Solving Java Puzzles: Common Traps Between OCA Parts
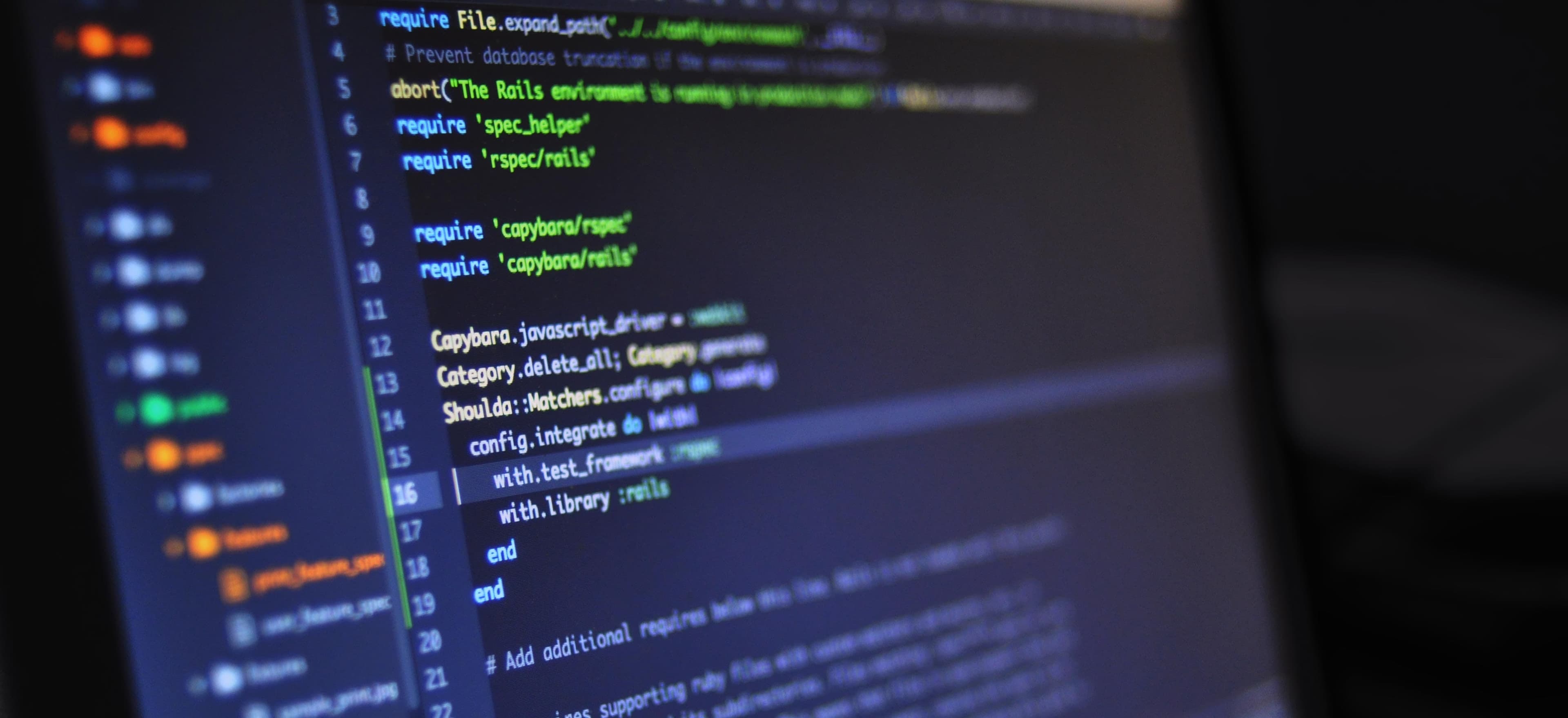
- Published on
Solving Java Puzzles: Common Traps Between OCA Parts
Java is a robust programming language that comes with its own set of quirks and intricacies. Preparing for the Oracle Certified Associate (OCA) exam can be tricky, especially when it comes to understanding common traps or puzzles that often throw off candidates. In this blog post, we will explore some of the common pitfalls and how to navigate them, enhancing your Java skills along the way.
Overview of the OCA Certification
The OCA certification serves as an entry point for Java professionals. It covers fundamental concepts, including:
- Variables and Data Types
- Control Flow Statements
- Object-Oriented Programming
- Exception Handling
- Java APIs
Understanding these areas is crucial, but candidates often find themselves confronting tricky questions that test their depth of knowledge.
1. Object References
One common trap involves object references. Many candidates forget that Java handles objects differently than primitive types.
Example Code Snippet
class Cat {
String name;
Cat(String name) {
this.name = name;
}
void changeName(String newName) {
this.name = newName;
}
}
public class Main {
public static void main(String[] args) {
Cat cat1 = new Cat("Whiskers");
Cat cat2 = cat1;
cat2.changeName("Purrfect");
System.out.println(cat1.name); // Output: Purrfect
}
}
Commentary
In the snippet above, cat1
and cat2
point to the same Cat
object in memory. When cat2.changeName("Purrfect");
is called, it modifies the name
attribute of the single object both references point to.
Why This Matters:
Candidates often assume that cat1
and cat2
are independent but fail to grasp that they reference the same object, leading to unexpected results in similar questions.
2. The Final Keyword
The final
keyword in Java often leads to confusion, particularly regarding variables versus references.
Example Code Snippet
final int x = 10;
// x = 20; // This line would cause a compile-time error
final List<String> list = new ArrayList<>();
list.add("Hello"); // Okay
// list = new ArrayList<>(); // This line would cause a compile-time error
Commentary
- The
final
keyword on primitive types likeint
means the value cannot be changed. - However, using
final
on an object reference, such as aList
, means you cannot reassign that reference. Still, you can modify the contents of the list.
Why This Matters: Candidates often confuse the immutability of the reference versus the mutability of the object it points to. A mechanism to review these differences is crucial for not being misled during the exam.
3. Autoboxing and Unboxing
Autoboxing and unboxing can be tricky, particularly in mixed-type operations.
Example Code Snippet
Integer a = 5; // Autoboxing
int b = a; // Unboxing
System.out.println(a == b); // Output: true
a = null;
// int c = a; // This line would cause a NullPointerException at runtime
Commentary
The auto-conversion between primitive types and their corresponding wrapper classes is one of Java’s conveniences but can be deceptive. When a
is null, attempting to unbox it will result in a NullPointerException
.
Why This Matters:
Candidates may not notice that a potential NullPointerException
can occur during unboxing and need to be cautious with null-checks.
4. Variable Scopes
Variable scope can create confusion, especially when nested blocks are involved.
Example Code Snippet
public class ScopeTest {
public static void main(String[] args) {
int x = 10;
if (x == 10) {
int y = 20;
System.out.println("Inside the if block: " + y); // Output: 20
}
// System.out.println(y); // This line would cause a compile-time error
}
}
Commentary
In this snippet, y
is scoped locally to the if
block. Attempting to reference y
outside of that block leads to a compile-time error.
Why This Matters: Candidates often overlook variable scopes, leading to incorrect assumptions about where they can use variables.
5. Exception Handling
Understanding exception handling can determine your success in interviews and the OCA exam.
Example Code Snippet
public class ExceptionTest {
public static void main(String[] args) {
try {
System.out.println("Attempting to divide by zero...");
int result = 10 / 0; // ArithmeticException
} catch (ArithmeticException e) {
System.out.println("Caught an exception: " + e.getMessage());
} finally {
System.out.println("Finally block executed.");
}
}
}
Commentary
This snippet showcases how the catch
block handles exceptions. While an ArithmeticException
is thrown, the program does not crash; instead, the catch block gracefully handles it.
Why This Matters:
Many candidates neglect the finally
block, which executes regardless of whether an exception occurs or not. It’s essential in resource management.
Tips for Preparing for OCA
-
Practice Mock Tests: Make use of online platforms that offer mock tests. This helps you identify patterns of mistakes.
-
Deep Dive into the Java Documentation: Familiarize yourself with the Java API Documentation. Knowing where to find information quickly is invaluable.
-
Code Everything: Writing code snippets and small programs is the best way to understand how Java works under the hood.
-
Join Study Groups: Collaborating with peers can expose you to different problem-solving approaches and solutions.
-
Review Java Puzzles: Engage with puzzles designed for Java practice. Sites such as JavaRanch can provide real-world programming challenges.
Key Takeaways
Navigating the traps in OCA's Java curriculum requires a nuanced understanding of the language's behavior. While this guide covers some common pitfalls, your learning journey should continue beyond these puzzles.
The world of Java is vast and ever-evolving. As you tackle these challenges, remember that mistakes are merely stepping stones toward mastery. Embrace them, learn from them, and soon enough, you'll be well-equipped to conquer the OCA exam and advance your Java career!
Checkout our other articles