Thread Pool Mismanagement: The Silent Performance Killer
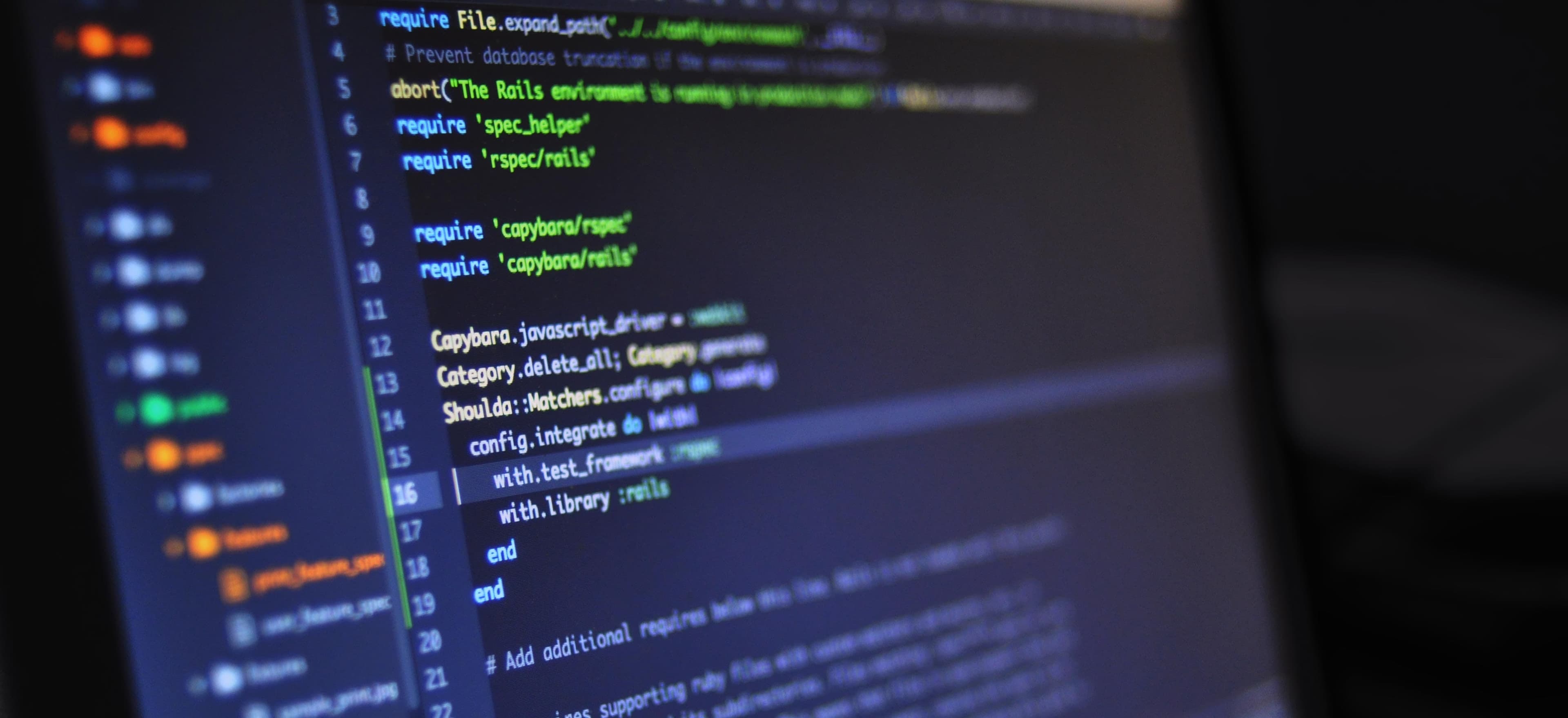
- Published on
Thread Pool Mismanagement: The Silent Performance Killer
In the ever-evolving world of Java development, managing thread pools is a critical task. Thread pools are meant to optimize resource usage and improve application performance. However, poor management can lead to a host of problems, ultimately becoming a silent performance killer. In this blog post, we will explore the intricacies of thread pools, common pitfalls associated with their mismanagement, and best practices to avert performance lags.
What is a Thread Pool?
A thread pool is a collection of worker threads that efficiently execute tasks concurrently. By reusing a limited number of threads to handle multiple tasks, thread pools minimize the overhead associated with thread creation and destruction. The ExecutorService
framework in Java offers a powerful way to manage thread pools effectively.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(5);
for (int i = 0; i < 10; i++) {
final int taskId = i;
executor.submit(() -> {
System.out.println("Executing task with ID: " + taskId);
});
}
executor.shutdown();
}
}
In this example, we create a thread pool with a fixed number of threads (5) to handle 10 tasks. This reusability is the core of why thread pools are critical in application performance.
The Consequences of Mismanagement
1. Thread Leak
One of the most profound issues stemming from poor thread pool management is thread leakage. When threads in a pool are not properly released or are waiting indefinitely, it can lead to exhaustion of system resources. This situation could result in new tasks being unable to execute, leading to a sluggish application.
2. Overutilization of Resources
While creating a large number of threads may seem beneficial at first glance, it's essential to understand the costs associated with it. Excessive threads can overwhelm the CPU and memory, resulting in increased context switching and reduced overall throughput.
For instance, consider this scenario:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class OverutilizationExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newCachedThreadPool();
for (int i = 0; i < 1000; i++) {
executor.submit(() -> {
// Simulating a time-sensitive task
try {
Thread.sleep(100);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
}
executor.shutdown();
}
}
In this code snippet, the usage of newCachedThreadPool()
, although suitable for short-lived tasks, can potentially lead to the creation of hundreds or thousands of threads, overwhelming system resources and degrading performance.
3. Increased Latency
Poorly managed thread pools can result in increased latency. This happens when tasks are queued for an unreasonably long period because the available threads are busy, leading to user frustration and potentially lost revenue.
Best Practices for Thread Pool Management
1. Define the Correct Pool Size
A fundamental step in preventing thread pool mismanagement is to ensure the pool size is appropriate for the task workload and system capabilities. A famous rule of thumb is using:
coreSize = number of CPU cores + 1
This size dynamically caters to I/O-bound tasks while maintaining high performance for CPU-bound tasks. You can further fine-tune this based on load tests that replicate real-world scenarios.
2. Use the Right Executor Type
Java's Executors
class provides various implementations for different use cases:
- Fixed Thread Pool: Ideal for steady workloads.
- Cached Thread Pool: Best for short-lived tasks but can lead to overutilization if not monitored.
- Single Thread Executor: Useful for simplicity and making sure tasks complete sequentially.
Each executor type has its advantages, and leveraging the right one is crucial to balance resource utilization effectively.
3. Monitor the Thread Pool
Implement monitoring for thread pool performance. Tools like Java Management Extensions (JMX) or third-party solutions such as Prometheus and Grafana can provide insight into thread activity. Knowing the number of active threads, queued tasks, and task completion rates will help you troubleshoot issues before they affect your application's performance.
// Monitoring Example with JMX
import java.lang.management.ManagementFactory;
import java.lang.management.ThreadMXBean;
public class ThreadMonitoring {
public static void main(String[] args) {
ThreadMXBean threadMXBean = ManagementFactory.getThreadMXBean();
long[] threadIds = threadMXBean.getAllThreadIds();
for (long id : threadIds) {
System.out.println("Thread ID: " + id + " - State: " + threadMXBean.getThreadInfo(id).getThreadState());
}
}
}
Monitoring can help you gain insight into any bottlenecks and allow for timely optimizations.
4. Graceful Shutdown
Ensuring that thread pools are properly shut down can prevent resource leaks. Always call shutdown()
once the work is done. Additionally, use awaitTermination()
to ensure all tasks complete before exiting the application.
executor.shutdown();
try {
if (!executor.awaitTermination(60, TimeUnit.SECONDS)) {
executor.shutdownNow();
}
} catch (InterruptedException e) {
executor.shutdownNow();
}
This pattern of shutting down ensures that your application cleans up resources effectively, minimizing the risk of memory bloat and performance degradation.
5. Avoid Long-Running Tasks in Thread Pools
If a task will take a significant amount of time, consider running it in a separate thread (not in the pool). Long-running tasks can block other tasks in the pool, which leads to poorer performance overall.
ExecutorService executor = Executors.newFixedThreadPool(5);
Runnable longRunningTask = () -> {
// Long-running task simulation
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
};
// Offloading this to a separate thread
new Thread(longRunningTask).start();
This approach keeps your thread pool responsive while still completing lengthy operations.
Wrapping Up
Thread pool mismanagement can become a silent performance killer in Java applications. By understanding the mechanics of thread pools and adhering to best practices, you can prevent potential pitfalls, ensuring your application runs smoothly.
For a deeper dive into Java's concurrency features, check out the Java Concurrency in Practice book, which offers extensive insights and practical examples. Additionally, the official Java documentation is a valuable resource for understanding the essential classes and their usage.
By implementing these best practices, you can maximize performance and reliability while yielding the benefits of multithreading in your Java applications. Remember, in the world of concurrent programming, being proactive is the key to success. Happy coding!