Mastering Exception Handling: Common Mistakes to Avoid
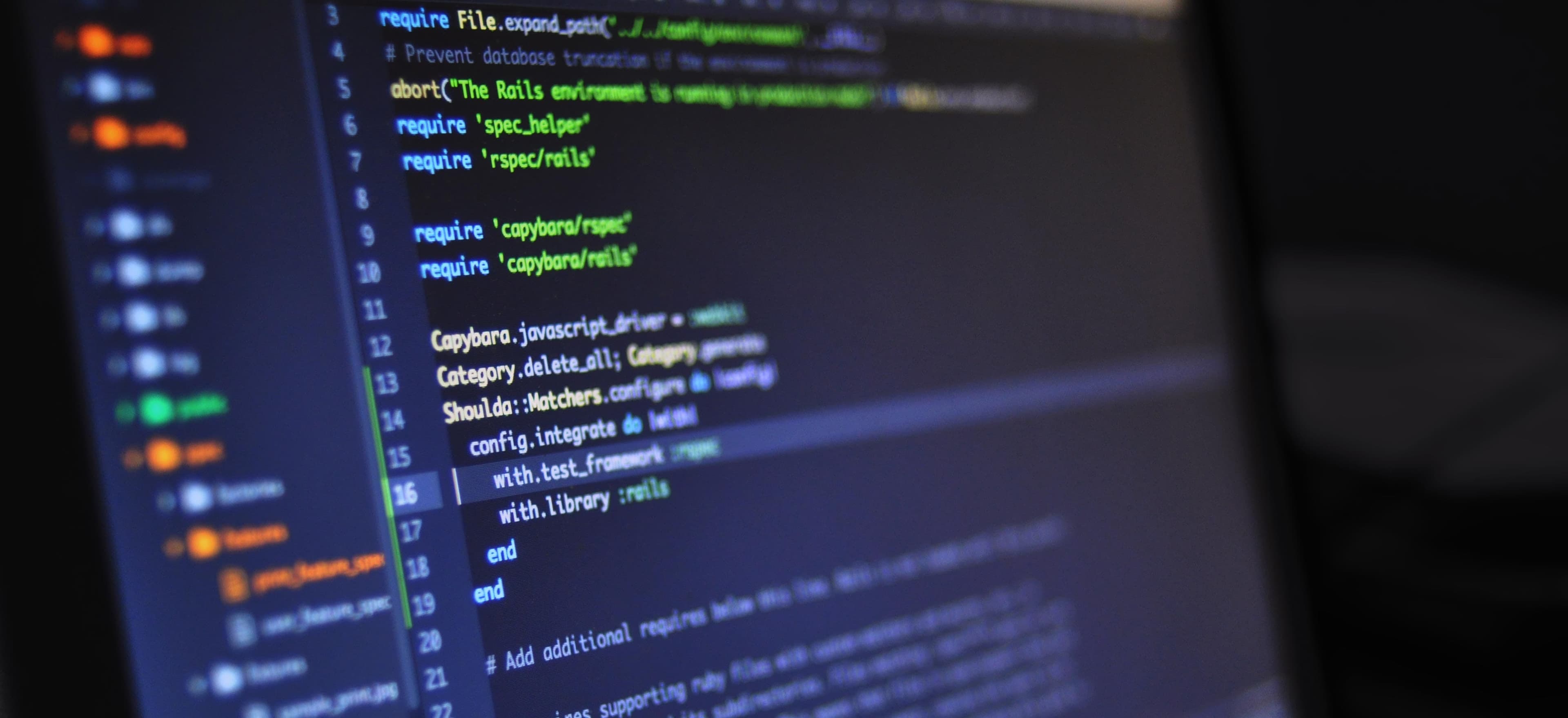
- Published on
Mastering Exception Handling: Common Mistakes to Avoid
Exception handling is a crucial aspect of programming in Java. It not only makes your code more robust but also ensures that your applications can gracefully manage unexpected situations. However, there are several common pitfalls that developers encounter when dealing with exceptions. In this blog post, we will explore these mistakes and provide you with best practices to master exception handling in Java.
Understanding Exceptions
Before diving into the common mistakes, it’s essential to understand what exceptions are in Java. An exception is an event that disrupts the normal flow of a program's execution. Java provides a robust mechanism for handling exceptions through the try
, catch
, finally
, throw
, and throws
keywords.
Here's a basic example:
public class ExceptionExample {
public static void main(String[] args) {
try {
int result = divide(10, 0);
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
System.out.println("Caught an exception: " + e.getMessage());
} finally {
System.out.println("This block always executes.");
}
}
public static int divide(int a, int b) {
return a / b; // potential for division by zero
}
}
Explanation:
- try: Wraps the code that might throw an exception.
- catch: Catches and handles the exception.
- finally: Executes irrespective of whether an exception occurred or not.
This basic structure allows for handling errors cleanly, but the way we handle exceptions can lead to poor practices. Let's explore the common mistakes and how to avoid them.
Common Mistakes in Exception Handling
1. Catching Generic Exceptions
One of the most critical mistakes developers make is catching generic exceptions, particularly Exception
or Throwable
. This practice makes it difficult to identify the source of problems, as it can obscure valuable error information.
Example of a Poor Practice:
try {
// some risky operation
} catch (Exception e) {
System.out.println("An error occurred.");
}
Solution: Always catch the most specific exception first.
try {
// risky operation
} catch (ArithmeticException e) {
System.out.println("Can't divide by zero.");
} catch (NullPointerException e) {
System.out.println("Null reference encountered.");
}
By targeting specific exceptions, you can provide more meaningful error messages and better control the flow of your application.
2. Ignoring Exception Handling
Another common mistake is to ignore exceptions altogether. It's tempting to simply log the exception and move on, but this can lead to inconsistent application behavior and obscure underlying issues.
Example of Inadequate Handling:
try {
// some database operation
} catch (SQLException e) {
// Just logging without taking action
e.printStackTrace();
}
Solution: Take appropriate action based on the exception. This might include retrying operations, aborting the current task, or informing the user.
try {
// some database operation
} catch (SQLException e) {
// Log and handle appropriately
System.err.println("Database operation failed: " + e.getMessage());
// Optionally, retry the operation or notify the user
}
3. Overusing Exception Handling
While it is essential to handle exceptions, overusing them for flow control can lead to code that is difficult to understand and maintain. Avoid using exceptions to manage normal operational logic.
Example of Overuse:
try {
// some logic that could fail
} catch (FileNotFoundException e) {
// This could actually happen often
}
Solution: Use exceptions sparingly. If a scenario is expected and should be handled, consider using conditional statements instead of exceptions.
if (fileExists("data.txt")) {
// Proceed with file operations
} else {
System.out.println("File not found, proceeding without it.");
}
4. Not Cleaning Up Resources
Failing to release resources such as database connections, file handles, or sockets can lead to memory leaks and performance issues. Always ensure that resources are properly closed.
Example of Resource Leak:
Connection conn = getConnection();
try {
// use connection
} catch (SQLException e) {
e.printStackTrace();
}
// Connection is not closed here
Solution: Use a finally
block or a try-with-resources statement to ensure that resources are always closed.
try (Connection conn = getConnection()) {
// use connection
} catch (SQLException e) {
e.printStackTrace();
}
// No need for finally; resource is automatically closed
5. Failing to Document Exceptions
Failing to document the exceptions that a method can throw is another common mistake. This puts the onus on the caller to guess what exceptions might arise.
Example of Undocumented Method:
public void readFile(String filePath) {
// method implementation
}
Solution: Always use Javadoc to document exceptions. This enhances code clarity and helps consumers of your API understand what to expect.
/**
* Reads a file from the given file path.
*
* @param filePath the path of the file to read
* @throws FileNotFoundException if the file does not exist
* @throws IOException if an I/O error occurs
*/
public void readFile(String filePath) throws FileNotFoundException, IOException {
// method implementation
}
Best Practices for Exception Handling
-
Use Checked Exceptions for Recoverable Conditions: Use checked exceptions for scenarios that a caller might want to recover from, such as file not found or invalid user input.
-
Use Unchecked Exceptions for Programming Errors: For errors that cannot be reasonably recovered from, such as
NullPointerException
orIndexOutOfBoundsException
, use unchecked exceptions. -
Consider Custom Exceptions: Create custom exceptions to represent specific error conditions in your application. This enhances clarity and allows for more targeted exception handling.
-
Log Relevant Information: When handling exceptions, include context (like user IDs or input parameters) in your logs to aid in troubleshooting.
-
Maintain Code Readability: Ensure that your exception handling code is readable and understandable. Avoid deep nesting of try-catch blocks.
In Conclusion, Here is What Matters
Mastering exception handling is essential for any Java developer. By avoiding common mistakes such as catching generic exceptions, ignoring exceptions, and not cleaning up resources, you can write robust and maintainable code. Always remember to document potential exceptions and use best practices to improve the quality of your applications.
For more in-depth understanding of exceptions, you can explore the official Java Documentation.
Effective exception handling not only enhances stability but also leads to a better user experience. Start refining your approach today!
Happy coding!