Common MyBatis Configuration Pitfalls and How to Avoid Them
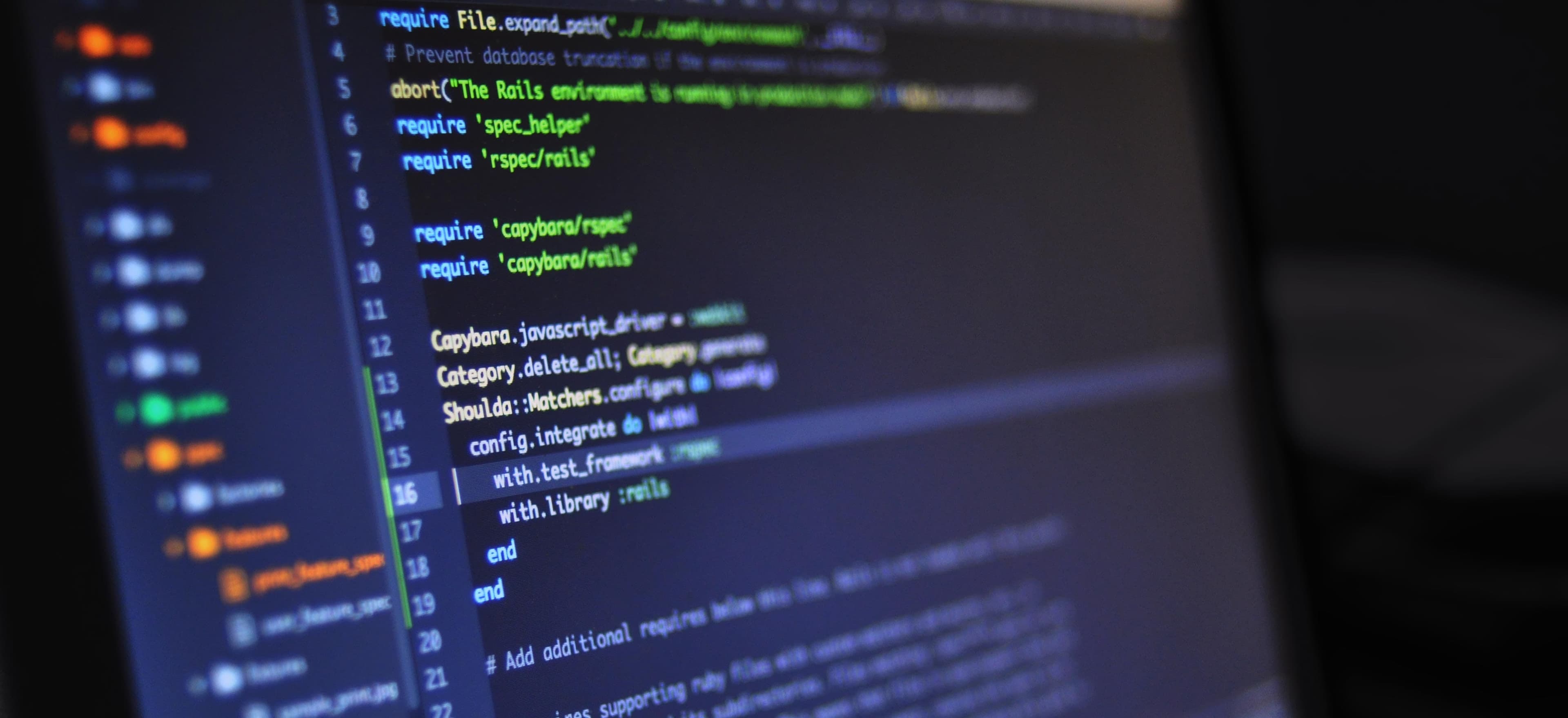
- Published on
Common MyBatis Configuration Pitfalls and How to Avoid Them
MyBatis is a popular Java framework used for mapping SQL databases to Java objects. While it is powerful and flexible, improper configuration can lead to various pitfalls that can compromise performance and maintainability. In this blog post, we will explore common MyBatis configuration pitfalls and provide actionable advice to avoid them.
Table of Contents
- Introduction to MyBatis
- Pitfall 1: Misconfiguring the DataSource
- Pitfall 2: Incorrect Mapper Scanning
- Pitfall 3: Poor SQL Mapping
- Pitfall 4: Overusing Lazy Loading
- Pitfall 5: Ignoring Best Practices for Caching
- Conclusion
Stepping into the Topic to MyBatis
MyBatis simplifies the database interaction by allowing developers to write SQL and map it to Java objects. It gives you the freedom of fine-tuning your SQL queries while taking care of object mapping. However, the convenience can quickly turn into confusion without proper configuration. Below are some pitfalls you should watch out for when configuring MyBatis.
Pitfall 1: Misconfiguring the DataSource
One of the most common mistakes in setting up MyBatis is misconfiguring the DataSource
. If the connection details are not correct, you might face runtime exceptions that could be difficult to diagnose.
How to Avoid It
Always double-check your database URL, username, and password. Here’s how to configure a DataSource in your mybatis-config.xml
:
<configuration>
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="com.mysql.cj.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/mydb"/>
<property name="username" value="user"/>
<property name="password" value="pass"/>
</dataSource>
</environment>
</environments>
</configuration>
This code initializes a pooled DataSource
, which is ideal for production environments as it manages connections efficiently. Make sure that you have the appropriate JDBC driver in your classpath. If you're using Maven, add the dependency:
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.29</version>
</dependency>
Pitfall 2: Incorrect Mapper Scanning
Mapping the SQL queries with Java methods can be tricky. If the MyBatis configuration does not point to the correct mapper locations, you may face IllegalArgumentException
or other runtime errors.
How to Avoid It
Ensure you specify the correct locations where your mappers are located. Here's an example:
<mappers>
<mapper resource="com/example/mappers/UserMapper.xml"/>
<mapper class="com.example.mappers.ProductMapper"/>
</mappers>
In this configuration, the first mapper is defined by the XML file, while the second mapper is defined by the Java class. It’s crucial to maintain proper naming conventions and package structures to avoid confusion.
Pitfall 3: Poor SQL Mapping
SQL mapping can easily become unwieldy if not carefully structured. Complex repetitive queries not only lead to inefficient performance but also make your code harder to read and maintain.
How to Avoid It
Utilize MyBatis's support for SQL fragments, which allows you to define reusable SQL snippets. Here’s an example of using SQL fragments:
<sql id="Base_Column_List">
id, username, email
</sql>
<select id="selectUser" resultType="User">
SELECT
<include refid="Base_Column_List"/>
FROM users
WHERE id = #{id}
</select>
By encapsulating frequently used columns in an <sql>
tag, you can keep your mapper files cleaner and easier to manage.
Pitfall 4: Overusing Lazy Loading
Lazy loading is a powerful feature in MyBatis that defers loading a referenced object until it’s explicitly accessed. However, excessive reliance on this feature can lead to performance issues due to repeated database hits.
How to Avoid It
Use lazy loading judiciously. When you're confident that a collection will always be accessed together, consider eager loading to reduce the number of queries executed. You can configure lazy loading as follows:
<settings>
<setting name="lazyLoadingEnabled" value="true"/>
<setting name="aggressiveLazyLoading" value="false"/>
</settings>
Remember, it’s a balance; use lazy loading where appropriate, but don’t hesitate to switch to eager loading when iterations are guaranteed.
Pitfall 5: Ignoring Best Practices for Caching
MyBatis supports caching at different levels. However, failing to configure caching appropriately may lead to stale data or increased latency.
How to Avoid It
Implement both level 1 (session) cache and level 2 (global) cache to optimize performance. You can enable caching in your mapper like this:
<mapper namespace="com.example.mappers.UserMapper">
<cache/>
<select id="selectUser" resultType="User">
SELECT * FROM users WHERE id = #{id}
</select>
</mapper>
This configuration enables caching for the UserMapper
, thus accelerating subsequent queries. It’s crucial to also handle cache invalidation correctly for data consistency.
Closing the Chapter
MyBatis can be a robust tool for managing database interactions when configured correctly. By understanding and avoiding common pitfalls—such as misconfiguring your DataSource, incorrect mapper scanning, poor SQL mapping, overusing lazy loading, and ignoring caching—you can ensure a smoother development process and maintain high performance.
For further reading on MyBatis configuration and best practices, consider visiting the official MyBatis documentation for comprehensive resources and detailed examples.
Whether you're a seasoned developer or just starting, keeping these considerations in mind will pave the way for effective MyBatis usage in your Java applications. Happy coding!