Avoiding Common Tech Mistakes That Cost You Time and Money
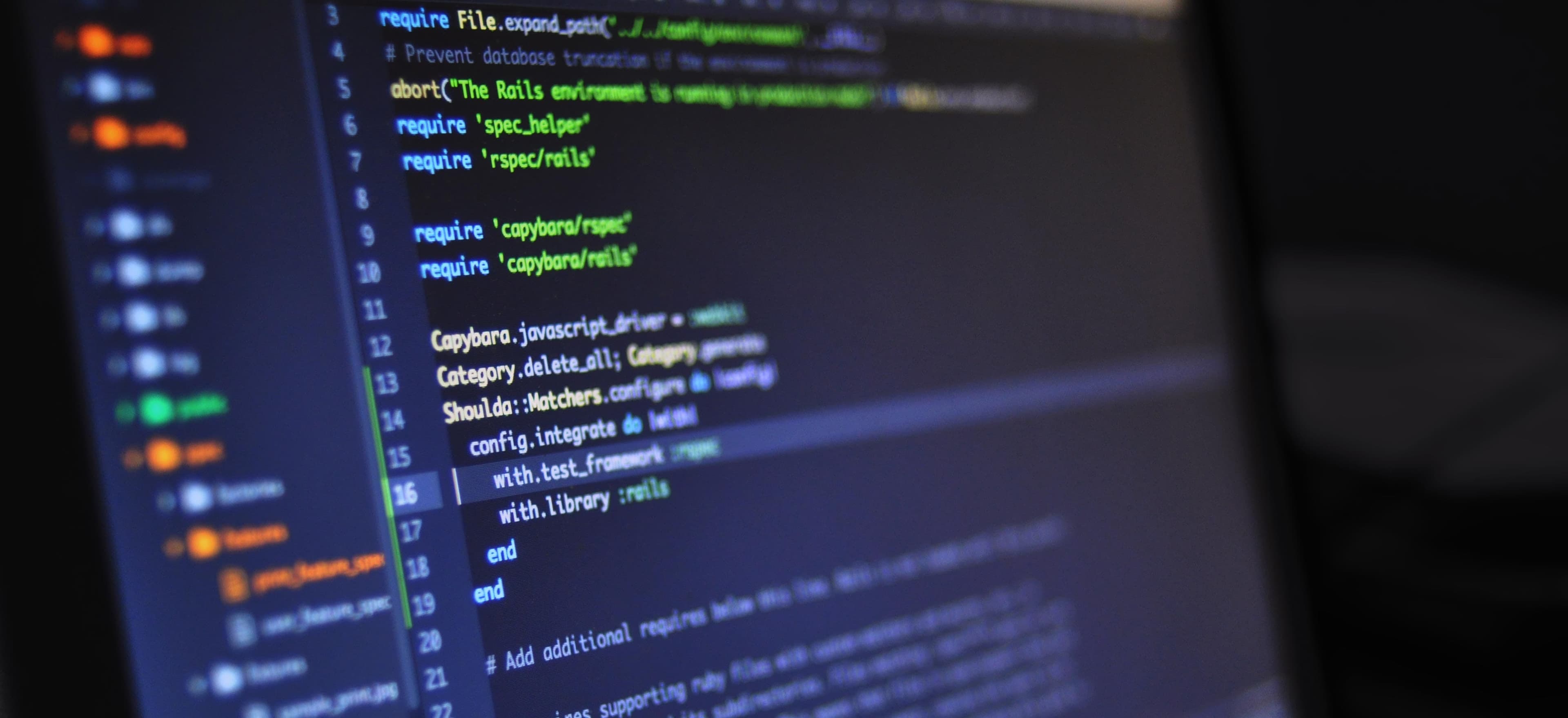
- Published on
Avoiding Common Tech Mistakes That Cost You Time and Money
In today's digital age, technology is the backbone of business operations. However, navigating the tech landscape isn't always smooth sailing. Mistakes can snowball into significant time and monetary losses. In this blog post, we will explore common tech mistakes in software development and IT management, and how to avoid them.
1. Underestimating Project Scope
One of the most prevalent mistakes in tech projects is underestimating the project scope. This often occurs during the planning phase.
Why It Happens:
- Lack of experience
- Poor requirement gathering
- Overconfidence in the development team's capabilities
How to Avoid It:
-
Conduct thorough requirement analysis: Take the time to involve stakeholders from the outset and gather detailed requirements. A simple technique is creating user stories that outline user needs.
-
Use the Agile Methodology: Agile allows for regular revision of project scope. According to Scrum Alliance, using Agile can provide flexibility to adapt to changing requirements.
Code Example:
public class UserStory {
private String role;
private String goal;
private String reason;
public UserStory(String role, String goal, String reason) {
this.role = role;
this.goal = goal;
this.reason = reason;
}
public void displayStory() {
System.out.println(role + " wants to " + goal + " so that " + reason);
}
}
// Usage
UserStory us = new UserStory("Admin", "manage user permissions", "they can control access");
us.displayStory();
This user story encapsulates goals and motivations clearly, ensuring everyone is on the same page.
2. Ignoring Vendor Support
Choosing software or platforms without considering vendor support can lead to significant downtime and frustrations.
Why It Happens:
- A focus solely on features and pricing
- Insufficient research
How to Avoid It:
- Evaluate vendor reputation: Look for user reviews, testimonials, and case studies.
- Request support documentation: Ensure the vendor provides ample resources for troubleshooting.
Example:
If you're using a cloud provider, assess the Service Level Agreement (SLA) they offer. Knowing what guarantees they provide can make or break your uptime.
3. Not Planning for Security
In today's digital environment, overlooking cybersecurity is a grave mistake that can compromise your organization.
Why It Happens:
- Assumption that security is 'someone else's job'
- Overconfidence in existing safeguards
How to Avoid It:
- Implement a security-first approach: Ensure security is a primary focus from the onset of software development.
- Regularly conduct security audits: This proactive measure identifies vulnerabilities before they become issues.
Code Example:
Implementing basic password security can start with checking for strength before allowing account creation.
public class UserRegistration {
public boolean isPasswordStrong(String password) {
return password.length() >= 8 &&
password.matches(".*[A-Z].*") &&
password.matches(".*[a-z].*") &&
password.matches(".*\\d.*") &&
password.matches(".*[@#$%^&+=].*");
}
}
// Usage
UserRegistration registration = new UserRegistration();
if (!registration.isPasswordStrong("Pswd123$")) {
System.out.println("Weak password! Please choose a stronger one.");
} else {
System.out.println("Registration successful!");
}
This simple function ensures that users create secure passwords, an essential part of safeguarding information.
4. Failing to Document the Process
Documentation is often an afterthought, but neglecting it can lead to redundancy and inefficiency.
Why It Happens:
- Pressuring timelines that prioritize getting things done over recording processes
How to Avoid It:
- Establish a documentation culture: Make documentation a key part of each sprint or project phase.
- Utilize tools for documentation: Tools like Confluence or Notion can centralize documentation and make it easily accessible.
5. Skipping Testing
Failing to rigorously test software can lead to buggy releases, diminishing user experience and eroding trust.
Why It Happens:
- Pressure to meet deadlines
- Belief that testing is a formality
How to Avoid It:
- Incorporate automated testing: Automated tests can save time and ensure consistency across builds.
- Encourage a testing mindset: Every team member should understand the importance of testing.
Code Example:
Here’s an example of a simple JUnit test case to validate the functionality of a function.
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class MathUtilTest {
@Test
public void testAddition() {
MathUtil mathUtil = new MathUtil();
assertEquals(5, mathUtil.add(2, 3), "2 + 3 should equal 5");
}
}
// The MathUtil class
public class MathUtil {
public int add(int a, int b) {
return a + b;
}
}
Through automated tests, you ensure your code performs as expected.
6. Neglecting Training and Onboarding
Investing in technology is paramount, but neglecting the human element can prove detrimental.
Why It Happens:
- Assumption that employees will automatically adapt to new tech
- Focus shifts away from human resources during tech upgrades
How to Avoid It:
- Develop onboarding processes: Create a structured program to educate users on new tools and software.
- Provide ongoing training: Continuous education can keep staff up-to-date with evolving technology.
A Final Look
Avoiding common tech mistakes requires diligence, planning, and a commitment to best practices. By scrutinizing project scope, ensuring vendor support, prioritizing security, documenting processes, implementing thorough testing, and investing in training, you can significantly reduce the risks associated with technology.
In our fast-paced tech world, where every second counts, making informed decisions can translate not only into time saved but also substantial cost reductions. Embrace these practices, and set your digital transformation on the right path.
For further reading on DevOps practices that can enhance your project management, check out Atlassian's guide on DevOps.
By staying vigilant against these common tech mistakes, you not only protect your investment but also foster a culture of continuous improvement within your organization.
Checkout our other articles