Reduce Java Docker Image Size: Tackling Bloat with Alpine
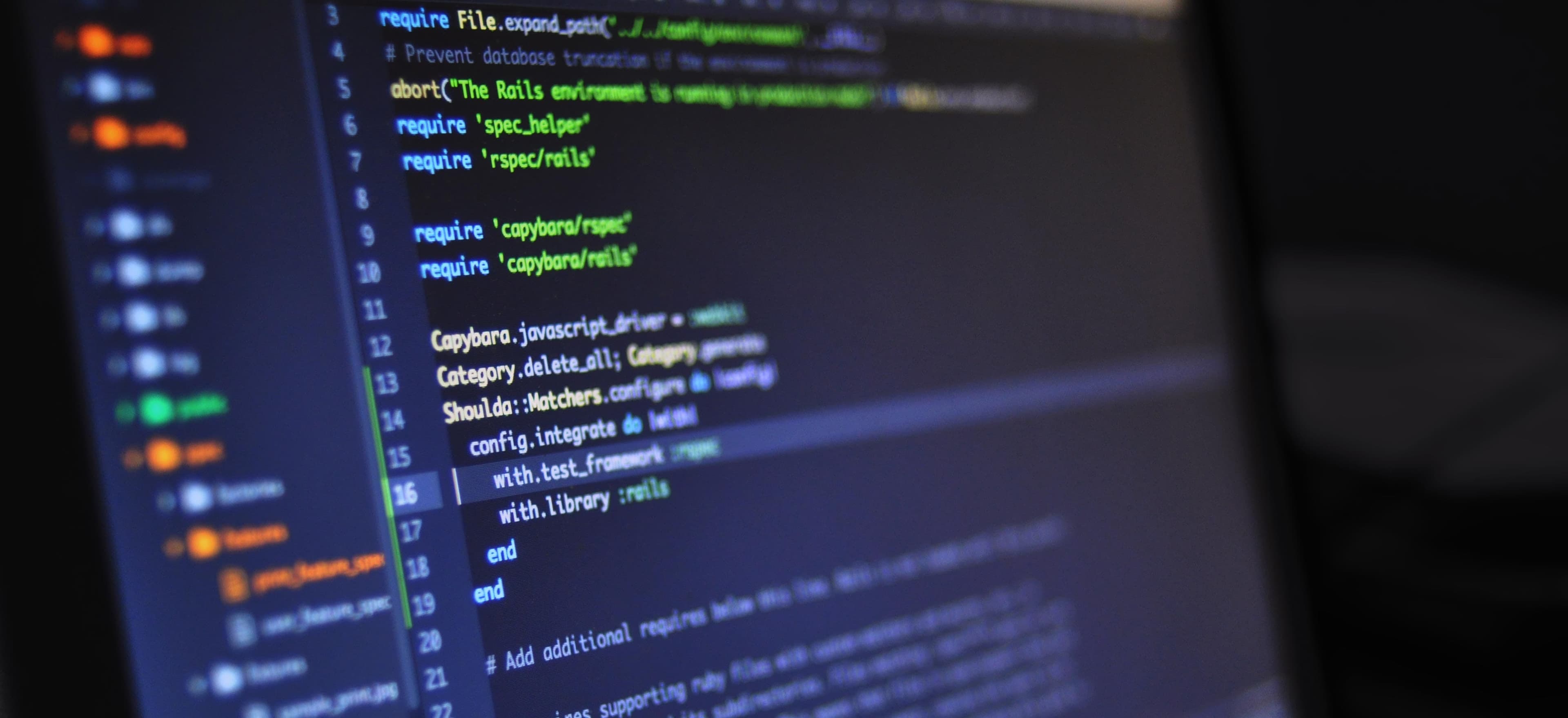
- Published on
Reduce Java Docker Image Size: Tackling Bloat with Alpine
In the world of modern application development, the size of your Docker images can have a significant impact on deployment speed, storage costs, and overall application performance. Java applications, known for their robustness, often lead to hefty Docker images due to the inclusion of the Java Development Kit (JDK) and all dependencies.
In this blog post, we will explore how to reduce Java Docker image size by leveraging Alpine Linux, a lightweight distribution that provides a minimal base image for Docker containers. We will cover the steps required to create a slim Java image using Alpine, provide code snippets for better understanding, and share best practices.
What is Alpine Linux?
Alpine Linux is a security-oriented, lightweight Linux distribution based on musl libc and busybox. Its small size makes it ideal for Docker containers, ensuring that your images are as compact as possible—perfect for enhancing performance and reducing deployment times.
Why Optimize Docker Image Size?
-
Faster Deployments: Reduced image sizes lead to quicker pull times from registries, which can significantly speed up deployment.
-
Improved Performance: Smaller images can reduce overhead in memory and CPU usage, thus improving overall application performance.
-
Cost Efficiency: Minimizing the size of your Docker images can help lower storage and bandwidth costs when hosting containers in cloud environments.
Getting Started with a Java Application on Alpine
Before we start optimizing, let’s create a simple Java application.
// HelloWorld.java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
You can compile this simple program by using the JDK on your machine.
javac HelloWorld.java
This will generate a HelloWorld.class
file that can be executed with the Java Runtime Environment (JRE).
Creating a Dockerfile for Your Java Application
Here’s an example of how to structure a basic Dockerfile that uses the OpenJDK base image (which is typically larger).
# Use OpenJDK image
FROM openjdk:17
COPY HelloWorld.class /app/
WORKDIR /app
CMD ["java", "HelloWorld"]
Running the above Dockerfile will give you a bulky image since the OpenJDK image itself is around 600 MB. Now let's dive into using Alpine.
Transitioning to Alpine
First, we need to switch to an OpenJDK version that is compatible with Alpine. Fortunately, official lightweight OpenJDK Alpine images are available.
Here’s how to modify the existing Dockerfile:
# Use a lightweight OpenJDK Alpine image
FROM openjdk:17-alpine
COPY HelloWorld.class /app/
WORKDIR /app
CMD ["java", "HelloWorld"]
Build the Docker Image
You can now build this image with the following command:
docker build -t java-alpine-example .
Verifying the Image Size
To check the size of your Docker image, you can run:
docker images
You will note a significant reduction in size compared to the earlier image. While the OpenJDK base image could be around 600 MB, the Alpine variant is roughly 140 MB.
Why Use openjdk:17-alpine
?
-
Reduced Size: The Alpine image is much smaller, thus reducing the overall application footprint.
-
Speed: Smaller images lead to faster downloads and deployments, particularly suitable for CI/CD pipelines.
-
Security: Alpine’s minimalistic approach means fewer packages, resulting in a smaller attack surface.
Multi-Stage Builds for Further Reduction
For production-ready applications, you may want to leverage multi-stage builds to further minimize your image size. This approach allows you to compile your Java application and then copy over only the necessary artifacts.
Here is an example:
# Stage 1: Build the application
FROM openjdk:17-alpine AS build
COPY HelloWorld.java /app/
WORKDIR /app
RUN javac HelloWorld.java
# Stage 2: Create the smaller image
FROM openjdk:17-alpine
COPY --from=build /app/HelloWorld.class /app/
WORKDIR /app
CMD ["java", "HelloWorld"]
Explanation of Multi-Stage Builds
-
Stage 1: We use the OpenJDK image to compile our application. Once compiled, only the
.class
files are exported. This phase ensures that we are not carrying over the entire JDK or build tools into the final image. -
Stage 2: This image is based on Alpine and contains only the compiled classes, making it even smaller. You’re shipping only what you need, keeping your image lean.
Final Thoughts
Using Alpine can drastically reduce the size of Java Docker images, leading to a more efficient and manageable environment. While there can be trade-offs concerning compatibility with certain libraries and tools, the performance benefits often outweigh these concerns.
For more advanced understanding and scenarios, consider diving deeper into discussions surrounding Docker optimization and building lean images.
Additional Recommendations:
- Always monitor your image sizes and optimize as required.
- Regularly update your base images to include security patches.
- Explore further optimizations using tools like GraalVM Native Images if applicable.
By employing these best practices, not only will you enhance your application’s performance, but you will also become adept at managing your Docker images with greater efficiency.
Resources
Your lean Java Docker images await! Start implementing these strategies today and experience the difference. Happy coding!
Checkout our other articles