Jumpstart Your Development: Common Pitfalls in Spring Roo
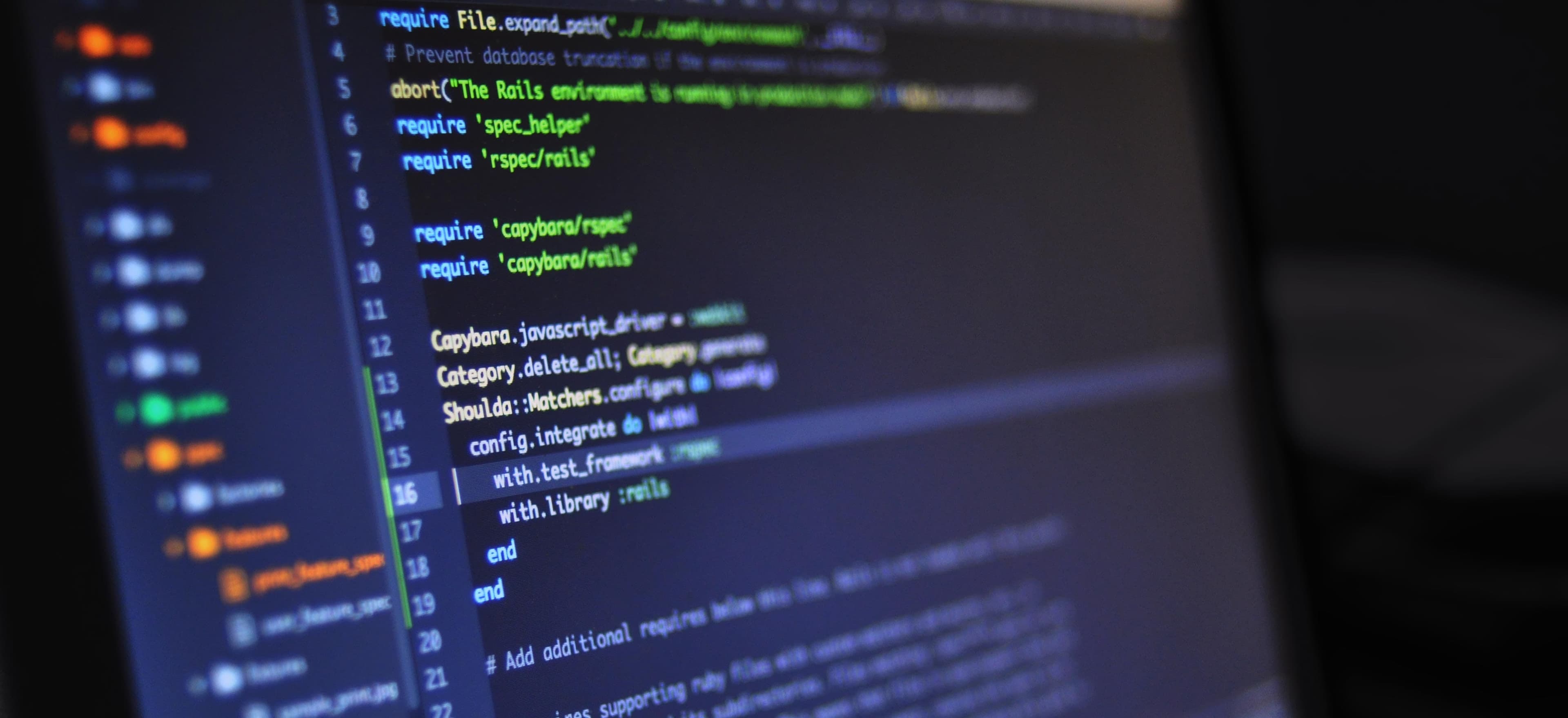
- Published on
Jumpstart Your Development: Common Pitfalls in Spring Roo
Spring Roo is a powerful tool that allows Java developers to rapidly create enterprise applications using a command line interface. While it can significantly accelerate development, it is not without its complexities. In this post, we’ll explore common pitfalls that developers face when using Spring Roo, share effective strategies to avoid them, and provide examples to illustrate key concepts.
What is Spring Roo?
Spring Roo is an open-source tool designed to make it easier and faster to build Java applications. By leveraging a software development approach that emphasizes convention over configuration, it enables developers to focus on coding rather than dealing with boilerplate tasks. With Spring Roo, you can auto-generate code, manage various modules, and easily integrate different technologies like Spring MVC, Spring Data, and database systems.
The Benefits of Spring Roo
Before diving into the pitfalls, it’s important to acknowledge the benefits Spring Roo offers:
- Rapid Application Development: Automatic code generation reduces time spent on repetitive tasks.
- Easy Integration: Compatibility with various Spring projects simplifies integration processes.
- Active Community Support: A strong community means more resources and quicker solutions to issues.
Common Pitfalls in Spring Roo
Despite its advantages, there are some pitfalls that developers must navigate effectively:
1. Over-Reliance on the Tool
It’s tempting to let Spring Roo handle everything, but this can lead to a disconnect between understanding the generated code and actual implementation.
For example, when you use Spring Roo to create an entity, the tool generates several classes for you. While this is efficient, it is crucial to inspect and understand them.
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@NotNull
private String username;
@NotNull
@Email
private String email;
// Getters and setters
}
Why This Matters: Without a clear understanding of the structure and lifecycle of objects, you may struggle when it comes to modifications or custom behaviors down the line.
Tip: Always review and analyze the generated code. Make adjustments as needed to align with your specific business logic.
2. Ignoring Spring Roo Documentation
Many developers skip reading through the Spring Roo documentation or fail to leverage its extensive offerings.
The Consequence: Without proper knowledge, you may miss out on valuable commands and features that can enhance your development process.
For instance: Using the Roo shell, you can generate a complete web application skeleton with just a few commands:
roo> project setup --projectName demo
roo> entity jpa --class ~.domain.User
By not being familiar with these commands, you could waste time on manual setups that Roo can automate.
Tip: Set aside time to read through the documentation. Take note of key commands, features, and configurations.
3. Neglecting Testing
When code is generated automatically, developers can sometimes overlook the importance of testing. Relying solely on Spring Roo's generated tests (or lack thereof) can lead to problems.
For instance, while it does generate basic tests for your entities, they might not cover various edge cases or integration points. Thus, do not shy away from enhancing the testing suite:
@RunWith(SpringRunner.class)
@SpringBootTest
public class UserServiceTests {
@Autowired
private UserService userService;
@Test
public void testUserCreation() {
User user = new User("test@example.com", "testUser");
User createdUser = userService.createUser(user);
assertNotNull(createdUser.getId());
}
}
Why This Matters: Testing is crucial for ensuring application reliability. Skipping it could lead to undetected bugs and system failures post-deployment.
Tip: Establish a testing strategy early in your development. Use JUnit and Mockito to create comprehensive unit and integration tests.
4. Failure to Keep the Dependencies Up-to-Date
Spring Roo is frequently updated. If you're not paying attention, you could miss important upgrades such as new libraries or security patches.
The consequences of outdated dependencies include missing out on performance improvements and missed security fixes.
Tip: Regularly check for updates on the Spring Roo GitHub page or by using build tools like Maven or Gradle.
5. Ignoring Customization Opportunities
Spring Roo makes it easy to generate the scaffolding you need, but it also has extensive customization options that some developers overlook. Customize your Roo projects to meet specific business requirements or enhance usability and performance.
For example, you can create custom aspects:
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Executing: " + joinPoint.getSignature().getName());
}
}
Why This Matters: Customization allows your application to stand out and perform optimally as per your unique requirements.
Tip: Explore Roo’s available options for customization, such as defining custom services, controllers, and repository structures.
6. Not Utilizing Spring Roo Features Fully
Spring Roo offers many features meant to simplify everyday tasks. Not leveraging these features limits your productivity.
For instance, you can use Roo's web-based interface feature, which is very powerful for new developers learning the framework.
roo> web mvc setup
This command creates a Spring MVC structure complete with models, views, and controllers.
Tip: Attend Spring Roo workshops or watch tutorials online to familiarize yourself with underutilized features that can enhance your workflow.
Wrapping Up
Spring Roo can transform the way you develop Java applications, but it is crucial to be mindful of its inherent pitfalls. By understanding the tool, leveraging documentation, prioritizing testing, keeping dependencies updated, customizing your applications, and fully utilizing Spring Roo features, you can maximize your productivity and build robust applications with confidence.
Additional Resources
By staying aware of these considerations, you can avoid commonplace mistakes and streamline your development process with Spring Roo at every turn. Happy coding!
Checkout our other articles