Java's Evolution: Why It Must Adapt to Survive
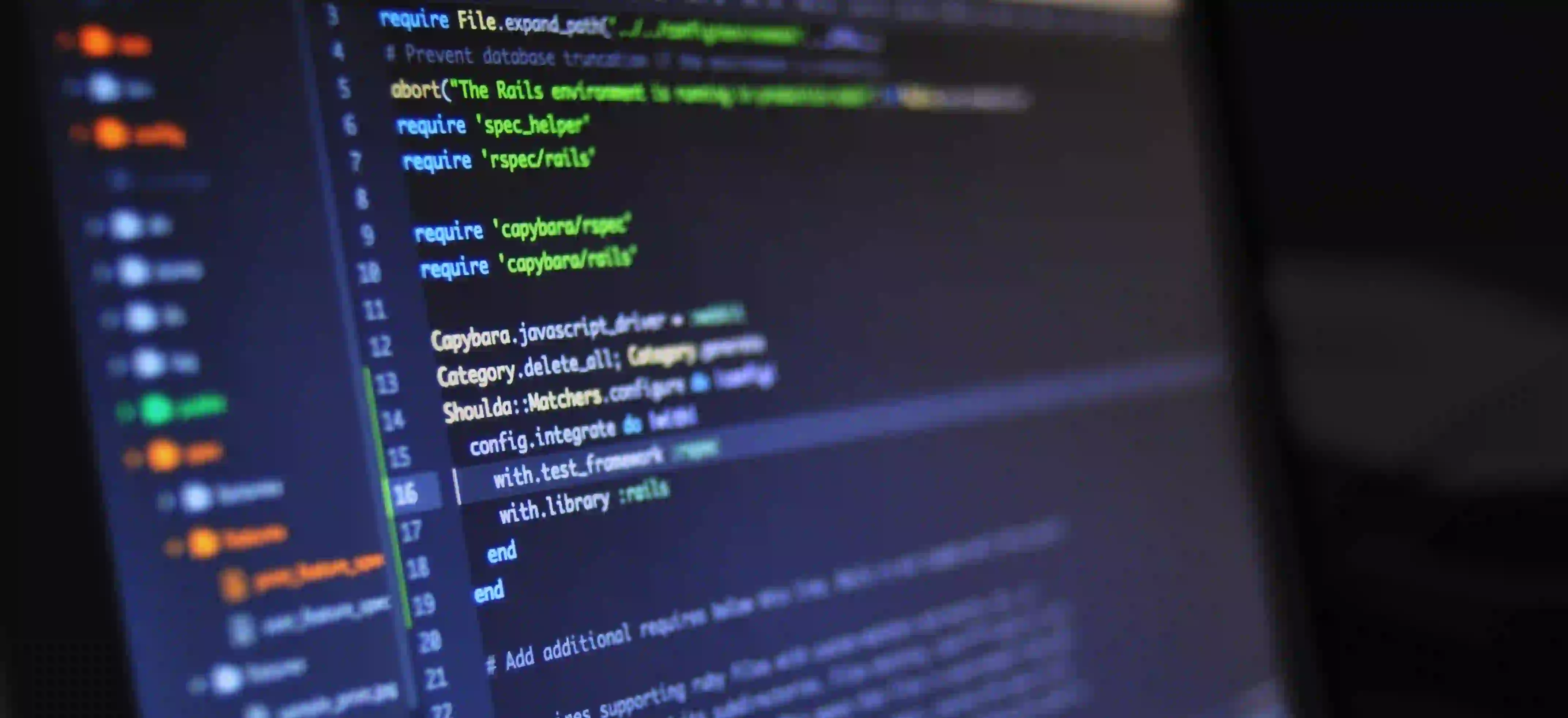
Java's Evolution: Why It Must Adapt to Survive
In the realm of programming languages, few have had a more enduring legacy than Java. Created in 1995 by Sun Microsystems, Java was designed to be portable, secure, and robust. Its "write once, run anywhere" (WORA) capability turned it into a favorite among developers. However, as technology continues to evolve, Java must also adapt to meet the demands of contemporary software development.
In this blog post, we will explore the evolution of Java, the factors driving its adaptation, and the latest features that will help it remain relevant in the competitive landscape of programming languages.
The Impact of Rapid Technological Change
The tech industry is characterized by lightning-fast change. With the advent of artificial intelligence, machine learning, microservices, and cloud computing, the requirements of modern applications have shifted dramatically. For Java to maintain its relevancy, it must evolve and incorporate characteristics tailored to these emerging trends.
Performance Enhancements
One of the primary criticisms leveled against Java is its performance compared to languages like C++ or Go. Historically, Java's garbage collection and runtime efficiency posed challenges in high-performance applications.
To counter this, recent versions have introduced new optimizations. For instance, with Java 9’s introduction of the Java Platform Module System, applications can be more modular, improving performance by creating smaller, faster applications.
module my.module {
exports com.example.myapp;
requires java.sql;
}
In this snippet, we define a module that exports a package and requires another module. This modularity allows for better organization and potentially better performance at runtime.
Embracing Functional Programming
With the rise of functional programming concepts, Java had to find a way to incorporate these ideas to remain competitive. Java 8 introduced major features like Lambdas and Streams, allowing developers to write more concise and expressive code.
Here’s a simple example of using Streams to filter a list:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class FunctionalExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
System.out.println(filteredNames); // Output: [Alice]
}
}
Using Streams allows us to process collections in a functional style. This increases code readability and maintainability, crucial components in modern software development.
Enhancing Concurrency Support
As more applications move toward distributed architectures, concurrency management becomes critical. Java recognized this and has introduced features like CompletableFuture and reactive programming frameworks.
With CompletableFuture, developers can write asynchronous non-blocking code that’s easier to understand:
import java.util.concurrent.CompletableFuture;
public class AsyncExample {
public static void main(String[] args) {
CompletableFuture.supplyAsync(() -> {
// Simulating a long-running task
return "Result from asynchronous task";
}).thenAccept(result -> {
System.out.println(result); // Output the result
});
}
}
Java's CompletableFuture improves the ability to handle tasks in parallel, which is essential for todays high-throughput applications.
The Role of the Community and Ecosystem
Adapting to modern demands wouldn't be possible without the vibrant Java community and ecosystem. The plethora of frameworks, libraries, and tools developed around Java fuels its evolution. The popularity of frameworks such as Spring, Hibernate, and JavaFX demonstrates how the language can be adapted to various use cases, from web applications to microservices.
Evolving into Cloud-Native Applications
As cloud computing becomes the norm, Java has initiated moves to become more cloud-friendly. Projects like Spring Boot and MicroProfile are specifically designed for cloud-native Java applications. These frameworks streamline the development of microservices, enabling developers to deploy applications quickly and efficiently.
Here's a quick snippet demonstrating the simplicity of a Spring Boot REST Controller:
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api")
public class HelloWorldController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, World!";
}
}
In this controller, we define a simple REST API endpoint. Spring Boot makes it incredibly easy to create production-ready applications with minimal configuration.
Java's Ongoing Challenges
Despite its strengths, Java faces several challenges. One critical concern is the competition from other languages that are specifically designed for speed and efficiency, such as Go or Rust. These languages capitalize on simpler syntax and concurrency models.
Java will need to make the following adjustments to meet this challenge:
-
Increase Performance: Continue optimizing runtime performance and memory consumption.
-
Simplify Syntax: Improve expressiveness without sacrificing type safety.
-
Maintain Relevance: Keep up with modern paradigms, such as reactive programming and functional programming.
The Future of Java
Looking ahead, Java's adaptability will hinge on three key aspects:
Continuous Improvement
Java is scheduled for updates every six months, which means continuous improvement is built into its lifecycle. Each new version introduces features that streamline development and enhance performance.
Community-Driven Innovation
The open-source nature of Java ensures that the community can innovate freely. Features like the Project Loom (for lightweight concurrency) and Project Panama (for native code integration) promise exciting future developments.
Ecosystem Expansion
With a robust ecosystem of libraries and frameworks, Java will continue benefiting from innovations built on top of it. The constant evolution of cloud services, coupled with an active Java community, ensures that Java will find its place in future technological advancements.
In Conclusion, Here is What Matters
Java's journey from a simple programming language to a cornerstone of enterprise development has been remarkable. While the underlying principles of Java remain essential, its ability to adapt and embrace change will determine its survival in the fast-paced tech environment. By focusing on performance, functional programming, cloud-native applications, and leveraging an enthusiastic community, Java is set to continue its legacy for years to come.
For further insights into Java's evolution, consider visiting Oracle's Java Resources or exploring the Java documentation.
Java’s narrative is not merely about preservation; it is about evolution, ensuring that it remains an essential tool in a developer's repository. As we embrace the technological advancements of the 21st century, we can look forward to a vibrant and exciting future for Java.