Bridging the Gap: Agile Practices vs Architecture Challenges
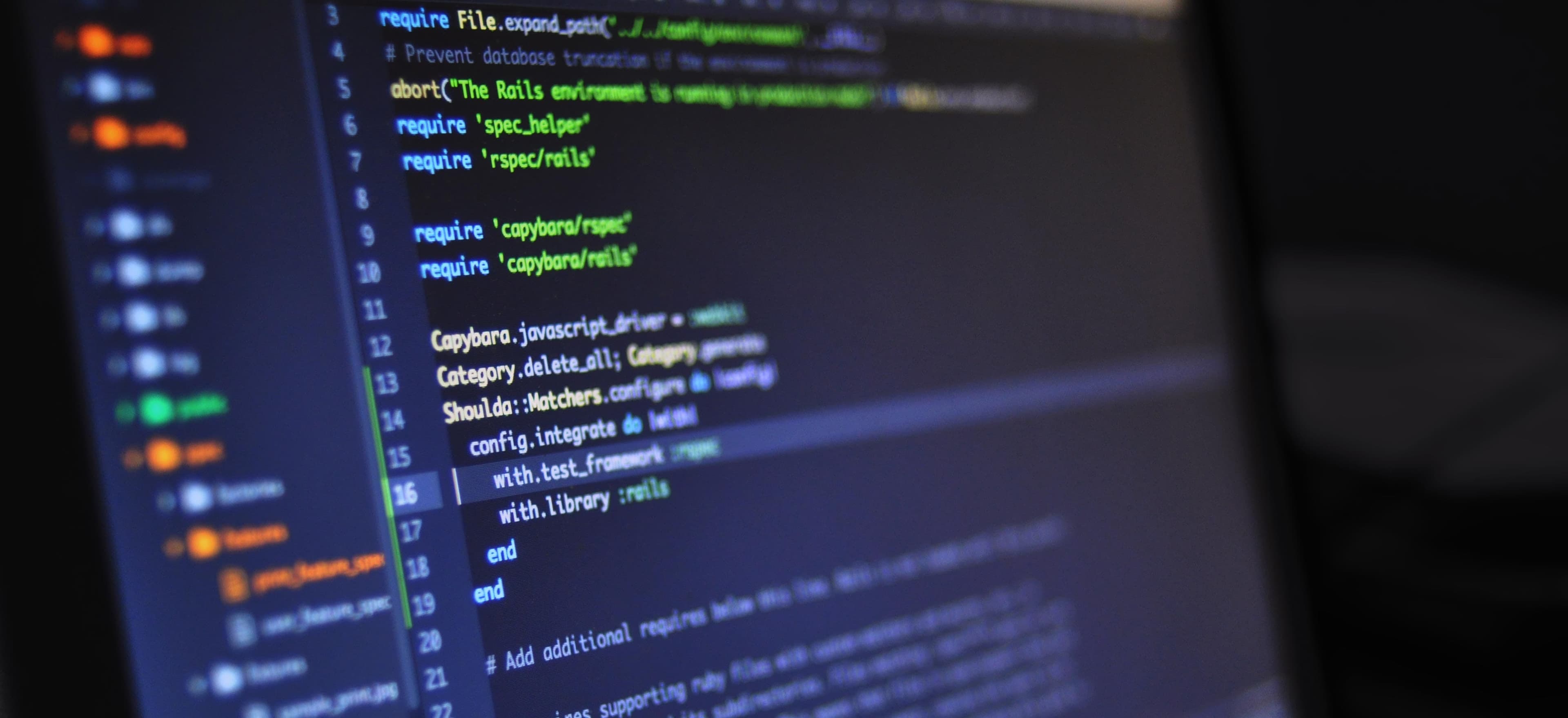
- Published on
Bridging the Gap: Agile Practices vs Architecture Challenges
In the fast-paced world of software development, the intersection of Agile practices and architectural concerns has emerged as a focal point for teams seeking a balance between speed and structural integrity. As Agile methodologies promote incremental development and responsiveness to change, architecture often appears rigid and resistant to transformation. This blog post dives into the nuances of this relationship, exploring how teams can bridge the gap between Agile practices and architecture challenges.
Understanding Agile Practices
Before we delve into the architecture aspect, let’s clarify what Agile development is. At its core, Agile is a methodology that emphasizes:
- Iterative Development: Building software in small, manageable increments.
- Collaboration: Encouraging communication between cross-functional teams.
- Customer Feedback: Adapting processes and products based on user feedback.
Key Frameworks and Principles
Among the various Agile frameworks, Scrum and Kanban are prominent. Both offer unique structures to foster flexibility and adaptability:
- Scrum: Uses fixed-length iterations called sprints, where teams deliver small, usable pieces of software.
- Kanban: Focuses on visualizing work, limiting work in progress, and improving flow.
For detailed insights about these frameworks, check out the Scrum Guide and Kanban Guide.
The Role of Software Architecture
Software architecture refers to the fundamental structures of a software system and the discipline of creating these structures. It involves:
- Defining Components: What parts the system consists of and how they interact.
- Establishing Patterns: Using proven design patterns to solve recurring problems.
- Ensuring Quality Attributes: Factors such as scalability, performance, and maintainability.
Common Architectural Styles
Understanding different architectural styles can help us identify potential challenges when integrating them with Agile practices:
- Monolithic Architecture: A single, unified software system. Simple but often inflexible.
- Microservices Architecture: An approach that divides applications into smaller services. Offers greater scalability but requires complex inter-service communication.
Each style has its pros and cons. Monoliths are easier to manage for small teams, while microservices can scale better for larger applications.
Bridging the Gap: The Challenges
Challenge 1: Changing Requirements
Agile's nature allows frequent changes in project requirements. While this is beneficial for meeting customer needs, it can create tension for architectural decisions made early in the project cycle.
Solution: Adopt a flexible architecture approach. Design systems that allow for easy updates without heavy refactoring. For example, implement strategies such as the Strategy Pattern in Java:
// Strategy interface for sorting
public interface SortingStrategy {
void sort(List<String> data);
}
// Concrete strategy for BubbleSort
public class BubbleSort implements SortingStrategy {
public void sort(List<String> data) {
// Bubble sort implementation
}
}
// Context class
public class Sorter {
private SortingStrategy strategy;
public Sorter(SortingStrategy strategy) {
this.strategy = strategy;
}
public void setStrategy(SortingStrategy strategy) {
this.strategy = strategy;
}
public void sortData(List<String> data) {
strategy.sort(data);
}
}
In this code snippet, we use the Strategy Pattern to allow sorting strategies to be changed at runtime, favoring adaptiveness.
Challenge 2: Technical Debt
In Agile environments, rushing to deliver working software can lead to technical debt—shortcomings in design or code quality that may require rework.
Solution: Establish a regular refactoring cycle. Encourage teams to prioritize code quality by utilizing tools such as SonarQube for continuous inspection of code quality.
Challenge 3: Communication Gaps
Poor communication between architects and Agile teams can result in misaligned goals. While Agile emphasizes collaboration, it can sometimes overlook architectural concerns.
Solution: Foster a culture of collaboration. Organize regular workshops where architects and Agile teams discuss design principles, trade-offs, and feedback loops.
Best Practices for Integration
To successfully marry Agile methodologies with architectural best practices, consider the following strategies:
1. Foster Incremental Architecture
Consider an approach where architecture evolves iteratively rather than being designed in full upfront. This evolution allows the architecture to adapt alongside features being developed.
2. Define Architectural Sprint Goals
Set specific architecture-focused goals within your sprints. This could involve refactoring code, assessing the performance of existing architecture, or dividing monoliths into microservices.
3. Document Architectural Decisions
Utilize lightweight documentation techniques to track architectural decisions. By maintaining a clear decision log, teams can understand the context behind architecture choices and the rationale for those decisions.
4. Create Shared Understanding
Ensure that everyone in the team, from developers to product owners, understands the architectural vision. Tools like C4 Model can help provide a clear visual representation of systems, making it easier to communicate designs.
To Wrap Things Up
Bridging the gap between Agile practices and architectural challenges is not a trivial task, but it is certainly achievable. By adopting flexible architectural strategies, fostering collaboration, and embracing incremental improvements, teams can create robust software that is receptive to change.
Ultimately, the goal is to build systems that not only meet current needs but can also evolve and grow with the demands of tomorrow. Implement these strategies in your workflow, and watch how they transform your engineering process.
Have you faced challenges integrating Agile practices with architectural decisions? We'd love to hear your thoughts and experiences in the comments below.
For further reading on Agile practices, check out The Agile Manifesto, and for insights into software architecture, explore Martin Fowler's articles on architecture.