Handling Data Loss: Undo Feature Challenges in Neo4j
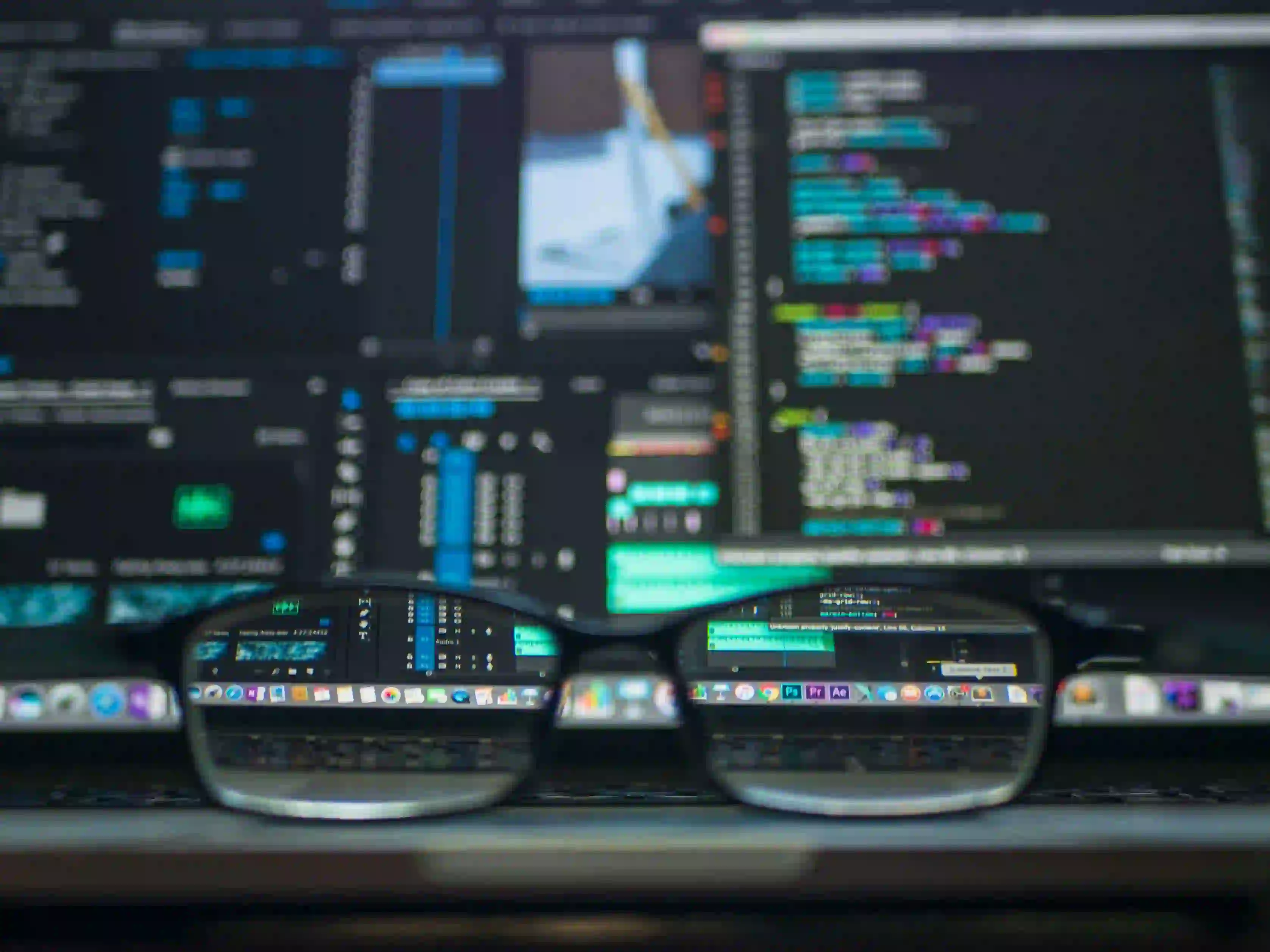
Handling Data Loss: Undo Feature Challenges in Neo4j
In the world of database management, data integrity and the ability to recover from data loss are critical features. This is particularly true for graph databases like Neo4j, which rely on relationships between data points. One notable feature designed to mitigate risks related to data loss is the Undo feature. While it's a powerful tool, it comes with its own set of challenges. In this blog post, we will explore the intricacies of this feature, discuss its limitations, and provide actionable solutions to handle data loss effectively in Neo4j.
Understanding Neo4j and the Undo Feature
Neo4j is a native graph database that leverages the power of relationships to enhance data retrieval and manipulation. When you make changes to the database, such as creating or modifying nodes and relationships, Neo4j keeps track of these changes using transaction logs. The Undo feature allows users to revert their changes, making it easier to recover from mistakes or unexpected issues.
What Is the Undo Feature?
The Undo feature in Neo4j primarily works through transaction management. When you execute a transaction, Neo4j tracks the changes in a log. If you need to revert a transaction, you can simply roll it back. However, it's essential to understand that the Undo feature is not foolproof. Let's look at the functionality through an example.
// Example of starting a transaction in Neo4j
try (Transaction tx = graphDb.beginTx()) {
Node node = graphDb.createNode(Label.label("Person"));
node.setProperty("name", "John Doe");
tx.success(); // Marks the transaction as successful
}
In the example above, we start a transaction to create a new node representing a Person. If something goes wrong after this line, the transaction can be rolled back, preventing data loss.
Challenges with the Undo Feature
While the Undo feature appears straightforward, there are several challenges to consider:
1. Complexity of Relationships
Neo4j’s strength lies in its ability to manage complex relationships between data points. When you make changes, such as deleting a node that has several relationships, the Undo feature can inadvertently leave orphaned relationships, creating inconsistency in your database.
Solution: Always consider the relationships dependent on the data you are modifying. When using the Undo feature, ensure you verify that all dependencies are intact.
2. Performance Overhead
Maintaining an undo log for every transaction can introduce performance overhead, especially in high-transaction environments. The overhead can lead to memory issues and slower response times, particularly when rolling back larger transactions.
// Example: Rollback with a large transaction might consume resources
try (Transaction tx = graphDb.beginTx()) {
Node nodeA = graphDb.createNode(Label.label("Person"));
Node nodeB = graphDb.createNode(Label.label("Person"));
nodeA.createRelationshipTo(nodeB, RelationshipType.withName("KNOWS"));
// Perform more operations...
// Simulate an issue that requires a rollback
tx.failure();
}
In this scenario, rolling back will undo the relationship and nodes created. If this transaction were to involve many nodes or relationships, it could result in a significant performance hit during the rollback process.
3. Limitations on Undo History
Neo4j does not maintain an indefinite undo history. The undo logs can be cleared over time due to various factors, such as database restarts or log management settings. Once cleared, you can no longer revert to previous states.
Solution: Regularly back up your data to ensure you have a valid recovery point. You can follow Neo4j backup documentation for guidance.
Best Practices for Handling Data Loss in Neo4j
To effectively manage the challenges of the Undo feature, consider implementing these best practices:
1. Transaction Management
Make use of proper transaction management. Always encapsulate your operations within transactions to ensure atomicity.
try (Transaction tx = graphDb.beginTx()) {
// Perform operations
tx.success(); // Commit only if all operations succeed
} catch (Exception e) {
// Log exception and decide on rollback if necessary
}
2. Regular Backups
Frequent backups are crucial. Set up a backup strategy to ensure your data can be restored in emergencies. Tools like the Neo4j Admin tool or Neo4j Ops Manager can facilitate this process.
3. Monitoring and Alerts
Implement monitoring on your Neo4j database. Systems like Prometheus can help detect performance issues and send alerts when something goes wrong.
4. Documentation and Procedures
Maintain clear documentation on your data model and relationships. Awareness of what each part of your database does will assist in making better decisions about what can be modified or deleted without unintended fallout.
5. Testing Changes
Before deploying significant changes or updates, especially in production, test them in a staging environment. This minimizes the risk and ensures that all potential pitfalls, particularly those related to data loss, are addressed.
Key Takeaways
The Undo feature in Neo4j provides a safety net for accidental changes; however, relying solely on it isn't advisable. Understanding the challenges and implementing best practices can significantly enhance data integrity and recovery in your Neo4j database.
For further insights, consider delving into more specific topics surrounding Neo4j's transaction management and backup strategies. Additionally, exploring the broader field of database management systems will enrich your understanding of potential pitfalls and best practices.
For more information on Neo4j graph databases, check out Neo4j Official Documentation. Happy coding!