Troubleshooting Location Issues in Your Weather App
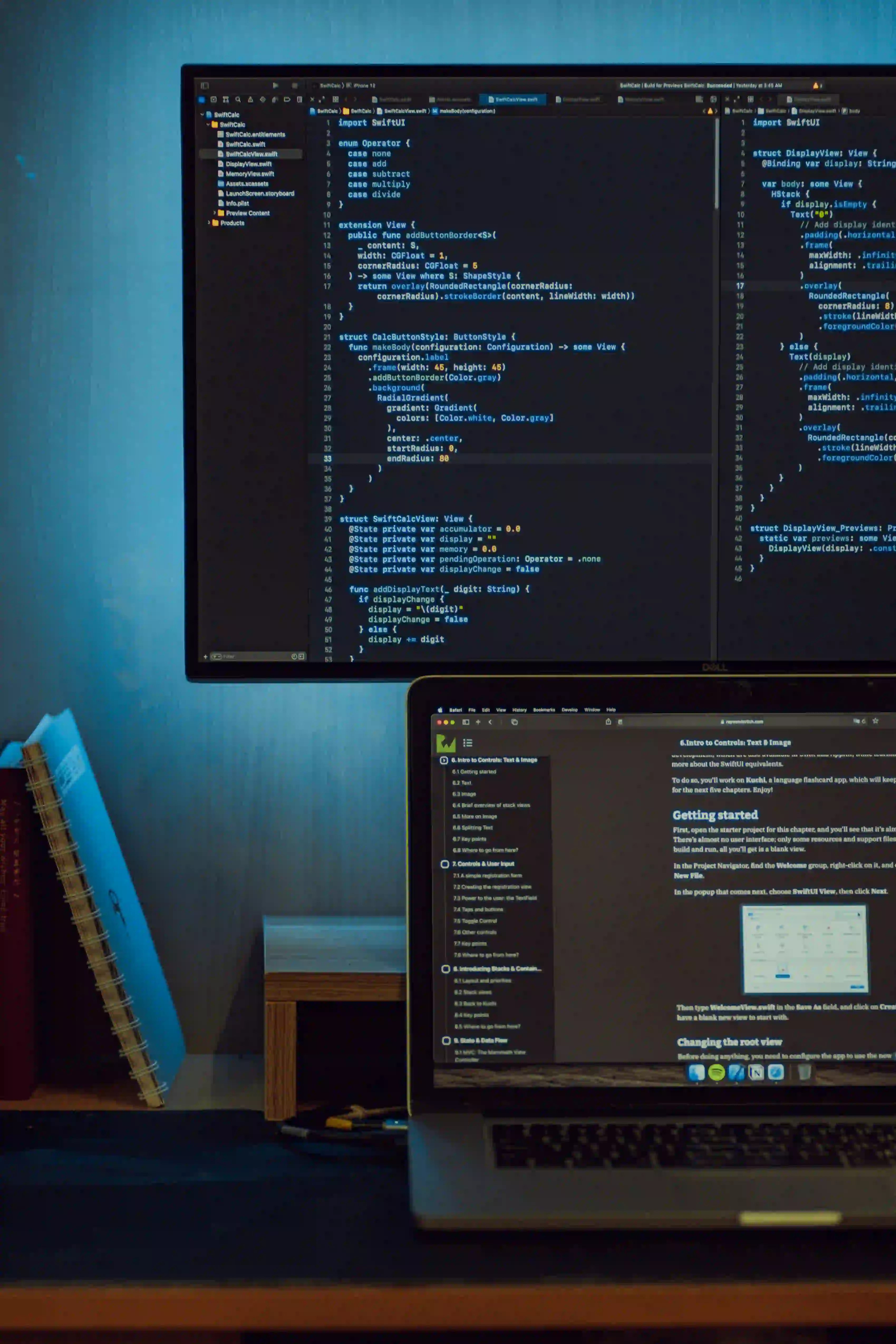
Troubleshooting Location Issues in Your Weather App
Creating a weather app can be an exciting endeavor. However, it can also come with its own set of challenges, especially when it comes to location services. This post will guide you through the common location issues you might encounter while developing your weather app, and provide step-by-step solutions to debug them effectively.
Understanding Location Services
Location services are vital for any weather app. They retrieve the current geographical position of the user, which is used to display weather data for that specific location. The main ways you can get a user's location include GPS, Wi-Fi positioning, and cell tower triangulation.
Why Location Matters
Your users need accurate and timely information. A slight error in their location could lead to misreporting of weather conditions, which could ultimately lead to a loss of trust in your application.
Common Issues with Location Services
-
User Permission Denied
One of the most common issues is the failure to obtain user permissions for location access. This requires explicit consent, and if not handled correctly, your app may fail.
-
GPS Signal Unavailable
GPS services can be hindered by various factors such as tall buildings, trees, and even bad weather. This could result in inaccuracies.
-
Network Limitations
When using Wi-Fi or cellular data for location services, network reliability plays a crucial role. Users on a poor network might experience delays or inaccuracies.
-
Location Accuracy Settings
Different devices and operating systems have different default settings regarding how precisely they gather location data.
-
Outdated Libraries or APIs
If you are using outdated libraries or APIs to get location data, you may encounter unwanted bugs.
Implementing Location Services in Your App
Let’s delve into coding a basic example to implement location services effectively.
Step 1: Requesting User Permissions
Java Code Snippet
import android.Manifest;
import android.content.pm.PackageManager;
import androidx.annotation.NonNull;
import androidx.core.app.ActivityCompat;
import androidx.fragment.app.FragmentActivity;
import android.os.Bundle;
public class MainActivity extends FragmentActivity {
private static final int LOCATION_PERMISSION_REQUEST_CODE = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
requestLocationPermission();
}
private void requestLocationPermission() {
if (ActivityCompat.checkSelfPermission(this,
Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(this,
new String[]{Manifest.permission.ACCESS_FINE_LOCATION},
LOCATION_PERMISSION_REQUEST_CODE);
}
}
@Override
public void onRequestPermissionsResult(int requestCode,
@NonNull String[] permissions,
@NonNull int[] grantResults) {
if (requestCode == LOCATION_PERMISSION_REQUEST_CODE) {
if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
// Permission granted, get the location
} else {
// Permission denied
}
}
}
}
Commentary
This code snippet ensures you explicitly request location permission from users. The requestLocationPermission()
method checks if the permission has been granted. If not, it requests permission. Handling permissions correctly is crucial to providing a robust user experience.
Step 2: Getting the User’s Location
Once you have the necessary permissions in place, you can retrieve the user's location.
Java Code Snippet
import android.location.Location;
import android.location.LocationListener;
import android.location.LocationManager;
public class MainActivity extends FragmentActivity {
private LocationManager locationManager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
locationManager = (LocationManager) getSystemService(LOCATION_SERVICE);
getUserLocation();
}
private void getUserLocation() {
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
return;
}
locationManager.requestLocationUpdates(LocationManager.GPS_PROVIDER, 0, 0, new LocationListener() {
@Override
public void onLocationChanged(Location location) {
// Handle the location update
double latitude = location.getLatitude();
double longitude = location.getLongitude();
// Send the coordinates to your API to fetch the weather data
}
// Other overridden methods such as onStatusChanged, onProviderEnabled, and onProviderDisabled can go here
});
}
}
Commentary
This snippet listens for location updates. The getUserLocation()
function checks for necessary permissions and requests location updates through LocationManager
. When the location changes, it retrieves the latitude and longitude, which are critical for fetching weather data.
Best Practices for Troubleshooting Location Issues
-
Debugging Permissions Issues
Always check the permission status before attempting to access the location. This can be done using methods like
checkSelfPermission()
andrequestPermissions()
. -
Implement Fallback Strategies
If GPS is unavailable, consider using network-based location by switching to
LocationManager.NETWORK_PROVIDER
. This can improve reliability. -
Handling Location Settings
Direct users to check their location settings. If they have set their device to a battery-saving mode, the accuracy of location data may also be compromised.
Example for Network-Based Location
locationManager.requestLocationUpdates(LocationManager.NETWORK_PROVIDER, 0, 0, new LocationListener() {
@Override
public void onLocationChanged(Location location) {
// Handle network location updates
double latitude = location.getLatitude();
double longitude = location.getLongitude();
}
});
-
Testing in Different Environments
Always test your app in various locations and network conditions. Use services like Google Maps to verify that location data retrieval is working correctly.
-
Utilizing Error Handlers
Implement error handlers that provide feedback to the user. For instance, if the app fails to determine location, inform users and suggest troubleshooting steps.
Closing Remarks
In conclusion, troubleshooting location issues in your weather app is a multifaceted challenge that involves attention to detail and rigorous testing. By correctly implementing location services, managing permissions efficiently, and adhering to best practices, you can significantly enhance user satisfaction.
If you’re interested in deepening your knowledge about location services in mobile development, I recommend checking the Android Developer Guide on Location.
Your app can only be as reliable as how well you manage the underlying services. So take the time to not only fix issues but also to continuously optimize your approach to location queries. Happy coding!