How to Detect and Fix Memory Leaks in Your Code
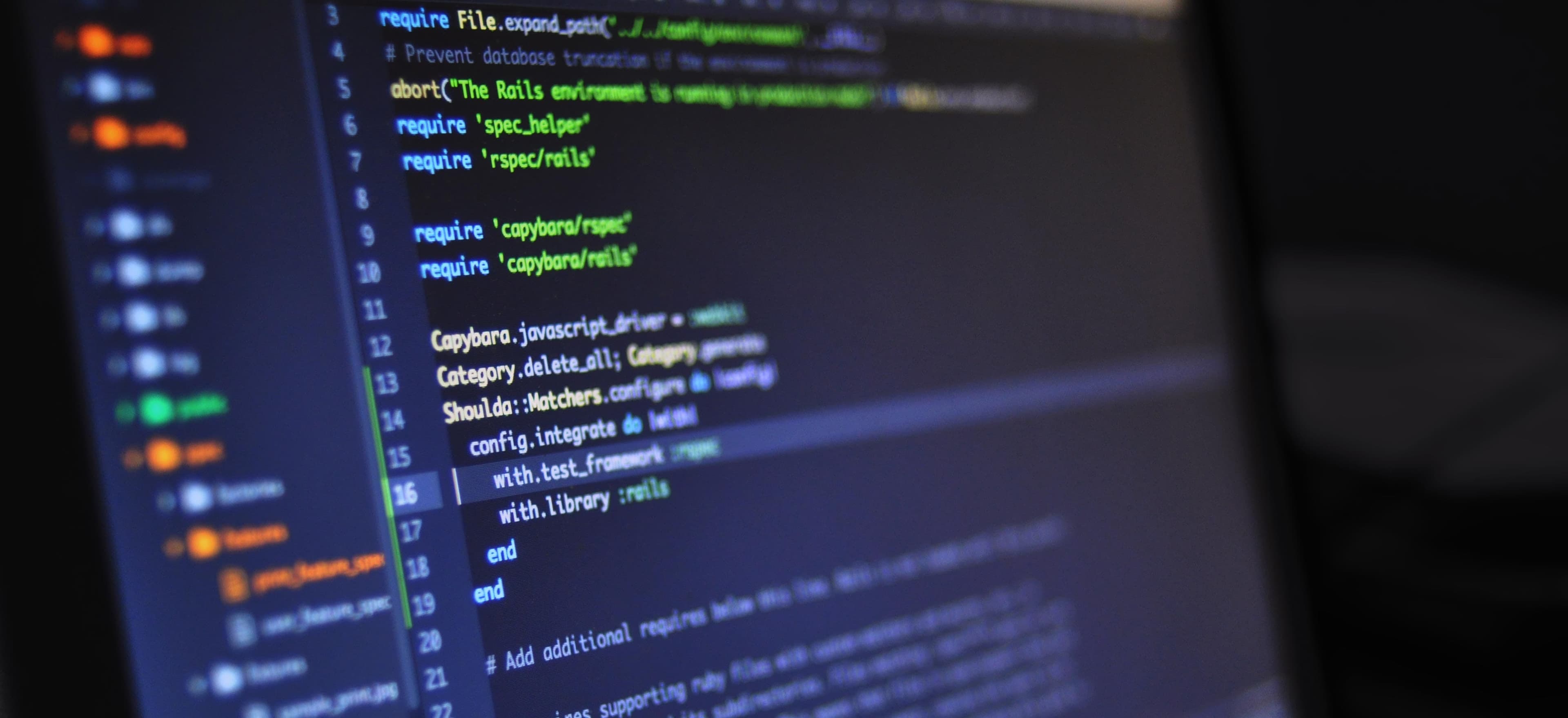
- Published on
How to Detect and Fix Memory Leaks in Your Java Code
Memory management is a crucial aspect of software development, particularly in languages like Java where Automatic Garbage Collection (GC) is employed. However, even with garbage collection in place, memory leaks can happen. In this blog post, we will explore how to detect and fix memory leaks in your Java applications.
Understanding Memory Leaks
A memory leak occurs when a program retains references to objects that are no longer needed, preventing the garbage collector from reclaiming memory. Over time, these uncollected objects can lead to high memory usage, reduced performance, and even application crashes.
Recognizing memory leaks early is essential for maintaining a robust application.
How to Detect Memory Leaks
1. Monitor Application Behavior
The first step in identifying potential memory leaks is to monitor your application's performance. Watch for the following indicators:
- Increasing memory usage over time.
- Slow application response.
- OutOfMemoryError exceptions.
Tools like Java VisualVM and YourKit can help visualize memory usage.
2. Use Profiling Tools
Profiling tools are invaluable for detecting memory leaks. Java offers several options:
Java VisualVM
Java VisualVM is a free tool bundled with the JDK. It provides real-time monitoring and profiling capabilities.
To use Java VisualVM:
- Start your application with the
-Dcom.sun.management.jmxremote
option. - Launch VisualVM and connect to your running application.
- Explore the ‘Memory’ and ‘Threads’ tab to identify memory usage patterns.
Eclipse Memory Analyzer (MAT)
Eclipse MAT is another powerful tool for analyzing memory dumps. You can capture a heap dump by running:
jmap -dump:format=b,file=heapDump.hprof <pid>
Then, open the heap dump in MAT. This tool can help identify objects with high retention rates.
3. Analyze Heap Dumps
After capturing a heap dump, you can analyze object retention using MAT. The tool provides insights into:
- Dominator trees, which help visualize object ownership.
- Leak suspects that could indicate potential leaks.
For a detailed guide on using Eclipse MAT, check here.
Fixing Memory Leaks
Upon detection of a memory leak, the next step is to resolve it. Here are several strategies:
1. Review Code for Unreleased References
Start by reviewing your code for unintentional references. This often occurs in collections or static variables.
For example, consider the following code snippet:
public class MemoryLeakExample {
private List<String> dataStorage = new ArrayList<>();
public void addData(String data) {
dataStorage.add(data);
}
}
In this example, the dataStorage
list can grow indefinitely if addData()
is called repeatedly, leading to memory leaks. A fix would be to clear the list when it's no longer required:
public void clearData() {
dataStorage.clear(); // Clear the list to free memory
}
2. Utilize Weak References
Weak references allow the garbage collector to reclaim memory when there are no strong references to an object. You can achieve this using WeakHashMap
or WeakReference
:
import java.lang.ref.WeakReference;
import java.util.HashMap;
public class WeakReferenceExample {
private HashMap<String, WeakReference<MyObject>> weakMap = new HashMap<>();
public void addObject(String key, MyObject object) {
weakMap.put(key, new WeakReference<>(object));
}
}
In this scenario, MyObject
instances can be garbage collected if there are no strong references left, effectively preventing memory leaks.
3. Manage Collection Data Types
When using collections, consider using structures that allow for automatic cleanup. For example, use ConcurrentHashMap
or WeakHashMap
to automatically manage the lifecycle of objects.
4. Implement Proper Lifecycle Management
In applications such as Java EE, ensure proper lifecycle management for resources. For instance, closing database connections, and IO streams is crucial.
Here’s an example of lifecycle management with a database connection:
Connection conn = null;
try {
conn = DriverManager.getConnection(url, user, password);
// Perform database operations
} catch (SQLException e) {
e.printStackTrace();
} finally {
if (conn != null) {
try {
conn.close(); // Ensure connection is closed
} catch (SQLException e) {
e.printStackTrace();
}
}
}
By closing resources in a finally
block, we prevent potential memory leaks.
5. Regular Code Review
Conduct regular code reviews focusing on memory management. Engaging other developers can unveil oversight or patterns causing memory leaks.
Best Practices to Avoid Memory Leaks
To prevent memory leaks from occurring in the first place, consider implementing the following best practices:
1. Limit Use of Static Variables
Static variables can lead to memory leaks if not managed properly. Avoid excessive use of static data members, especially when they hold references to large objects.
2. Dispose of Resources Properly
Always dispose of resources (such as listeners, threads, and database connections) when they are no longer needed.
3. Analyze Performance Regularly
Regularly analyze your application’s memory performance during the development and testing phases. Use profiling and monitoring tools consistently.
Conclusion
Memory leaks can severely impact application performance. Detecting and fixing them requires diligence and the right tools. By leveraging tools like Java VisualVM and Eclipse MAT, as well as adopting best coding practices, developers can identify and mitigate memory leaks effectively.
Further Reading
For more in-depth discussions on memory management and profiling in Java, visit Oracle's Java Performance Tuning Guide and explore the features of YourKit for advanced profiling strategies.
By understanding how to detect and address memory leaks, you will improve your application’s efficiency and reliability. Happy coding!
Checkout our other articles