Common Pitfalls When Integrating jQGrid with Spring MVC
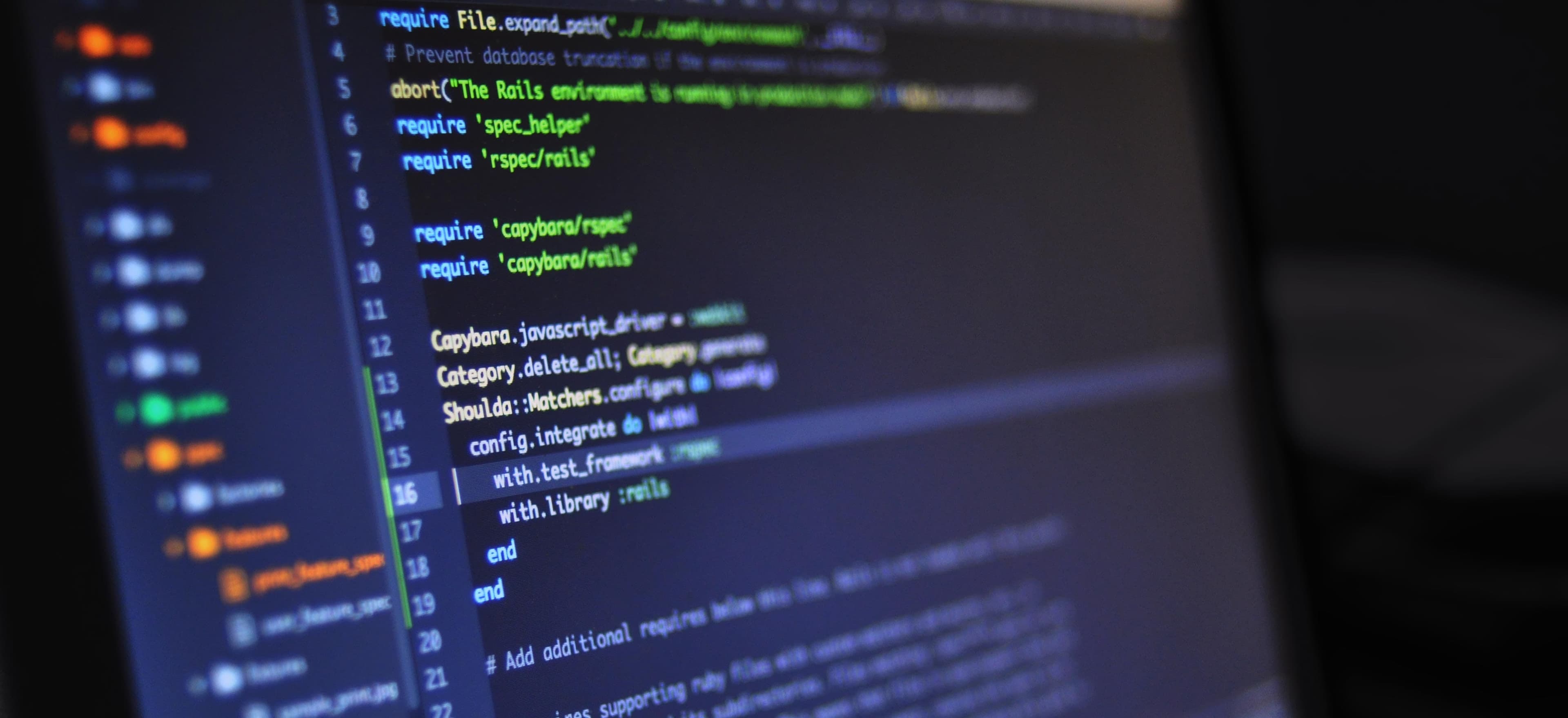
- Published on
Common Pitfalls When Integrating jQGrid with Spring MVC
Integrating jQGrid with Spring MVC can unleash enormous potential for your web applications. jQGrid, a powerful jQuery plugin for displaying tabular data, coupled with the robust MVC architecture of Spring, offers a highly responsive and dynamic user interface. However, like any integration, pitfalls lurk beneath the surface. This post discusses the common issues developers face when integrating jQGrid with Spring MVC and provides solutions and best practices to help mitigate those challenges.
Understanding jQGrid and Spring MVC
Before diving into the pitfalls, let's briefly understand how jQGrid and Spring MVC work together.
jQGrid is a table plugin that allows for features such as sorting, filtering, and paging, among others. It leverages AJAX to fetch data dynamically, which enhances the user experience.
Spring MVC is a web framework that follows the Model-View-Controller design pattern, making it intuitive and manageable. It can serve data to jQGrid through RESTful services, allowing for a neat separation of concerns.
With this overview in mind, let’s explore some common pitfalls.
Pitfall 1: Incorrect URL Mapping
One of the most common issues occurs when the URL used to fetch data in jQGrid does not map correctly to your Spring controller. This can lead to "404 Not Found" errors or unexpected behavior in your grid.
Solution: Verify URL Mapping
Ensure that the URL defined in your jQGrid matches the endpoint defined in your Spring controller. Here’s an example:
@Controller
public class MyController {
@RequestMapping(value = "/data", method = RequestMethod.GET)
@ResponseBody
public List<MyData> getData() {
// Retrieve and return data here
return myDataService.getAllData();
}
}
In your jQGrid setup, make sure you're using the correct URL:
$("#grid").jqGrid({
url: '/data',
datatype: 'json',
mtype: 'GET',
// Additional grid configurations
});
Pitfall 2: JSON Serialization Issues
Another frequent stumbling block comes from issues with JSON serialization. When Spring MVC returns data, it might not be properly structured for jQGrid, leading to grid rendering issues.
Solution: Use @JsonProperty
When returning a list of objects, you might need to annotate fields with @JsonProperty
to ensure proper serialization.
public class MyData {
@JsonProperty("id")
private Long id;
@JsonProperty("name")
private String name;
// Getters and Setters
}
This ensures that the keys used in the JSON response match the expectations of jQGrid.
Pitfall 3: Handling Pagination and Sorting Properly
Pagination and sorting can be tricky as jQGrid might send parameters that need proper handling on the server side.
Solution: Implement Custom Logic
You should implement custom logic in your controller to handle pagination and sorting correctly:
@RequestMapping(value = "/data", method = RequestMethod.GET)
@ResponseBody
public Map<String, Object> getData(@RequestParam int page,
@RequestParam int rows,
@RequestParam String sidx,
@RequestParam String sord) {
// Data retrieval logic
List<MyData> dataList = myDataService.getData(page, rows, sidx, sord);
Map<String, Object> response = new HashMap<>();
response.put("total", totalPages);
response.put("page", page);
response.put("records", totalRecords);
response.put("rows", dataList);
return response;
}
By dynamically calculating total records, total pages, and structuring your response accordingly, you prepare the grid to handle pagination smoothly.
Pitfall 4: AJAX Method Mismatch
jQGrid uses AJAX to interact with the server. If your Spring controller does not expose appropriate methods to handle AJAX requests, it can lead to method not allowed errors.
Solution: Verify HTTP Methods
Check that you've allowed the necessary HTTP methods in your controller, especially when dealing with data edits (CREATE, UPDATE, DELETE):
@PostMapping("/data")
public ResponseEntity<MyData> createData(@RequestBody MyData newData) {
MyData createdData = myDataService.createData(newData);
return new ResponseEntity<>(createdData, HttpStatus.CREATED);
}
Pitfall 5: JavaScript and jQGrid Configuration Issues
Misconfigurations in the jQGrid initialization can cause it not to display data or work as expected.
Solution: Review your Grid Initialization
Make sure that you’ve properly set the necessary options during the initialization of jQGrid:
$("#grid").jqGrid({
datatype: 'json',
colModel: [
{ label: 'ID', name: 'id', width: 75 },
{ label: 'Name', name: 'name', width: 150 },
// Additional columns
],
height: 'auto',
viewrecords: true,
rowNum: 10,
pager: "#pager"
});
Pitfall 6: CSS and Theme Compatibility
Sometimes, jQGrid does not render as expected due to CSS conflicts. Not all themes are compatible with jQGrid.
Solution: Choose Compatible Themes
Use jQGrid themes that are well-supported and compatible with your overall site design. Check out the jQGrid Documentation for theme selection assistance.
A Final Look
Integrating jQGrid with Spring MVC can significantly enhance your web application but comes with its own set of challenges. By being aware of these common pitfalls and implementing the solutions discussed, you can streamline your integration process for a more efficient workflow.
Further Reading
For more information and advanced configurations, check out:
- The jQGrid Documentation
- Spring MVC official documentation on JSON handling
Happy coding! Whether you're enhancing user interfaces with jQGrid or building robust RESTful services with Spring MVC, each project brings new learning opportunities. Stay curious, and keep building!
Checkout our other articles