Top Strategies to Overcome Production Delays
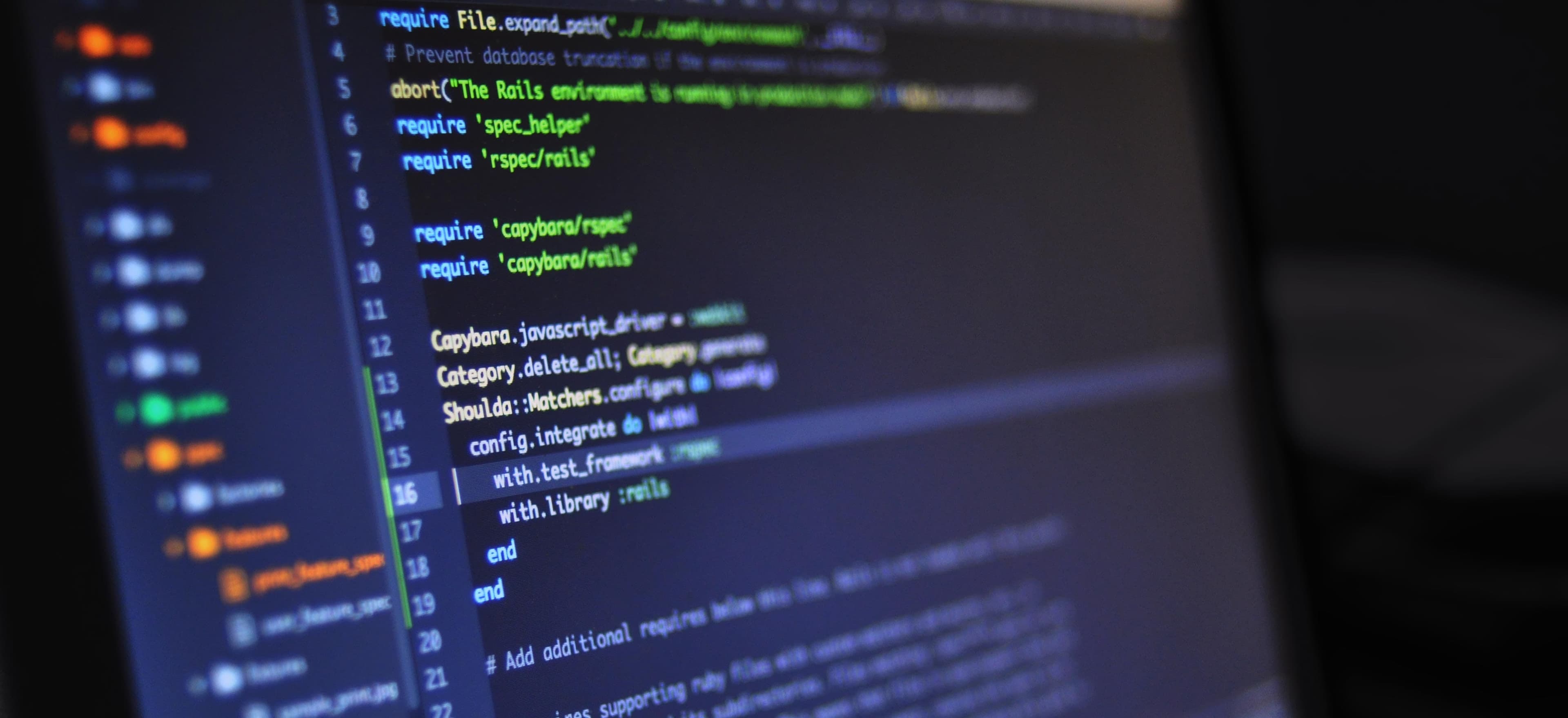
- Published on
Top Strategies to Overcome Production Delays
Production delays can be a significant hurdle in any industry, especially in software development. These delays can lead to missed deadlines, unsatisfied clients, and, ultimately, lost revenue. In this blog post, we will explore several key strategies that can help you mitigate and overcome production delays effectively.
Understanding Production Delays
Before diving into strategies, it’s essential to understand why production delays occur. Common reasons include:
-
Poor Planning: Inadequate project scoping or vague requirements can throw a wrench into the workflow.
-
Resource Allocation: Insufficient human or technical resources can slow down project timelines.
-
Technical Challenges: Unexpected technical issues can derail the production process.
-
Communication Gaps: Misaligned teams can lead to misunderstandings and, thus, delays.
Understanding these root causes is the first step towards effective solutions.
1. Prioritize Effective Planning
The foundation of any successful project lies in thorough planning. Here is how you can create a more robust plan:
-
Set Clear Goals: Define what success looks like. Utilize the SMART criteria (Specific, Measurable, Achievable, Relevant, Time-bound).
-
Break Down Tasks: Decompose larger projects into manageable tasks. This approach allows for better tracking and accountability.
-
Implement Agile Methodologies: Agile frameworks like Scrum or Kanban help keep projects adaptable and streamlined.
Example Code for Agile Planning
// A simple Task class to manage tasks in an Agile board
public class Task {
private String title;
private String description;
private boolean isCompleted;
public Task(String title, String description) {
this.title = title;
this.description = description;
this.isCompleted = false;
}
public void complete() {
this.isCompleted = true;
}
public boolean isCompleted() {
return this.isCompleted;
}
@Override
public String toString() {
return this.title + " - " + (isCompleted ? "Completed" : "Pending");
}
}
Commentary:
Here we define a simple Task
class that manages whether a task is completed. This approach reflects Agile principles by keeping tasks discreet and assessable, making it easier to track progress and avoid delays.
2. Optimize Resource Management
Proper resource allocation can eradicate the problem of bottlenecks caused by scarcity. Here’s how to achieve this:
-
Balance Your Team's Workload: Regularly assess your team's capacity and redistribute tasks if necessary to prevent overwhelm.
-
Invest in Training: Ensure your team is well-versed in the technologies and practices necessary for their roles.
-
Use Project Management Tools: Tools like Jira, Trello, or Asana can significantly contribute to organizing workloads.
3. Embrace Automation
Automation is your ally in reducing time spent on repetitive tasks. Automate wherever possible, from build processes to testing phases.
Example: Automated Testing with JUnit
import org.junit.jupiter.api.*;
import static org.junit.jupiter.api.Assertions.*;
public class CalculatorTest {
private Calculator calculator;
@BeforeEach
public void setUp() {
calculator = new Calculator();
}
@Test
public void testAddition() {
assertEquals(5, calculator.add(2, 3));
}
@Test
public void testSubtraction() {
assertEquals(1, calculator.subtract(3, 2));
}
}
Commentary:
Automated tests like the JUnit example above allow developers to validate code changes quickly. Rapid feedback leads to faster iterations and reduces the chances of delays.
4. Foster Open Communication
Creation of a culture that promotes open and honest communication is vital. Here are some effective ways to enhance communication:
-
Regular Stand-Ups: Daily meetings can keep everyone informed about progress and roadblocks.
-
Feedback Loops: Encourage team members to give and receive constructive feedback frequently.
-
Use Collaboration Tools: Platforms like Slack or Microsoft Teams can facilitate instant messaging and updates.
5. Address Technical Challenges Early
Identify technical challenges at the earliest possible stage. Here’s how:
-
Conduct Code Reviews: Regular reviews can catch issues before they snowball.
-
Utilize Version Control: Systems like Git allow for better tracking of changes and quick revert to stable states.
-
Establish a Testing Phase: Implement a robust testing phase before the final release, allowing enough time to tackle any surfacing issues.
6. Learn from Past Delays
Reflecting on previous projects can yield valuable insights. Key actions include:
-
Post-Mortems: Conduct these sessions after completing projects to analyze what went wrong and what can be improved.
-
Maintain a Lessons Learned Log: Document findings and keep them accessible for future reference.
7. Set a Contingency Plan
No project should be without a contingency plan. Prepare for worst-case scenarios and allocate a buffer in timelines.
-
Allocate Extra Time: Build in a time buffer for tasks that might take longer than expected.
-
Plan for Resource Availability: If a critical team member is unavailable, ensure others can fill in their role.
Final Thoughts
Overcoming production delays involves a combination of effective planning, meticulous resource management, embracing automation, and fostering a culture of open communication. By recognizing the common causes of delays and implementing the suggested strategies, your team can significantly streamline production timelines while maintaining compatibility with modern development practices.
To further enhance your project management capabilities, consider exploring additional resources like the Agile Alliance and Scrum Alliance, which offer extensive content and community support for Agile practices.
By adhering to these guidelines and continuously learning from experiences, you can create a resilient development cycle that not only meets deadlines but also delights clients with quality outcomes. Stay proactive, and let these strategies be your roadmap toward overcoming production delays!
Checkout our other articles