Common Pitfalls in Spring Security Maven Projects
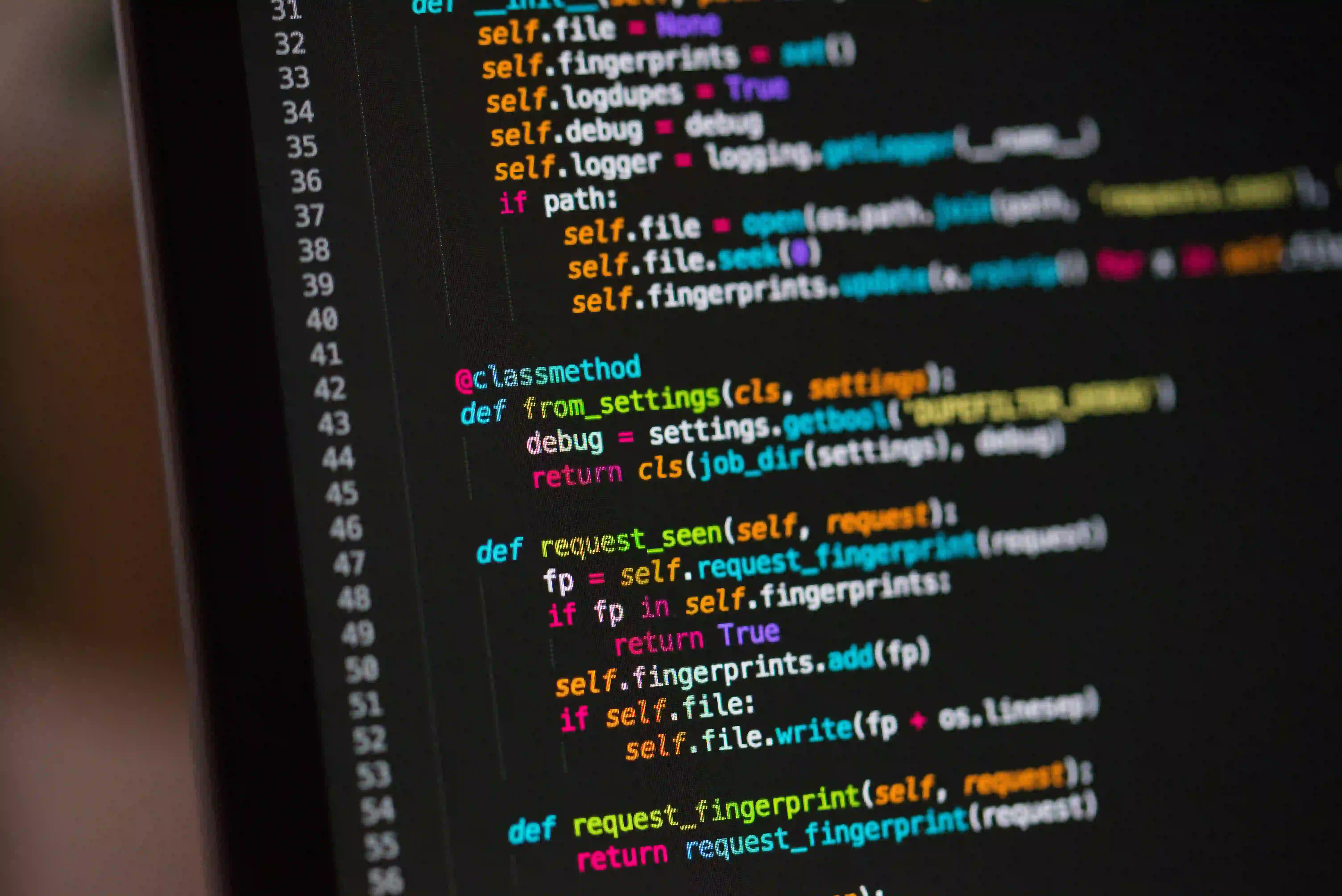
Common Pitfalls in Spring Security Maven Projects
When working on Java applications using the Spring Framework, developers often choose Spring Security to handle authentication and authorization. Although Spring Security offers robust functionality, it can also introduce complexities, especially within Maven projects. In this blog post, we will explore some common pitfalls associated with Spring Security in Maven projects and how to address them effectively.
Understanding Spring Security
Before delving into the pitfalls, let’s quickly recap what Spring Security does. It provides comprehensive security services for Java applications, primarily aimed at securing REST APIs, web applications, and microservices. Key features include:
- Authentication and Authorization
- Password Encoding
- Preventing Cross-Site Request Forgery (CSRF)
- Method-level Security
Why Choose Maven for Your Spring Security Project?
Maven is a build automation and dependency management tool that simplifies the process of managing libraries and project dependencies in Java. Using Maven with Spring Security allows for easy integration and proper version control. However, even the best tools can lead to issues if not utilized correctly.
Common Pitfalls
1. Dependency Conflicts
Maven dependencies can lead to jar hell, where different versions of the same library conflict with one another. This conflict is especially prevalent in security projects due to the variety of dependencies required.
Solution: Manage Dependencies Wisely
Use the Maven Dependency plugin to analyze dependency trees and manage version conflicts.
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-core</artifactId>
<version>5.5.2</version>
</dependency>
To analyze dependencies, run:
mvn dependency:tree
This command illustrates how dependencies relate and can help pinpoint conflicts. It’s essential to ensure that your dependencies are compatible with each other, especially when dealing with Spring Security.
2. Incorrect Configuration of Security Filters
In Spring Security, filters manage the security flow of requests. Misconfiguring security filters can lead to vulnerabilities or application failures.
Solution: Properly Define Security Filter Chains
Ensure that you define the order of your security filters correctly. Misordering can lead to unexpected behaviors.
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable() // disable CSRF in non-browser clients (like REST)
.authorizeRequests()
.antMatchers("/api/public/**").permitAll()
.antMatchers("/api/private/**").authenticated()
.and()
.httpBasic(); // enable Basic Authentication
}
Here, disabling CSRF for a RESTful service allows clear communication. Always be mindful of which endpoints should be secured.
3. Using Hardcoded Credentials
Hardcoding credentials in your application can lead to security vulnerabilities and makes it difficult to rotate passwords.
Solution: Use Externalized Configuration
Spring Security can integrate easily with external configuration management systems or use Spring’s support for environment variables.
# application.properties
spring.security.user.name=${SECURITY_USER_NAME}
spring.security.user.password=${SECURITY_USER_PASSWORD}
By utilizing environment variables, your application gains security and flexibility, allowing for easier deployments.
4. Forgetting to Encode Passwords
When handling user passwords, never store them in plain text. Failing to encode passwords correctly can open your application to attacks.
Solution: Use Password Encoders
Spring Security offers various password encoders. Here’s how to use a BCryptPasswordEncoder
:
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
Whenever you create or update a user password, ensure you encode it:
String encodedPassword = passwordEncoder().encode(user.getPassword());
Using a strong password encoder, like BCrypt, is crucial for securing user credentials.
5. Misconfigured CORS Policy
Cross-Origin Resource Sharing (CORS) errors are common when developing APIs. Improper CORS configurations can hinder API requests from valid sources.
Solution: Configure CORS Properly
You can enable CORS in Spring Security as follows:
@Override
protected void configure(HttpSecurity http) throws Exception {
http.cors().and()
.authorizeRequests() // further configuration...
}
Additionally, define a CorsConfigurationSource
bean:
@Bean
public CorsConfigurationSource corsConfigurationSource() {
CorsConfiguration configuration = new CorsConfiguration();
configuration.setAllowedOrigins(Arrays.asList("http://localhost:3000"));
configuration.setAllowedMethods(Arrays.asList("GET", "POST", "PUT", "DELETE"));
configuration.setAllowCredentials(true);
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
source.registerCorsConfiguration("/**", configuration);
return source;
}
Ensure that CORS settings are comprehensive enough to prevent unauthorized access but strict enough to prevent abuse.
6. Ignoring Security Annotations
Many developers overlook the use of Spring Security annotations. Failing to use these annotations can mean that security policies are handled in a non-optimized way.
Solution: Leverage Security Annotations
Use annotations like @PreAuthorize
, @Secured
, and @RolesAllowed
to enforce method-level security.
@PreAuthorize("hasRole('ADMIN')")
public void adminOnlyMethod() {
// Business logic for admin users only
}
By utilizing these annotations, you give your application finer granularity over role-based access.
7. Not Implementing Logging and Monitoring
A common pitfall in Spring Security is the lack of adequate logging or monitoring. Failing to log security events can obscure potential security breaches.
Solution: Implement Logging Mechanisms
Utilize Spring Security’s events and integrate with logging frameworks.
import org.springframework.context.ApplicationListener;
import org.springframework.security.authentication.event.AuthenticationSuccessEvent;
public class CustomAuthenticationSuccessListener implements ApplicationListener<AuthenticationSuccessEvent> {
@Override
public void onApplicationEvent(AuthenticationSuccessEvent event) {
System.out.println("User " + event.getAuthentication().getName() + " authenticated successfully.");
}
}
By doing this, you create an auditable record of authentication events, which can help in monitoring potential threats.
My Closing Thoughts on the Matter
Using Spring Security in Maven projects is undoubtedly a robust approach for securing Java applications. However, developers must pay close attention to common pitfalls, from managing dependencies and correctly configuring security filters to ensuring users' passwords are handled securely and implementing proper logging. By following best practices outlined in this guide, developers can lead their projects away from these pitfalls and toward secure, efficient applications.
For further reading on the implementation of Spring Security, you might find the official Spring Security Documentation and the Spring Guides extremely helpful.
By honing in on these common issues and understanding their solutions, you can elevate your Spring Security projects to a level of excellence, ensuring a safer environment for users and data. Happy coding!