Common Maven Pitfalls Every Java EE Developer Should Avoid
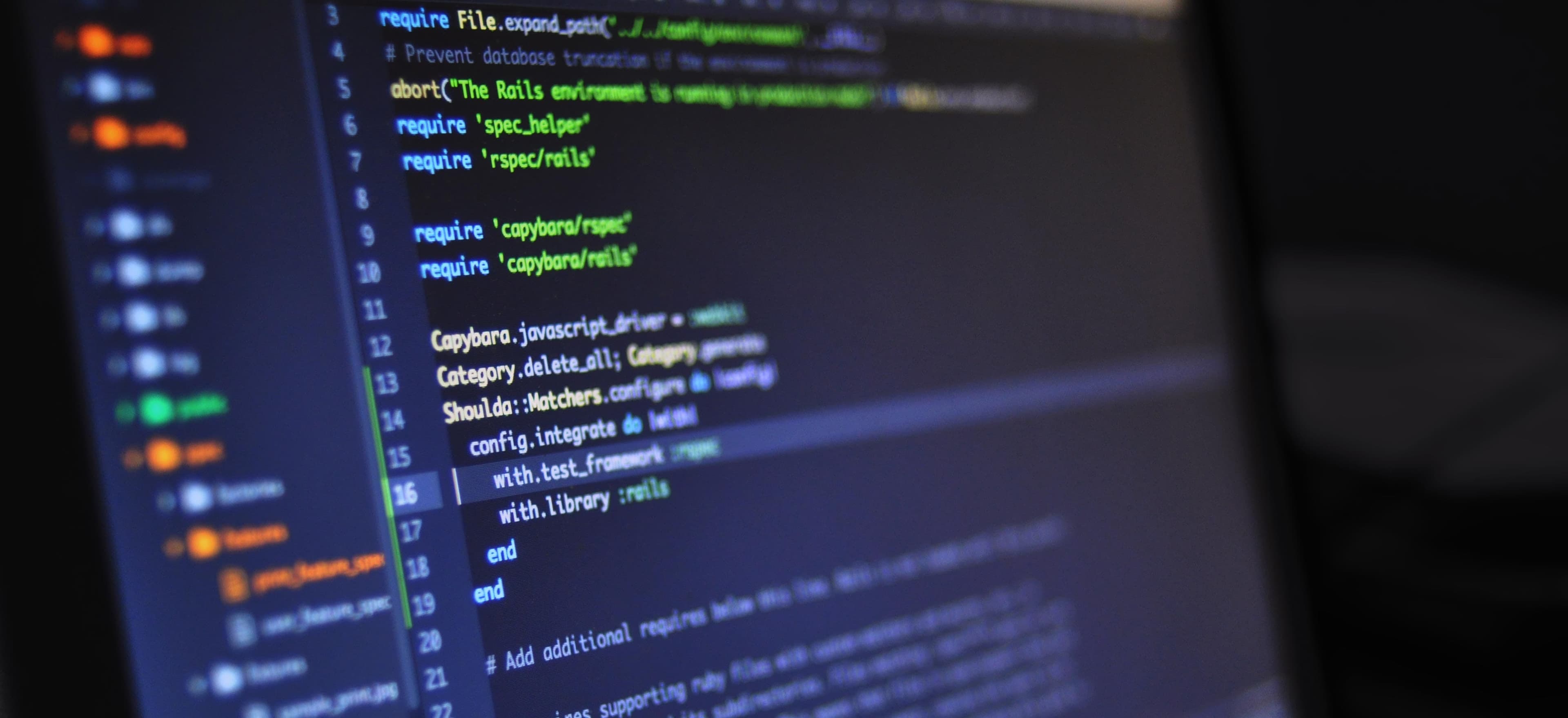
- Published on
Common Maven Pitfalls Every Java EE Developer Should Avoid
Maven is an essential tool for Java EE developers. It manages project builds, dependencies, and documentation, and it offers a standardized way to configure a project. However, for newcomers and even seasoned developers, Maven can introduce unexpected challenges. In this article, we'll discuss some common Maven pitfalls and how to avoid them, ensuring your Java EE development experience remains smooth and enjoyable.
1. Ignoring Dependency Scopes
One of the fundamental concepts in Maven is dependency management. Every dependency can have a scope, which defines how and when it is used. Ignoring these scopes can lead to bloated packages and runtime errors.
Example of Dependency Scoping
In your pom.xml
, you can define dependencies with different scopes:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.10</version>
<scope>compile</scope>
</dependency>
Here, the default scope is compile
. However, you might want to use test
when the library is only needed for testing.
Why It's Important
By using the correct scope, you minimize the risk of including unnecessary libraries in your production environment and avoid potential version conflicts.
Tip: Always review dependency scopes and consider what each library is necessary for to streamline your application.
2. Not Cleaning Up Unused Dependencies
As projects evolve, dependencies may become obsolete. Failing to remove unused dependencies can bloat your application and introduce security vulnerabilities.
Identifying Unused Dependencies
To find unneeded dependencies, utilize the maven-dependency-plugin
:
mvn dependency:analyze
This command analyzes your project and lists unused declared dependencies.
Why It's Important
Keeping your dependency management clean not only improves build performance but also reduces your attack vector in terms of vulnerabilities.
Tip: Regularly run the analyze command to maintain a lean project structure.
3. Mismanaging the pom.xml
Your pom.xml
is the heart of any Maven project. Misconfigurations can lead to build failures that can be tricky to troubleshoot.
Best Practices for Managing Your pom.xml
- Organize dependencies logically: Group related dependencies together and add comments for clarity.
- Use properties for versions: Define versions in a property section for easier updates.
For example:
<properties>
<spring.version>5.3.10</spring.version>
</properties>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>${spring.version}</version>
</dependency>
Why It's Important
Well-organized and structured pom.xml
helps in maintaining clarity, enabling easier collaborative development and upgrades.
Tip: Use version control tools like Git to track changes in your pom.xml
to ensure you can revert to a stable state when necessary.
4. Overusing the dependencyManagement
Section
The dependencyManagement
tag allows for centralized dependency version control but can lead to confusion when not used properly.
What You Should Know
When you declare dependencies in dependencyManagement
, they are not automatically included in your project. You'll still need to declare them in the dependencies section to use them.
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>5.3.10</version>
</dependency>
</dependencies>
</dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
</dependency>
</dependencies>
Why It's Important
Improper usage can lead to missing dependencies and confusing builds.
Tip: Only use dependencyManagement
when necessary. It’s typically great for multi-module projects or when you have to manage a set of libraries across multiple projects.
5. Neglecting the Maven Lifecycle
Maven has a defined lifecycle with distinct phases: These phases include validate, compile, test, package, verify, install, and deploy.
Understanding the Lifecycle Phases
Every phase represents a stage in the build process.
- compile: Compiles the source code.
- test: Runs the tests using a suitable testing framework.
- package: Packages the compiled code into a distributable format.
Utilizing the right phase can improve efficiency.
Why It's Important
Neglecting the lifecycle can lead to unnecessary build times or missed steps in the development process.
Tip: Familiarize yourself with lifecycle commands and plugin executions to harness Maven effectively.
6. Not Utilizing a Central Repository
Setting up a local Maven repository is easy, but relying solely on it without utilizing a central repository can limit what dependencies you can use.
Benefits of a Central Repository
Using a central repository allows for access to a wide array of libraries and tools available on Maven Central.
Why It's Important
Relying solely on local repositories can lead to a lack of updated libraries, eventually causing compatibility issues in larger projects.
Tip: Configure your settings.xml
to add mirrors of popular repositories for redundancy and wider access.
7. Ignoring Plugins
Maven's plugin system provides additional functionality for builds. Ignoring this can hinder your productivity.
Commonly Used Plugins
- maven-surefire-plugin: For executing tests.
- maven-compiler-plugin: To set source and target Java versions.
Here’s an example of configuring the compiler plugin in your pom.xml
:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>11</source>
<target>11</target>
</configuration>
</plugin>
</plugins>
</build>
Why It's Important
Leveraging plugins can automate and optimize aspects of your builds, making it more efficient.
Tip: Stay updated on popular plugins and consider automating tasks with profiles tailored for different environments.
Final Thoughts
Maven is an incredibly powerful tool for Java EE developers, but it comes with its pitfalls. By understanding dependency management, organizing your pom.xml
, leveraging the Maven lifecycle, and utilizing plugins effectively, you can significantly improve your development workflow. Regularly review your project structure and maintain good software hygiene to keep your project in top shape.
Ultimately, informed and mindful use of Maven will not only enhance your productivity as a developer but will also contribute to more robust and maintainable software.
Should you want to dive deeper into each topic, consider checking out Maven's official documentation for comprehensive insights. Happy coding!