Streamlining Configuration Management in Java EE Applications
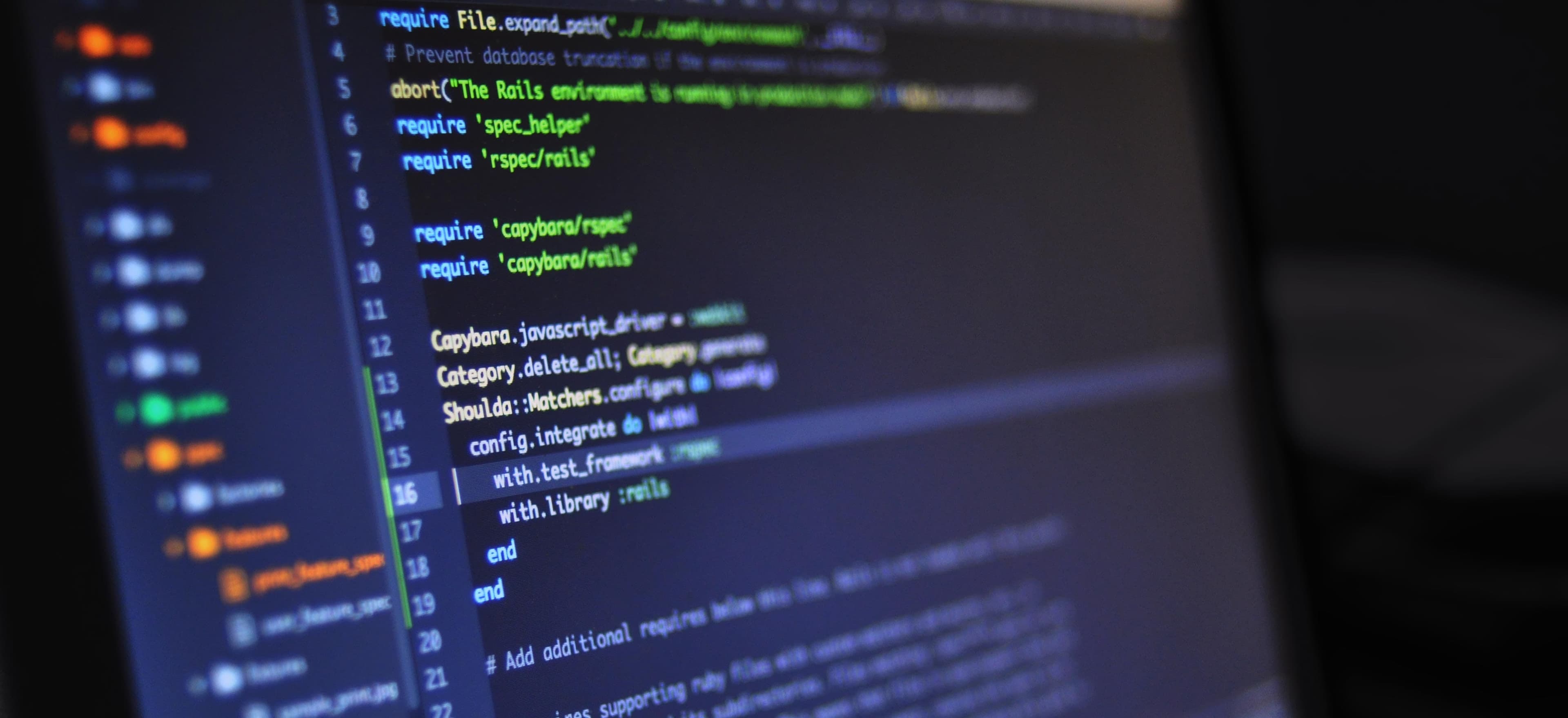
- Published on
Streamlining Configuration Management in Java EE Applications
In the fast-paced environment of software development, managing application configurations effectively is crucial. Incorrect or outdated configurations can lead to application downtime or failures. This blog post will explore how to streamline configuration management in Java EE applications using various strategies, tools, and best practices.
Understanding Configuration Management
Configuration management involves handling and documenting the configuration of systems and applications throughout their lifecycle. For Java EE applications, configuration management extends across multiple environments, including development, testing, and production. The goal is to ensure that the right configuration is applied at the right time and in the right context.
Why Configuration Management Matters
- Consistency: Proper configuration management ensures that applications behave consistently across different environments.
- Auditability: Tracking changes in configurations allows teams to audit deployments and identify the causes of issues.
- Automation: Streamlined configurations enable easier automation of deployment processes.
Configuring Java EE Applications
The Challenge
Java EE applications can be complex, composed of multiple modules and configurations ranging from data sources to application servers. Each environment might require different configurations, making it essential to address how to manage configs efficiently.
Traditional VS Modern Approaches
Traditionally, configuration settings were hard-coded or maintained as XML files. While functional, these methods often led to inflexibility and error-prone management. Modern frameworks and tools now offer more dynamic solutions:
- Environment Variables: These reduce hardcoding by retrieving configuration directly from the environment.
- Property Files: Java applications can read properties from files, allowing for externalized configurations.
- Configuration Servers: Tools like Spring Cloud Config can centralize property management for microservices.
Sample Code: Reading Configuration via Properties File
import java.io.IOException;
import java.io.InputStream;
import java.util.Properties;
public class ConfigurationManager {
private Properties properties;
public ConfigurationManager(String propertiesFile) {
properties = new Properties();
try (InputStream input = getClass().getClassLoader().getResourceAsStream(propertiesFile)) {
if (input == null) {
throw new IOException("Unable to find " + propertiesFile);
}
properties.load(input);
} catch (IOException exception) {
exception.printStackTrace(); // Handle the exception suitably
}
}
public String getProperty(String key) {
return properties.getProperty(key);
}
}
Commentary on the Code
- Why Use Properties Files?: The
ConfigurationManager
class allows you to externalize configurations to a properties file, enabling different environments to specify their own configurations without code changes. - Error Handling: The code implements basic error handling. In production, it's essential to log these errors effectively.
Best Practices for Configuration Management
1. Use Profiles
Many Java frameworks support the concept of profiles, allowing you to define different configurations for development, testing, and production environments. Spring, for example, supports profiles natively.
2. Use Environment Variables Wisely
Utilizing environment variables (via system properties) allows you to load configurations without exposing sensitive data directly in your codebase. This is highly recommended for database credentials or API keys.
3. Version Control for Configuration Files
Just as with code, version controlling configuration files ensures that changes are tracked, and you can roll back configurations easily if needed.
4. Secure Sensitive Information
Consider using tools like HashiCorp Vault or AWS Secrets Manager for managing sensitive information. This ensures that passwords, API keys, and other secrets are stored securely and are only accessible at runtime.
5. Centralized Configuration Management
As applications scale, consider centralizing configuration management to prevent distribution problems. Tools like Spring Cloud Config can fetch necessary configurations from a central server, reducing the overhead of managing multiple configuration files across microservices.
Sample Code: Spring Cloud Config Client
This example assumes you have a Spring Boot application that uses Spring Cloud Config.
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ConfigController {
@Value("${app.name}")
private String appName;
@GetMapping("/app-name")
public String getAppName() {
return appName; // Fetching the configuration from Spring Cloud Config
}
}
Commentary on Spring Example
- Why Use @Value Annotation?: This simplifies property injection by automatically binding configuration properties to class fields.
- Dynamic Configurations: If properties change in the Spring Cloud Config server, the application can refresh them dynamically, supporting zero-downtime deployments.
A Final Look
In conclusion, streamlining configuration management in Java EE applications can significantly improve software reliability and deployment processes. By adopting modern practices such as external property files, environment variables, and centralized configuration tools, you can enhance not only the agility but also the security and maintainability of your applications.
Additional Resources
- Learn more about Spring Profiles for managing environment-specific configurations.
- For a deeper dive into Spring Cloud Config, consider exploring its rich set of features for managing external configurations.
By following these strategies and best practices, you'll not only embrace modern configuration management but also master the intricacies of building robust Java EE applications. Happy coding!