Debugging Common Issues in JavaFX Tile Engine Development
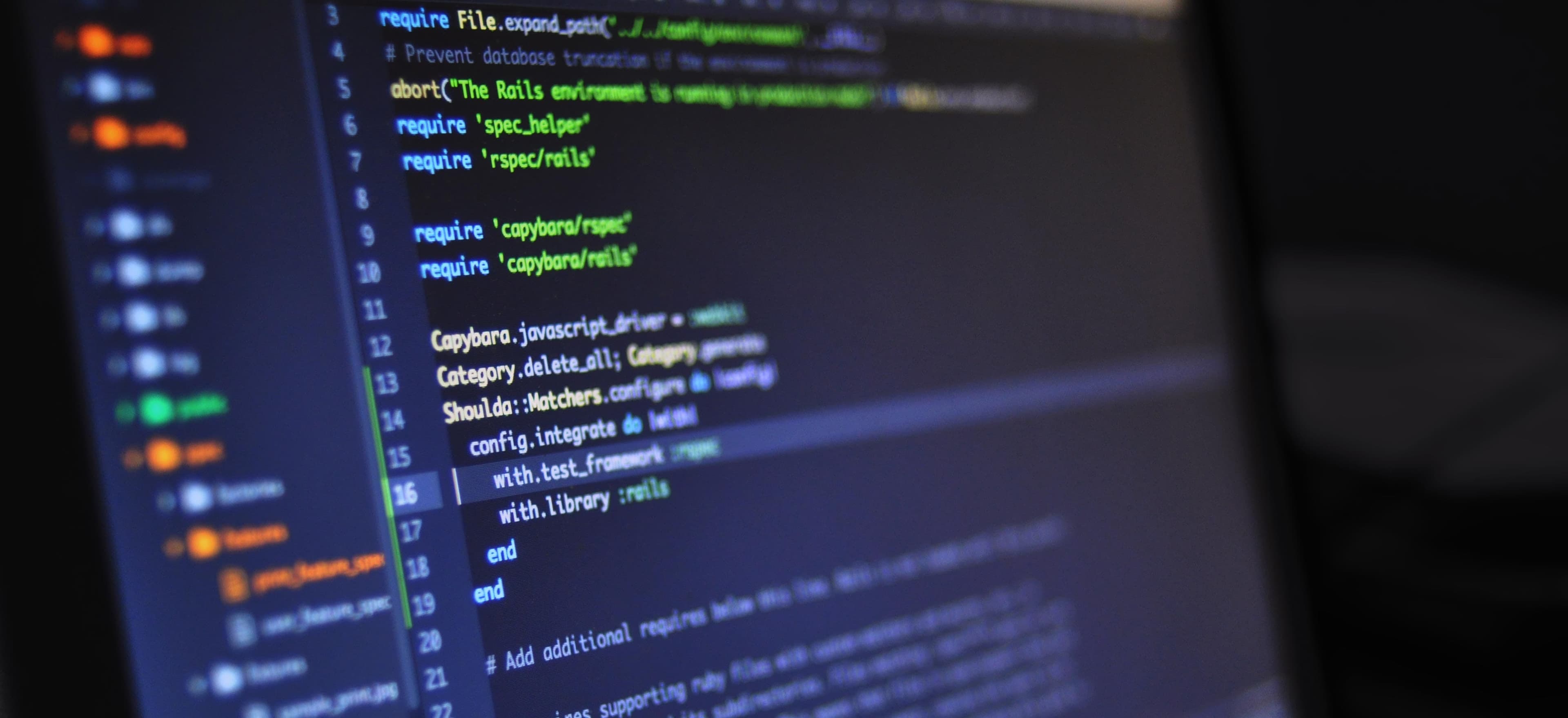
- Published on
Debugging Common Issues in JavaFX Tile Engine Development
JavaFX is a powerful framework for building rich desktop applications in Java. One of its popular use cases is game development, particularly for creating 2D games using a tile engine. However, like any development process, it comes with its own set of challenges. In this blog post, we will explore common issues encountered in JavaFX tile engine development and provide actionable solutions to debug them effectively.
Understanding the JavaFX Tile Engine
Before diving into debugging, let’s briefly understand what a tile engine is. A tile engine allows developers to create a game world using a grid composed of tiles, which represent different elements of the game (like terrain, obstacles, etc.). This modular approach makes it easy to design and manage level layouts.
Common Issues
-
Tiles Not Appearing
Problem: Tiles fail to render on the screen.
Solution: Check the rendering code. You need to ensure that the tiles are being drawn correctly on the canvas or the scene. For instance:
canvas.getGraphicsContext2D().drawImage(tileImage, x, y);
Make sure that:
- The
tileImage
is correctly initialized. - The
x
andy
coordinates are within the visible area. - The canvas or your game loop is correctly updating and displaying.
Often, if images do not load correctly, it can lead to blank spaces in the expected tile positions. Verify that the image paths are correct and that images are being loaded asynchronously if needed.
- The
-
Incorrect Tile Placement
Problem: Tiles are being placed in the wrong location.
Solution: Debugging tile placements often involves keeping track of your indexes and grid coordinates. When updating the tile grid, for example:
public void setTile(int x, int y, Tile tile) { if (x >= 0 && y >= 0 && x < width && y < height) { grid[x][y] = tile; } }
Ensure that:
- The coordinates
x
andy
are correctly interpreted according to your tile grid. - Adjust tile placement logic to match your grid structure.
- The coordinates
-
Lagging or Stuttering Performance
Problem: The game is running too slowly.
Solution: Performance issues are typically related to unexpected inefficiencies in the code. Start by examining your game loop and rendering calls:
public void render() { GraphicsContext gc = canvas.getGraphicsContext2D(); gc.clearRect(0, 0, canvas.getWidth(), canvas.getHeight()); for (Tile[] row : grid) { for (Tile tile : row) { if (tile != null) { gc.drawImage(tile.getImage(), tile.getX(), tile.getY()); } } } }
Consider:
- Only redrawing the portion of the screen that needs updating.
- Using double buffering techniques to prevent flickering and improve performance.
- Profiling your application to find bottlenecks, using tools like Java VisualVM.
-
Key Input Issues
Problem: The game does not respond to keyboard inputs.
Solution: Input handling needs to be carefully implemented. For example:
scene.setOnKeyPressed(event -> { switch (event.getCode()) { case LEFT: // move left player.moveLeft(); break; case RIGHT: // move right player.moveRight(); break; // Handle other keys } });
Confirm that:
- The focus is set on the correct node to capture key inputs.
- Your key event handlers are correctly set up.
-
Memory Leaks
Problem: The application consumes excessive memory.
Solution: Memory leaks can lead to crashes and poor performance. Check for lingering references to unused objects. Utilize the Java garbage collector and make sure to nullify references when objects are no longer needed.
For instance, if you’re using large images for tiles, ensure they are disposed of properly:
tileImage = null; // Release reference to allow GC to collect
Regularly profile your application to monitor memory usage with tools like JVisualVM.
Debugging Strategies
Logging
The first rule of debugging is to log what’s happening. Use Java's logging framework to log important events in your game, such as tile placements, user inputs, and rendering phases. For example:
logger.info("Tile placed at " + x + ", " + y);
This provides you with a clear and trackable history of your application’s runtime behavior.
Breakpoints and Debuggers
Use IDEs like IntelliJ IDEA or Eclipse with integrated debugging tools. Set breakpoints to inspect variable values and execution flow step by step, ensuring every part of your code behaves as expected.
Unit Testing
Incorporate unit tests for critical components in your tile engine. Test functions like rendering and tile placement in isolation. Libraries like JUnit can help simplify this process:
@Test
public void testSetTile() {
TileEngine engine = new TileEngine(5, 5);
engine.setTile(1, 1, new Tile());
assertNotNull(engine.getTile(1, 1), "Tile should be placed correctly.");
}
Game State Management
Make sure to properly manage your game state, especially if the game contains different levels or screens. Debugging issues often arise when transitioning between states or when game logic doesn’t update in sync.
Final Considerations
Debugging a JavaFX tile engine can seem daunting at first, but by systematically addressing each common issue, you can enhance both your skills and application performance.
This involves understanding your code’s flow and maintaining clear structures for handling input, rendering, and game state. Utilize tools like logging, debugging, and unit testing to methodically track down and resolve issues.
Finally, remember that the game development community is rich with resources, including forums and tutorials. Engaging with others can provide further insights and troubleshooting ideas, enabling you to build a robust tile engine.
If you're looking for more detailed information on JavaFX game development, check out Oracle's JavaFX Documentation. Happy coding!
Checkout our other articles