Reviving Legacy Code: Challenges in Visitor Pattern Usage
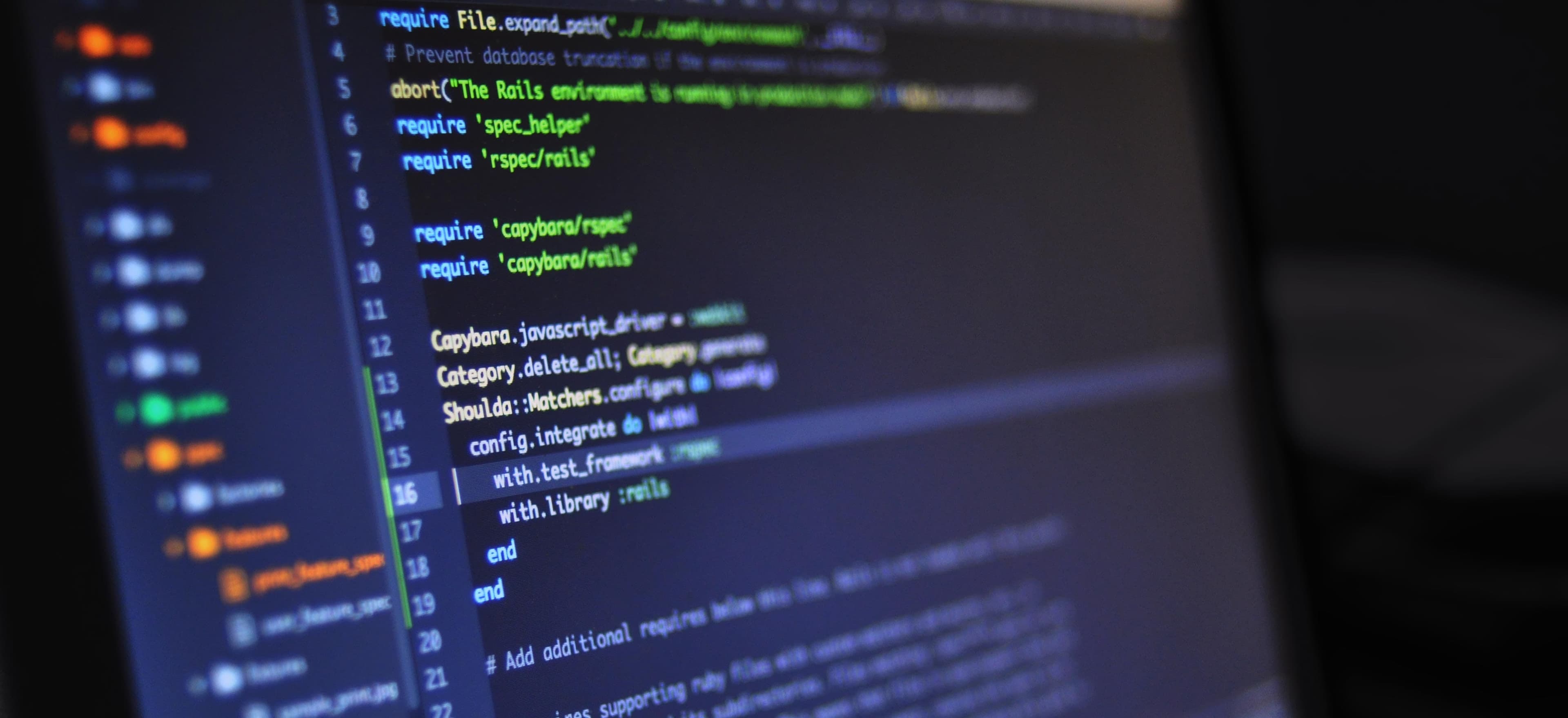
- Published on
Reviving Legacy Code: Challenges in Visitor Pattern Usage
When it comes to software development, maintaining and reviving legacy code is a daunting challenge. One design pattern that often finds its way into discussions about refactoring legacy systems is the Visitor Pattern. The Visitor Pattern can offer a systematic approach for executing operations across different classes without changing the classes themselves. However, using this pattern in legacy systems poses unique challenges. In this blog post, we'll explore these challenges and discuss strategies to effectively implement the Visitor Pattern in your legacy codebase.
Understanding the Visitor Pattern
Before diving into its challenges, let's recap what the Visitor Pattern really is. The Visitor Pattern allows you to add further operations to objects without modifying them. The idea is to define a new operation by creating a visitor class, which implements a specific interface.
Key Components of the Visitor Pattern
- Visitor interface: This defines a visit method for each element class.
- Element interface: This requires each concrete element class to accept a visitor.
- Concrete visitors and elements: These are the actual implementations of the visitor and element interfaces.
An Example of Visitor Pattern Implementation
Let's clarify the concept with a simple Java example:
// The Element interface
interface Element {
void accept(Visitor visitor);
}
// Concrete Elements
class Book implements Element {
private String title;
public Book(String title) {
this.title = title;
}
public String getTitle() {
return title;
}
public void accept(Visitor visitor) {
visitor.visit(this);
}
}
class Fruit implements Element {
private String name;
public Fruit(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void accept(Visitor visitor) {
visitor.visit(this);
}
}
// The Visitor interface
interface Visitor {
void visit(Book book);
void visit(Fruit fruit);
}
// Concrete Visitor
class PrintVisitor implements Visitor {
public void visit(Book book) {
System.out.println("Book Title: " + book.getTitle());
}
public void visit(Fruit fruit) {
System.out.println("Fruit Name: " + fruit.getName());
}
}
// Using the Visitor
public class Main {
public static void main(String[] args) {
Element[] elements = {new Book("1984"), new Fruit("Apple")};
Visitor visitor = new PrintVisitor();
for (Element element : elements) {
element.accept(visitor);
}
}
}
Commentary on the Code
In this code, the Visitor
interface separates the operations from the actual data structures (Book and Fruit). This means if we decide to create a new operation, we can do so by implementing a new Visitor without altering the Element
classes themselves. This makes it easy to introduce new functionalities.
However, there are several challenges when incorporating this pattern into legacy systems.
Challenges of Implementing the Visitor Pattern in Legacy Code
-
Tight Coupling: Legacy systems often possess tightly coupled components. Introducing the Visitor Pattern requires a restructuring of these components. For instance, all elements in your system must be modified to include the
accept
method, which can prove cumbersome. -
Existing Inheritance Structures: Legacy code may have established inheritance hierarchies. Introducing the Visitor Pattern may conflict with existing class structures, particularly if the classes are final or if you can't alter them.
-
Lack of Interfaces: In older systems, it is common to encounter code that does not utilize interfaces effectively. Since both the
Element
andVisitor
patterns rely heavily on well-defined interfaces, you may need to introduce them from scratch. -
Compiler and Runtime Overhead: Because the Visitor Pattern often adds additional layers of abstraction, it can introduce complexity. Performance issues may arise, especially in systems already strained by scalability issues.
-
Understanding Existing Functionality: Before implementing the Visitor Pattern, developers need a comprehensive understanding of the legacy codebase. This often requires time-consuming reverse engineering to figure out how everything interacts, increasing the risk of introducing bugs.
-
Refactoring Costs: Refactoring legacy systems to adhere to the Visitor Pattern implicates costs in terms of developer time and resources. Evaluating the ROI is necessary before deciding to adopt this approach.
Overcoming the Challenges
-
Incremental Refactoring: Instead of radically changing the entire system, apply the Visitor Pattern incrementally. Start with a few elements that are more manageable and gradually extend the pattern throughout your system.
-
Use Adapter Patterns: When multiple inheritance is a concern, consider using the Adapter Pattern to wrap existing classes, allowing you to implement the Visitor Pattern without invasive changes.
-
Implement Interfaces Gradually: Where legacy code lacks interfaces, introduce them gradually. This requires some upfront investment, but it will pay off by making your codebase more modular and easier to maintain.
-
Automated Testing: Introduce automated testing to ensure that existing functionalities remain intact post-refactoring. Unit tests can help catch regressions early on.
-
Documentation: As you untangle legacy code and implement the Visitor Pattern, invest time in documenting your changes. This will aid current and future developers who work on this code.
Lessons Learned
The Visitor Pattern presents a powerful method for extending functionalities in legacy code without the need for intrusive modifications. However, it comes with its set of challenges. By understanding these hurdles and employing systematic strategies, you can rejuvenate your legacy code while implementing the Visitor Pattern effectively.
In summary, the key to successfully reviving legacy code is a balanced approach—addressing immediate concerns while setting the groundwork for better architectural practices.
For more information on design patterns, check out the Gang of Four book or explore resources such as Refactoring Guru for deeper insights into the Visitor Pattern.
With these strategies in hand, you can simplify the path towards modernizing your legacy application, harnessing new capabilities without losing sight of its established foundations.
Checkout our other articles