Dynamic Log Level Management in Spring Boot Actuator
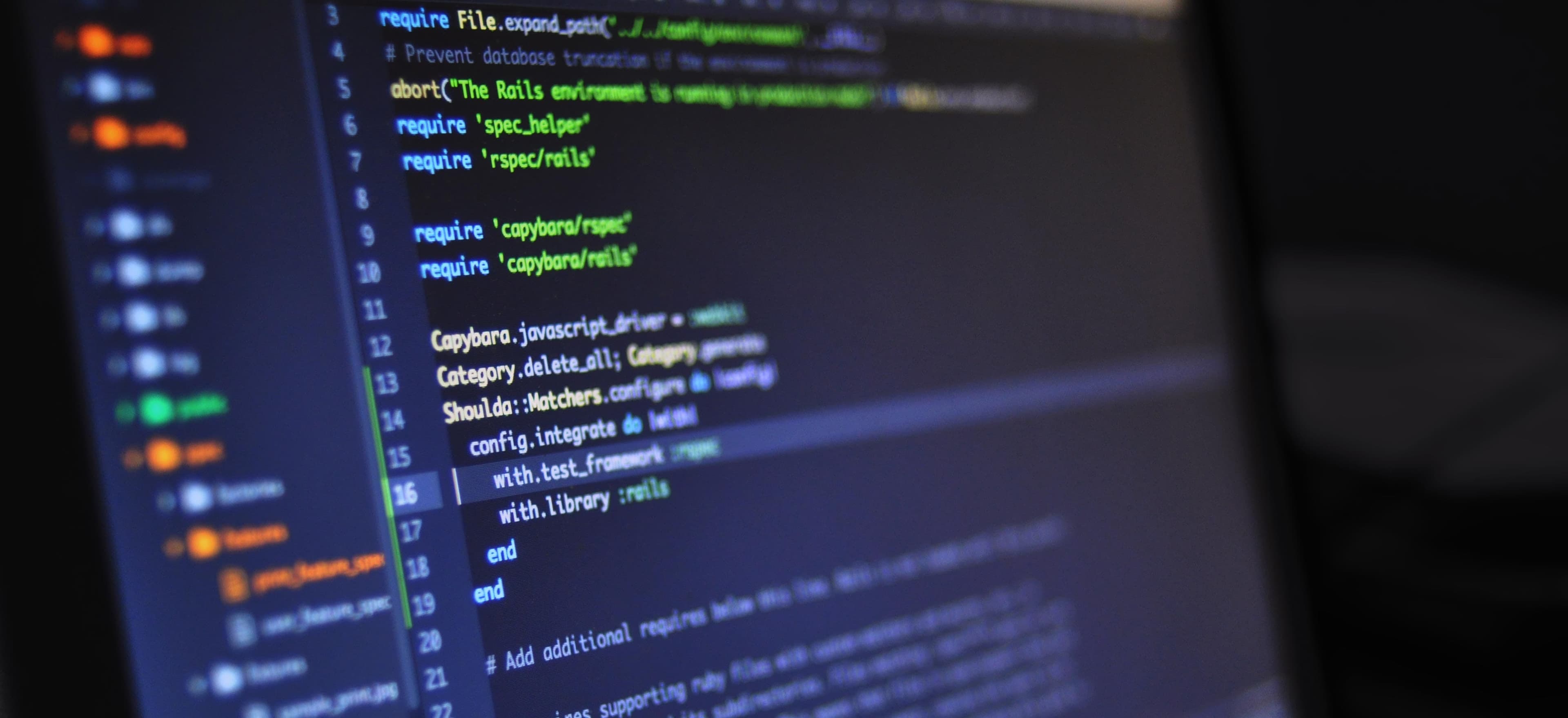
- Published on
Dynamic Log Level Management in Spring Boot Actuator
In modern application development, logging is an essential practice that significantly aids in monitoring and debugging. With the widespread use of Spring Boot for microservices, developers often face the challenge of efficient log management. Dynamic log level management is a critical feature offered by Spring Boot Actuator, allowing you to change log levels at runtime without the need to restart your application.
In this blog post, we will explore how to implement and use dynamic log level management in a Spring Boot application using Spring Boot Actuator. We will also provide example code snippets along with explanations to enhance your understanding of the concept.
What is Spring Boot Actuator?
Spring Boot Actuator is a set of built-in tools designed to help you monitor and manage your Spring Boot application. It provides a wide array of features, such as health checks, metrics, environment details, and, importantly for our discussion, logging configuration.
You can learn more about Spring Boot Actuator on the official Spring website.
Why Dynamic Log Level Management?
The ability to change log levels dynamically offers several advantages:
- Troubleshooting: You can increase logging granularity for specific packages or components without restarting the application.
- Performance: Reducing logging levels in high-traffic environments can improve performance.
- Flexibility: Different environments might require different logging levels, which you can change on the fly based on real-time demands.
Prerequisites
Before we begin, ensure you have the following:
- Latest version of Java Development Kit (JDK).
- A Spring Boot application set up (if you need help getting started, check out Building a RESTful Web Service).
- Maven or Gradle to manage dependencies.
Setting Up Spring Boot Actuator
In your Spring Boot application, you need to add the Spring Boot Actuator dependency. Open your pom.xml
(for Maven) and include:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
For Gradle, add the following to your build.gradle
:
implementation 'org.springframework.boot:spring-boot-starter-actuator'
After adding the dependency, you need to enable the logging endpoint in your application.properties
(or application.yml
):
management.endpoints.web.exposure.include=loggers
Checking the Current Log Levels
Once you've set up everything, you can check the current log levels in the application. Using curl
, you can query the /actuator/loggers
endpoint:
curl -X GET http://localhost:8080/actuator/loggers
This will return a JSON response detailing the current log levels for all packages.
Changing Log Levels Dynamically
One of the standout features of Spring Boot Actuator is the ability to change log levels dynamically. Here's how you can do it.
Changing Log Level using REST
To change the log level of a specific package, you can send a POST
request to the same /actuator/loggers/{logger-name}
endpoint. Here’s a straightforward example using curl
:
curl -X POST \
-H "Content-Type: application/json" \
-d '{"configuredLevel": "DEBUG"}' \
http://localhost:8080/actuator/loggers/com.example.yourpackage
Explanation:
In this command, replace com.example.yourpackage
with the actual package name for which you want to set the log level. The configuredLevel
field defines the desired log level, such as TRACE
, DEBUG
, INFO
, WARN
, ERROR
, or OFF
.
Example Code Snippet
Here’s an example of how you might structure a controller in your Spring Boot application to facilitate changing log levels:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import org.springframework.boot.actuate.logging.LoggersEndpoint;
@RestController
@RequestMapping("/log")
public class LogLevelController {
@Autowired
private LoggersEndpoint loggersEndpoint;
@PostMapping("/{loggerName}/{level}")
public void changeLogLevel(@PathVariable String loggerName, @PathVariable String level) {
loggersEndpoint.configureLogLevel(loggerName, level);
System.out.println("Log level for " + loggerName + " set to " + level);
}
}
Why this Design?:
This approach encapsulates the logic to change log levels in a REST endpoint, allowing developers or administrators to change log levels securely and conveniently via HTTP requests.
Testing the REST Controller
After implementing the controller, you can test it with a cURL command:
curl -X POST http://localhost:8080/log/com.example.yourpackage/INFO
This will set the logging level of the specified package to INFO
.
Monitoring the Changes
To ensure the log level changes have taken effect, you can re-query the /actuator/loggers
endpoint:
curl -X GET http://localhost:8080/actuator/loggers/com.example.yourpackage
The returned JSON response will confirm the updated log level.
In Conclusion, Here is What Matters
Dynamic log level management is a powerful feature of Spring Boot Actuator that can streamline troubleshooting and enhance your application's performance. By following the instructions in this post, you have successfully implemented a mechanism to change log levels at runtime without restarting your application.
While we provided a RESTful way of managing log levels, you could also integrate this functionality into a user interface or admin dashboard for even greater convenience.
For further reading, consider checking out Spring Boot Logging Documentation for more detailed information on logging configurations.
By adopting this practice, you can significantly enhance your microservices' observability, leading to better-maintained applications and improved developer productivity.
Happy logging!