Common Pitfalls in Java Microservices Development
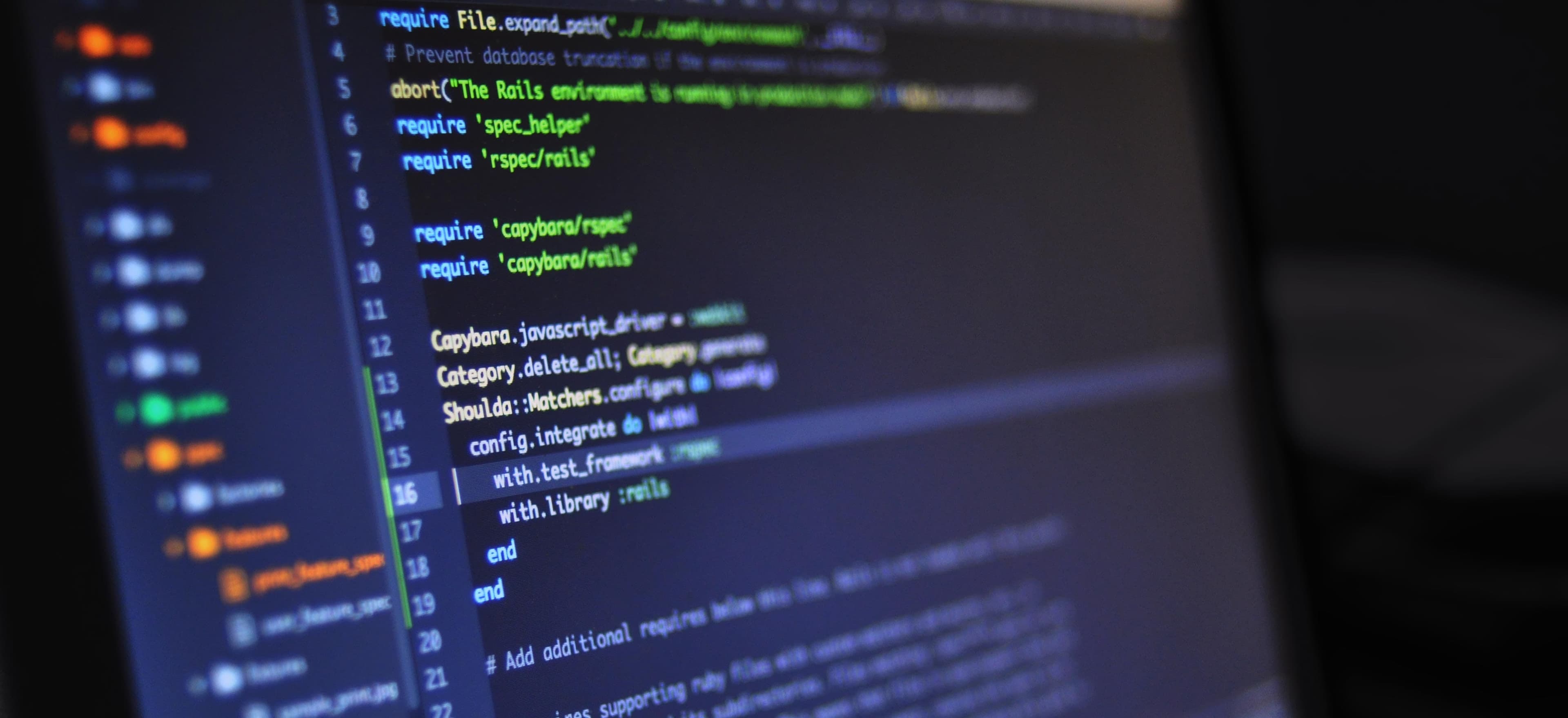
- Published on
Common Pitfalls in Java Microservices Development
Microservices architecture has gained immense popularity in recent years, especially in the Java ecosystem. With its promises of scalability, flexibility, and independent deployability, it has become the go-to model for many enterprises. However, along with these advantages come several potential pitfalls that developers must navigate. This article will discuss these common pitfalls and present strategies to avoid them, ensuring a smoother microservices development experience in Java.
1. Over-Engineering Microservices
When transitioning from a monolithic application to microservices, it's tempting to create several fine-grained services. However, over-engineering can lead to a bloated architecture that is challenging to manage and maintain.
Why this is a Pitfall
Microservices should serve well-defined business capabilities. Breaking down services too finely can result in excessive inter-service communication, increased latency, and complex orchestration.
Solution
Start with a few well-defined services, and grow the architecture over time. Follow the Single Responsibility Principle (SRP) to ensure that each microservice concentrates on a single business function.
public class UserService {
public User getUserById(String userId) {
// Logic to retrieve user by ID
}
}
// Avoid creating services that do unrelated tasks
public class UserService {
// Violation of SRP: This service attempts to handle user-related logic
// AND account settings logic
}
2. Ignoring API Versioning
As your microservices evolve, maintaining backward compatibility with clients can become challenging. Ignoring API versioning can lead to broken clients when updates are made.
Why this is a Pitfall
When you make changes to an API that existing clients depend on, those clients may fail to function, leading to considerable downtime and frustration.
Solution
Implement API versioning from the start. There are several strategies to achieve this, such as URL versioning or using headers.
URL Versioning Example
@RestController
@RequestMapping("/api/v1/users")
public class UserController {
@GetMapping("/{userId}")
public User getUser(@PathVariable String userId) {
// Returns user data
}
}
// Maintain a separate route for v2
@RestController
@RequestMapping("/api/v2/users")
public class UserV2Controller {
@GetMapping("/{userId}")
public User getUserV2(@PathVariable String userId) {
// Returns user data with updated structure
}
}
3. Lack of Centralized Logging
In a microservices environment, each service may run independently, making tracking down issues difficult. Lack of centralized logging can turn small issues into major headaches.
Why this is a Pitfall
Without centralized logging, developers may struggle to identify the root cause of issues, leading to wasted time and ineffective troubleshooting.
Solution
Use tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Splunk for centralized logging. This allows you to monitor and trace requests across services seamlessly.
# Example Logstash configuration to aggregate logs
input {
file {
path => "/var/log/myapp/*.log"
start_position => "beginning"
}
}
filter {
# Include any filtering logic here
}
output {
elasticsearch {
hosts => ["http://localhost:9200"]
index => "microservices-logs"
}
}
4. Not Designing for Failure
Microservices are inherently more complex than monolithic applications, and network issues can easily cause failures. Not considering failure scenarios can lead to cascading failures across the system.
Why this is a Pitfall
A single service failure can bring down the whole application if not mitigated. Re-establishing service availability can involve significant time and effort.
Solution
Implement patterns like Circuit Breaker and Retry mechanisms to handle service failures gracefully. Libraries like Hystrix or Resilience4j can help in implementing these patterns.
@CircuitBreaker
public User getUser(String userId) {
// Attempt to fetch user data
// If failed, the circuit will open, preventing further calls
}
5. Inefficient Data Management
In a microservices architecture, managing data can be complicated. Each service could manage its own data, which can lead to data inconsistency if not handled correctly.
Why this is a Pitfall
Avoiding data consistency issues while enforcing domain boundaries can lead to either duplicate efforts or complex transactional management.
Solution
Consider using the Database per Service pattern to isolate the data. Additionally, you can implement an event sourcing or CQRS (Command Query Responsibility Segregation) approach to maintain consistency.
// Sample CQRS design
public class UserCommandService {
public void registerUser(User user) {
// Execute write action here
}
}
public class UserQueryService {
public User getUser(String userId) {
// Execute read action here
}
}
6. Poor Monitoring and Observability
Microservices can create a "black box" effect, where you cannot see how well services are performing or if they are healthy. Poor monitoring and observability can obscure issues until they escalate.
Why this is a Pitfall
Without proper monitoring, problems can linger until they severely impact users, leading to loss of trust in your system.
Solution
Incorporate monitoring tools like Prometheus, Grafana, or Spring Boot Actuator. Use distributed tracing with tools like Zipkin or Jaeger to trace requests across multiple services.
@EnableActuator
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Lessons Learned
Microservices development in Java offers numerous benefits, but it also presents unique challenges. By identifying and addressing common pitfalls such as over-engineering, ignoring versioning, lack of logging, failure design, data management issues, and poor observability, developers can foster a more robust architecture.
Microservices should enhance your development capabilities, not complicate them. As you embark on your microservices journey, keep these pitfalls in mind to build a resilient, efficient, and scalable system.
Now it is your turn! Share your experiences, challenges, and solutions when working with microservices in Java. The discussion can help many others in the community to avoid the common pitfalls we've discussed.
For further reading, check out these resources:
- Spring Cloud - Building Microservices
- Understanding Microservices: Key Concepts to Watch
- Microservices Patterns: With examples in Java