Common Pitfalls When Writing Spring WebFlux Filters
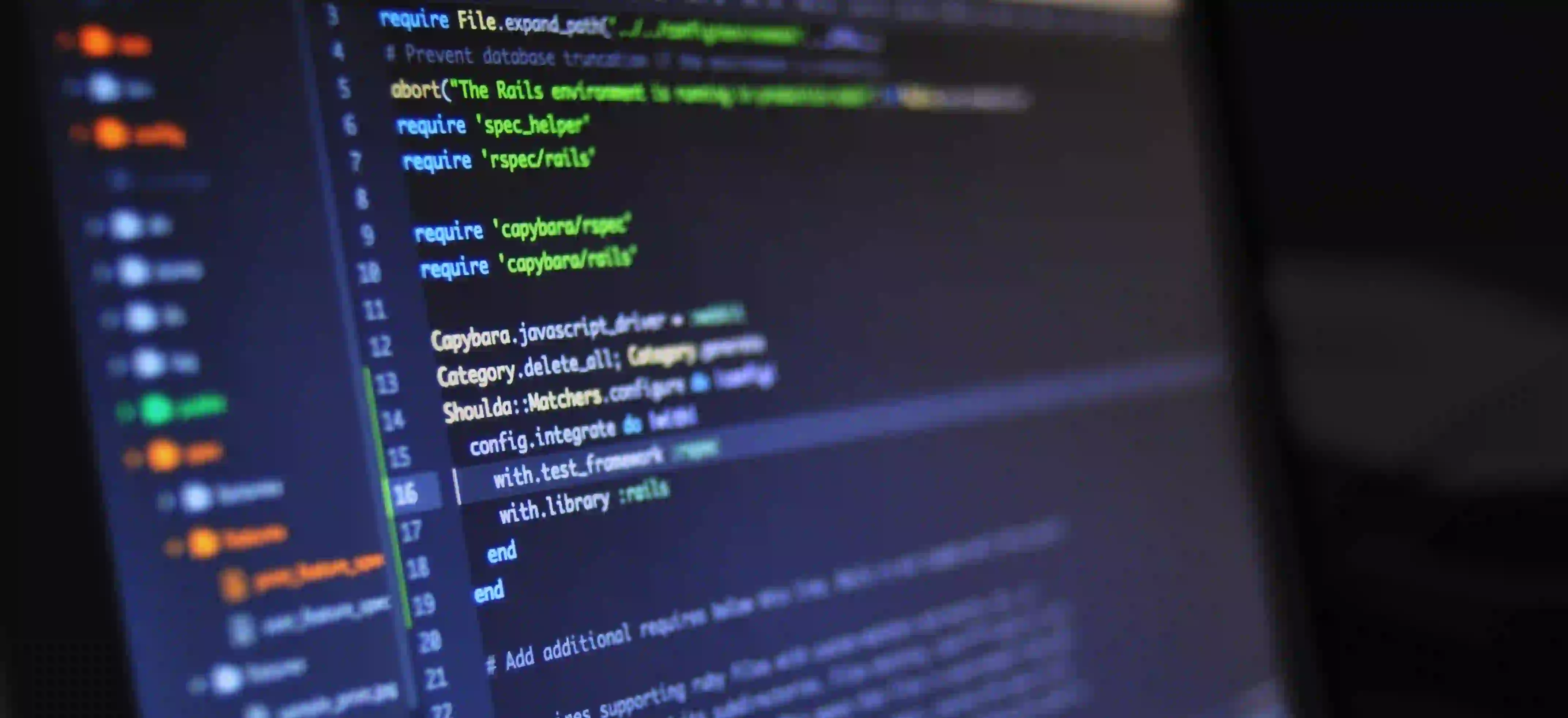
Common Pitfalls When Writing Spring WebFlux Filters
Spring WebFlux is a powerful and reactive framework for building non-blocking applications in Java. One of the critical components of WebFlux is the ability to use filters for handling requests and responses efficiently. Filters provide a way to intercept requests before they reach your controller, and they can also modify the response as needed. However, developing these filters can come with its own set of challenges.
In this blog post, we will explore some common pitfalls that developers encounter when writing Spring WebFlux filters, along with practical tips to avoid them.
Understanding Filters in Spring WebFlux
Before we dive into the pitfalls, let's clarify what filters are and how they work in the context of Spring WebFlux. A filter allows you to perform operations both before the request is handled and after the response is produced. Filters can be used for logging, modifying request or response attributes, authentication, and error handling.
Filters in Spring WebFlux are implemented using the WebFilter
interface, and you typically define them as beans. Here’s a simple example of a logging filter:
import org.springframework.stereotype.Component;
import org.springframework.web.server.ServerWebExchange;
import org.springframework.web.server.WebFilter;
import org.springframework.web.server.WebFilterChain;
import reactor.core.publisher.Mono;
@Component
public class LoggingFilter implements WebFilter {
@Override
public Mono<Void> filter(ServerWebExchange exchange, WebFilterChain chain) {
System.out.println("Request Path: " + exchange.getRequest().getPath());
return chain.filter(exchange).doOnSuccess(aVoid ->
System.out.println("Response Status Code: " + exchange.getResponse().getStatusCode()));
}
}
Why This Matters
In this example, we log the request path and the response status code. This kind of logging can be invaluable for debugging and monitoring your application.
Common Pitfalls
1. Blocking Operations
One of the most significant mistakes developers make when using WebFlux filters is performing blocking operations. WebFlux is built on a non-blocking reactive model, and blocking calls will undermine its performance.
Avoid this by:
Using non-blocking APIs whenever possible. For example, if you need to call a database or an external service, make sure you use a reactive library.
public Mono<Void> filter(ServerWebExchange exchange, WebFilterChain chain) {
return someReactiveService.callAsync()
.flatMap(result -> {
// process the result
return chain.filter(exchange);
});
}
2. Not Handling Errors Properly
When filters perform operations that could lead to errors, it’s crucial to handle those errors gracefully. If not handled, uncaught exceptions in filters can lead to a poor user experience or leaks sensitive information.
Handle errors like this:
You can catch exceptions that occur in your filters and return an appropriate error response.
@Override
public Mono<Void> filter(ServerWebExchange exchange, WebFilterChain chain) {
return chain.filter(exchange)
.onErrorResume(e -> {
exchange.getResponse().setStatusCode(HttpStatus.INTERNAL_SERVER_ERROR);
return exchange.getResponse().setComplete();
});
}
3. Not Using the Exchange Correctly
WebExchange carries important information about the request and response. Mismanaging this can lead to issues like headers not being modified or response body being incorrect.
Tip: Always clone the exchange when you need to modify it, especially for headers.
@Override
public Mono<Void> filter(ServerWebExchange exchange, WebFilterChain chain) {
ServerWebExchange mutatedExchange =
exchange.mutate()
.request(exchange.getRequest().mutate().header("X-Custom-Header", "value").build())
.build();
return chain.filter(mutatedExchange);
}
4. Long-Running Filters
Avoid the temptation to place time-consuming logic in your filters. Filters should be lightweight. Long-running logic should be shifted to service layers or asynchronous processing.
Keep it simple: Your filters should concentrate only on authentication, validation, or logging, and allow other components to handle requests more thoroughly.
5. Not Following Reactive Programming Principles
Spring WebFlux is based on the reactive paradigm, and you should consider using a reactive type like Mono
or Flux
for your filter methods. Avoid returning void
or other blocking types.
Use the reactive types wisely:
For example, if you need to read a request body, you can convert it to a Mono<String>
:
@Override
public Mono<Void> filter(ServerWebExchange exchange, WebFilterChain chain) {
return exchange.getRequest().getBody()
.map(dataBuffer -> {
// handle dataBuffer
return dataBuffer.toString(); // or some other manipulation
})
.flatMap(content -> {
// process content ...
return chain.filter(exchange);
});
}
6. Ignoring Request Context
In reactive applications, the request context can change between the filter and the controller. Always ensure that the context is consistent throughout. Failing to do so can lead to data inconsistency.
Store critical data in a way that it is always available by leveraging the ServerWebExchange.getAttributes()
method.
7. Failing to Test Filters
Lastly, publishers often overlook writing tests for filters. It's crucial to ensure that each filter behaves as expected, both in isolation and within the context of the entire application.
Unit Testing with MockWebServer:
Here’s a simple way to test your filter using the WebTestClient
:
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.reactive.AutoConfigureWebTestClient;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.web.reactive.server.WebTestClient;
@SpringBootTest
@AutoConfigureWebTestClient
public class LoggingFilterTest {
@Autowired
private WebTestClient webTestClient;
@Test
public void testLoggingFilter() {
webTestClient.get().uri("/some-path").exchange()
.expectStatus().isOk();
}
}
The Bottom Line
Writing filters in Spring WebFlux can enhance your application's capabilities, but it also comes with its set of challenges. By avoiding common pitfalls—such as blocking operations, error handling issues, and misunderstanding the non-blocking paradigm—you can implement effective filters that harness the full power of Spring WebFlux.
For more insights into reactive programming, check out Spring WebFlux documentation and to delve deeper into Java reactive programming concepts, visit Project Reactor.
Understanding these nuances will enable you to write cleaner, more efficient filters that contribute to a robust reactive application. Happy Coding!