Simplifying Migration: Lombok to Java Records Made Easy
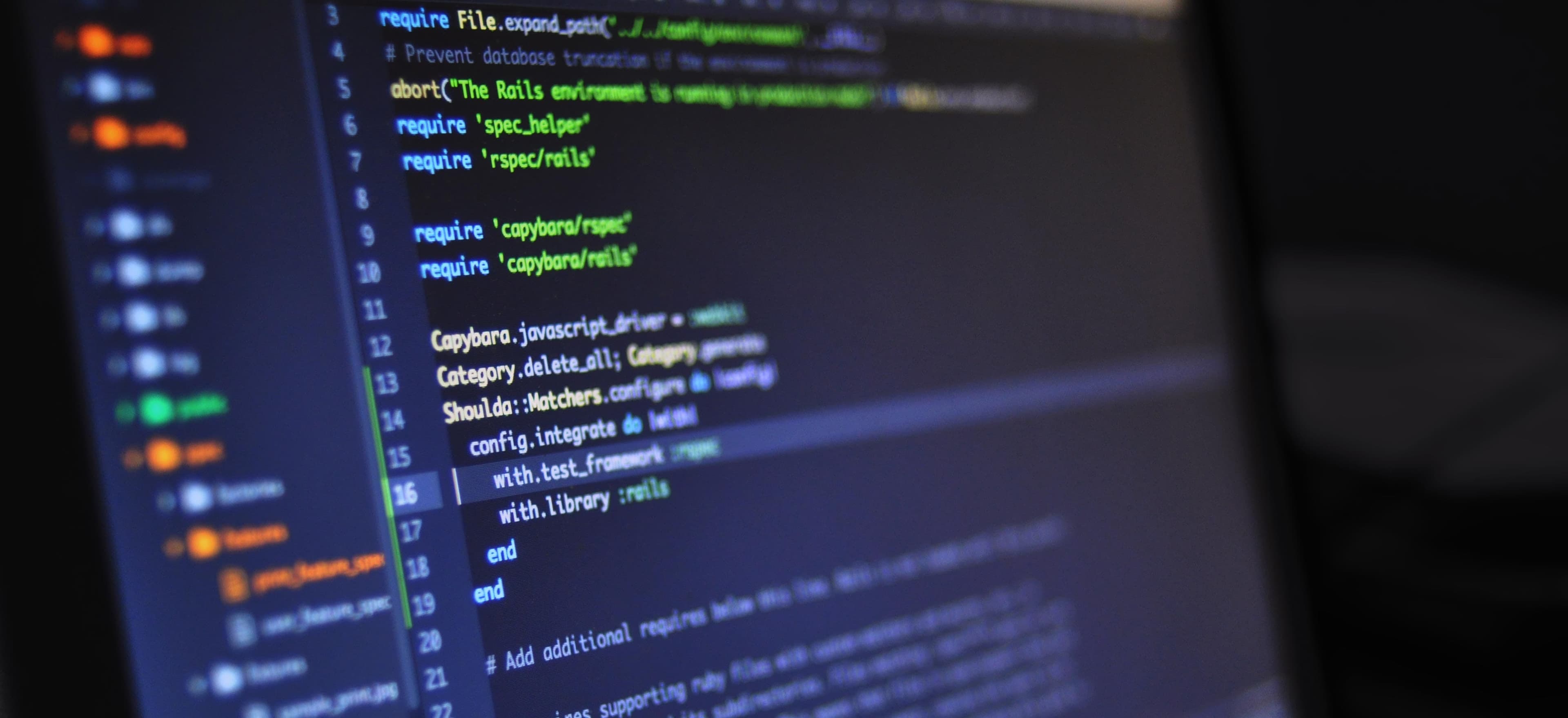
- Published on
Simplifying Migration: Lombok to Java Records Made Easy
In recent years, Java has embraced various enhancements aimed at simplifying programming tasks. One such improvement is the introduction of Java Records in Java 14 as a preview feature and a standard feature in Java 16. Records are lightweight data carriers that make it easy to create immutable data classes without boilerplate code. This evolution has created a question for many developers: How do we migrate from Lombok to Java Records?
In this blog post, we'll walk you through this migration process, comparing Lombok and Java Records, showcasing code snippets, and highlighting the “why” behind this migration. Let’s dive in!
Understanding Lombok
Project Lombok is a popular Java library that helps you reduce boilerplate code in your Java applications. By using annotations like @Getter
, @Setter
, @Builder
, and @ToString
, Lombok can generate getter and setter methods, construction methods, and utility functions at compile time.
A Simple Lombok Example
Consider a typical data class using Lombok.
import lombok.Data;
@Data
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
}
Why Lombok?
- Boilerplate Reduction: Lombok eliminates the boilerplate code needed for simple data classes.
- Readability: Your classes are more readable, focusing on the essential structure without distractions.
However, while Lombok serves its purpose well, it can introduce some complexities:
- Dependency Management: You must manage Lombok as an external dependency.
- IDE Support: Some IDE features may not work seamlessly with Lombok, leading to potential confusion during development.
Introducing Java Records
Java Records offer an alternative approach. They are designed to serve as a simple way to define immutable data objects. By declaring a record, the JVM generates everything you need behind the scenes—including getters, toString methods, and equals/hashCode implementations.
A Simple Record Example
Let’s refactor our Lombok-based Person
class to use Java Records instead.
public record Person(String name, int age) {}
Why Java Records?
- Simplification: Java Records provide a cleaner, more concise syntax compared to Lombok. You only need to define the fields, and the rest is handled for you.
- Immutability: Records are immutable, which is a great fit for many data model scenarios, removing the risk of unexpected state changes.
- Built-in Methods: You get all the methods you need out-of-the-box: getters, toString(), hashCode(), and equals().
Key Migration Steps
Migrating from Lombok to Records can be done systematically. Here are the steps you can follow:
Step 1: Identify Lombok Classes
Start by identifying all the Lombok classes you’d like to migrate. Given our previous Person
class, we note that it has two fields: name
and age
.
Step 2: Create Java Records
For each class, create a corresponding record. For our Person
example:
public record Person(String name, int age) {}
This new declaration automatically generates the constructor, getters, and utility methods.
Step 3: Update Usage
Next, update all usages of the Lombok class methods and constructors to use the records. Here’s how to adapt the usage from Lombok:
Before (Lombok)
Person person = new Person("Alice", 30);
System.out.println(person.getName());
After (Records)
Person person = new Person("Alice", 30);
System.out.println(person.name());
Step 4: Modify Third-party Integration
If your class integrates with third-party libraries or frameworks (e.g., Jackson for JSON serialization), make sure the interaction still functions correctly with records.
import com.fasterxml.jackson.databind.ObjectMapper;
public class Main {
public static void main(String[] args) throws Exception {
ObjectMapper objectMapper = new ObjectMapper();
Person person = new Person("Alice", 30);
// Serializing the record
String jsonString = objectMapper.writeValueAsString(person);
System.out.println(jsonString);
// Deserializing the record
Person deserialized = objectMapper.readValue(jsonString, Person.class);
System.out.println(deserialized);
}
}
Considerations for Migration
1. Compatibility
- Ensure your project uses Java 16 or above to leverage records.
- Review any Lombok-specific code generation, as records manage this differently.
2. Design Patterns
Evaluate how your design patterns may need to adapt with the immutability constraint of records. For example, if you were using mutable objects extensively, you may need to rethink your approach.
3. IDE Support
Modern IDEs have excellent support for Java Records. Ensure your development environment is up to date to leverage this functionality fully.
Best Practices with Java Records
- Use Meaningful Names: Names should clearly articulate the purpose of the record.
- Limit Responsibility: Records should remain simple, serving primarily as data containers.
- Leverage Immutability: Use records to your advantage by making data flow discernable and predictable.
Final Thoughts
Migrating from Lombok to Java Records can simplify your codebase significantly by reducing boilerplate and enhancing readability. While Lombok has helped developers for a long time, Java Records bring a new level of simplicity and clarity to Java programming.
To summarize, Java Records provide a clean alternative for defining data classes alongside built-in immutable structure—making them a compelling choice moving forward. Whether working independently or in collaboration with modern frameworks, adopting records in your Java application can lead to better, cleaner, and more maintainable code.
For further reading, check out the official Java Language Documentation and provide yourself with additional guidelines on working with records effectively.
Happy coding!