Maximizing Efficiency: Common Pitfalls in Polyglot Persistence
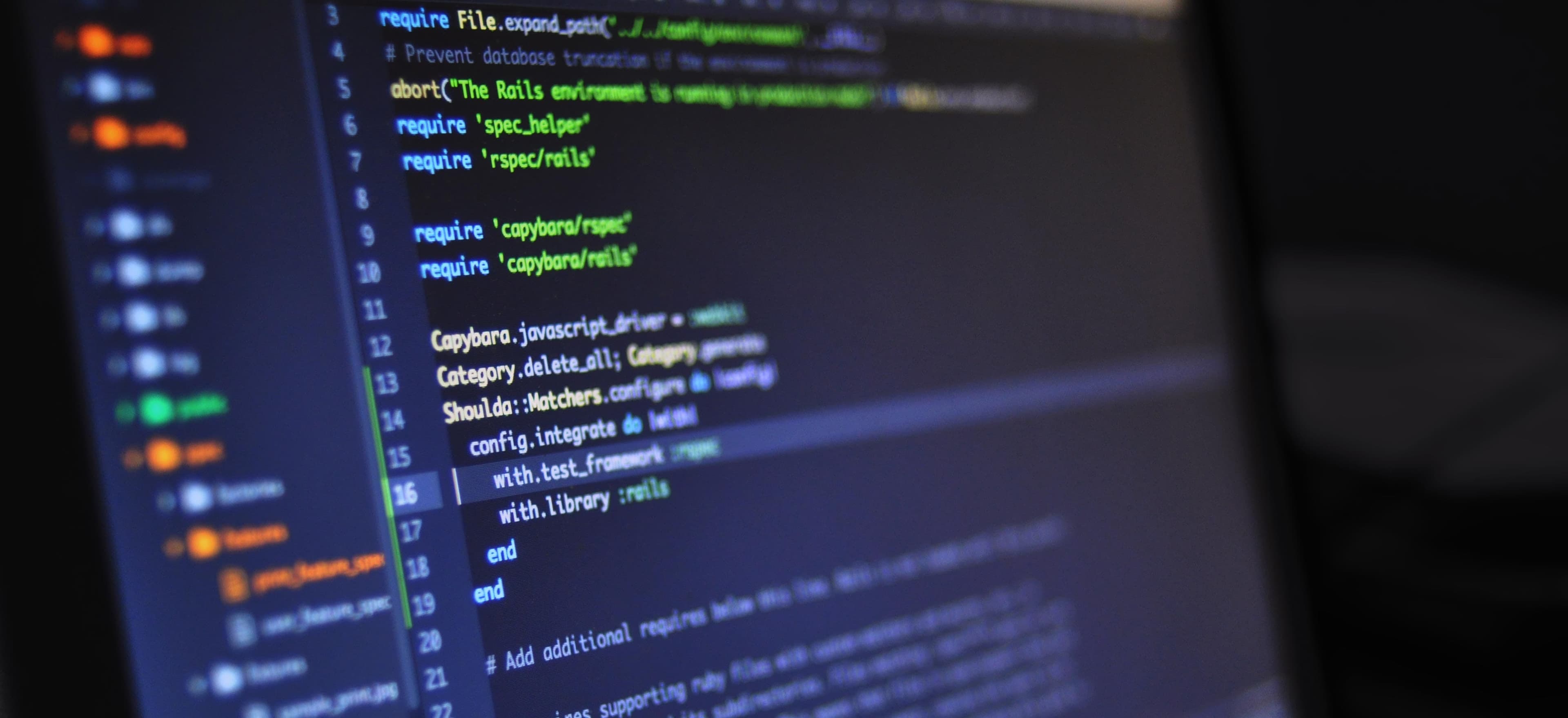
- Published on
Maximizing Efficiency: Common Pitfalls in Polyglot Persistence
In today’s fast-paced application development environment, polyglot persistence offers a powerful way to manage data using various storage technologies. This approach encompasses the use of multiple data storage technologies to handle varying data types and application needs. While polyglot persistence can lead to significant enhancements in performance and scalability, it also introduces complexity. This blog post will explore common pitfalls in polyglot persistence and how to avoid them, ensuring that you can maximize efficiency in your applications.
What is Polyglot Persistence?
Polyglot persistence is the combination of different data storage solutions within an application to leverage the strengths of each technology. For instance, you may choose to use a relational database for structured data, a NoSQL database for unstructured data, and an in-memory database for caching. This strategic use of technologies can provide optimized access patterns, increased speed, and enhanced scalability.
Advantages of Polyglot Persistence
Before diving into the common pitfalls, let's look at a few advantages of polyglot persistence:
- Scalability: Different databases can be scaled independently based on their usage patterns.
- Performance: Each database can be tuned for specific queries, leading to improved performance.
- Flexibility: Teams can choose the best data storage solution for their requirements without being locked into a single technology.
However, these advantages come with challenges. Let’s explore these pitfalls further.
Common Pitfalls in Polyglot Persistence
1. Over-Engineering
One of the most significant pitfalls of polyglot persistence is over-engineering. Teams can be tempted to use multiple storage technologies without a clear justification. For example:
// Sample code demonstrating over-engineering with unnecessary polyglot use
class UserService {
private UserRepository userRepository; // Relational DB
private SessionRepository sessionRepository; // NoSQL DB
public UserService(UserRepository userRepo, SessionRepository sessionRepo) {
this.userRepository = userRepo;
this.sessionRepository = sessionRepo;
}
public User getUser(String id) {
// Retrieve user from the relational database
return userRepository.findById(id);
}
public Session getSession(String id) {
// Retrieve session from NoSQL database
return sessionRepository.findById(id);
}
}
Why is this a problem? Over-engineering can lead to increased development and maintenance costs without any tangible benefit. It’s crucial to assess if the complexity added by integrating multiple databases is warranted by the application’s requirements.
Solution:
Before adopting a polyglot persistence strategy, conduct a thorough analysis to determine if it is necessary. If your use case can be satisfied with a single technology but still remains efficient, stick to it.
2. Data Consistency Challenges
Another prevailing issue in polyglot persistence is data consistency. When multiple databases are in use, maintaining consistent data across them can become challenging. If a user updates their profile information in a relational database, how do you ensure that any related data in the NoSQL database is also updated?
Consider this simplified scenario:
public void updateUserProfile(User user) {
userRepository.update(user); // Update in the relational DB
// Attempt to update related session data in NoSQL
sessionRepository.updateSessionForUser(user.getId(), user.getProfile());
}
Why is this problematic? If the update to the NoSQL database fails, you might end up with inconsistent data, which can lead to bugs and user dissatisfaction.
Solution:
Implement eventual consistency patterns and utilize distributed transactions only when necessary. This means that instead of ensuring synchronous updates, you accept that updates will propagate over time and can design your application to handle those eventual updates gracefully.
3. Incomplete or Insufficient Documentation
With multiple databases, the complexity of your system increases, and so does the necessity for thorough documentation. Insufficient documentation can lead to misunderstandings and mismanagement of data storage strategies.
Why is this a problem? Lack of documentation could result in disastrous mistakes such as:
- Incorrect database interactions.
- Unclear ownership of data responsibilities.
- Difficulty onboarding new developers.
Solution:
Create comprehensive documentation that outlines the purpose of each database, its schema, and how it interacts with other data stores. Additionally, keep an updated architecture diagram that clearly shows how the different components communicate.
4. Ignoring Performance Implications
Choosing the right database for the right task is fundamental, yet it's common for teams to overlook performance implications. Using multiple databases increases the cognitive load for developers as they must understand the performance characteristics of each database.
For example, if your relational database is performing slow joins, switching to a NoSQL database might be a tempting avoidance strategy. However, understanding how to optimize the relational database may be the better solution.
public List<Order> getOrdersByUserId(String userId) {
return orderRepository.findOrdersByUserId(userId);
// If performance issues arise, reevaluation of the database configuration may be necessary
}
Why is this an issue? Focusing on leveraging technology rather than improving the overall architecture may detract from the performance gains you are trying to achieve.
Solution:
Regularly conduct performance assessments and tuning of your databases. Utilize performance monitoring tools to capture data on queries, latency, and throughput. Understand all the options offered by the databases in use and regularly revisit your choices.
5. Lack of Unified Query Language
With polyglot persistence, you may end up with multiple query languages across your databases (SQL for relational databases, MongoDB query language for NoSQL, etc.). Maintaining consistency in handling data can be cumbersome.
Why is this important? Developers must switch contexts depending on which database they are working with, leading to inefficiencies in development time.
Solution:
Deploy an abstraction layer that standardizes queries across different data stores. This approach will not only ease the burden on developers but also enhance maintainability.
Key Takeaways
Polyglot persistence offers powerful advantages in flexibility and performance. However, it also introduces complexity that can hinder development and operational efficiency if not managed correctly. By avoiding common pitfalls—such as over-engineering, data consistency issues, lack of documentation, overlooking performance, and fragmented query languages—you can maximize the benefits of polyglot persistence.
For more context on effective data management strategies, you can explore Martin Fowler's articles on database patterns and polyglot persistence. Also, feel free to reference DZone’s guide for more insights into database technologies.
Navigating the landscape of polyglot persistence requires deliberation, strategy, and continuous learning. Embrace the challenge and reap the rewards of optimized data handling in your applications.
Checkout our other articles