Overcoming Stateless Service Failures in OpenShift with Drools
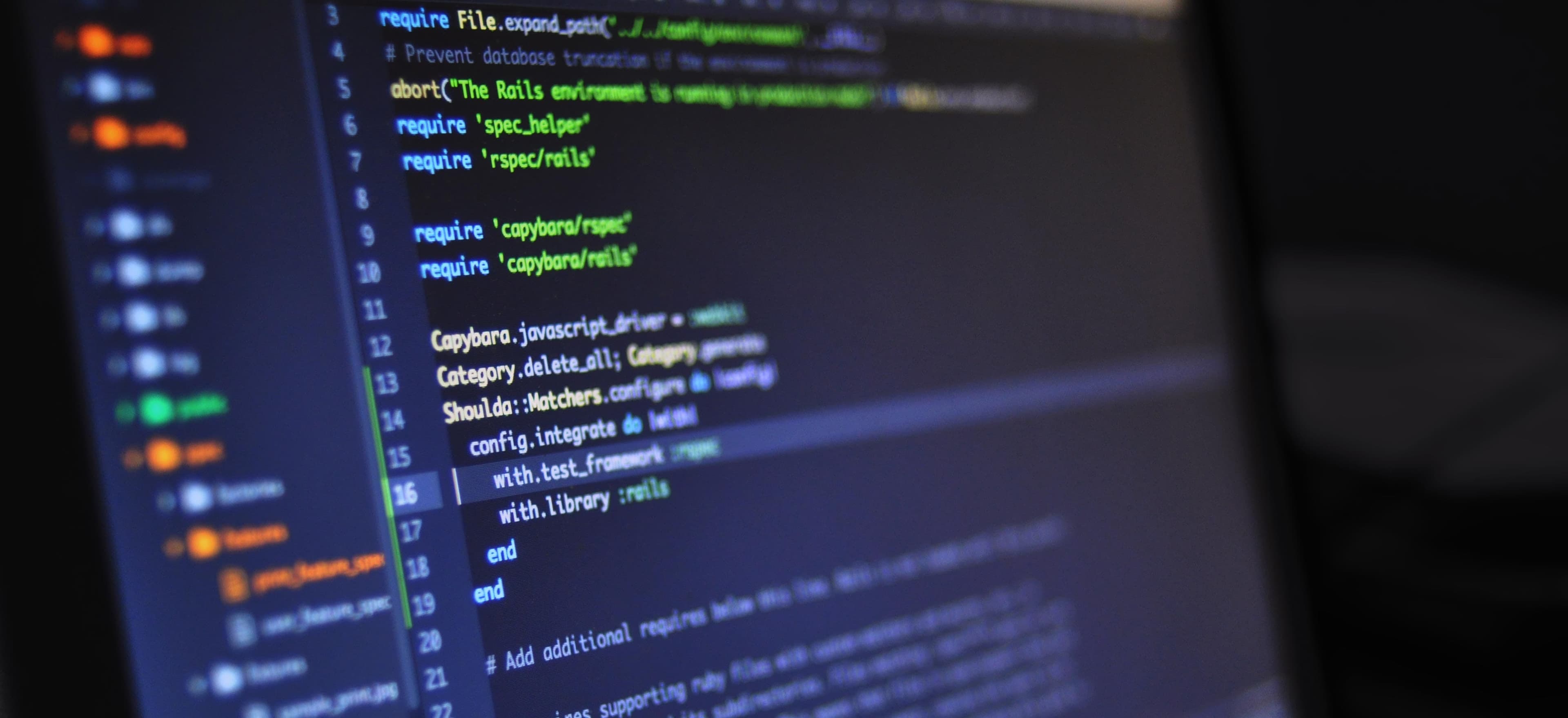
- Published on
Overcoming Stateless Service Failures in OpenShift with Drools
In today's cloud-native world, stateless services play an essential role in ensuring scalability and resilience. However, deploying these services in environments like OpenShift presents challenges, especially during failures. An intelligent way to manage these issues is by leveraging Drools, a powerful Business Rule Management System (BRMS). In this blog post, we will explore how to overcome stateless service failures in OpenShift using Drools, focusing on implementation, advantages, and best practices.
Understanding Stateless Services
Stateless services are those that do not persist state information between requests. Each request is treated independently, which allows for high levels of scalability. However, this approach also introduces complexities, especially when failures occur.
When a stateless service fails in OpenShift, the lack of stored contextual information can hinder recovery. For instance, if a request processing fails because of a business logic error, without the proper rules in place, it can fail silently or require manual intervention.
The Starting Line to Drools
Drools is an open-source rule engine that supports complex event processing and business rule management. It's particularly useful in scenarios where business logic needs to be decoupled from the application code.
By using Drools, developers can:
- Easily modify business rules without changing application code.
- Enhance maintainability and readability.
- Facilitate complex decision-making processes.
Drools Documentation offers in-depth guidance on how to get started.
Setting Up the Environment
Prerequisites
- OpenShift Cluster: Ensure you have access to an OpenShift cluster.
- Java Development Kit (JDK): Install JDK 8 or higher.
- Maven: Use Maven for dependency management.
Creating a Maven Project
First, create a basic Maven project structure. In your terminal, navigate to your desired directory and execute:
mvn archetype:generate -DgroupId=com.example.rules -DartifactId=stateless-service -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
This command generates a new Maven project with the group ID com.example.rules
and artifact ID stateless-service
.
Adding Drools Dependencies
Next, we need to add Drools dependencies to the pom.xml
file:
<dependencies>
<dependency>
<groupId>org.kie</groupId>
<artifactId>kie-api</artifactId>
<version>7.59.0.Final</version>
</dependency>
<dependency>
<groupId>org.kie</groupId>
<artifactId>drools-core</artifactId>
<version>7.59.0.Final</version>
</dependency>
</dependencies>
Developing a Rule
We will look at a simple rule that determines if a given order should be approved based on its value.
- Create a New Rule File
Create a file named OrderRules.drl in the src/main/resources
directory and add the following content:
package com.example.rules;
rule "Approve Order"
when
$order : Order(value > 1000)
then
$order.setApproved(true);
System.out.println("Order approved for value: " + $order.getValue());
end
- Why This Rule?
The above rule checks whether the order value exceeds 1000. If it does, the order is approved. This is a simple yet illustrative example to show how business logic can be externalized in Drools.
Implementing the Stateless Service
Now we will implement a basic stateless service using the Drools engine.
- Create a Java Class for the Service
Create a Java class named OrderService in the src/main/java/com/example/rules
directory.
package com.example.rules;
import org.kie.api.KieContainer;
import org.kie.api.KieServices;
import org.kie.api.runtime.KieSession;
public class OrderService {
public void processOrder(Order order) {
KieServices ks = KieServices.Factory.get();
KieContainer kContainer = ks.getKieClasspathContainer();
KieSession kSession = kContainer.newKieSession("ksession-rules");
kSession.insert(order);
kSession.fireAllRules();
kSession.dispose();
}
}
Explanation of the Implementation
- KieServices and KieContainer: These are used to access the rules defined in the
OrderRules.drl
file. - KieSession: Executes the rules against provided facts, in this case, the order being processed.
- fireAllRules(): Triggers evaluation of all rules based on the inserted facts.
Defining the Order Model
Next, we need an Order
class:
package com.example.rules;
public class Order {
private double value;
private boolean approved;
public Order(double value) {
this.value = value;
this.approved = false;
}
public double getValue() {
return value;
}
public void setApproved(boolean approved) {
this.approved = approved;
}
public boolean isApproved() {
return approved;
}
}
This class provides a simple model representing an order with a value and an approval state.
Deploying to OpenShift
Creating a Docker Image
To deploy your application, first, create a Docker image. Make sure you have a Dockerfile
in the project root:
FROM openjdk:8-jdk-alpine
COPY target/stateless-service-1.0-SNAPSHOT.jar app.jar
ENTRYPOINT ["java", "-jar", "/app.jar"]
Building the Docker Image
Run the following command to build your image:
mvn clean package
docker build -t stateless-service .
Pushing to OpenShift
- Login to OpenShift:
oc login <openshift-cluster-url>
- Create a New Project:
oc new-project stateless-service-project
- Deploy the Image:
oc new-app stateless-service
- Expose the Service:
oc expose svc/stateless-service
The application is now live on OpenShift!
Handling Failures with Drools
One of the significant challenges with stateless services is ensuring reliability during failures. Drools can help in creating fallback logic or complex decision flows that can mitigate service failures.
Example: Adding Recovery Logic
You can extend the rules engine to reprocess failing orders or log the errors without losing the state.
rule "Log Failed Orders"
when
$order : Order(approved == false)
then
System.out.println("Failed to approve order for value: " + $order.getValue());
end
This rule logs any order that could not be approved. You could pair this logic with external monitoring tools to alert you if a certain percentage of orders fail.
Wrapping Up
OpenShift and Drools together empower developers to create robust, stateless services that can gracefully handle failures. By externalizing business rules, you can improve maintainability, scalability, and decision-making processes.
In a modern cloud-native architecture, keeping services stateless and flexible using tools like Drools can ensure smooth operations and rapid response to failure scenarios. Implementing this structure requires upfront design but pays dividends in maintainability and performance.
For further reading on Java development with Drools, refer to Drools User Guide.
Feel free to comment below if you have questions or would like to share your experiences with stateless services and Drools in OpenShift!