Handling Spring Custom Namespaces: Common Issues and Fixes
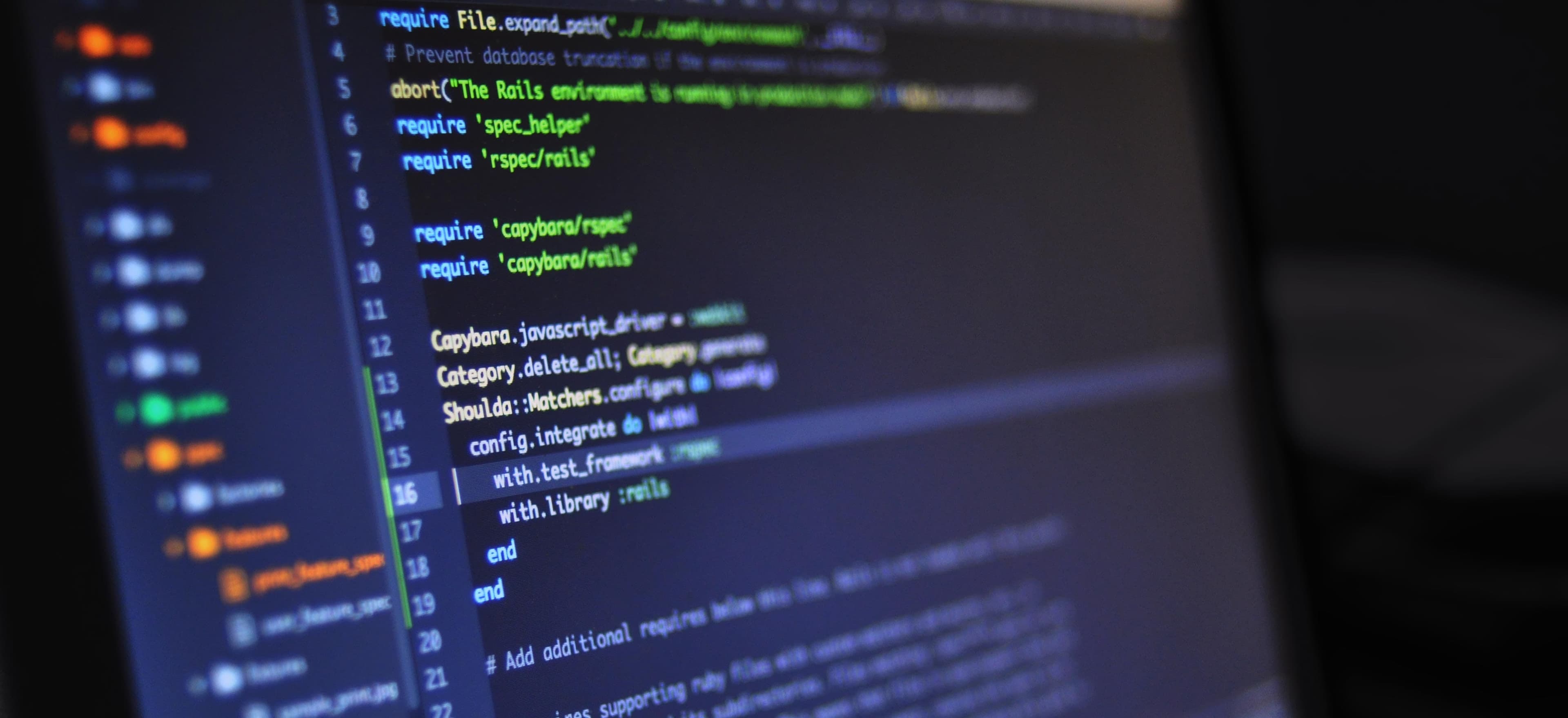
- Published on
Handling Spring Custom Namespaces: Common Issues and Fixes
Spring framework is a robust and versatile ecosystem that can be extended to meet various application needs. One such extension is through the use of custom namespaces in XML configuration. While they provide a powerful way to enhance configuration readability, developers often encounter certain common issues that can hinder their implementation. In this blog post, we will explore these common issues and the corresponding fixes.
What Are Spring Custom Namespaces?
Spring custom namespaces allow developers to define their custom XML tags that encapsulate specific functionalities and configurations. This not only makes the XML configuration cleaner but also enhances code readability and usability. Custom namespaces are particularly advantageous for libraries, where complex configurations might be needed.
For instance, instead of writing long, repetitive configurations, you can create a custom namespace with a concise syntax.
Example Custom Namespace
Here is a simplified example of a custom namespace definition:
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:custom="http://www.example.com/schema/custom"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.example.com/schema/custom
http://www.example.com/schema/custom.xsd">
<custom:myBean id="myUniqueBean" property1="value1" property2="value2"/>
</beans>
In this example, custom:myBean
is defined within the custom namespace custom
that you would implement yourself.
Common Issues with Custom Namespaces
While working with custom namespaces can be beneficial, several common issues may arise:
1. Schema Validation Errors
Issue Explanation: Schema validation errors occur when Spring is unable to locate the XSD file associated with your custom namespace. This often results in beans not being recognized.
Fix: Make sure that the schemaLocation is correctly declared. For example:
xsi:schemaLocation="http://www.example.com/schema/custom
http://www.example.com/schema/custom.xsd"
- Ensure the XSD file is accessible at the provided URL.
- Validate your path and correct any typos or incorrect URLs.
2. Mismatched Element Names
Issue Explanation: A frequent issue is using incorrect element names or not defining them correctly in the XSD.
Fix: Double-check your XSD definitions to ensure that the names match those used in your XML.
Here’s an example of what your XSD might look like:
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema"
targetNamespace="http://www.example.com/schema/custom"
xmlns="http://www.example.com/schema/custom"
elementFormDefault="qualified">
<xs:element name="myBean">
<xs:complexType>
<xs:attribute name="property1" type="xs:string" use="required"/>
<xs:attribute name="property2" type="xs:string" use="optional"/>
</xs:complexType>
</xs:element>
</xs:schema>
3. Class Not Found Exceptions
Issue Explanation: If your custom namespace is meant to instantiate specific beans, a "class not found" exception may arise if the class definition is omitted or not included in the build path.
Fix:
Ensure that all required classes are included in your project’s dependencies. For Gradle, add the necessary libraries in your build.gradle
file:
dependencies {
implementation 'org.springframework:spring-context:5.3.9'
}
For Maven, make sure your pom.xml
has the corresponding dependency:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.3.9</version>
</dependency>
4. Bean Instantiation Issues
Issue Explanation: Sometimes, you may define beans in your custom namespace but face issues during the instantiation, leading to runtime exceptions.
Fix: This usually happens because of incorrect argument definition in the constructor or setters.
Here's an example of proper bean definition:
<custom:myBean id="exampleBean" property1="exampleValue" property2="anotherValue"/>
Make sure your Java class has a corresponding constructor and setters:
public class MyBean {
private String property1;
private String property2;
public MyBean(String property1) {
this.property1 = property1;
}
// Setters...
public void setProperty2(String property2) {
this.property2 = property2;
}
}
5. Confusing Namespace Prefixes
Issue Explanation: In a large XML configuration, using abbreviations or prefixes that are too similar can lead to drag-and-drop compatibility issues.
Fix: Distinct and descriptive prefixes can significantly improve the readability of your XML. Keep it simple but precise:
xmlns:myCustom="http://www.example.com/schema/myCustom"
6. Documentation and Self-Contained Examples
When you are developing your custom namespace, ensure that proper documentation and examples of usage are available. This can dramatically reduce onboarding time for new developers in your project.
Finite Resource Allocation with Examples
Suppose you're creating a simple custom namespace for a configuration loader. By structuring both your namespace and documentation properly, new developers can adopt your custom namespace easily.
The Closing Argument
Utilizing custom namespaces in Spring can tidy up configuration files and enhance their functionality, but you may encounter some common issues along the way. By understanding these pitfalls and the suggested fixes, you can navigate these challenges more effectively.
For additional reading on Spring customization, consider visiting the Spring Framework documentation. Engaging with community forums such as Stack Overflow may also provide insights into better practices and solutions tailored to real-world challenges.
Whether you are a seasoned Spring developer or just starting, mastering these insights will enable you to build more reliable and maintainable Spring applications. With practice, handling Spring custom namespaces will become second nature, leading to cleaner configurations and less frustrating debugging. Happy coding!
Checkout our other articles