Transitioning from Imperative to Declarative in Java
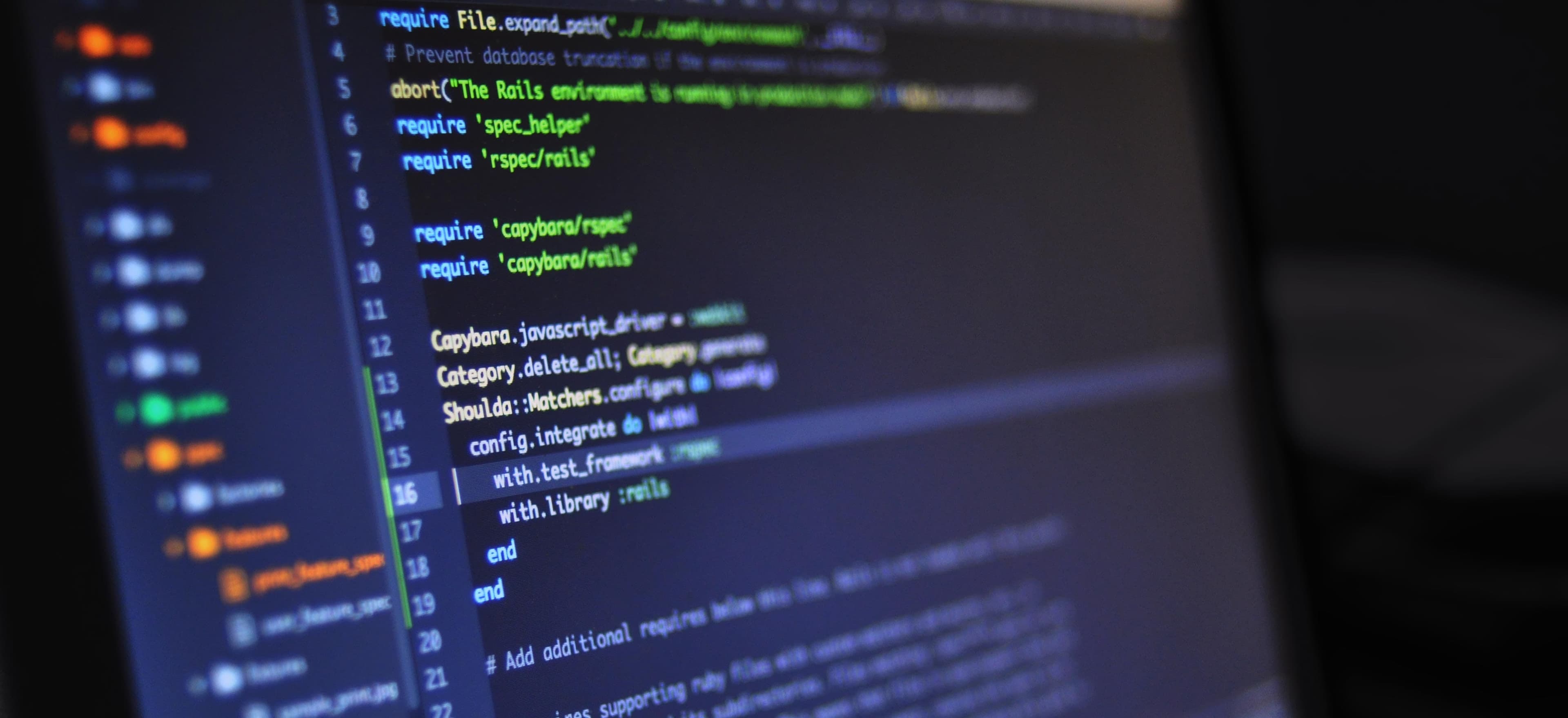
- Published on
Transitioning from Imperative to Declarative Programming in Java
Programming paradigms greatly influence how we think about and structure our code. Two of the most prominent paradigms are imperative and declarative programming. While the imperative approach describes how to perform tasks using step-by-step instructions, the declarative method emphasizes what the end result should be, allowing the underlying system to figure out the best way to achieve that result.
With the growing popularity of functional programming concepts, Java has begun embracing a more declarative style, particularly since the introduction of Java 8 and the Stream API. In this blog post, we will explore the transition from imperative to declarative programming in Java, discussing its benefits, key concepts, and practical examples to strengthen your understanding.
Why Transition to Declarative Programming?
-
Readability and Maintainability
- Declarative code often reads more like natural language, which can make it easier to understand.
- Reduces boilerplate code, leading to cleaner and more maintainable codebases.
-
Higher-Level Abstractions
- Allows developers to focus on the intent of the code rather than the intricacies of its implementation.
- Provides built-in functionalities that can optimize performance without manual tuning.
-
Enhanced Concurrency Support
- Declarative programming can simplify concurrent designs, as it abstracts away the complexity involved with managing state.
Example: Calculating the Sum of an Integer List
Let's look at a basic example of summing a list of integers using both imperative and declarative styles.
Imperative Approach
import java.util.List;
public class ImperativeExample {
public static void main(String[] args) {
List<Integer> numbers = List.of(1, 2, 3, 4, 5);
int sum = 0;
for (int number : numbers) {
sum += number; // Explicitly stating how to accumulate the sum
}
System.out.println("Sum: " + sum);
}
}
In the imperative approach, we explicitly specify how to iterate through the list and accumulate the sum. While this works, it can become unwieldy as complexity grows.
Declarative Approach
import java.util.List;
public class DeclarativeExample {
public static void main(String[] args) {
List<Integer> numbers = List.of(1, 2, 3, 4, 5);
int sum = numbers.stream().mapToInt(Integer::intValue).sum(); // Describing what we want
System.out.println("Sum: " + sum);
}
}
With the declarative approach, we express our intent with a high-level method stream()
, making the code cleaner and easier to follow. The use of mapToInt()
clearly indicates our intention to convert the list items and sum()
aggregates these values.
Key Concepts in Declarative Programming with Java
Understanding how to utilize Java’s Stream API effectively is essential for a smooth transition to declarative programming.
1. Stream API
The Stream API provides a powerful way to process sequences of elements (e.g., collections) in a functional style.
Example: Filtering Even Numbers
Imperative Approach
import java.util.ArrayList;
import java.util.List;
public class ImperativeFilter {
public static void main(String[] args) {
List<Integer> numbers = List.of(1, 2, 3, 4, 5);
List<Integer> evenNumbers = new ArrayList<>();
for (int number : numbers) {
if (number % 2 == 0) {
evenNumbers.add(number); // Explicitly handling the filter logic
}
}
System.out.println("Even Numbers: " + evenNumbers);
}
}
Declarative Approach
import java.util.List;
import java.util.stream.Collectors;
public class DeclarativeFilter {
public static void main(String[] args) {
List<Integer> numbers = List.of(1, 2, 3, 4, 5);
List<Integer> evenNumbers = numbers.stream()
.filter(number -> number % 2 == 0) // Clearly expressing the filter condition
.collect(Collectors.toList());
System.out.println("Even Numbers: " + evenNumbers);
}
}
In the declarative method, filter()
creates a natural expression of intent, showcasing how easily we can transform data without manual looping or intermediate storage.
2. Lazy Evaluation
Streams in Java utilize lazy evaluation: operations on a stream are not executed until a terminal operation (like collect()
, count()
, or forEach()
) is called.
3. Function References and Lambda Expressions
Java 8 introduced lambda expressions and function references, which are crucial for writing concise declarative code.
Example: Sorting with a Comparator
import java.util.Arrays;
import java.util.List;
public class DeclarativeSorting {
public static void main(String[] args) {
List<String> names = Arrays.asList("Charlie", "Alice", "Bob");
List<String> sortedNames = names.stream()
.sorted(String::compareToIgnoreCase) // Using method reference
.collect(Collectors.toList());
System.out.println("Sorted Names: " + sortedNames);
}
}
By utilizing sorted(String::compareToIgnoreCase)
, we reduce verbosity while enhancing readability.
Real-World Applications
The transition from imperative to declarative style is not just about syntax; it lays the foundation for elegantly addressing tasks such as:
-
Data Transformation: When working with large datasets, declarative programming allows you to transform data in complex ways without detailed procedural control.
-
Complex Filtering and Grouping: The capabilities of the Stream API enable intricate operations, such as grouping, joining, and partitioning collections with expressive methods.
-
Asynchronous Programming: Declarative styles lend themselves to more manageable asynchronous operations, especially with the use of
CompletableFuture
.
Example: Grouping by Year
import java.util.*;
import java.util.stream.Collectors;
class Event {
String name;
int year;
public Event(String name, int year) {
this.name = name;
this.year = year;
}
public int getYear() {
return year;
}
}
public class GroupingExample {
public static void main(String[] args) {
List<Event> events = List.of(
new Event("Event A", 2020),
new Event("Event B", 2021),
new Event("Event C", 2020)
);
Map<Integer, List<Event>> eventsGroupedByYear = events.stream()
.collect(Collectors.groupingBy(Event::getYear)); // Highlighting grouping capability
eventsGroupedByYear.forEach((year, eventList) ->
System.out.println(year + ": " + eventList.size() + " events")
);
}
}
Here, the collect(Collectors.groupingBy(Event::getYear))
succinctly indicates our goal of grouping events by their year.
The Bottom Line
Transitioning from imperative to declarative programming in Java can significantly enhance your development process by improving code readability, maintainability, and performance. By leveraging Java’s built-in functionalities like the Stream API and lambda expressions, you can write cleaner and more expressive code.
The shift may require a mindset adjustment, but the benefits of clarity and efficiency ultimately make the transition worthwhile. So why not start integrating these concepts into your Java programming today? Dive into the fascinating world of declarative programming and discover how it can transform your coding journey!
For more resources on Java programming, check out Oracle's Java Documentation and Functional Programming in Java.
Happy coding!
Checkout our other articles