Troubleshooting Java Integration with Dapr for Cloud Apps
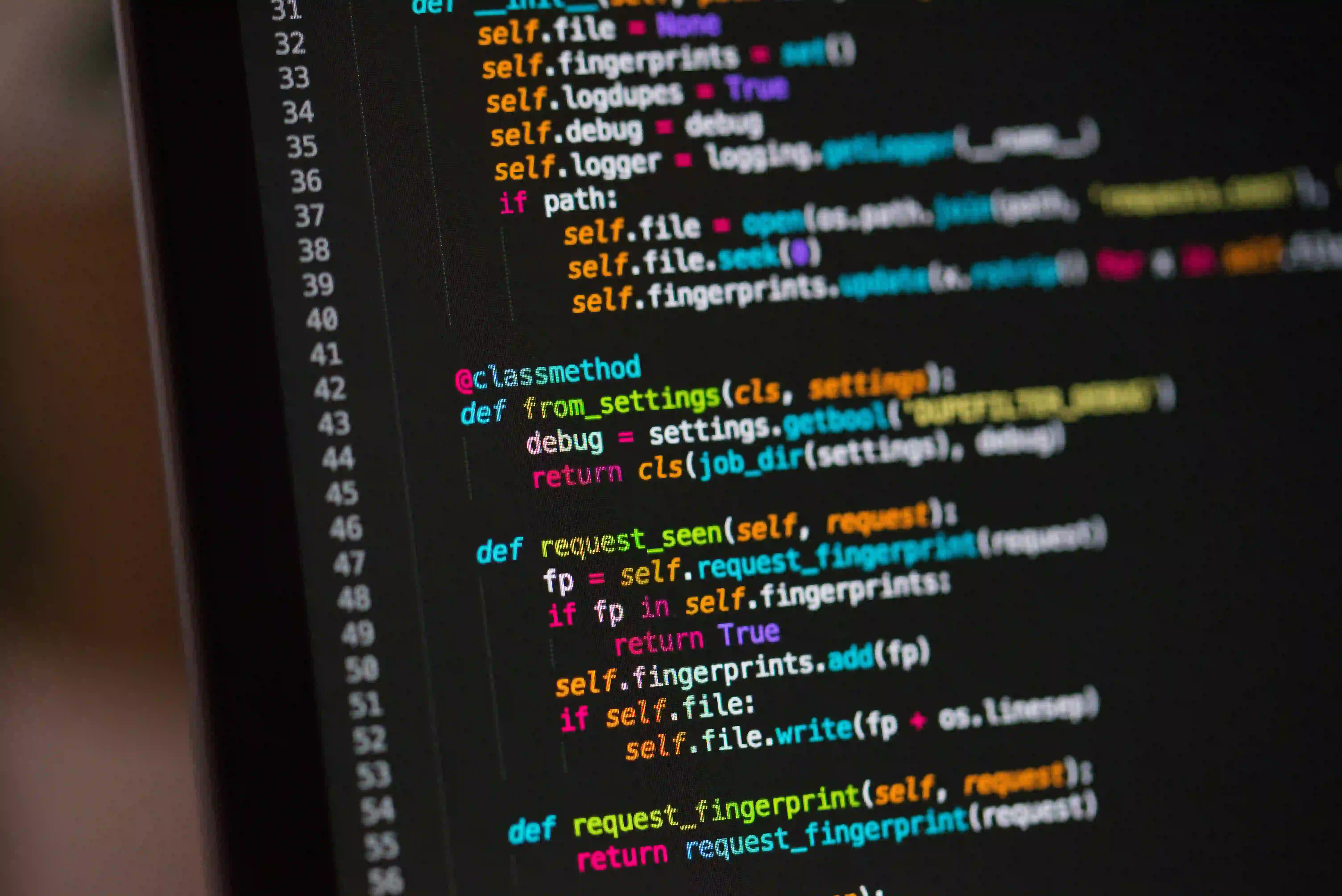
Troubleshooting Java Integration with Dapr for Cloud Apps
As cloud-native applications become increasingly popular, developers are often tasked with integrating various components seamlessly. Dapr (Distributed Application Runtime) is one such solution designed to simplify microservices architectures. In this blog post, we are going to delve into the integration of Dapr with Java applications, focusing on common issues and their resolutions.
What is Dapr?
Dapr is an open-source project that provides a set of building blocks for developing microservices. It allows you to focus on writing business logic instead of worrying about infrastructure concerns. Dapr simplifies service invocation, state management, pub/sub messaging, and more.
Key Features of Dapr:
- Programming Language Agnostic: Supports any language that can use HTTP or gRPC.
- Sidecar Architecture: Runs alongside your application, offering discovery, scaling, and resilience features.
- Components for Integration: Easy integration with cloud products and databases.
Dapr is increasingly becoming essential for developers working in cloud environments, especially those utilizing Java.
Setting Up Dapr with Java
Before we dive into troubleshooting, let's quickly go over how to set up a Java application with Dapr. When we use Spring Boot, for instance, the integration process is straightforward.
Step 1: Install Dapr CLI
To install the Dapr CLI, use the following command:
brew install dapr/tap/dapr
This command installs Dapr on macOS. Other operating systems have their installation procedures detailed in the Dapr documentation.
Step 2: Initialize Dapr
After installing the CLI, initialize Dapr and run the following command:
dapr init
This will set up Dapr with all the necessary components on your local machine.
Step 3: Create a Basic Java Application
You can create a simple Spring Boot application with Dapr dependencies. First, add the following dependency to your pom.xml
:
<dependency>
<groupId>io.dapr</groupId>
<artifactId>dapr-spring-boot-starter</artifactId>
<version>1.6.0</version> <!-- Check for the latest version -->
</dependency>
Next, you can create a sample controller to invoke a Dapr service.
@RestController
@RequestMapping("/api")
public class SampleController {
private final DaprClient daprClient;
@Autowired
public SampleController(DaprClient daprClient) {
this.daprClient = daprClient;
}
@GetMapping("/invoke")
public ResponseEntity<String> invokeService() {
String response = daprClient.invokeMethod("other-service", "methodName", HttpMethod.GET, null, String.class);
return ResponseEntity.ok(response);
}
}
This code snippet shows how to invoke another service using Dapr’s building blocks. Notice how we utilize DaprClient
to perform the service invocation.
Common Troubleshooting Scenarios
Even with an expected configuration, issues may arise during integration. Here are some of the most common scenarios developers face along with troubleshooting tips.
Scenario 1: Unable to Communicate Between Services
Symptoms: Services return connection refused errors or timeout responses.
Resolution:
-
Check Service Names: Ensure that the service name used in the invocation matches the name registered with Dapr. Verify your
dapr run
command to ensure it matches the service's name. -
Dapr Configuration: Confirm that the Dapr sidecar is running alongside your application. Run
dapr status
to check the status.🔧snippet.shdapr run --app-id myapp -- mvn spring-boot:run
-
Network Security: Check firewall and security groups, especially if you're in a cloud environment like AWS or Azure, to ensure that they allow communication over the required ports.
Scenario 2: Failed State Storage Operations
Symptoms: Error messages related to state retrieval or storage, such as "state store not found."
Resolution:
-
State Store Configuration: Ensure the state store component is correctly configured in your
components
directory. For Redis, for example, a sampleredis.yaml
might look like this:⚙️snippet.ymlapiVersion: dapr.io/v1alpha1 kind: Component metadata: name: statestore namespace: default spec: type: state.redis version: v1 metadata: - name: redisHost value: "localhost:6379"
-
Check Connectivity: Ensure your application can connect to the specified state store (e.g., Redis). You can use tools like
redis-cli
to verify connectivity.
Scenario 3: Issues with Pub/Sub Messaging
Symptoms: Messages not being sent or received.
Resolution:
-
Component Configuration: Make sure that your pub/sub component is properly defined. Using
pubsub.yaml
for RabbitMQ, for example:⚙️snippet.ymlapiVersion: dapr.io/v1alpha1 kind: Component metadata: name: pubsub namespace: default spec: type: pubsub.rabbitmq version: v1 metadata: - name: amqpURL value: "amqp://guest:guest@localhost:5672/" - name: queueName value: "messageQueue"
-
Subscriber Implementation: Ensure that your subscriber is correctly set up and matches the topic used in publishing:
☕snippet.java@PostMapping("/publish") public void publishMessage(@RequestBody String message) { daprClient.publishEvent("pubsub", "topicName", message); }
If none of these solutions work, verify your logs for potential clues on what might fail.
Performance Monitoring and Logging
Effective troubleshooting involves better monitoring and logging, which can considerably reduce the time spent on diagnosing issues:
- Use Dapr Dashboard: Dapr provides a dashboard to visualize services and track calls.
- Logging: Ensure you have proper logging in place (e.g., using SLF4J or Log4J) to gain insights during operation. Log the incoming requests and any response times for better diagnosis.
For more on Dapr observability, refer to the Dapr Observability Documentation.
Lessons Learned
Integrating Dapr with Java applications can greatly enhance your microservices architecture, but it is not without its challenges. By understanding the common issues and their solutions, you can leverage Dapr effectively in your cloud applications. Remember to keep your configurations consistent and your logs detailed for the best troubleshooting experience. As you develop, keep the documentation handy and don’t hesitate to explore additional resources to sharpen your Dapr skills.
Feel free to share your experiences or raise questions regarding any specific troubleshooting challenges you have encountered with Dapr and Java applications!