Common MyBatis Mapping Issues in Spring Boot Applications
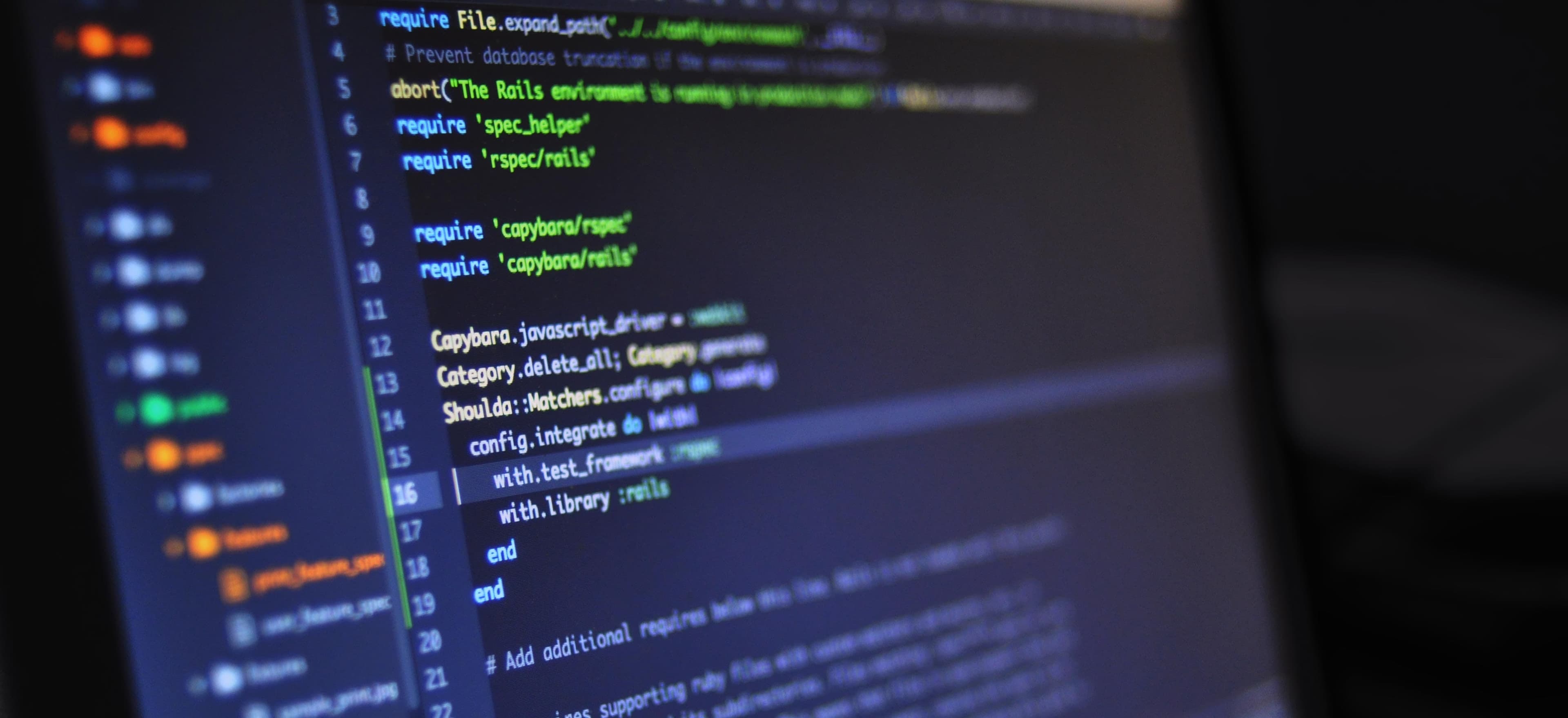
- Published on
Common MyBatis Mapping Issues in Spring Boot Applications
MyBatis is a powerful persistence framework that offers SQL statement mapping to objects in Java. When integrating MyBatis into Spring Boot applications, developers can face a variety of mapping issues. Understanding these common pitfalls can enhance efficiency and lead to smoother development processes. This blog post aims to explore these common MyBatis mapping issues, provide solutions, and explain the core concepts behind the code snippets provided.
What is MyBatis?
MyBatis is a Java framework that simplifies database access, enabling developers to execute SQL, retrieve and map records to Java objects. MyBatis allows for granular control over SQL code while ensuring easy integration with modern frameworks like Spring Boot. You can explore MyBatis documentation for in-depth information.
MyBatis and Spring Boot Integration
Spring Boot excels in simplifying the setup and configuration of Java applications. With MyBatis, integration involves traditionally setting up DataSource configurations and using MyBatis-Spring Boot Starter for ease of use.
Initial Configuration
A simplistic application setup might look like this:
@SpringBootApplication
@MapperScan("com.example.project.mapper") // Scans the mapper interfaces for MyBatis.
public class MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
}
Here, @MapperScan
automates the detection of MyBatis mapper interfaces.
Common Mapping Issues
1. Incorrect SQL Configuration
Issue: One common mistake developers make is misconfiguring SQL statements within the XML or Annotation-based mappers. This might lead to org.apache.ibatis.exceptions.PersistenceException
due to SQL syntax issues or mapping issues.
Solution: Always validate your SQL queries. For XML configurations, ensure that you are correctly referencing IDs and that they match the method signatures in the mapper interface.
Example of XML Mapper:
<mapper namespace="com.example.project.mapper.UserMapper">
<select id="findById" resultType="com.example.project.model.User">
SELECT * FROM users WHERE id = #{id}
</select>
</mapper>
Here, resultType
must map to a valid Java class. Make sure that the database schema and Java class properties align.
Why: SQL queries that don't align with class fields or method parameters lead to exceptions. Always keep mappings and queries synced.
2. Mapper Interface Annotations Confusion
Issue: Using annotations incorrectly within mapper interfaces can be problematic. For instance, the @Select
, @Insert
, @Update
, and @Delete
annotations must be accurately utilized to avoid mapping errors.
Solution: Ensure unique method names for each operation, and use the appropriate annotation for the intended query type:
@Mapper
public interface UserMapper {
@Select("SELECT * FROM users WHERE id = #{id}")
User findById(int id);
@Insert("INSERT INTO users(name, email) VALUES(#{name}, #{email})")
void insertUser(User user);
}
Why: Annotations must be clear so that MyBatis can properly associate methods with SQL statements. If a method has conflicting names or annotations, it results in ambiguous behavior.
3. Case Sensitivity Issues in Mapping
Issue: Another common pitfall is failing to recognize case sensitivity in database fields compared to Java properties. This often leads to null values or mapping errors.
Solution: Use the mapUnderscoreToCamelCase
setting in your MyBatis configurations:
mybatis:
configuration:
map-underscore-to-camel-case: true
With this, field names in the database like first_name
will automatically be mapped to firstName
in Java instances.
Why: This setting automates the required mapping effort and minimizes manual intervention.
4. Missing @Param Annotations
Issue: Failing to use the @Param
annotation can confuse MyBatis when multiple parameters are passed to a mapped method.
Solution: Use @Param
for clarity when passing multiple parameters:
@Mapper
public interface OrderMapper {
@Select("SELECT * FROM orders WHERE customer_id = #{customerId} AND order_date = #{orderDate}")
List<Order> findByCustomerAndDate(@Param("customerId") int customerId, @Param("orderDate") String orderDate);
}
Why: Without @Param
, MyBatis assumes a single object map, which may not align with SQL parameters, leading to unexpected results.
5. Lazy Loading Issues
Issue: Lazy loading is a performance enhancement feature, but it can also introduce issues, especially when dealing with complex object relationships. If not configured properly, you may encounter org.apache.ibatis.exceptions.BindingException
.
Solution: Ensure proper configuration in your configuration files:
<settings>
<setting name="lazyLoadingEnabled" value="true"/>
<setting name="aggressiveLazyLoading" value="false"/>
</settings>
Why: Using lazy loading can help in reducing the initial data load. However, when misconfigured, it might lead to runtime errors during data fetching.
6. Managing Transactions
Issue: Mismanagement of transactions can lead to data inconsistency, especially when updates involve multiple related tables.
Solution: Utilize Spring's transaction management capabilities:
@Service
@Transactional // Begin transaction for this service's methods
public class UserService {
@Autowired
private UserMapper userMapper;
public void createUser(User user) {
userMapper.insertUser(user);
// Additional operations that are related to this transaction
}
}
Why: Using @Transactional
ensures that all operations within the method either succeed or fail together, safeguarding data consistency.
Closing Remarks
Working with MyBatis in Spring Boot applications can be smooth if developers remain vigilant about common mapping issues. Always verify SQL statements, manage transactions, and ensure that mappings between database fields and Java properties are well-defined.
For more detailed insights into advanced MyBatis features, check out the MyBatis-Spring documentation. Taking note of these practices can streamline your development process and empower you to build robust applications efficiently.
With these strategies at your disposal, you are now equipped to handle common MyBatis mapping issues effectively in your Spring Boot applications. Happy coding!