When to Choose Soft Delete Over Hard Delete?
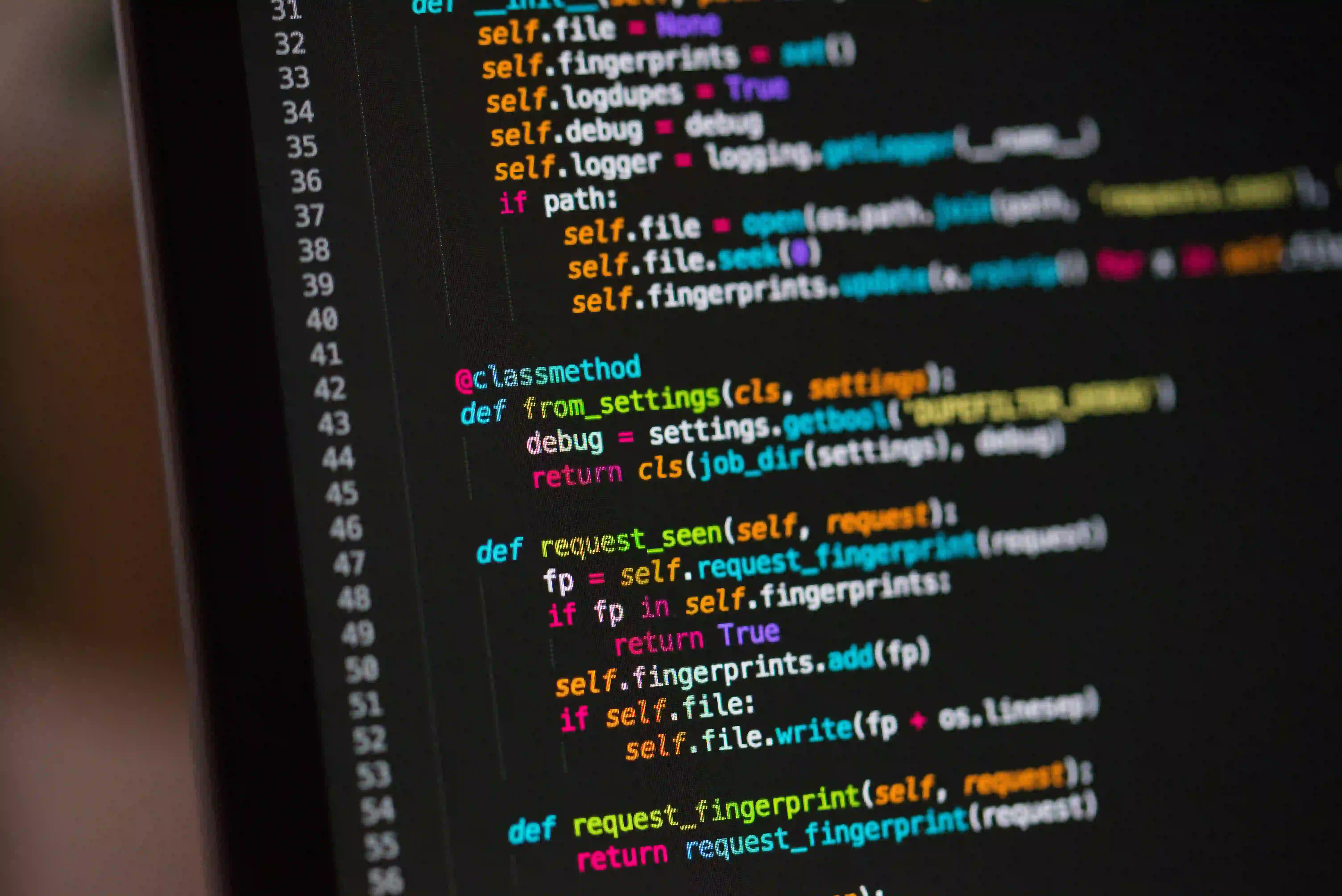
When to Choose Soft Delete Over Hard Delete in Database Design
In the world of software development, managing data efficiently is crucial. One fundamental aspect of data management lies in understanding the methods of deletion: soft deletes and hard deletes. In this blog post, we will explore these two approaches, discuss their benefits and drawbacks, and provide guidance on when to choose soft delete over hard delete.
Understanding Soft Delete and Hard Delete
Hard Delete
A hard delete permanently removes data from a database. Once executed, the deleted data cannot be recovered unless backed up. In SQL, a hard delete might look like this:
DELETE FROM users WHERE id = 1;
This command fully removes the user with an ID of 1 from the "users" table.
Soft Delete
In contrast, a soft delete marks a record as deleted without physically removing it from the database. Typically, this is achieved by adding a column to indicate whether a record is active or deleted. For example, you can add an is_deleted
boolean column to your table. When you soft-delete a record, you merely set this flag to true.
UPDATE users SET is_deleted = TRUE WHERE id = 1;
This update does not remove the record but instead flags it as deleted.
Pros and Cons of Each Approach
Understanding the advantages and disadvantages of these methods is essential for making an informed decision.
Pros of Hard Delete
- Space Efficiency: Hard deletes free up storage space, making the database more efficient.
- Simplicity: They simplify queries, as you do not need to filter out deleted records.
- Data Integrity: There are fewer chances for data inconsistencies if deleted records are completely removed.
Cons of Hard Delete
- Irrecoverability: Once deleted, the data cannot be recovered unless a backup exists. This poses a risk if deletions are made accidentally.
- Audit Limitations: You cannot track the history of changes or deletions in your data. This can be important for compliance in many businesses.
Pros of Soft Delete
- Irrecoverability: Easily recover deleted records, which is crucial for applications needing data retention.
- Audit Trails: It allows tracking of deletions, providing a history of the operations on records.
- User Experience: Users may expect deleted items can be recovered, especially in applications like email services or content management systems.
Cons of Soft Delete
- Data Bloat: Over time, soft deletes can lead to increased data volume, causing performance issues.
- Query Complexity: Every query must account for the soft delete flag, making SQL queries slightly more complex.
- Potential Inconsistencies: If developers forget to filter out soft-deleted records, it can lead to displaying stale data.
When to Choose Soft Delete Over Hard Delete
Making the decision between soft and hard deletion largely depends on the needs of your application. Here are key considerations that suggest opting for soft delete:
1. Data Recovery Needs
If your application requires the ability to recover deleted records due to user error or data loss, soft delete is the better choice. This is often a requirement for user-facing applications where users expect to retrieve deleted data.
2. Compliance and Audit Trails
Certain industries, particularly healthcare, finance, and e-commerce, are subject to regulatory frameworks requiring audit trails. Soft delete provides a straightforward way to maintain historical data while still respecting user privacy.
3. Logical Deletion
In scenarios where deletion is more about marking the data as no longer in use (as opposed to a true removal), soft deletes are favorable. For instance, in a task management app, marking a task as complete might simply require "deleting" it from the active view without truly removing it from the database for reporting purposes.
4. Feature Development
If there's a strong likelihood that features requiring swift restoration of deleted records will be developed in the future, implementing a soft delete mechanism from the beginning can save considerable time and resources later.
Implementation of Soft Delete in Java
Implementing a soft delete in Java can be done efficiently with frameworks like Hibernate, JPA, or even raw JDBC. Below is an example using JPA annotations:
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
// Other user properties
@Column(name = "is_deleted")
private boolean isDeleted = false;
// Getters and Setters
}
In the above code:
- The
User
class represents the user entity in your application. - The
isDeleted
boolean attribute is used to mark a user as soft-deleted.
Custom Repository Method for Soft Delete
To manage the soft deletion, you can extend the repository interfaces. Here is how you can implement it in a service:
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public void softDeleteUser(Long userId) {
User user = userRepository.findById(userId)
.orElseThrow(() -> new EntityNotFoundException("User not found"));
user.setDeleted(true);
userRepository.save(user);
}
}
In this service method:
- We retrieve the user by ID.
- If found, we set the user's
isDeleted
flag totrue
and save the changes.
Filter Soft Deleted Records on Retrieval
To ensure that only active users are retrieved, modify your repository method as follows:
public interface UserRepository extends JpaRepository<User, Long> {
@Query("SELECT u FROM User u WHERE u.isDeleted = false")
List<User> findAllActiveUsers();
}
This query filters out users that have been marked as deleted, ensuring your application logic maintains integrity.
A Final Look
In conclusion, the decision between soft delete and hard delete largely hinges on the specific requirements of the application and the broader context of data management practices. Soft deletes offer an excellent solution for applications needing recoverability, compliance, or logical deletion, while hard deletes can be suitable for simpler cases lacking these demands.
Choosing the right deletion strategy upfront can save valuable resources and enhance the user experience. If you're considering implementing soft deletion in your project, ensure that your data model, business logic, and database queries reflect this choice seamless.
For more on data management strategies, check out this resource on data retention best practices.
Key Takeaways
- Hard deletes are ideal for simplicity and space efficiency but come with risks of data loss.
- Soft deletes provide recoverability and audit trails but can lead to data bloat.
- The choice depends on specific application requirements and user expectations.
By recognizing the nuances of these deletion strategies, developers can create robust and user-friendly applications.