Common Pitfalls When Using JSON Item Reader/Writer in Spring Batch
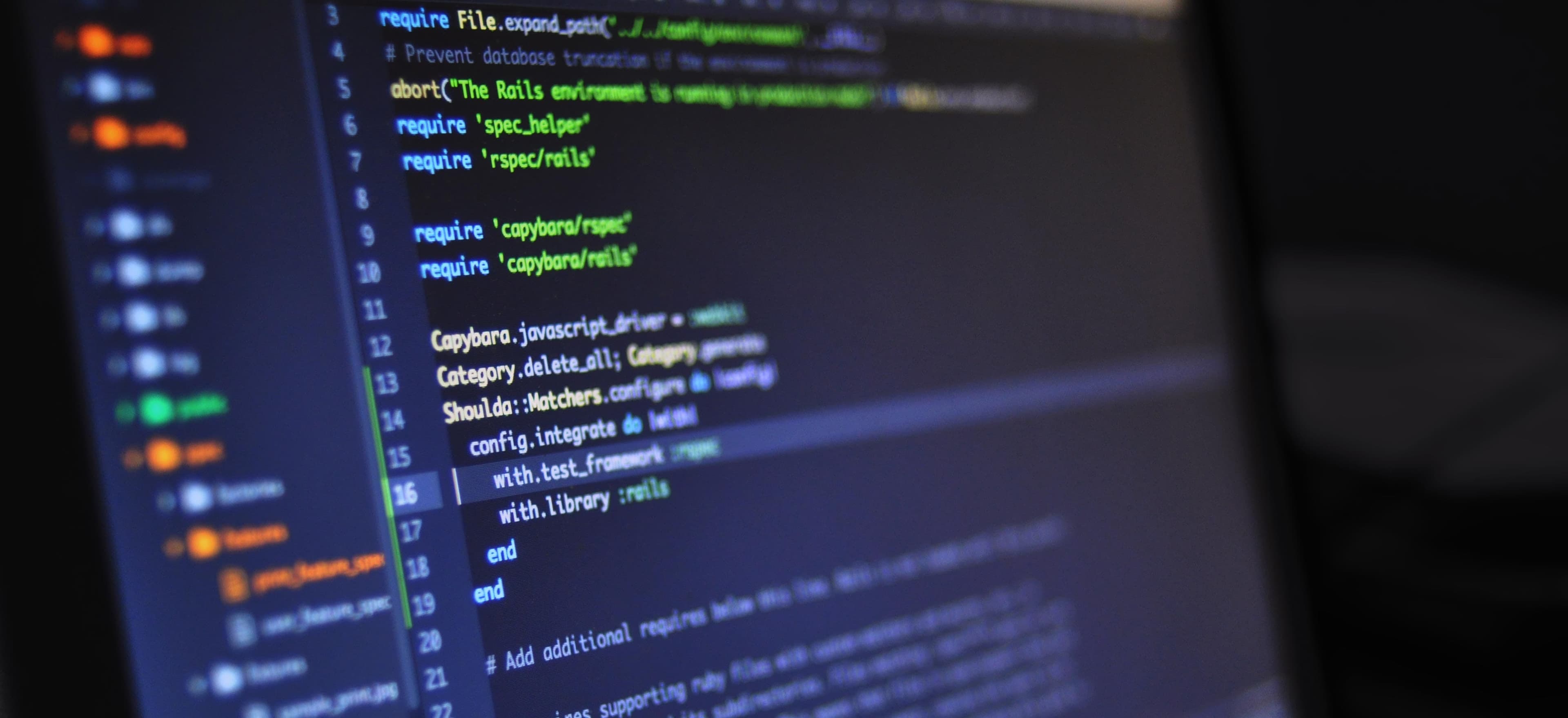
- Published on
Common Pitfalls When Using JSON Item Reader/Writer in Spring Batch
As data processing becomes increasingly critical to business applications, Spring Batch offers robust solutions for handling large volumes of data. Among these solutions, the JsonItemReader
and JsonItemWriter
are particularly useful for reading from and writing to JSON data sources. However, developers often encounter pitfalls during their implementation. This blog post explores common issues faced when using JSON Item Reader and Writer in Spring Batch, providing practical insights and code examples to avoid these traps.
Understanding JSON Item Reader and Writer
Before diving into common pitfalls, let’s clarify what the JSON Item Reader and Writer do in Spring Batch.
-
JsonItemReader: Reads data from a JSON source and maps it to an object. This is particularly useful for reading data from REST APIs or JSON files.
-
JsonItemWriter: Writes data to a JSON output, making it essential for outputting results back to a file or a database in JSON format.
Using these components effectively can significantly streamline data processing tasks. However, as with any technology, pitfalls exist.
Common Pitfalls
1. Mapping Issues
One of the most frequent issues developers encounter is improper mapping. JSON data often contains nested structures, and the mapping to Java objects may not be direct.
Code Example: Improper Mapping
Consider a JSON structure like this:
{
"id": 1,
"name": "John Doe",
"address": {
"street": "123 Main St",
"city": "New York"
}
}
An incorrect Java class might look like this:
public class User {
private int id;
private String name;
private String address; // Incorrectly defined as a String
// Getters and Setters
}
Pitfall Explanation
Here, the address
field should be a separate class, not a String. The correct class structure should look like:
public class Address {
private String street;
private String city;
// Getters and Setters
}
public class User {
private int id;
private String name;
private Address address; // Correctly mapped
// Getters and Setters
}
2. Error Handling
Error handling can be tricky with JSON Item Readers and Writers. If the JSON source has invalid data, it can cause the batch job to fail without proper exception handling.
Code Example: Enhancing Error Handling
Implementing a SkipPolicy
can help manage errors effectively.
public class CustomSkipPolicy implements SkipPolicy {
@Override
public boolean shouldSkip(Throwable t, int skipCount) {
return t instanceof JsonMappingException && skipCount <= 5;
}
}
Pitfall Explanation
Failing to implement a skip policy can cause your job to abort on the first encountered issue, while applying a smart skip policy allows you to continue processing while logging the error. The above example skips up to 5 JSON mapping exceptions.
3. Performance Issues with Large JSON Files
Spring Batch is designed for high throughput, but using JsonItemReader
with large JSON files can cause performance bottlenecks.
Code Example: Streaming
Instead of loading an entire JSON file at once, consider using streaming reads.
@Bean
public JsonItemReader<User> reader() {
JsonItemReader<User> jsonItemReader = new JsonItemReader<>();
jsonItemReader.setResource(new FileSystemResource("largeFile.json"));
jsonItemReader.setJsonObjectReader(new JacksonJsonObjectReader<>(User.class));
return jsonItemReader;
}
Pitfall Explanation
Avoid loading large files into memory. The use of streaming allows you to process large files in smaller chunks, minimizing memory usage and improving performance.
4. Not Configuring the ObjectMapper
The ObjectMapper
in your JSON reader and writer is crucial. Failing to configure it can lead to serialization and deserialization problems.
Code Example: Configuring ObjectMapper
Configuring an ObjectMapper
can be done as follows:
@Bean
public ObjectMapper objectMapper() {
ObjectMapper mapper = new ObjectMapper();
mapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
return mapper;
}
Pitfall Explanation
By default, the ObjectMapper is strict about unknown properties. Configuring it to ignore unknown properties can prevent crashes when the JSON structure changes unexpectedly.
5. Not Testing with Multiple JSON Structures
A common oversight is not testing the reader and writer with different JSON structures, especially when you expect variations in the input data.
Code Suggestion: Write Tests
To ensure robustness, write unit tests that validate against various JSON structures, including edge cases.
@Test
public void testJsonMapping() throws Exception {
String json = "{\"id\":1,\"name\":\"John Doe\",\"address\":{\"street\":\"123 Main St\",\"city\":\"New York\"}}";
User user = objectMapper().readValue(json, User.class);
assertEquals("John Doe", user.getName());
}
The Last Word
Using JsonItemReader
and JsonItemWriter
in Spring Batch provides seismic benefits for batch data processing. However, to leverage their full potential, developers must be cautious of common pitfalls such as incorrect mapping, inadequate error handling, performance issues with large files, misconfiguration of the ObjectMapper, and insufficient testing.
By keeping these pitfalls in mind and implementing the suggested best practices and code snippets, you'll ensure that your Spring Batch jobs run efficiently and reliably.
Further Reading
For additional context, you may find these resources helpful:
By being aware of these potential pitfalls and applying the corrective measures discussed, you can confidently work with JSON in Spring Batch, thus improving the quality and reliability of your data processing jobs. Happy coding!