInner() {
PrivateInner inner = new PrivateInner();
inner.display();
}
}
In this case, PrivateInner
can only be accessed through OuterClass
, emphasizing tight encapsulation.
Controlling Interface Visibility
Public Interfaces
Interfaces declared as public can be implemented by any class, irrespective of the package.
public interface PublicInterface {
void method();
}
Implementing this interface allows a class to be treated polymorphically, where different classes can provide their implementations of the method
.
Default Interfaces
Java 8 introduced default methods in interfaces, which can have a default implementation.
interface DefaultInterface {
default void defaultMethod() {
System.out.println("This is a default method in interface!");
}
}
Any class implementing DefaultInterface
has the option to override defaultMethod
or use the default implementation.
Protected Interfaces
While there is no direct declaration for a protected interface, the concept of protected members within an interface is leveraged through containing classes:
class BaseClass {
protected interface ProtectedInterface {
void show();
}
}
// Implementing class
class ImplementationClass extends BaseClass implements BaseClass.ProtectedInterface {
public void show() {
System.out.println("Showing from Protected Interface!");
}
}
This demonstrates how the interface is effectively "limited" but still usable by subclasses of BaseClass
.
Private Interfaces
Private interfaces can only be used within the containing class:
class MethodHolder {
// Private interface
private interface PrivateInterface {
void execute();
}
// Implementation within outer class
public void executeMethod() {
class InnerClass implements PrivateInterface {
public void execute() {
System.out.println("Private interface executed!");
}
}
InnerClass inner = new InnerClass();
inner.execute();
}
}
Private interfaces can add a layer of abstraction and encapsulation by limiting their visibility to where they are specifically needed.
Best Practices for Access Modifiers
When designing your classes and interfaces, consider the following best practices:
-
Minimize Visibility: Start with the least visibility needed. If your class doesn't need to be public, make it default or private. This limits your class's exposure and enhances security.
-
Use Protected Sparingly: If you're not planning to use inheritance heavily, consider default or public instead. Protected can lead to tight coupling between components.
-
Favor Private: For inner classes or helper classes that are not needed outside the outer class, use private visibility.
-
Document Intent: Clearly document your access modifiers and their intended use. This improves code readability and maintainability.
Final Considerations
Understanding how to control class and interface visibility through access modifiers is an essential skill in Java programming. The judicious application of these mechanisms is crucial for creating maintainable, secure, and robust applications.
For further reading, you may want to check out the Java Access Modifiers documentation.
In summary, embrace encapsulation, balance flexibility with control, and let your applications shine with clear visibility guidelines. Happy coding!