Resolving the HTTP 403 Forbidden Error in Java Applications
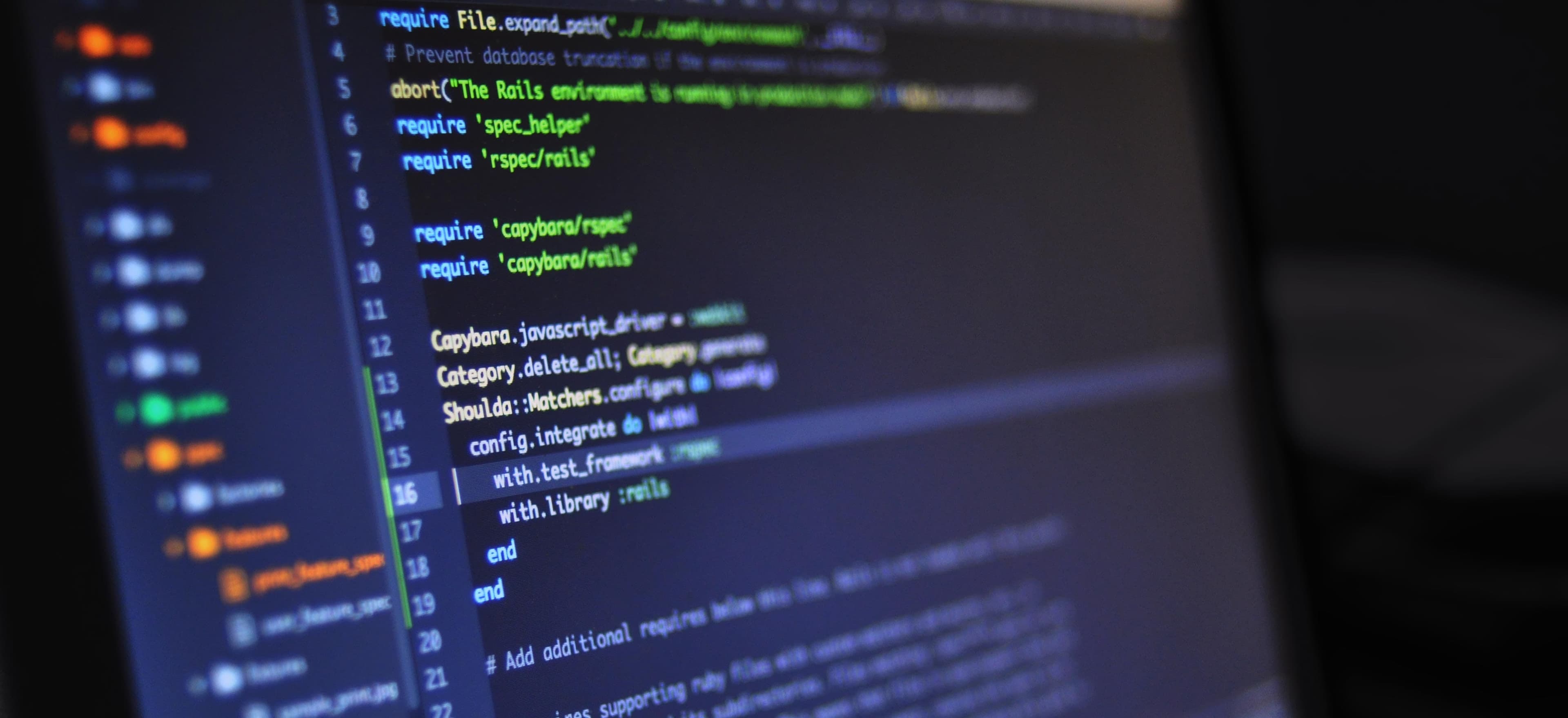
- Published on
Resolving the HTTP 403 Forbidden Error in Java Applications
When you develop Java applications, especially those involving web services and APIs, you might encounter various HTTP response statuses. One common status is the HTTP 403 Forbidden Error. This error indicates that the server understands the request but refuses to authorize it. Understanding and resolving this error can significantly enhance your application's functionality.
In this blog post, we will delve into the reasons behind the HTTP 403 error in Java applications, look at how to identify the source of the error, and explore strategies for resolving it.
Understanding the HTTP 403 Forbidden Error
The 403 Forbidden status code means that the client (you) does not have access rights to the content; the server is refusing to fulfill the request. This condition often arises due to permissions issues, server misconfigurations, or incorrect authentication.
Common Reasons for HTTP 403
-
Insufficient Permissions: The user account associated with the request may lack the necessary permissions to access the resource. This is common in systems requiring user roles.
-
Invalid IP Address: Servers may restrict access based on the originating IP address. If your IP is not white-listed, you will receive a 403 Forbidden error.
-
Incorrect URL: Accessing an invalid or restricted URL can lead to a 403 error.
-
Authentication Issues: If your application requires a token or session for access, an expired or missing token can trigger this error.
-
Web Server Configurations: Server-level configurations, such as
.htaccess
files in Apache or security settings in NGINX, may inadvertently block access.
Example Scenario
Imagine you are developing a RESTful API and trying to access a secured endpoint:
try {
URL url = new URL("https://api.example.com/secure-data");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setRequestProperty("Authorization", "Bearer YOUR_TOKEN_HERE");
int responseCode = conn.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
// successfully received response
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println("Response: " + response.toString());
} else if (responseCode == HttpURLConnection.HTTP_FORBIDDEN) {
System.out.println("Access Denied! HTTP error code: " + responseCode);
}
} catch (IOException e) {
e.printStackTrace();
}
In the above code snippet, we are attempting to connect to a secured endpoint using an access token. If the token is incorrect or the user lacks necessary permissions, a 403 status code will result.
Why the Code Matters
The block checks the response code after making an HTTP request. Specifically, if a 403 error is returned, it signifies that the client's attempt to access the resource has been denied. Proper error handling helps in diagnosing issues during development. Any developer leveraging REST APIs should have this in mind.
Resolving the 403 Forbidden Error
To resolve the HTTP 403 Forbidden error, you can take several approaches:
1. Check User Permissions
Ensure that the user account making the request has the required permissions. You might need to review your role-based access control (RBAC) configuration.
// Example of checking user roles before allowing access
if (userHasAccess(userId, requiredRole)) {
// proceed with processing
} else {
throw new SecurityException("User does not have access.");
}
2. Verify the Authentication Token
Ensure that the provided authentication token is valid and has not expired. Implementing token refresh logic is crucial for maintaining user sessions.
if (isTokenExpired(token)) {
throw new TokenExpiredException("Authentication token has expired!");
}
3. Check Server Configuration
For applications hosted on servers, verify the configurations. Misconfigurations can often lead to unauthorized responses.
For instance, if using an Apache server, check the contents of an .htaccess
file for any restrictive directives:
# Example .htaccess content that could cause a 403 error
<Files "restricted.html">
Order Allow,Deny
Deny from all
</Files>
In this example, the Deny from all
directive outright denies access to restricted.html
, leading to a possible 403 error for users attempting to access it.
4. Monitor IP Restrictions
If your application has IP whitelisting enabled, ensure your application requests originate from avitelisted IP.
5. Enhance Error Handling
Integrate better error handling in your Java codebase. An error response can allow the application to handle situations gracefully instead of failing abruptly.
// Handling possible 403 errors with detailed logging
} else if (responseCode == HttpURLConnection.HTTP_FORBIDDEN) {
log.error("403 Forbidden: " + conn.getResponseMessage());
throw new AccessDeniedException("Access denied to the requested resource");
}
6. Consult Documentation
When in doubt, referring to documentation or error logs can provide insights that help illuminate the cause of the issue. Resources like Mozilla Developer Network's HTTP Status Codes are invaluable.
The Bottom Line
HTTP 403 Forbidden errors can be frustrating, particularly in the early stages of Java application development. By understanding common causes and implementing the strategies discussed, you can resolve these errors efficiently.
Always remember: effective error handling and user experience design (UX) is crucial for application reliability.
If you encounter further issues, consider seeking help from community resources or consulting your framework's documentation for any specific guidance on authentication and authorization mechanisms.
For additional context, check the Spring Security documentation for implementing role and permission checks.
By maintaining awareness of these elements, your Java applications will be better positioned to handle authorization hurdles with grace. Happy coding!