Troubleshooting Common RabbitMQ Connection Issues
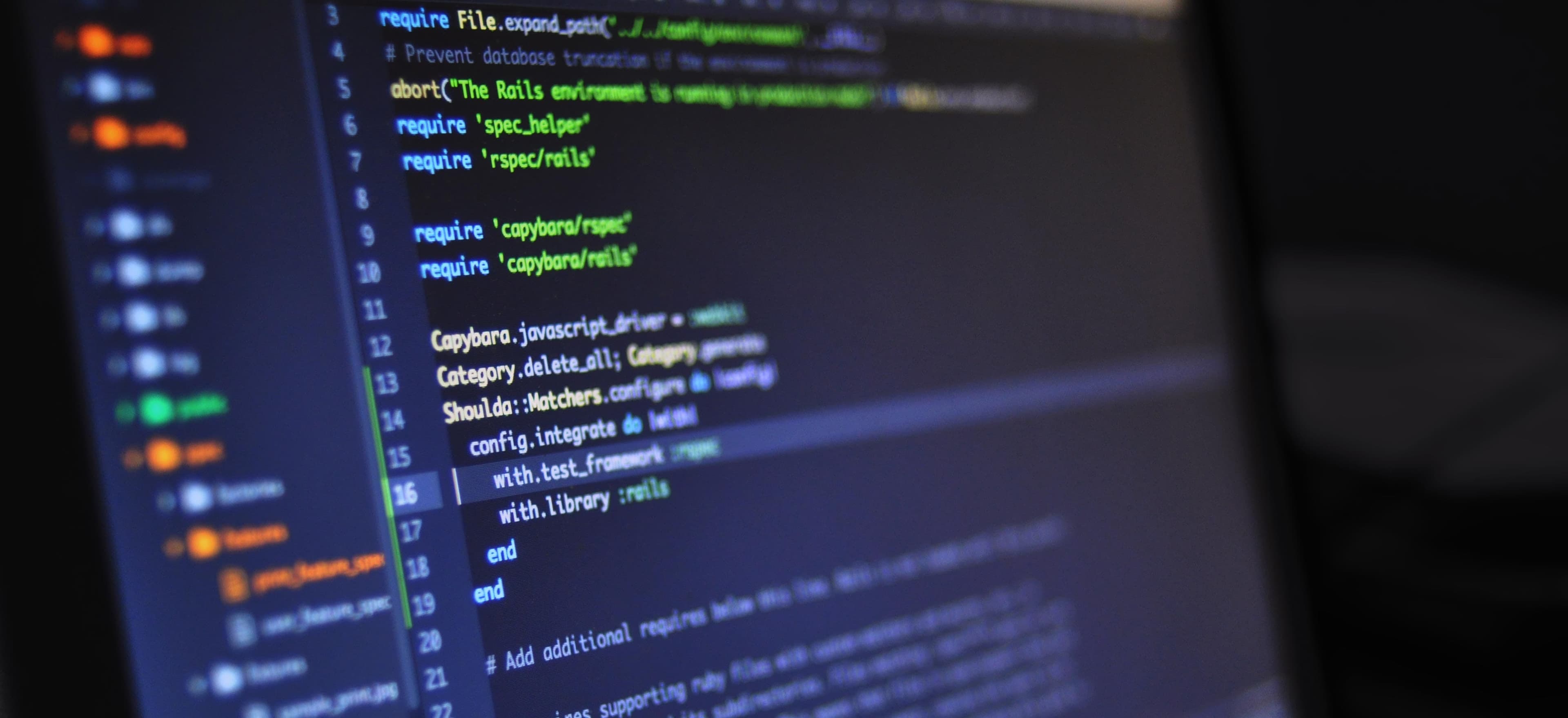
- Published on
Troubleshooting Common RabbitMQ Connection Issues
RabbitMQ is a powerful message broker that allows applications to communicate and scale effectively. However, like any system, you may encounter connection issues from time to time. This blog post delves into common RabbitMQ connection problems, their root causes, and methods to troubleshoot them effectively. Whether you're a seasoned developer or just starting, this guide will help you resolve these issues with ease.
Understanding RabbitMQ Connection
RabbitMQ uses the Advanced Message Queuing Protocol (AMQP) to facilitate the seamless transfer of messages between servers and clients. The connection issues can arise from various factors, including network configuration, SSL/TLS setup, or misconfiguration of RabbitMQ itself.
Key Error Messages to Look For
When troubleshooting connection issues, you may come across several common error messages:
- Connection Refused: Usually indicates that RabbitMQ is not running or is blocking access to the client.
- Connection Timeout: Suggests network connectivity problems or firewall settings.
- Authentication Failure: Occurs when the credentials used do not match the RabbitMQ user credentials.
Before diving into troubleshooting, make sure you have RabbitMQ and its management plugin installed. For installation instructions, visit the RabbitMQ Installation Documentation.
Common RabbitMQ Connection Issues
1. RabbitMQ Not Running
One of the most straightforward issues to diagnose is whether RabbitMQ is running at all.
# Check RabbitMQ status
sudo systemctl status rabbitmq-server
Solution
If RabbitMQ isn't running, start it using the following command:
sudo systemctl start rabbitmq-server
2. Socket Connection Error
This error shows that your application cannot establish a connection with the RabbitMQ server.
Possible Causes:
- The RabbitMQ server might be listening on the wrong port.
- Network-related issues between the client and the server.
Solution:
Check the RabbitMQ server's configuration file, typically located at /etc/rabbitmq/rabbitmq.conf
. Look for the listener configuration:
listeners.tcp.default = 5672
Ensure your application is trying to connect to the correct host and port combination.
3. Firewall or Security Group Issues
If RabbitMQ is running but clients cannot connect, a firewall or security group may be blocking access. This is particularly common in cloud environments.
Solution:
For Linux servers, use iptables
to check your firewall rules:
sudo iptables -L
If the server is hosted in a cloud provider, ensure that the security group allows inbound traffic on port 5672.
4. Authentication Errors
RabbitMQ checks client credentials against its internal user database. If the credentials are incorrect, you will receive an authentication failure error.
Solution:
You can add or update users using the RabbitMQ command-line interface:
# Add user
sudo rabbitmqctl add_user myuser mypassword
# Set permissions
sudo rabbitmqctl set_permissions -p / myuser ".*" ".*" ".*"
Additionally, ensure that your application is using the correct username and password to connect.
5. SSL/TLS Configuration Issues
If you are using Secure Sockets Layer (SSL) or Transport Layer Security (TLS) to encrypt RabbitMQ connections, misconfiguration here can lead to connection issues.
Solution:
Enable SSL in your RabbitMQ configuration file:
listeners.ssl.default = 5671
ssl_options.cacertfile = /path/to/cacert.pem
ssl_options.certfile = /path/to/cert.pem
ssl_options.keyfile = /path/to/key.pem
Further, verify that your client is configured to connect using SSL:
ConnectionFactory factory = new ConnectionFactory();
factory.useSslProtocol();
6. Connection Timeout
Timeout errors often result from prolonged attempts to connect to RabbitMQ, usually due to network issues or misconfigurations like incorrect DNS settings.
Solution:
Test the connection from your application server to the RabbitMQ server using telnet
:
telnet rabbitmq_host 5672
If the connection fails, examine your network setup, DNS resolution, and ensure that RabbitMQ listens on that port.
Debugging Connection Issues
Use RabbitMQ Logs
RabbitMQ provides extensive logging features, which can assist in troubleshooting. You can find logs at /var/log/rabbitmq/
. Look for any errors that occur around the same time as your connection attempts.
Monitoring Tools
Leverage RabbitMQ's built-in management interface to check the status of connections, channels, and other metrics. Access it by navigating to http://<hostname>:15672/
after enabling it.
Code Example
Here’s an example demonstrating how to handle failed connections using RabbitMQ in Java.
import com.rabbitmq.client.Connection;
import com.rabbitmq.client.ConnectionFactory;
public class RabbitMQConnection {
public static void main(String[] args) {
ConnectionFactory factory = new ConnectionFactory();
factory.setHost("localhost");
try (Connection connection = factory.newConnection()) {
System.out.println("Connection established successfully!");
} catch (IOException | TimeoutException e) {
System.err.println("Connection failed: " + e.getMessage());
}
}
}
Why Handle Exceptions?
Handling exceptions accurately informs you about the nature of connection issues while providing a graceful way to deal with failures in runtime.
Key Takeaways
RabbitMQ is an invaluable tool for building scalable, reliable applications. By understanding common connection issues and their troubleshooting steps, you can effectively resolve problems quickly and ensure optimal communication between your services.
For additional reading, explore:
Leveraging these solutions and monitoring techniques allows you not only to troubleshoot efficiently but also to maintain a robust message broker environment in your applications. Happy coding!
Checkout our other articles