Overcoming Common Pitfalls When Writing Spark DataFrames to CSV
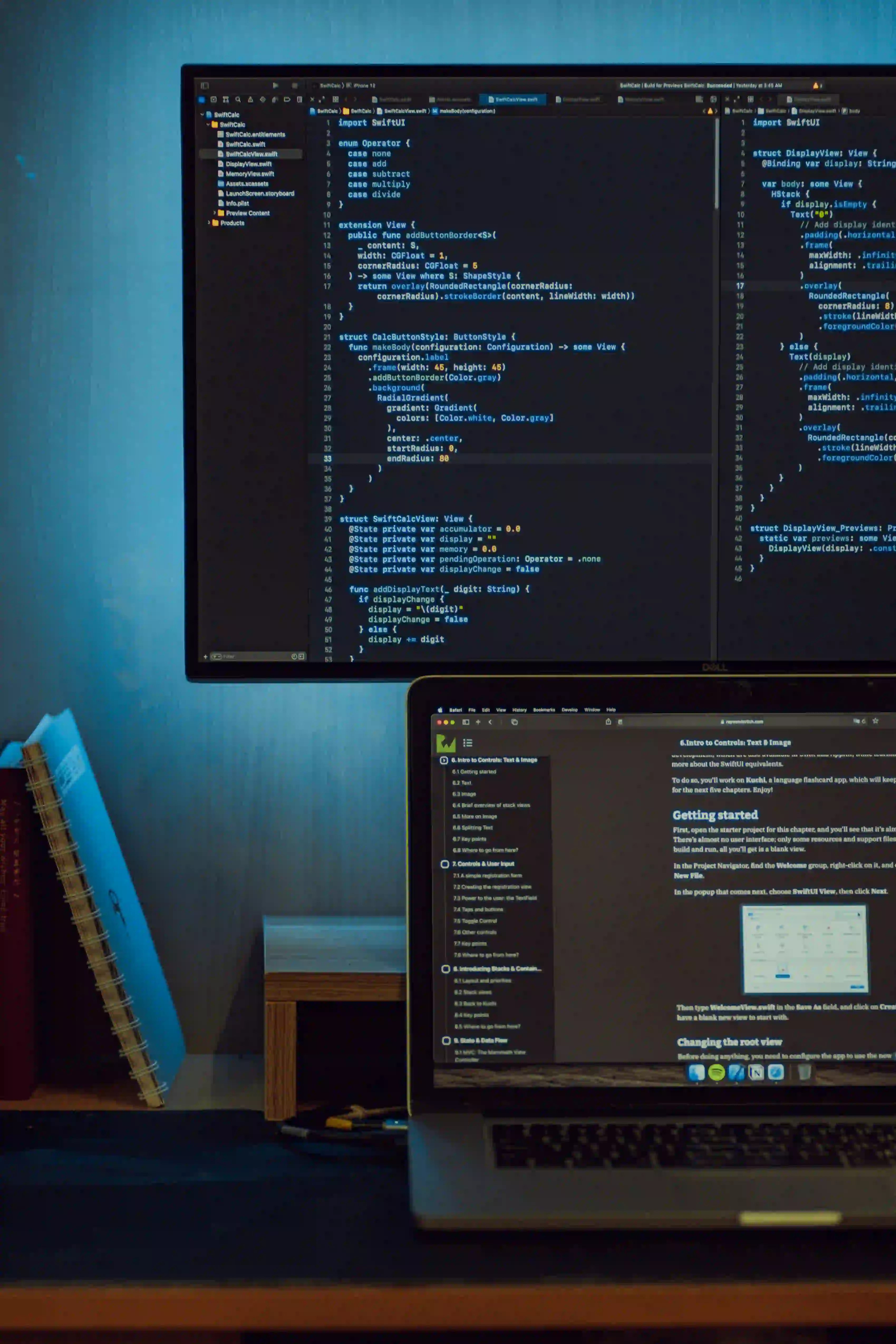
Overcoming Common Pitfalls When Writing Spark DataFrames to CSV
Apache Spark is a powerful and widely used tool for handling large-scale data processing. Its versatility allows developers to work with various data formats, including CSV, which is fundamental in data science and analytics. However, when writing Spark DataFrames to CSV files, there can be several pitfalls. In this blog post, we will explore common issues encountered during this process, along with best practices for overcoming them.
Understanding Spark DataFrames
Before we dive into the specifics, it’s essential to understand what a Spark DataFrame is. DataFrames in Spark are similar to tables in a database, providing a structured way to work with distributed data. They are optimized for both batch and streaming data processing, which makes them an excellent choice for big data applications.
Creating a Sample DataFrame
Let’s begin with a simple example of creating a Spark DataFrame:
import org.apache.spark.sql.Dataset;
import org.apache.spark.sql.Row;
import org.apache.spark.sql.SparkSession;
public class SparkDataFrameExample {
public static void main(String[] args) {
SparkSession spark = SparkSession.builder()
.appName("DataFrame Example")
.master("local")
.getOrCreate();
// Sample data
String data = "name,age,city\nAlice,29,New York\nBob,34,Los Angeles\nCharlie,25,Chicago";
// Creating DataFrame from CSV String
Dataset<Row> df = spark.read()
.option("header", "true")
.option("inferSchema", "true")
.csv("data.csv");
df.show();
spark.stop();
}
}
In this code, we create a Spark session and read a CSV into a DataFrame. The option("header", "true")
indicates that the first line of the CSV contains headers for the columns, and option("inferSchema", "true")
allows Spark to automatically infer the data types.
Common Pitfalls When Writing DataFrames to CSV
1. Improper File Path Handling
One of the first issues that developers often encounter is related to file paths. Specify a path that your Spark job has permissions to write to; otherwise, it will throw an I/O exception.
Best Practice
Always use absolute paths instead of relative paths. Here’s a code snippet showing this:
df.write()
.option("header", "true")
.csv("/absolute/path/to/output/directory");
Ensure that /absolute/path/to/output/directory
is accessible by your Spark application.
2. Data Overwrite Issues
When writing DataFrames to a CSV file, by default, Spark will throw an error if the target location already exists. This can be frustrating during iterative development.
Best Practice
Use .mode("overwrite")
to specify that you want Spark to overwrite any existing files in that directory. Here’s how to do this:
df.write()
.mode("overwrite")
.option("header", "true")
.csv("/absolute/path/to/output/directory");
Using the overwrite
mode allows for seamless updates to the CSV output, making it especially useful in iterative environments.
3. Not Specifying the Correct Delimiter
CSV files can be separated by various delimiters, with commas being the default. If your data contains commas, the file may not parse correctly without configuring a different delimiter.
Best Practice
Utilize the option("delimiter", ",")
argument to specify a delimiter that matches your data. For example, if using a tab as a delimiter, do the following:
df.write()
.option("header", "true")
.option("delimiter", "\t")
.csv("/absolute/path/to/output/directory");
4. Ignoring Character Encoding
Another common pitfall is not accounting for character encoding. If your DataFrame contains special characters, the default UTF-8 encoding might not be suitable, leading to corrupted files.
Best Practice
Specify the correct encoding using the option("charset", "UTF-8")
argument. Adjust the case as needed:
df.write()
.option("header", "true")
.option("charset", "ISO-8859-1")
.csv("/absolute/path/to/output/directory");
5. Not Flushing or Completing Write Operations
Finally, another potential issue is not ensuring file completion. Spark executes actions lazily. This means that, even if you write data, it may not necessarily finish writing it before the Spark application terminates.
Best Practice
Ensure that your application handles the completion of all write operations. It’s also good to add a count()
or collect()
operation afterward for confirmation:
long rowCount = df.count();
df.write()
.mode("overwrite")
.option("header", "true")
.csv("/absolute/path/to/output/directory");
System.out.println("Written " + rowCount + " rows to CSV file.");
Recommended Resources
For further reading, consider checking out the Apache Spark Documentation and Databricks Guide to Spark DataFrames. These resources provide comprehensive details about optimization and best practices for handling DataFrames.
Lessons Learned
Writing Spark DataFrames to CSV files doesn’t have to be a daunting task. By being mindful of the common pitfalls discussed in this post, such as file path handling, duplication issues, delimiter mismatches, encoding, and write operation management, you can streamline your data export process. Remember always to test your code and validate your outputs to ensure data quality and consistency.
In the world of big data, attention to detail matters. Utilize the best practices outlined in this blog post, and you’ll find your work with Spark and CSV files to be more efficient and successful. Happy coding!