Overcoming CI/CD Pipeline Bottlenecks for Swift Delivery
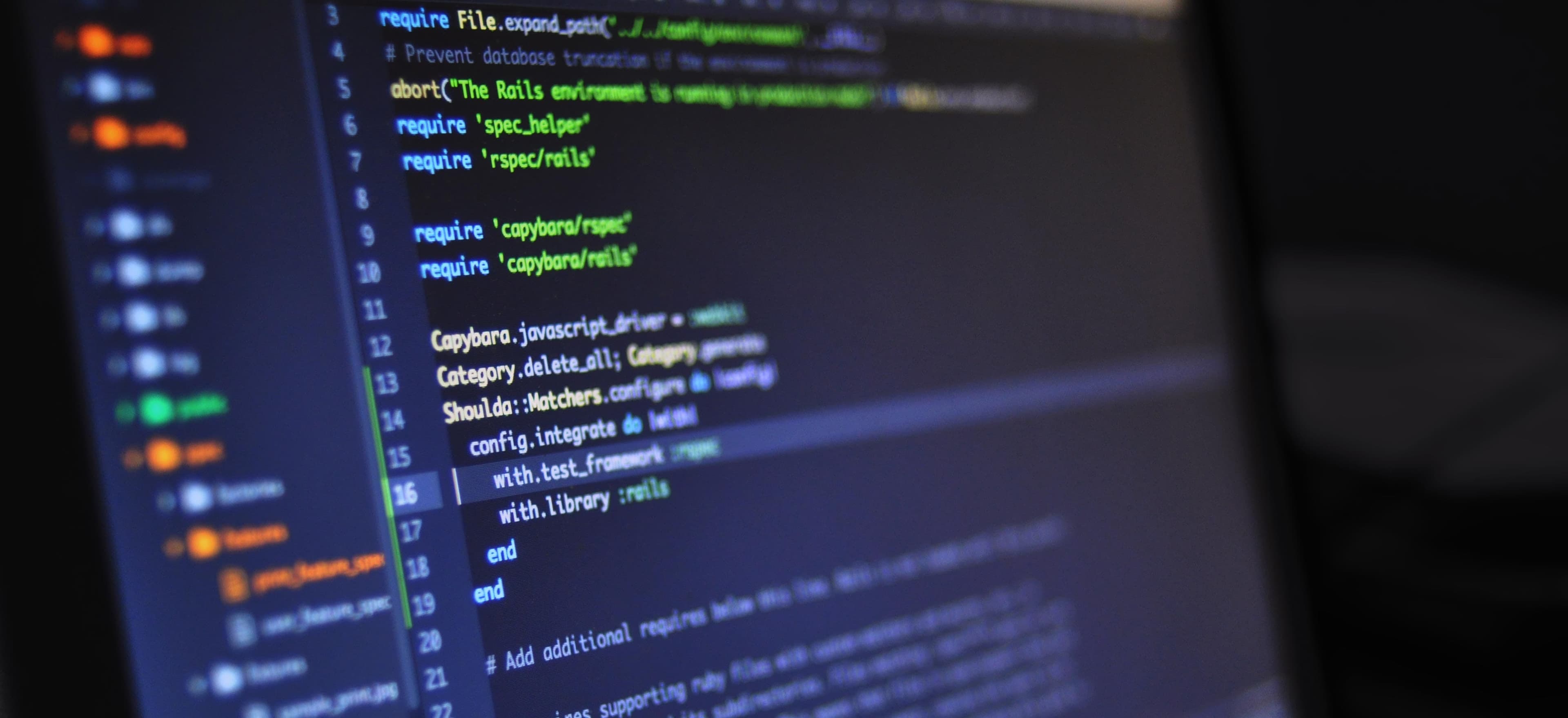
- Published on
Overcoming CI/CD Pipeline Bottlenecks for Swift Delivery
Continuous Integration and Continuous Delivery (CI/CD) pipelines are essential components of modern software development. They allow teams to build, test, and deploy applications swiftly and reliably. However, there can be numerous bottlenecks in a CI/CD pipeline that hinder this efficiency. In this blog post, we will explore common bottlenecks, their impact, and effective strategies to overcome them.
Understanding CI/CD Pipelines
Before diving into the bottlenecks, it's crucial to understand what a CI/CD pipeline is. A CI/CD pipeline automates the process of integrating code changes and delivering them to production swiftly and securely. The core components include:
- Continuous Integration (CI): This is about integrating code changes regularly to detect errors quickly.
- Continuous Delivery (CD): This ensures that code changes are automatically prepared for release to production.
To implement CI/CD effectively, teams often use tools like Jenkins, GitHub Actions, Travis CI, and GitLab CI. These tools streamline the process, but not without challenges.
Common CI/CD Pipeline Bottlenecks
1. Slow Build Times
Build time is a common bottleneck. If your builds take too long, your team cannot integrate new changes efficiently. This creates frustration and slows down production.
Solution: Use incremental builds. Instead of rebuilding the entire application, only build what has changed. CI tools like Jenkins support incremental builds, allowing for quicker feedback loops.
# Example of incremental build in Jenkins
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
sh './gradlew build --daemon'
}
}
}
}
}
Why? Incremental builds preserve time by focusing only on relevant changes, improving overall project efficiency.
2. Inefficient Testing Practices
Automated testing is critical in a CI/CD process, but flaky or extensive tests can become a bottleneck. If tests take too long or fail intermittently, developers may face unnecessary delays in the deployment pipeline.
Solution: Invest in test optimization. Break down large test suites into smaller, more manageable parts and introduce parallel testing where possible.
// Example of parallel testing with Jest
describe('My App Tests', () => {
test.concurrent('Test A', async () => {
// Test code for A
});
test.concurrent('Test B', async () => {
// Test code for B
});
});
Why? Running tests in parallel minimizes wait times, allowing for quicker feedback and deployment cycles.
3. Environment Issues
Inconsistent environments can lead to the "works on my machine" syndrome. If your team is testing in different environments, errors tend to be harder to pick up.
Solution: Utilize containerization technologies like Docker to create consistent environments across development, testing, and production.
# Dockerfile for a Java application
FROM openjdk:11-jdk
COPY target/myapp.jar /usr/app/myapp.jar
WORKDIR /usr/app
CMD ["java", "-jar", "myapp.jar"]
Why? Docker containers ensure that your application behaves the same way across different setups, drastically reducing deployment issues.
4. Manual Approval Processes
Long manual approval processes can slow down the deployment of new features. When approvals require extensive awaiting, the entire pipeline can come to a standstill.
Solution: Implement automated approvals for certain types of deployments, such as low-risk features. For higher-risk deployments, use well-defined policies to streamline the approval process.
5. Lack of Visibility and Monitoring
If your team cannot monitor CI/CD processes effectively, identifying and resolving bottlenecks becomes increasingly difficult.
Solution: Implement advanced monitoring tools, like Prometheus or Grafana, to keep a pulse on your pipeline's health. Utilize dashboards to visualize build statuses, test results, and deployment statistics.
# Example configuration to send metrics to Prometheus
prometheus:
enabled: true
prometheusPort: 9090
Why? Being able to visualize your CI/CD pipeline in real-time allows for proactive identification of potential bottlenecks before they escalate.
Best Practices for CI/CD Optimization
Now that we’ve identified specific bottlenecks and their solutions, it's time to look at best practices for optimizing your CI/CD pipeline:
1. Regularly Monitor Pipeline Performance
Stay on top of your pipeline's performance metrics. This allows teams to pinpoint which stages are consistently slow and need intervention. Create reports to analyze trends over time.
2. Foster a Culture of Collaboration
Encourage collaboration between development, operations, and QA teams. When everyone works together, it's easier to spot problems and facilitate smoother deployments.
3. Prioritize Tests
Not all tests are created equal. Categorize tests into unit, integration, and end-to-end tests, and prioritize them according to their importance.
4. Automate Everything Possible
Automation is the key to efficiency. From the build process to deployment strategies, minimizing manual intervention reduces the scope for human error.
5. Keep Dependencies Updated
Always keep your CI/CD tools and dependencies updated. Outdated tools can harbor security vulnerabilities and contribute to performance issues.
Closing Remarks
Overcoming CI/CD pipeline bottlenecks requires a keen understanding of the challenges, practical solutions, and adherence to best practices. As software development continues to evolve, the importance of efficient CI/CD pipelines cannot be overstated. By implementing the strategies discussed, your team can improve delivery speed and software quality.
For a more in-depth exploration of CI/CD practices, you may visit Atlassian's CI/CD Overview or check out Jenkins CI/CD Best Practices.
By taking these steps, your team will be better equipped to navigate the complexities of modern software development, delivering high-quality software quickly and efficiently. Happy coding!
Checkout our other articles