Overcoming Challenges with Serialized Names in Gson
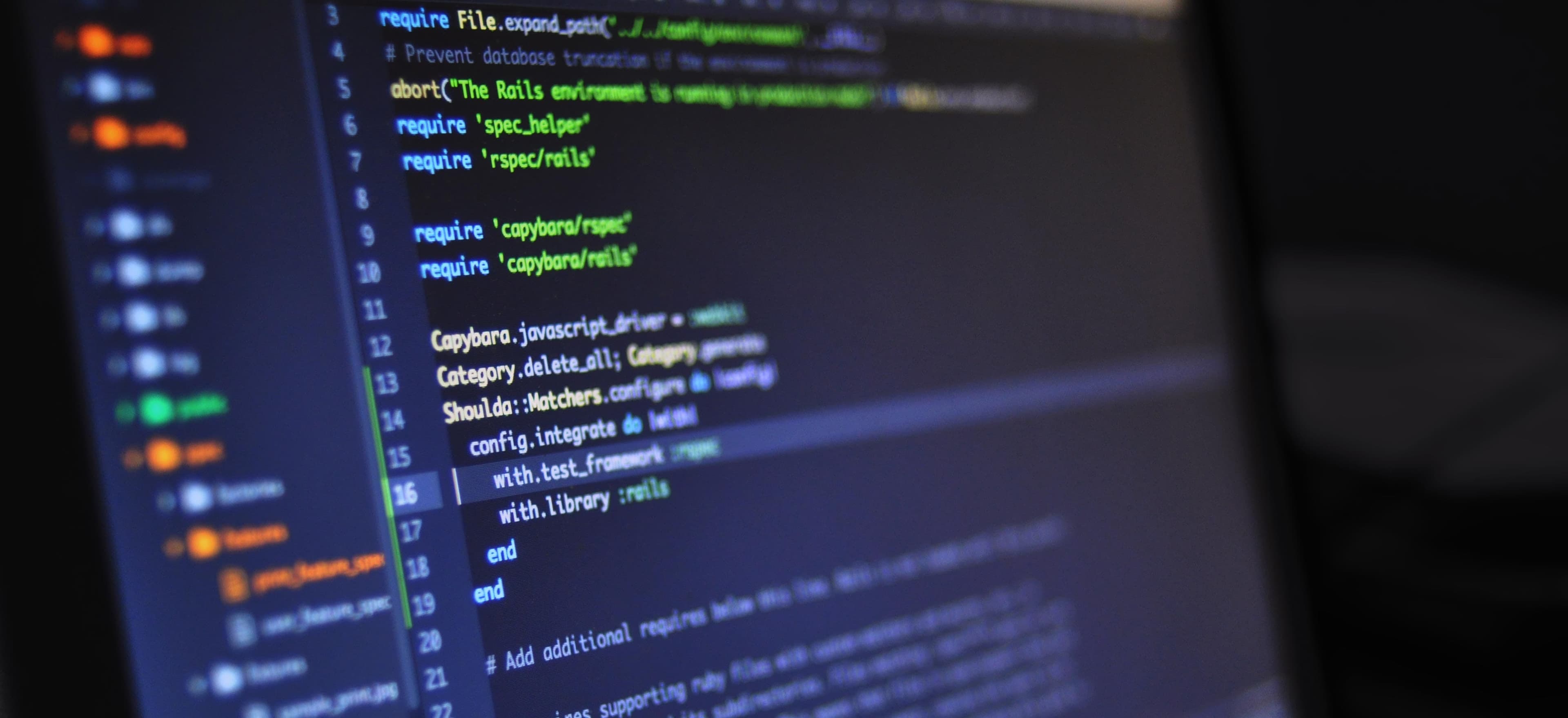
- Published on
Overcoming Challenges with Serialized Names in Gson
When working with Java and JSON, developers often turn to libraries that can handle the serialization and deserialization of complex data structures with ease. One such library is Gson, developed by Google. While Gson is robust and easy to use, developers might encounter challenges with serialized names, especially when the JSON structure differs from the Java class structure. In this blog post, we’ll discuss how to effectively handle serialized names in Gson, providing solutions to common problems and offering code snippets to illustrate key concepts.
Understanding Gson and Serialized Names
Gson is a powerful library that simplifies the process of converting Java objects to JSON and vice versa. It allows for customization of how Java fields are mapped to JSON properties using annotations. One such annotation is @SerializedName
, which is critical when the property names in your JSON do not match the field names in your Java class.
Consider the following scenario. You are fetching user data from an API, and the JSON response looks something like this:
{
"user_id": 1,
"full_name": "John Doe",
"email_address": "john.doe@example.com"
}
However, in your Java application, you might have a class that looks like this:
public class User {
private int id;
private String name;
private String email;
// Getters and Setters...
}
As we can see, there’s a mismatch between the JSON keys and Java fields. This is where @SerializedName
becomes extremely useful.
Using SerializedName to Prevent Errors
To address this discrepancy, you can use the @SerializedName
annotation to define how Gson should map the JSON fields to your Java class. Here’s how you can modify your User
class accordingly:
import com.google.gson.annotations.SerializedName;
public class User {
@SerializedName("user_id")
private int id;
@SerializedName("full_name")
private String name;
@SerializedName("email_address")
private String email;
// Getters and Setters...
}
In this example, each field in the User
class now has an associated @SerializedName
that links it to the corresponding JSON property.
Serialization and Deserialization
Now that we have set up our class with the correct annotations, let's move on to how we can serialize and deserialize JSON using Gson.
Deserializing JSON to Java Object
To convert JSON into a Java object, we can use Gson.fromJson()
. Here’s how you can achieve this:
import com.google.gson.Gson;
public class Main {
public static void main(String[] args) {
String json = "{\"user_id\": 1, \"full_name\": \"John Doe\", \"email_address\": \"john.doe@example.com\"}";
Gson gson = new Gson();
User user = gson.fromJson(json, User.class);
System.out.println("User ID: " + user.getId());
System.out.println("Name: " + user.getName());
System.out.println("Email: " + user.getEmail());
}
}
Output Interpretation
This code snippet will produce the following output, showing that the JSON has been successfully converted to a Java object.
User ID: 1
Name: John Doe
Email: john.doe@example.com
Serializing Java Object to JSON
Conversely, if you need to convert your User
object back into JSON, you can use the Gson.toJson()
method:
User user = new User();
user.setId(2);
user.setName("Jane Smith");
user.setEmail("jane.smith@example.com");
Gson gson = new Gson();
String jsonOutput = gson.toJson(user);
System.out.println(jsonOutput);
Expected Output
The output of this code will provide the following JSON string:
{"user_id":2,"full_name":"Jane Smith","email_address":"jane.smith@example.com"}
Handling Nested JSON Objects
In real-world applications, JSON structures can become significantly more complicated, often featuring nested objects. Let’s say our API response looks like this:
{
"user_id": 1,
"full_name": "John Doe",
"email_address": "john.doe@example.com",
"address": {
"street": "123 Main St",
"city": "New York",
"zip_code": "10001"
}
}
We can create an Address
class and link it to the User
class using the @SerializedName
annotation:
public class Address {
@SerializedName("street")
private String street;
@SerializedName("city")
private String city;
@SerializedName("zip_code")
private String zipCode;
// Getters and Setters...
}
public class User {
@SerializedName("user_id")
private int id;
@SerializedName("full_name")
private String name;
@SerializedName("email_address")
private String email;
@SerializedName("address")
private Address address;
// Getters and Setters...
}
My Closing Thoughts on the Matter
By employing the @SerializedName
annotation in Gson, developers can effectively handle discrepancies between Java class fields and JSON properties, as well as work with nested objects seamlessly. This kind of flexibility is invaluable in real-world applications where APIs can introduce unexpected challenges in data structure.
To further your understanding and explore more advanced features in Gson, you may refer to the official Gson documentation and Gson's GitHub repository.
Final Notes
In this blog post, we highlighted how to overcome challenges with serialized names in Gson. We provided a comprehensive overview on how to use @SerializedName
, showcased serialization and deserialization processes, and discussed nested JSON objects. By mastering these concepts, you can improve the robustness of your Java applications when interfacing with JSON data.
Checkout our other articles