Avoid These 5 Common Mistakes When Learning Java
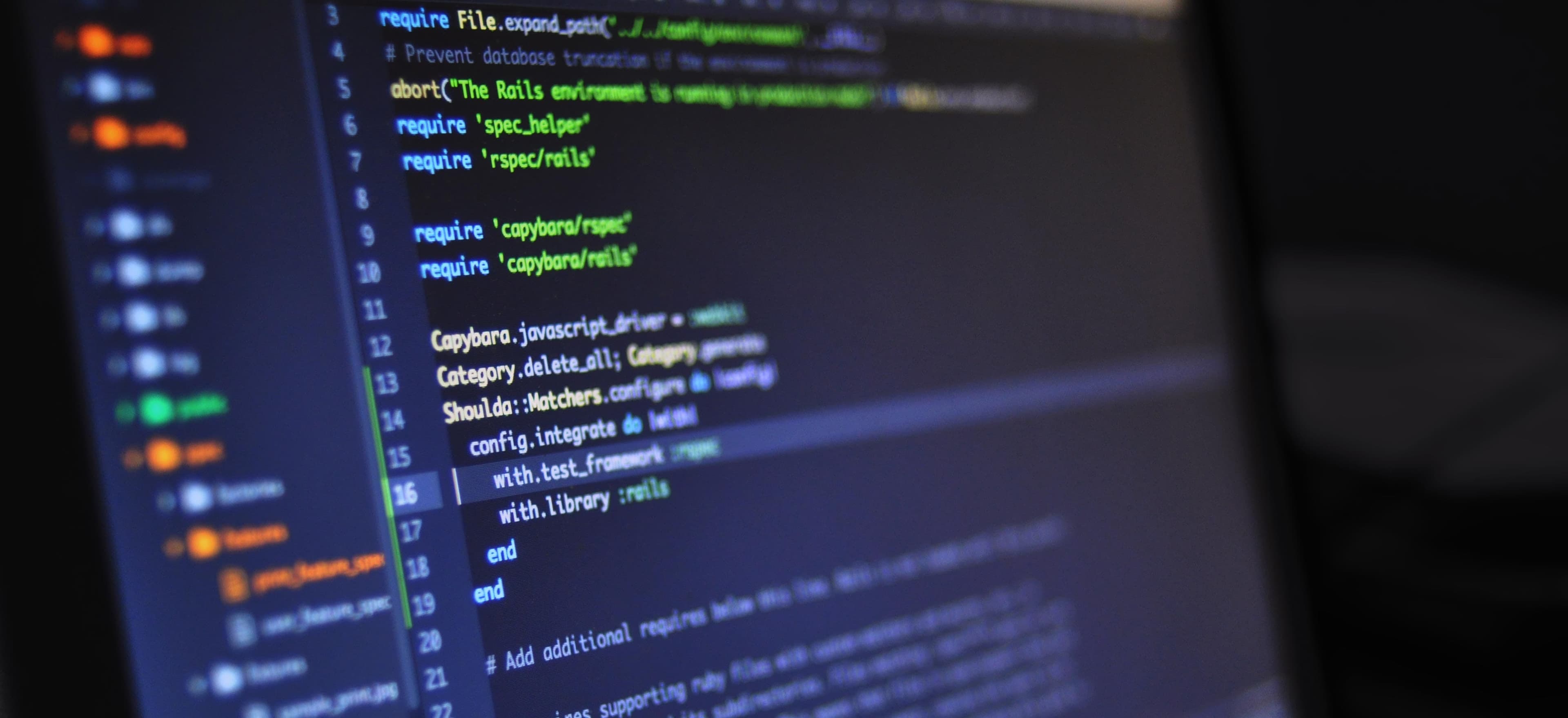
- Published on
Avoid These 5 Common Mistakes When Learning Java
Java is a powerful, versatile programming language used worldwide, but like any skill, mastering Java requires dedication and awareness of common pitfalls. With a global community driven by a passion for coding, navigating the complexities of Java can be daunting for beginners. This article outlines five common mistakes you should avoid when learning Java to ensure a smoother development journey.
1. Neglecting the Basics
Why Understand the Fundamentals?
Newcomers might rush to implement advanced concepts without a firm grasp of the basics. Understanding fundamentals such as data types, variables, loops, and conditional statements is essential. These core concepts form the foundation of Java programming and ultimately influence your ability to learn more complex topics like Object-Oriented Programming (OOP).
Example of Poor Understanding:
Here's a simple piece of code that demonstrates improper usage of data types.
public class Main {
public static void main(String[] args) {
int x = "Hello"; // Error: incompatible types
}
}
In this example, the mistake lies in trying to assign a string value to an integer variable. By grasping the basic types, you can avoid such errors. Take the time to study Java's primitive data types:
int
char
boolean
float
double
Familiarize yourself with how these types work, and your coding will improve exponentially.
2. Ignoring Object-Oriented Principles
The Importance of OOP
Java is built on the object-oriented programming paradigm, which emphasizes the concepts of encapsulation, inheritance, and polymorphism. Many beginners overlook these principles, neglecting their significance in writing efficient and maintainable code.
Example of Suboptimal Use:
Consider the following example, which fails to utilize OOP effectively:
public class Car {
String make;
String model;
void displayInfo() {
System.out.println("Make: " + make + ", Model: " + model);
}
}
// Usage
Car car1 = new Car();
car1.make = "Toyota";
car1.model = "Corolla";
car1.displayInfo();
While this code works, it lacks encapsulation. Encapsulation refers to bundling the data (attributes) and the methods (functions or procedures) that manipulate the data into a single unit. Here’s a revised version that follows OOP principles properly:
public class Car {
private String make;
private String model;
public Car(String make, String model) {
this.make = make;
this.model = model;
}
public void displayInfo() {
System.out.println("Make: " + make + ", Model: " + model);
}
}
// Usage
Car car1 = new Car("Toyota", "Corolla");
car1.displayInfo();
Why This Matters
By using constructors and encapsulation, we protect our data from unintended interference. Grasping these principles will prepare you for more advanced concepts and improve your problem-solving skills.
3. Overlooking Exception Handling
Understanding the Need for Error Management
Java provides a robust exception handling mechanism that is often overlooked by beginners. Neglecting to handle exceptions can lead to application crashes and poor user experience. Learning to use try
, catch
, and finally
blocks is crucial for creating resilient applications.
Example of Ineglecting Error Handling:
public class Division {
public static void main(String[] args) {
int numerator = 10;
int denominator = 0;
int result = numerator / denominator; // Causes ArithmeticException
}
}
In the above code, dividing by zero will throw an ArithmeticException
, causing the program to crash. Instead, you can handle the exception gracefully:
public class Division {
public static void main(String[] args) {
int numerator = 10;
int denominator = 0;
try {
int result = numerator / denominator; // May throw ArithmeticException
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
System.out.println("Error: Cannot divide by zero.");
}
}
}
Key Takeaway
By incorporating exception handling, you create fault-tolerant applications that enhance the user experience. Always remember to verify and validate input to prevent runtime errors.
4. Failing to Utilize Java Libraries and Frameworks
The Power of Java Ecosystem
Java has a rich ecosystem of libraries and frameworks — but many beginners choose to reinvent the wheel. Familiarity with popular libraries such as Java Standard Library, Apache Commons, and frameworks like Spring can drastically reduce development time and increase efficiency.
Example of Redundant Code:
Here’s why you might want to avoid overdoing it:
public class StringUtils {
public static String reverse(String input) {
String result = "";
for (int i = input.length() - 1; i >= 0; i--) {
result += input.charAt(i);
}
return result;
}
}
// Usage
String reversed = StringUtils.reverse("Java");
While this custom implementation works, Java's standard library offers a more concise way. Consider using StringBuilder
:
public class Main {
public static void main(String[] args) {
String original = "Java";
String reversed = new StringBuilder(original).reverse().toString();
System.out.println(reversed);
}
}
Why Leverage Libraries?
Utilizing existing libraries not only saves time but also ensures the reliability and optimization of your code. Familiarize yourself with Maven for dependency management and learn about Java libraries to maximize your coding efficiency.
5. Inadequate Practice and Project Application
The Necessity of Hands-On Practice
Finally, one of the most common mistakes is inadequate practice and failure to work on real-world projects. Reading books and tutorials alone are not enough. Active coding and project building solidify your understanding of Java concepts.
Getting Started with Projects:
Start small. Consider building:
- A simple game (like Tic-Tac-Toe)
- A personal finance tracker
- A to-do list application
Walkthrough of a Simple Project
To walk you through a simple project idea, let's code a basic console-based calculator:
import java.util.Scanner;
public class Calculator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter first number:");
double num1 = scanner.nextDouble();
System.out.println("Enter second number:");
double num2 = scanner.nextDouble();
System.out.println("Choose an operation (+, -, *, /):");
char operation = scanner.next().charAt(0);
double result;
switch (operation) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
if (num2 != 0) {
result = num1 / num2;
} else {
System.out.println("Error: Cannot divide by zero!");
return;
}
break;
default:
System.out.println("Invalid operation!");
return;
}
System.out.println("Result: " + result);
}
}
Closing Remarks
Writing a simple calculator project allows you to explore various Java elements, such as conditional statements, loops, and user input. This practical experience solidifies your learning and enhances your confidence in navigating Java's ecosystem.
By avoiding these five common mistakes — neglecting the basics, overlooking OOP principles, ignoring exception handling, failing to utilize libraries, and lacking hands-on practice — you're setting the stage for a rewarding learning experience in Java.
Empower yourself, immerse in the community, and develop your skills. Happy coding!
Further Reading
- Java Fundamentals
- Understanding OOP in Java
- Java Exception Handling
- Java Libraries and Frameworks
For more tips and resources, check out Java Programming on Stack Overflow.