Overcoming Challenges in Spring Quartz Custom Integration
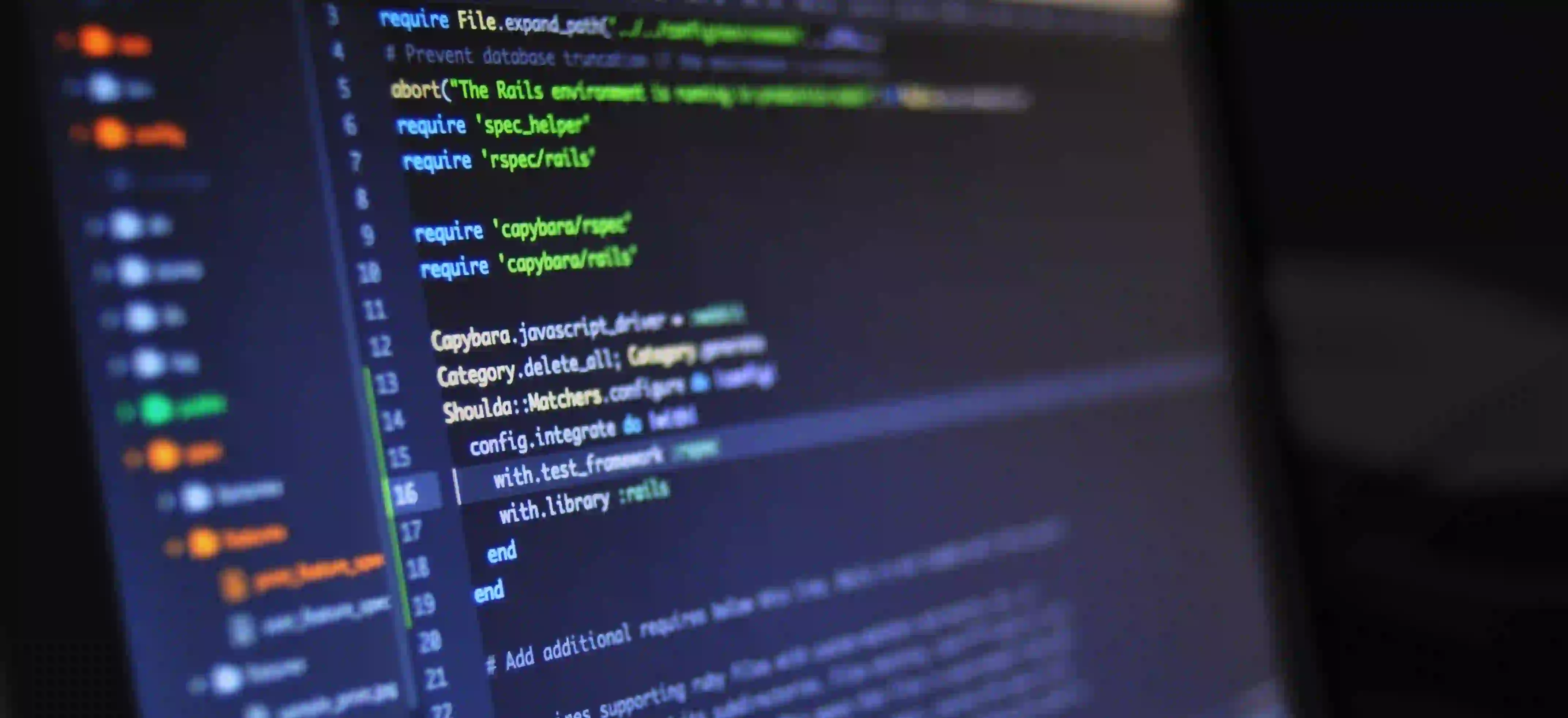
Overcoming Challenges in Spring Quartz Custom Integration
Integrating Spring with Quartz can elevate your Java applications by adding robust scheduling capabilities. However, integrating these powerful frameworks can be tricky due to different challenges that may arise during implementation. This blog post aims to guide you through these challenges, providing practical solutions and code snippets to make your integration seamless.
What is Spring and Quartz?
Before we dive into challenges and solutions, let’s briefly discuss what Spring and Quartz are.
Spring Framework: A powerful framework that simplifies Java development through its comprehensive set of features, including dependency injection, aspect-oriented programming, and transaction management.
Quartz Scheduler: An open-source job scheduling library that enables you to execute tasks at specified intervals, providing flexibility and reliability in job execution.
Setting Up Spring and Quartz
To kick off, let's ensure we set up a Spring Boot application with Quartz. Here is a simple configuration to get you started.
<!-- Maven Dependency for Spring and Quartz -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-quartz</artifactId>
</dependency>
This dependency simplifies the integration process. The Spring Boot Starter handles most configurations, so you can focus on building your application logic.
Common Challenges in Custom Integration
While integrating Spring with Quartz, several challenges can arise. Let's address these challenges along with effective solutions.
Challenge 1: Job Configuration
One of the primary hurdles developers face is configuring jobs properly. You must define the job and its triggering mechanism accurately.
Solution: Use Spring's JobDetail and Trigger classes.
Here’s an example of configuring a simple job.
@Component
public class MyJob implements Job {
@Override
public void execute(JobExecutionContext context) throws JobExecutionException {
System.out.println("Executing MyJob...");
// Job logic here
}
}
@Configuration
public class QuartzConfig {
@Bean
public JobDetail myJobDetail() {
return JobBuilder.newJob(MyJob.class)
.withIdentity("MyJob")
.storeDurably()
.build();
}
@Bean
public Trigger myJobTrigger() {
SimpleScheduleBuilder scheduleBuilder = SimpleScheduleBuilder.simpleSchedule()
.withIntervalInSeconds(10)
.repeatForever();
return TriggerBuilder.newTrigger()
.forJob(myJobDetail())
.withIdentity("MyJobTrigger")
.withSchedule(scheduleBuilder)
.build();
}
}
Why This Code Works
- Job Implementation: Your job class must implement the
Job
interface. This encapsulates the logic you want to execute. - JobDetail and Trigger: The
JobDetail
holds information about the job while theTrigger
defines when the job runs. - Simple Schedule: In this configuration, the job executes every ten seconds, showcasing the flexibility of Quartz.
For more complex scheduling options, you can explore Quartz Scheduler Documentation.
Challenge 2: Handling Dependencies in Jobs
A common issue arises when your job needs to access Spring-managed beans. You might find that the Quartz job is not aware of the Spring Application Context, which leads to dependency injection issues.
Solution: Use Spring's job factory mechanism.
Make use of the SpringBeanJobFactory
to handle this.
@Bean
public SchedulerFactoryBean schedulerFactoryBean() {
SchedulerFactoryBean schedulerFactoryBean = new SchedulerFactoryBean();
schedulerFactoryBean.setJobFactory(springBeanJobFactory());
return schedulerFactoryBean;
}
@Bean
public SpringBeanJobFactory springBeanJobFactory() {
SpringBeanJobFactory jobFactory = new SpringBeanJobFactory();
jobFactory.setApplicationContext(applicationContext);
return jobFactory;
}
Why This Code Works
- Job Factory: By extending
SpringBeanJobFactory
, you ensure that your job automatically gets application context, allowing it to use Spring's features.
Challenge 3: Job Persistence
If your application is restarted, any unscheduled jobs may be lost if they are not properly persisted. If jobs need to be long-lived, state persistence is crucial.
Solution: Use a database for job persistence by configuring Quartz with a JDBC JobStore.
# application.yml configuration
spring:
quartz:
job-store-type: jdbc
datasource:
url: jdbc:h2:mem:testdb
driver-class-name: org.h2.Driver
username: sa
password:
Why This Code Works
- JDBC Store: By setting
job-store-type
to JDBC, you can persist jobs in a database, ensuring job data isn't lost on application restarts.
Challenge 4: Handling Job Failures
Handling job failures is crucial for maintaining application reliability. If a job fails and isn't retried, you may miss critical tasks.
Solution: Use Quartz's job listener functionality along with exception handling.
public class MyJobListener implements JobListener {
@Override
public String getName() {
return "MyJobListener";
}
@Override
public void jobExecutionVetoed(JobExecutionContext context) { }
@Override
public void jobToBeExecuted(JobExecutionContext context) { }
@Override
public void jobWasExecuted(JobExecutionContext context, JobExecutionException jobException) {
if (jobException != null) {
System.out.println("Job failed: " + jobException.getMessage());
// Logic to handle the failure
}
}
}
@Bean
public JobDetail myJobDetail() {
// Define job
}
@Bean
public Trigger myJobTrigger() {
// Define trigger
}
@Bean
public MyJobListener myJobListener() {
return new MyJobListener();
}
Why This Code Works
- Job Listener: Implementing a
JobListener
allows you to handle job execution outcomes, giving you an opportunity to react to failures in real-time.
Bringing It All Together
Integrating Spring with Quartz offers powerful scheduling capabilities to your Java applications. However, it comes with its set of challenges. By following the solutions outlined in this post, such as properly configuring jobs, using dependency injection, implementing job persistence, and managing job failures, you can efficiently handle these hurdles.
Remember that proper logging, monitoring, and testing are essential to ensure that your scheduling works correctly and that you can handle any issues that may arise promptly.
As you continue your journey of mastering Spring and Quartz integration, do not hesitate to refer to the Quartz Scheduler Documentation for further details.
Enjoy building your scheduled tasks with Spring and Quartz, and overcome those challenges with confidence!