Understanding Unintended Side Effects of Java String in JDK 9
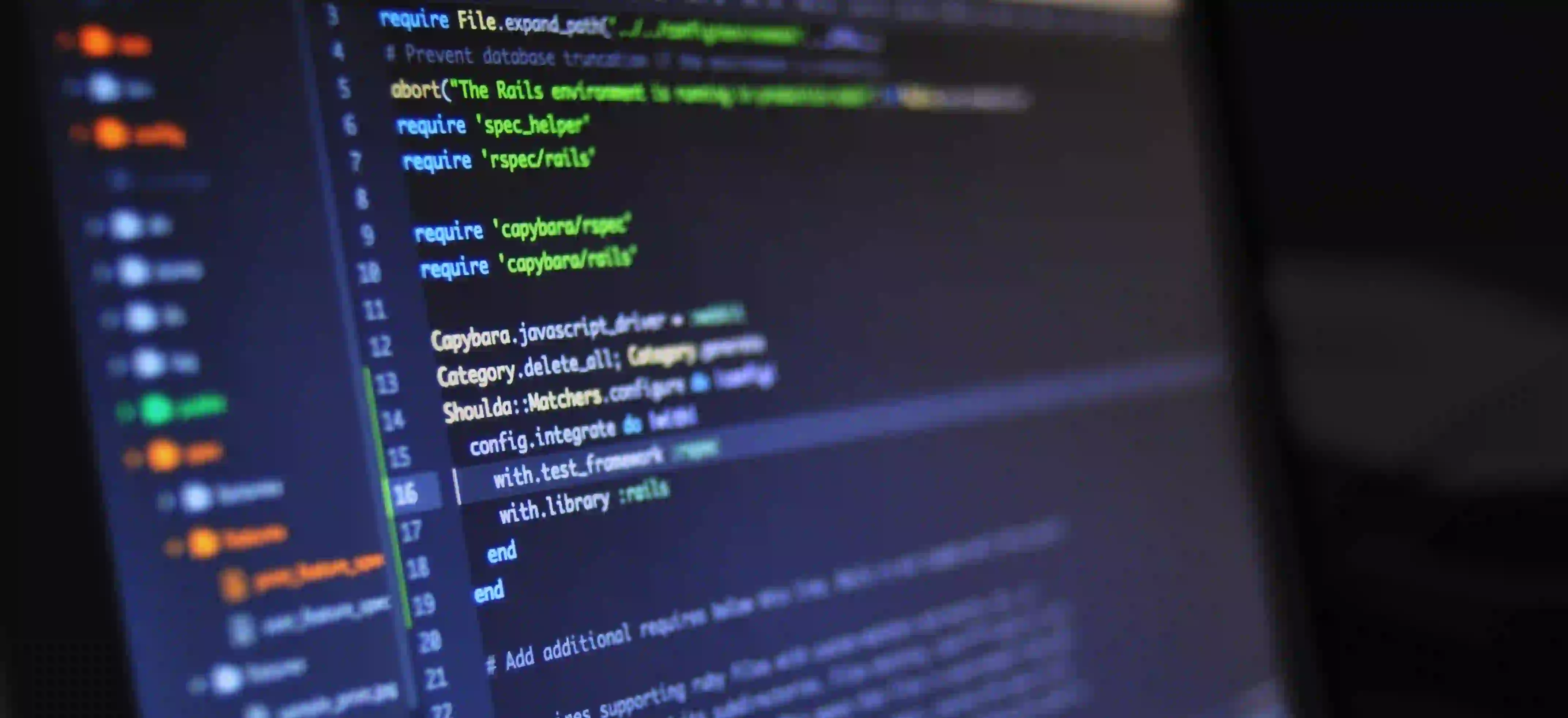
Understanding Unintended Side Effects of Java String in JDK 9
The introduction of Java Development Kit (JDK) 9 brought with it several significant changes and enhancements. One of the updates that sparked considerable interest and discussion among Java developers was the modification of the String
class. While the update aimed at improving memory efficiency, it also led to unintended side effects that many developers overlooked. In this blog post, we will explore what changed in the String
class in JDK 9, the concept of memory representation in Java, and the potential unintended side effects that can occur as a result.
The Memory Representation of Strings
Before diving into the changes introduced in JDK 9, it's essential to understand how Java represents strings under the hood.
Pre-JDK 9 String Implementation
Prior to JDK 9, a Java String
was represented in memory as an array of char
and an associated length. This means that every string consumed a significant amount of memory, especially for large strings. The String
class looked something like this:
public final class String {
private final char value[];
private final int offset; // For substring support
private final int count; // String length
...
}
This implementation, while straightforward, was inefficient when it came to memory consumption, particularly for applications dealing with a large number of strings.
The JDK 9 Change
To address the memory consumption issue, JDK 9 introduced a new representation of strings. The key enhancement included changing the internal character array from char[]
to a byte[]
. Here’s the critical piece of code from the new implementation:
public final class String {
private final byte[] value; // The bytes for the new String
// Other existing variables
...
}
This change means that strings now utilize a more memory-efficient approach where characters are stored as bytes whenever possible. This alteration supports ASCII characters with a much smaller memory footprint. For characters that fall outside this range, the data is still transformed and managed appropriately.
Why the Change?
The drive for this modification hinged on performance. Reducing memory usage can lead to improvements in speed and effectiveness for applications with high string usage. Consequently, this seems like a great improvement—but as many would discover, it comes with unintended side effects.
Unintended Side Effects
With the new representation of strings, developers began to notice several unintended side effects. Some of the fundamental issues arise in regards to the representation and manipulation of strings, particularly with encoding.
1. Incompatibility with Legacy Code
One immediate side effect is that some legacy applications faced compatibility issues due to the way characters are handled in different encodings. Previously benign code could suddenly lead to unexpected behavior due to changes in the way certain characters are represented.
Example
Consider this code that leverages the String
class with a standard substring operation:
String originalString = "Hello, World!";
String subString = originalString.substring(0, 5);
System.out.println(subString); // Output: Hello
In JDK 9, the substrings still work as expected, but if one were to deal with characters outside the ASCII range or different encodings, the behavior might vary dramatically.
2. Increased Complexity for Multibyte Character Encodings
If you're working with multibyte character encodings such as UTF-8 or UTF-16, the representation of strings in JDK 9 adds a layer of complexity. Even if you assume your strings are straightforward ASCII strings, encoding can introduce hidden pitfalls due to the transformation to a byte array.
Example
The following code attempts to manipulate a string containing multibyte characters:
String multiByteString = "Java ❤️";
byte[] bytes = multiByteString.getBytes();
System.out.println(new String(bytes)); // Output could be problematic depending on encoding
Due to changes in how strings are represented, it’s crucial to ensure that character encoding is handled correctly.
3. Memory Consumption Behavior
While the change aimed at reducing memory consumption, it had the contradictory potential of increasing overhead. The added complexity of byte management can sometimes lead to higher memory usage in less optimized or older code with poor handling of these new byte representations.
Best Practices for Mitigating Side Effects
To navigate the potential side effects brought on by changes in JDK 9, here are some best practices that developers should consider:
-
Test Legacy Code Extensively: If you have an existing codebase, it's vital to thoroughly test your applications to identify any behavior changes when transitioning to JDK 9.
-
Understand Character Encoding: Before manipulating strings, especially if they include special characters or come from external sources, familiarize yourself with Java's character encoding and ensure proper conversions.
-
Profile Memory Usage: Use profilers to examine your application's memory consumption patterns. This can help you detect odd behaviors that stem from the new string implementation.
-
Use Informative Comments: When working with strings, especially when using legacy or external libraries, include comments explaining any potential pitfalls arising from the new representation.
Closing the Chapter
In summary, the modifications in the String
class introduced in JDK 9 brought significant improvements in performance and memory efficiency, but they also led to unintended side effects that developers must be wary of. As you continue developing in Java, keeping these architectural changes in mind will help you avoid common pitfalls and ensure that your applications run smoothly.
For more information on the intricacies of string manipulation and character encoding in Java, you can refer to the comprehensive Java Documentation or explore detailed discussions on Java String Performance to deepen your understanding of how these changes impact overall application design.
Keep coding with awareness, and happy Java programming!