Troubleshooting Common Issues in Spring Boot STS Setup
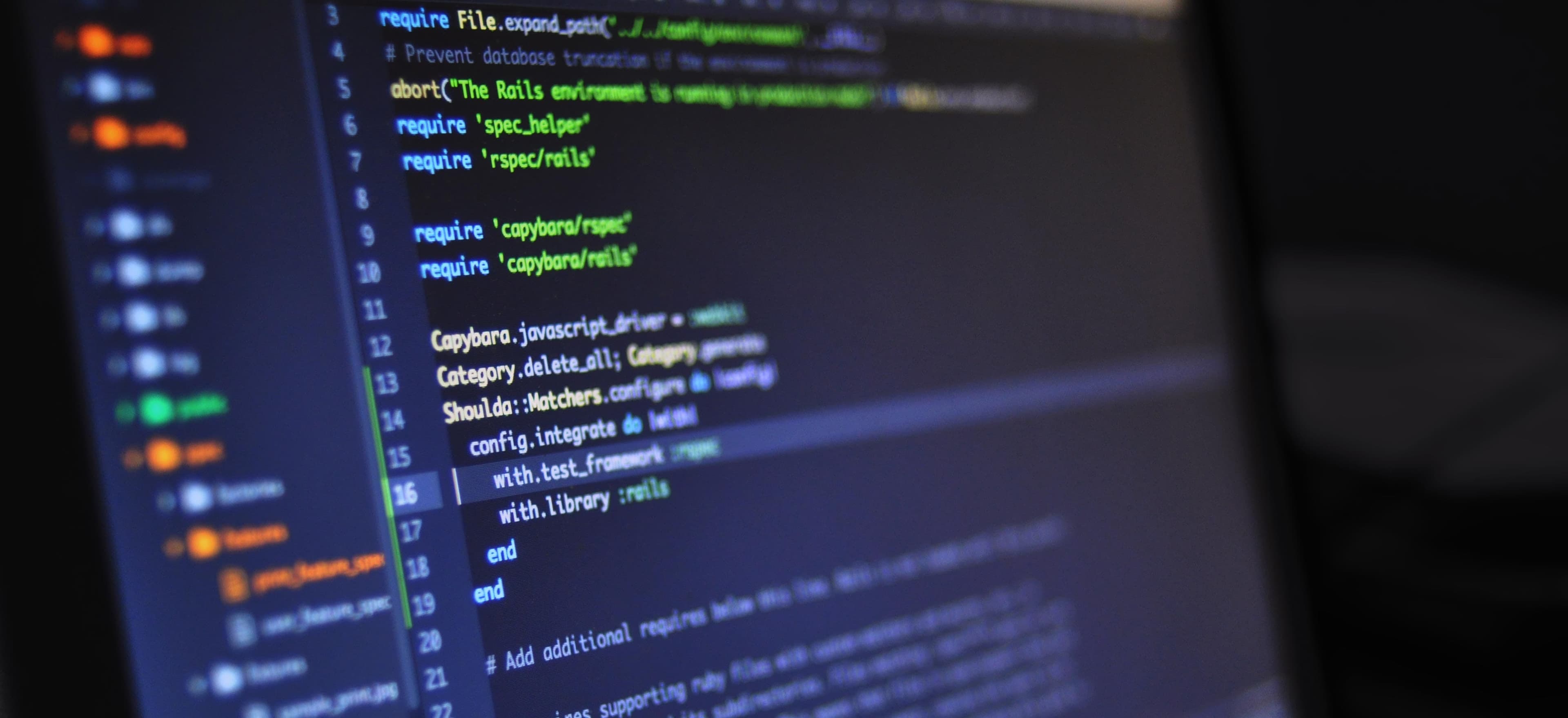
- Published on
Troubleshooting Common Issues in Spring Boot STS Setup
Setting up a Spring Boot application can be a smooth experience if you have everything configured correctly. However, like any other development environment, you might encounter issues while setting up Spring Tool Suite (STS) for Spring Boot development. This blog post will guide you through some common issues and their solutions, focusing on practical approaches to troubleshooting.
Table of Contents
- What is Spring Tool Suite?
- Setting Up Spring Tool Suite
- Common Issues in Spring Boot STS Setup
- Conclusion
What is Spring Tool Suite?
Spring Tool Suite (STS) is an integrated development environment (IDE) specifically designed for developing Spring applications. Built on Eclipse, STS comes loaded with features that enhance development productivity, including:
- Built-in support for Spring Boot.
- Enhanced code assistance and navigation.
- Easy integration with various databases and cloud services.
For more details, visit the official Spring Tool Suite page.
Setting Up Spring Tool Suite
Before delving into troubleshooting, ensure you have the basic setup completed:
- Download STS: Download the latest version of STS from the Spring website.
- Install Java Development Kit (JDK): Make sure JDK is installed on your system. You can download it from Oracle or AdoptOpenJDK.
- Install Maven: STS supports Maven for project management. If it is not available, download it from the Apache Maven website.
- Configure STS: After installation, configure your JDK and Maven settings in STS preferences.
Common Issues in Spring Boot STS Setup
Let’s look at the most frequent problems and their potential solutions.
Java Installation Issues
Symptoms:
- STS fails to start.
- Errors related to JVM not found.
Solution:
-
Check JAVA_HOME: Ensure your JAVA_HOME environment variable points to your JDK installation directory. You can check this in the terminal:
echo $JAVA_HOME
If it is not set, you can set it using:
export JAVA_HOME=/path/to/jdk
-
Check the JDK version: Spring Boot requires at least JDK 8. In your terminal, check the installed JDK version using:
java -version
-
Configure STS to Point to JDK:
- Go to Window > Preferences > Java > Installed JREs.
- Ensure that the JDK is selected and not just a JRE.
Maven Issues
Symptoms:
- Errors related to Maven dependencies.
- Build failures.
Solution:
-
Update Maven Settings: Navigate to Window > Preferences > Maven > User Settings and configure it to point to your settings.xml file, usually found in your home directory under
.m2
folder. -
Force Update of Snapshots/Releases: Right-click on your project, select Maven > Update Project, and check the option Force Update of Snapshots/Releases.
-
Make sure Maven is installed: Verify that Maven is correctly installed by running:
mvn -v
Spring Initializr Problems
Symptoms:
- Unable to create a new Spring Boot project.
- STS hangs or crashes when accessing Initializr.
Solution:
-
Check Network Connectivity: The Spring Initializr requires internet access to fetch dependencies. Ensure your IDE can access the internet.
-
Use Local Initializr: If the online version is problematic, consider using local Spring Initializr alternatives such as Spring CLI.
-
Clear Cache: STS might need a cache clear. You can clear the cache by following:
- File > Switch Workspace > Other… and then select a different workspace.
Dependency Resolution Failures
Symptoms:
- Missing dependencies after project creation.
- Errors in
pom.xml
file.
Solution:
-
Check pom.xml: Ensure that all necessary dependencies are present. Here’s an example snippet of a typical Spring Boot pom.xml:
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies>
Each dependency should point to the correct version as specified in the Spring Boot documentation.
-
Check for Conflicts: Look for dependency version conflicts. You can analyze the dependency tree by running:
mvn dependency:tree
Database Connection Issues
Symptoms:
- Exceptions during application startup.
- Application unable to connect to the database.
Solution:
-
Configure Database Properties: Ensure your
application.properties
orapplication.yml
file has the correct database configuration:spring.datasource.url=jdbc:mysql://localhost:3306/yourdb spring.datasource.username=root spring.datasource.password=yourpassword spring.jpa.hibernate.ddl-auto=update
-
Check Database Status: Ensure your database service is running and accessible.
-
Expected Driver Dependency: If using MySQL, for instance, ensure the driver dependency is included in your
pom.xml
:<dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <scope>runtime</scope> </dependency>
In Conclusion, Here is What Matters
Setting up Spring Boot with STS can be straightforward, but it might come with its fair share of challenges. By systematically troubleshooting common issues like Java installation failures, Maven-related problems, and database connection hiccups, you can ensure a smoother development experience.
Always remember to check the configuration files, dependencies, and the environment settings thoroughly. By understanding the root cause of these common issues, you'll not only set up your Spring Boot application but also gain deeper insights into your development environment.
For more advanced troubleshooting techniques and community support, check out the Spring Boot GitHub repository or visit Stack Overflow for a wealth of community-contributed solutions. Happy coding!
Checkout our other articles