Mastering Functional Programming: Common Pitfalls to Avoid
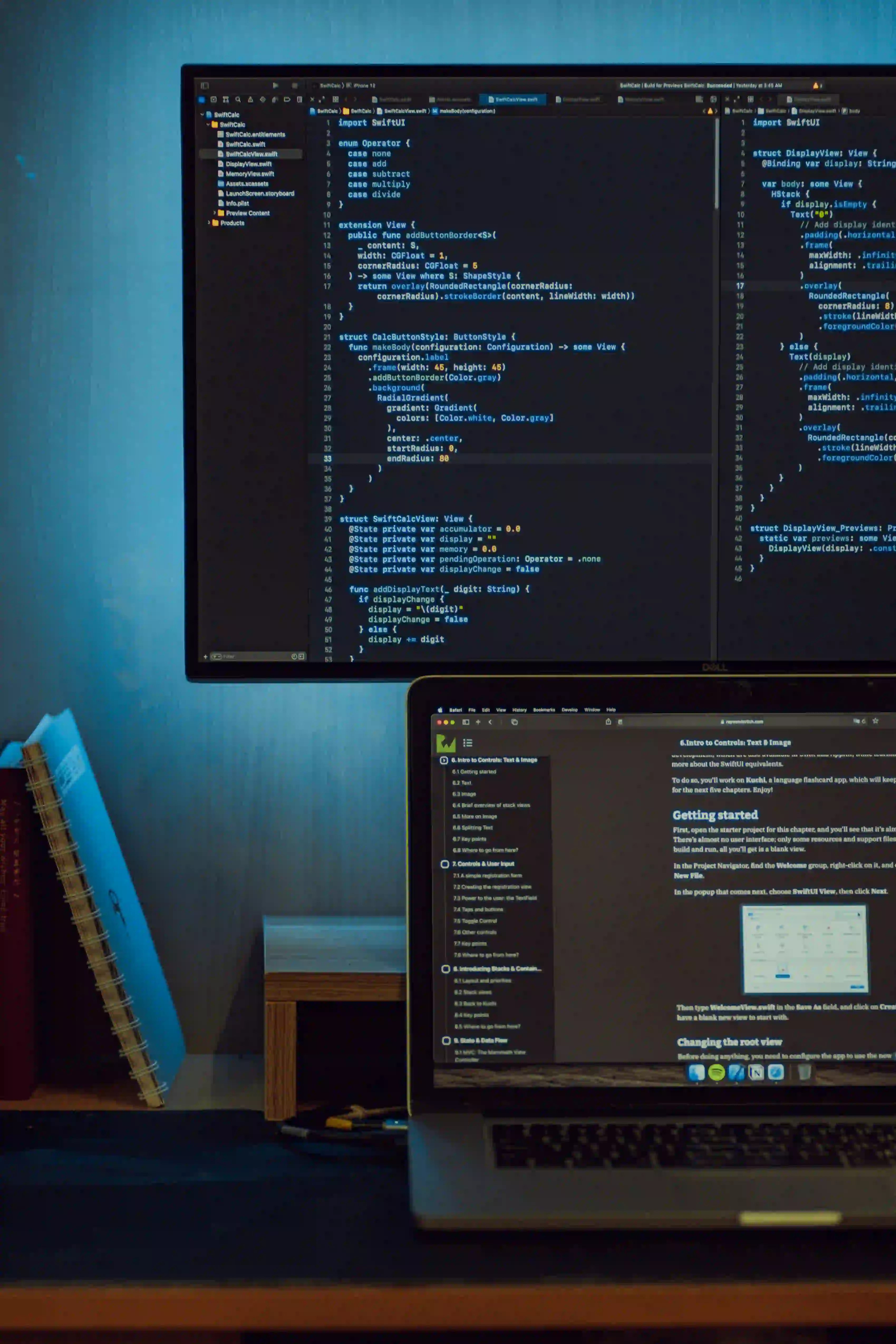
Mastering Functional Programming: Common Pitfalls to Avoid
Functional programming has evolved from a niche theoretical concept to a mainstream paradigm embraced by numerous programming languages, including Java. While the functional style promotes clearer and more predictable code, it is not free from its own set of challenges. Understanding and avoiding common pitfalls can significantly enhance your coding experience and improve code maintainability.
In this blog post, we will delve into functional programming in Java, identify classic mistakes, and arm you with best practices to circumvent them. This discussion is complemented with code snippets to clarify concepts and enable practical understanding.
What is Functional Programming?
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state or mutable data. It emphasizes:
- First-class functions: Functions can be treated like any other variable.
- Pure functions: Output solely depends on provided arguments, eliminating side effects.
- Higher-order functions: Functions that can take other functions as arguments or return them.
Java introduced functional programming features in version 8, primarily through lambdas and streams.
Common Pitfalls in Functional Programming
1. Ignoring Immutability
Overview
One of the central tenets of functional programming is immutability. By not changing existing data and creating new copies instead, you reduce side effects. Yet, many developers still opt for mutable data structures, leading to unintentional bugs.
Example
import java.util.ArrayList;
import java.util.List;
public class MutableListExample {
public static void main(String[] args) {
List<String> names = new ArrayList<>();
names.add("John");
// Mutating the names list
names.add("Jane");
System.out.println(names);
}
}
Why This is a Pitfall
In the example above, the list is mutable. While it may work for a small application, in larger, concurrent applications, mutability can lead to unpredictable behaviors and bugs. For a more functional approach, consider using immutable lists.
Best Practice
Use the List.of()
method available in Java 9 to create immutable lists.
import java.util.List;
public class ImmutableListExample {
public static void main(String[] args) {
List<String> names = List.of("John", "Jane"); // Immutable list
// Attempting to modify the list throws UnsupportedOperationException
System.out.println(names);
}
}
2. Overusing Lambdas
Overview
Lambdas are a powerful feature of functional programming in Java. However, overusing them can lead to confusing, unreadable code, particularly when trying to cram complex logic into a single expression.
Example
import java.util.Arrays;
import java.util.List;
public class OverusingLambdas {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", "Jane", "Doe");
// Overly complex lambda
names.forEach(name -> {
if (name.startsWith("J") && name.length() > 3) {
System.out.println(name.toUpperCase());
}
});
}
}
Why This is a Pitfall
The lambda expression in the example above is quite complex, making it hard to understand. It becomes difficult to maintain and test.
Best Practice
Refactor complex lambdas into named methods for better readability.
import java.util.Arrays;
import java.util.List;
public class ReadableLambdas {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", "Jane", "Doe");
names.forEach(ReadableLambdas::printIfValid);
}
private static void printIfValid(String name) {
if (name.startsWith("J") && name.length() > 3) {
System.out.println(name.toUpperCase());
}
}
}
3. Misusing Streams
Overview
Java streams are a powerful abstraction that allows for functional-style operations on sequences of elements. However, misusing them—such as ignoring their lazy nature—can lead to performance issues and misunderstandings.
Example
import java.util.Arrays;
import java.util.List;
public class StreamMisuseExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", "Jane", "Doe");
// Using stream but making unnecessary intermediate operations
long count = names.stream()
.filter(name -> name.startsWith("J"))
.map(String::toUpperCase) // Irrelevant intermediate
.count(); // Terminal operation
System.out.println(count);
}
}
Why This is a Pitfall
The map
operation is unnecessary before the count
. It creates an intermediate result that is not used, impacting performance as the entire stream is processed.
Best Practice
Minimize the number of intermediate operations and use short-circuiting methods where applicable.
import java.util.Arrays;
import java.util.List;
public class EfficientStreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", "Jane", "Doe");
// Simplified stream usage
long count = names.stream()
.filter(name -> name.startsWith("J"))
.count(); // Directly count filtered results
System.out.println(count);
}
}
4. Not Leveraging Optional Effectively
Overview
Java Optional
is a powerful tool to avoid null references and facilitate functional programming. However, it is still commonly misused.
Example
import java.util.Optional;
public class OptionalMisuseExample {
public static void main(String[] args) {
Optional<String> optionalName = Optional.empty();
// Overcomplicated null check
String name = optionalName.isPresent() ? optionalName.get() : "Default Name";
System.out.println(name);
}
}
Why This is a Pitfall
This example unnecessarily complicates how you handle possible absence using Optional
. It breaks the intention of Optional
, which is to signal that a value may or may not be present.
Best Practice
Use built-in methods to handle absence of values effectively without verbose null checks.
import java.util.Optional;
public class EfficientOptionalUsage {
public static void main(String[] args) {
Optional<String> optionalName = Optional.empty();
String name = optionalName.orElse("Default Name"); // Cleaner usage
System.out.println(name);
}
}
5. Forgetting to Unit Test Functional Code
Overview
Unit testing is crucial regardless of the programming paradigm used. However, functional programming can lead developers to overlook thorough testing, especially when using complex streams and lambdas.
Why This is a Pitfall
Without proper tests, edge cases may evade detection, leading to unanticipated behavior during runtime.
Best Practice
Always write comprehensive tests for your functional code. Use JUnit or any other testing framework to create unit tests for your functions.
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
import java.util.Optional;
class OptionalTest {
@Test
void testOptionalWithValue() {
Optional<String> optionalName = Optional.of("Jane");
assertEquals("Jane", optionalName.orElse("Default Name"));
}
@Test
void testOptionalWithoutValue() {
Optional<String> optionalName = Optional.empty();
assertEquals("Default Name", optionalName.orElse("Default Name"));
}
}
Closing the Chapter
Functional programming in Java offers a robust framework for building clean, maintainable code. However, it comes with its own challenges. By understanding the common pitfalls, developers can better leverage functional programming constructs while ensuring the durability and readability of their code.
By focusing on immutability, avoiding excessive lambda usage, correctly utilizing streams, making effective use of Optional
, and emphasizing comprehensive testing, developers can navigate the landscape of functional programming with greater confidence.
For an in-depth exploration of more advanced concepts in functional programming, consider consulting Functional Programming in Java.
With every new paradigm, practice makes perfect. Embrace functional programming while being attentive to its nuances. And remember: the aim is not merely to get results but to achieve them in a way that is both elegant and maintainable. Happy coding!