Unlocking IntelliJ IDEA: Overcoming Common User Pitfalls
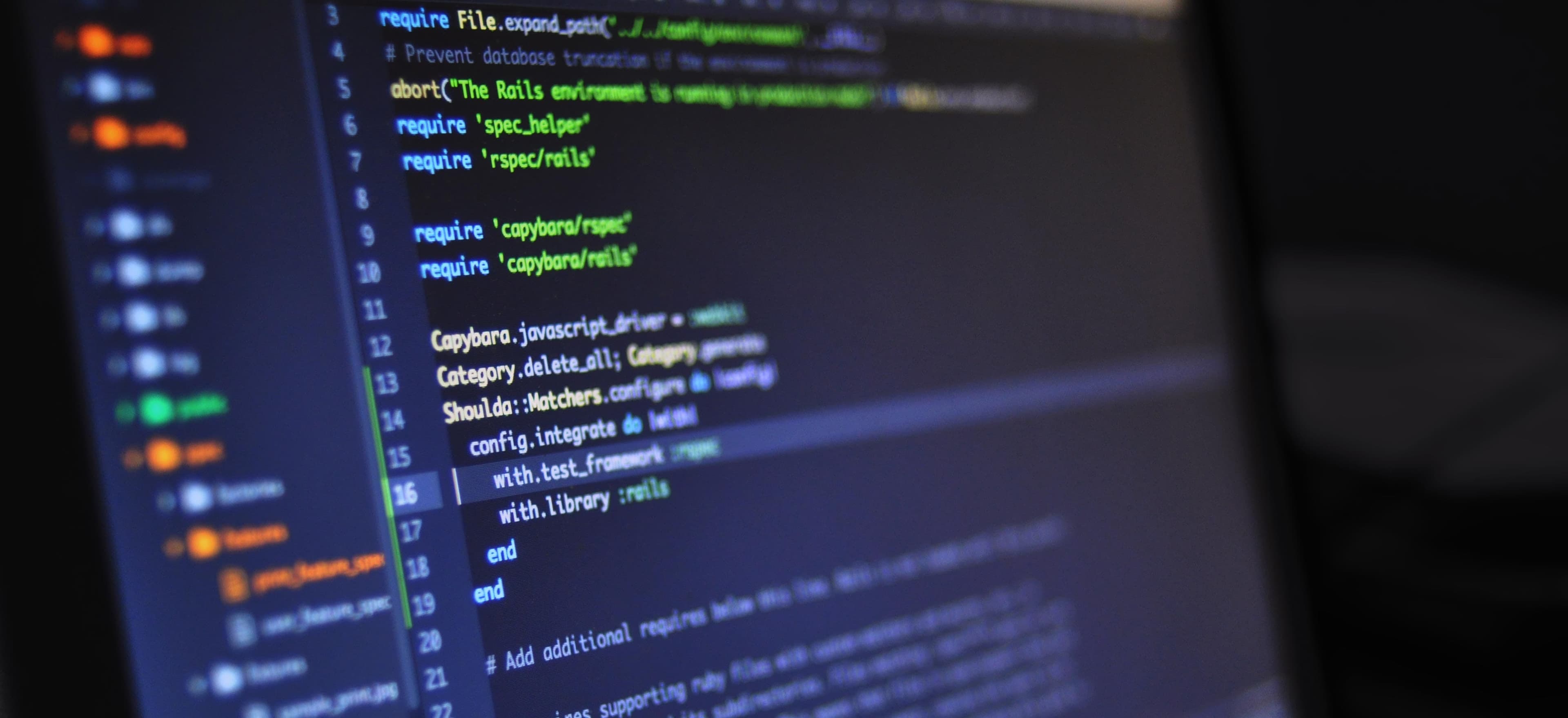
- Published on
Unlocking IntelliJ IDEA: Overcoming Common User Pitfalls
IntelliJ IDEA, developed by JetBrains, is one of the most popular integrated development environments (IDEs) available for Java development. Its powerful features and intelligent code assistance are designed to help developers write code faster and more efficiently. However, as with any complex tool, new users often face common challenges that can hinder their productivity. In this blog post, we will explore these pitfalls and provide practical solutions to enhance your experience with IntelliJ IDEA.
Table of Contents
- Understanding the User Interface
- Configuring IntelliJ IDEA for the First Time
- Common Code Editing Issues
- Debugging Like a Pro
- Version Control Integration
- Performance Optimization Tips
- Conclusion
1. Understanding the User Interface
When you first launch IntelliJ IDEA, the user interface can be overwhelming. The myriad of panels, toolbars, and menus can confuse even seasoned developers.
Key Components of the UI:
- Project View: Navigate your project structure efficiently.
- Editor Window: Where the magic happens—your code comes to life here.
- Tool Windows: Access essential tools like Terminal, Version Control, and more.
Better Navigation: Use keyboard shortcuts to save time. Press Ctrl + E
(or Cmd + E
on Mac) to open the recent file list.
Tip:
Spend some time familiarizing yourself with IntelliJ IDEA's keyboard shortcuts. Customizing these can significantly speed up your workflow.
2. Configuring IntelliJ IDEA for the First Time
A common pitfall arises when developers dive straight into coding without configuring their IDE. Setting up the environment properly is crucial.
Configuration Steps:
-
JSDK Setup: Ensure your Java SDK is set up correctly. Go to
File > Project Structure > SDKs
to add or modify your SDK. -
Plugins: IntelliJ offers a plethora of plugins. Check out the "Plugins" section to enhance functionality. Some recommendations include CheckStyle for code quality and Lombok for reducing boilerplate code.
Code Snippet: Setting Up a Simple Java Project
Here’s a minimal setup for your first Java class:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, IntelliJ IDEA!");
}
}
Why do this? Running this simple program can help you ensure that the editor, compiler, and runtime are all configured correctly.
3. Common Code Editing Issues
New users often struggle with common code editing challenges. One frequent complaint is slow code completion.
Solution: Optimize Code Completion
If your IDE feels sluggish, consider the following:
-
Increase Heap Size: Edit the
idea.vmoptions
file located in thebin
directory. Change the-Xms
and-Xmx
values to allocate more memory. -
Disable Unused Plugins: Too many active plugins can slow down code completion. Disable non-essential ones from the Plugins menu.
Code Snippet: Example of Quick Fix
Consider formatting code for better readability:
public class MySampleClass {
public void myMethod() {
// Ensuring proper indentation
if (true) {
System.out.println("Indentations matter for readability!");
}
}
}
Why? Properly formatted code is easier to navigate, reducing the cognitive load as you write and revise your code.
4. Debugging Like a Pro
Debugging can be intimidating. However, IntelliJ IDEA provides powerful debugging tools that simplify the process.
Steps for Effective Debugging:
- Set Breakpoints: Click in the left margin next to the line number to set a breakpoint.
- Start Debugger: Use the
Debug
icon (or pressShift + F9
) to run your application in debug mode. - Inspect Variables: During execution, hover over variables to see their current values.
Code Snippet: Using Breakpoints
Consider a scenario where you want to inspect an iterative process:
public class DebugExample {
public static void main(String[] args) {
for (int i = 0; i < 5; i++) {
// Set a breakpoint here to inspect the value of i
System.out.println("Current Value: " + i);
}
}
}
Why? Setting breakpoints allows you to pause execution and examine the state of your application, which is essential for identifying problems.
5. Version Control Integration
Managing source code without a version control system is risky. IntelliJ IDEA integrates with Git, Mercurial, and other version control tools seamlessly.
Setup Tips:
- Initialize a Repository: Use
VCS > Enable Version Control Integration
to set up version control in your project. - Commit Changes: Regularly commit your work using
Ctrl + K
(orCmd + K
on Mac). This ensures your progress is saved.
Code Snippet: Basic Git Commands
Here’s how you can quickly check the status, add files, and commit:
# Check the status
git status
# Add all changes
git add .
# Commit changes
git commit -m "Initial commit"
Why? Regular commits help keep a clean project history, allowing you to track changes and revert if necessary.
6. Performance Optimization Tips
Performance can be a deciding factor in your productivity. Here are a few tips to consider:
Tips for Optimization:
- Increase IDE Memory: As mentioned earlier, adjusting the VM options can significantly improve performance.
- Use a Lightweight Theme: Dark themes can strain resources, while default themes might perform better.
Code Snippet: Disable Unused Inspections
To customize inspections, navigate to File > Settings > Editor > Inspections
and uncheck inspections that you do not need. This can help improve IDE responsiveness.
<profile>
<option name="INHERITED" value="false" />
</profile>
Why? Reducing the number of active inspections minimizes the overhead, allowing IntelliJ IDEA to run more smoothly.
7. Conclusion
IntelliJ IDEA is a powerful tool that can greatly enhance your Java development experience. However, understanding the common pitfalls faced by new users is essential for unlocking its full potential.
By familiarizing yourself with the user interface, configuring your environment, addressing code editing issues, mastering debugging, integrating version control, and optimizing performance, you’re well on your way to becoming a proficient IntelliJ IDEA user.
If you're looking to dive deeper into IntelliJ IDEA for Java development, JetBrains offers extensive documentation that is worth exploring.
Whether you are a seasoned developer or just starting, overcoming these common pitfalls will ensure you maximize the capabilities of IntelliJ IDEA and improve your coding prowess. Happy coding!
Checkout our other articles