Common Hibernate Errors and How to Fix Them
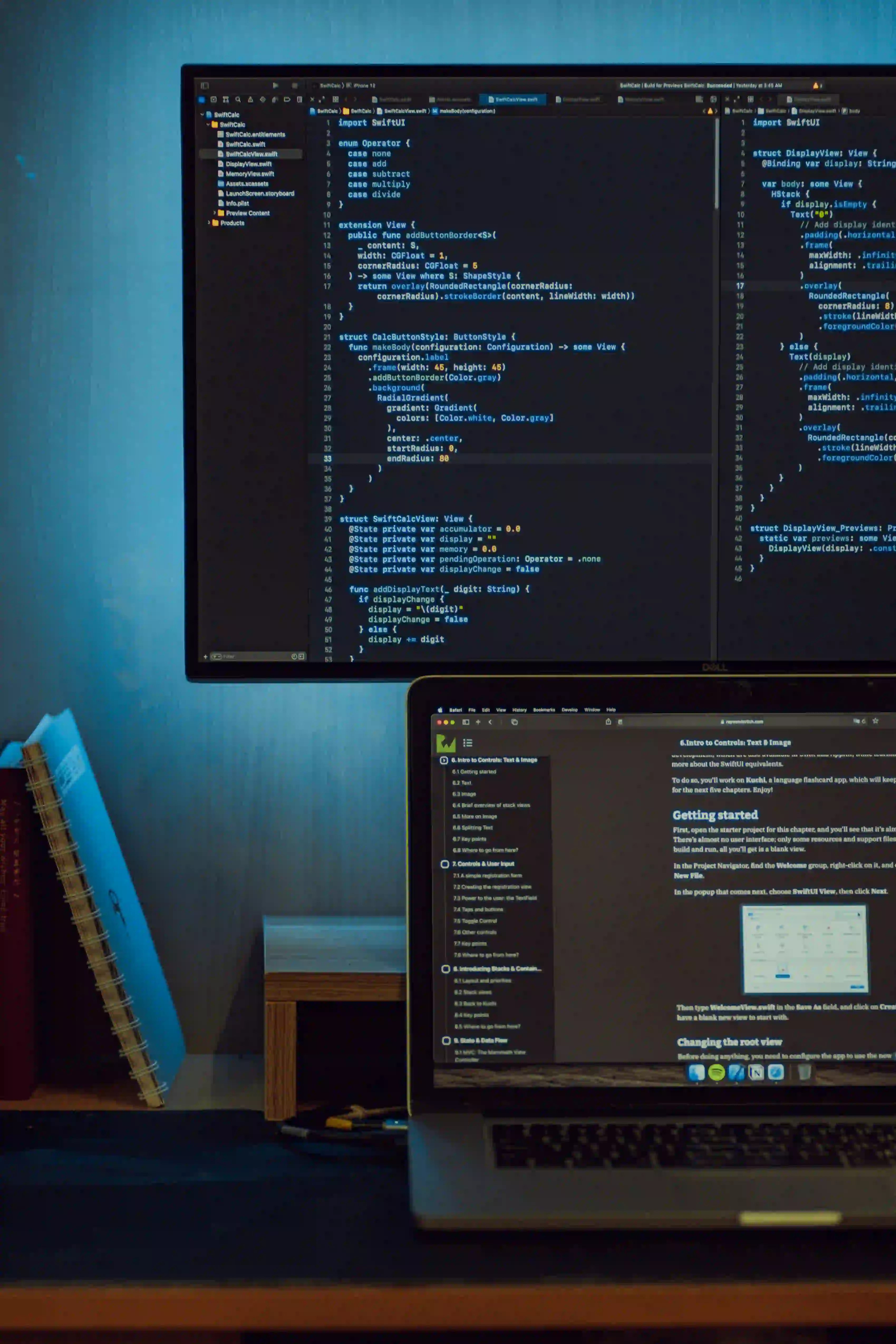
Common Hibernate Errors and How to Fix Them
Hibernate is a powerful Object-Relational Mapping (ORM) tool for Java that simplifies data handling in applications. However, working with Hibernate can sometimes lead to frustrating errors. Understanding these common issues can save you significant debugging time and enhance your productivity. In this blog post, we will explore some prevalent Hibernate errors, their causes, and effective solutions.
Table of Contents
Understanding Hibernate
Hibernate provides a framework for mapping an object-oriented domain model to a relational database. This allows developers to perform database operations without writing a lot of SQL. The main objectives of using Hibernate include improved productivity, abstraction from the underlying database, and better object manipulation through its session management and transaction handling features.
Key Hibernate Features
- Session Management: It provides a way to manage database sessions and transactions effectively.
- Caching: Enhances performance by storing frequently accessed data in memory.
- Automatic Schema Generation: Automatically generates the database schema based on the entity mappings.
Common Hibernate Errors
Now let's dive into some of the most common errors encountered while using Hibernate.
1. LazyInitializationException
The LazyInitializationException
occurs when an attempt is made to access a loaded entity that has been associated with a session that has already been closed.
Cause
Typically, this happens when retrieving an entity or collection with lazy loading outside an active Hibernate session, such as when using detached objects.
Solution
To resolve this issue, you have multiple options:
- Open session in view: Keep your session open until the view is rendered. This can be achieved through Open Session in View pattern.
- Eager fetching: Modify your mappings to fetch collections eagerly.
Here’s a code snippet that demonstrates eager fetching:
@Entity
public class User {
@Id
@GeneratedValue
private Long id;
@OneToMany(fetch = FetchType.EAGER)
private Set<Order> orders;
// getters and setters
}
In this code, the orders
collection associated with the User
entity is fetched eagerly, loading all orders every time the user is loaded.
2. No Session Found
This error typically occurs when operations on the database are attempted without an active Hibernate session.
Cause
This can happen if the session is not properly opened or has already been closed by the time a query is executed.
Solution
Always ensure that a session is active when performing database operations. Here is an example of how to manage sessions effectively:
Session session = sessionFactory.openSession();
Transaction transaction = session.beginTransaction();
try {
// Perform your data operations here
transaction.commit();
} catch (Exception e) {
if (transaction != null) transaction.rollback();
e.printStackTrace();
} finally {
session.close();
}
This code ensures that even if an exception occurs, the transaction is rolled back, and the session is properly closed afterward.
3. Duplicate Entry Error
The Duplicate Entry Error arises when a unique constraint is violated, primarily during an insert operation.
Cause
An attempt to insert a new entity with a primary key or unique field that already exists in the database is the central issue.
Solution
Before inserting, make sure to check if the entity already exists. Here’s how you can do this using Hibernate:
User newUser = new User();
newUser.setEmail("example@mail.com");
// Check if the user exists
User existingUser = session.get(User.class, newUser.getId());
if (existingUser == null) {
session.save(newUser);
} else {
System.out.println("User already exists!");
}
4. PropertyNotFoundException
This exception arises when Hibernate is unable to find a property specified in the mapping.
Cause
Usually, this is caused by a typo in property names or trying to access a property that is not part of the entity.
Solution
Double-check your entity class and mapping files. Ensure that the property names match correctly. For example:
@Entity
public class Product {
@Id
private Long id;
@Column(name = "product_name")
private String productName; // Ensure field names are correct
// getters and setters
}
In this scenario, verify that the name of the property "productName" corresponds to the database field "product_name".
5. QuerySyntaxException
This error occurs when there is a syntax error within an HQL (Hibernate Query Language) or JPQL (Java Persistence Query Language) statement.
Cause
Commonly, this is due to incorrectly formed queries.
Solution
Review your query syntax carefully. Here’s an example of how to construct a proper HQL statement:
String hql = "FROM User u WHERE u.email = :email";
Query query = session.createQuery(hql);
query.setParameter("email", "example@mail.com");
List<User> result = query.list();
Make sure the query syntax adheres to the correct HQL/JPQL standards.
Best Practices to Avoid Common Errors
To minimize errors while using Hibernate, consider the following best practices:
- Use Transactions: Always wrap database operations in transactions. This helps you manage rollbacks and keep data consistent.
- Clean Up Resources: Ensure sessions and transactions are closed even in cases of exceptions.
- Check Entity State: Use methods such as
session.exists()
before saving new entities to prevent duplication. - Leverage Eager Loading cautiously: While eager loading can prevent
LazyInitializationException
, it might lead to performance issues if misused. - Use Hibernate Validator: If you have validation rules for your entities, consider using Hibernate Validator to enhance data integrity.
Lessons Learned
Hibernate is a robust tool that, when utilized effectively, can significantly simplify database operations in Java applications. However, it is essential to understand the common errors that may occur, such as LazyInitializationException
, No Session Found
, and others discussed above. Armed with the right solutions and practices, you can navigate through these issues with ease.
By following the guidelines and code snippets provided in this post, you should feel more confident in troubleshooting Hibernate errors and writing more resilient applications. If you're looking for more in-depth information about Hibernate, consider checking the official Hibernate documentation or exploring Hibernate's GitHub repository for sample projects and community advice.
Happy coding!