Mastering Long Polling in Tomcat with Spring: Common Pitfalls
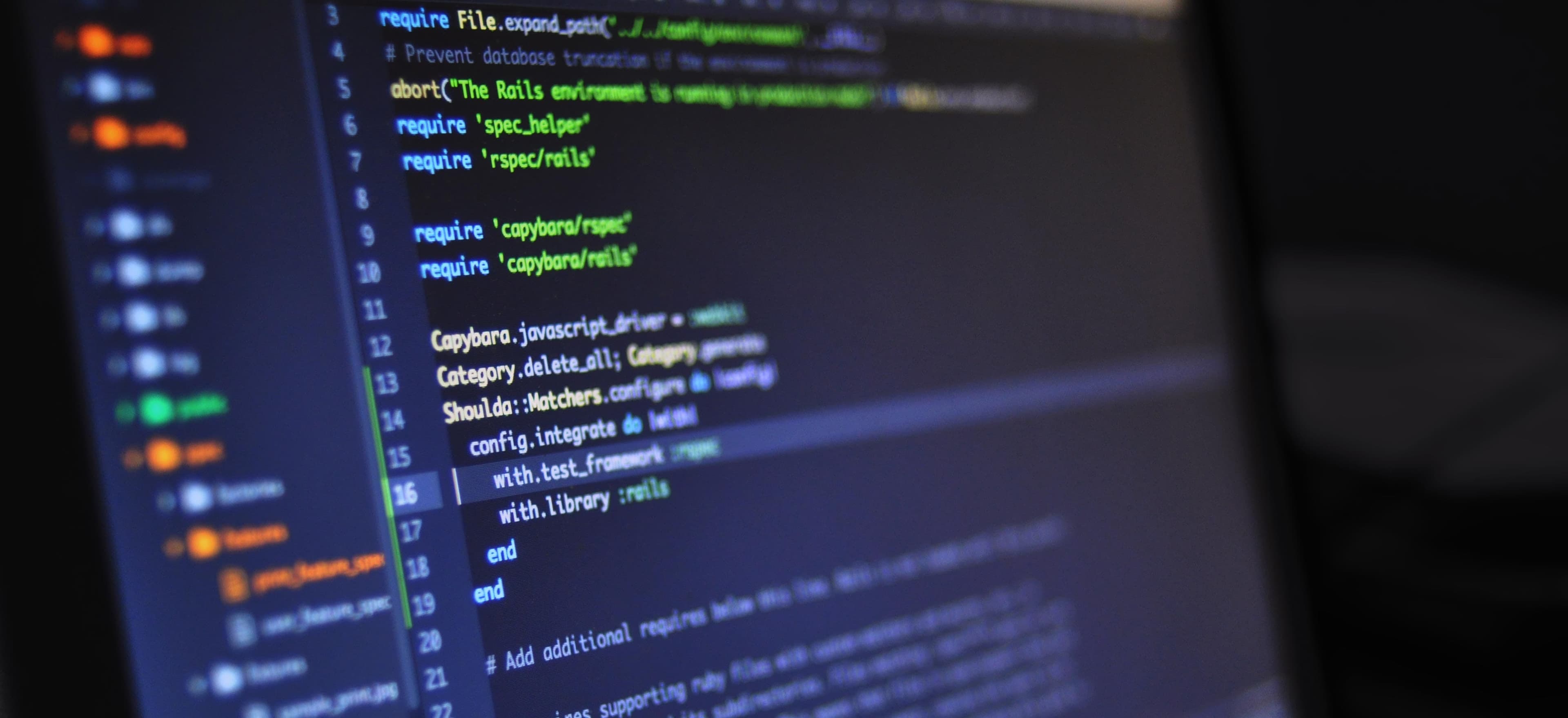
- Published on
Mastering Long Polling in Tomcat with Spring: Common Pitfalls
Long polling is a well-known technique in web development, designed to facilitate asynchronous communication between clients and servers. Especially in environments where you want real-time updates without using full-duplex communication protocols like WebSockets, long polling proves to be incredibly valuable. In this blog, we'll delve into mastering long polling within the Tomcat server using the Spring framework while highlighting common pitfalls you could encounter.
Understanding Long Polling
Long polling is somewhat similar to traditional polling; however, it offers enhanced efficiency. A client sends a request to the server, but instead of an immediate response, the server holds the request open until it has an update to send back.
The Mechanics of Long Polling
- Client Request: The client sends a request to the server.
- Hold Execution: The server holds the connection open until an event happens or a timeout occurs.
- Server Response: Once an event is detected or the timeout is reached, the server responds with the information.
- Repeat: The client immediately re-establishes the connection, starting the process anew.
This essentially creates a pseudo real-time experience.
Setting Up Long Polling in a Spring Application: Initialization
Before we get into the common pitfalls, let’s provide a clear example of how to implement long polling with Spring on a Tomcat server.
1. Spring MVC Configuration
You can create a simple Spring MVC controller that supports long polling.
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import java.util.concurrent.TimeUnit;
@Controller
public class LongPollingController {
@GetMapping("/long-poll")
@ResponseBody
public String longPoll() throws InterruptedException {
// Simulating a delay to mimic an event occurring
TimeUnit.SECONDS.sleep(10);
// Return a simple message
return "Message from server after delay";
}
}
Commentary:
@Controller
indicates that this class not only defines web endpoints but also manages business logic.- The
longPoll
method simulates a scenario where the server will hold the request for 10 seconds before responding. This simulates the delayed response typical of long polling.
2. Configuring Web.xml
If you're using XML-based configuration, ensure you have the correct servlet settings.
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd"
version="3.1">
<servlet>
<servlet-name>dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dispatcher</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
</web-app>
Additional Setup
Do not forget to add Spring dependencies in your project’s pom.xml
if you are using Maven.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
Common Pitfalls in Long Polling with Spring
1. Timeout Configurations
Pitfall: Default Timeout Values
Long polling can become inefficient if server configurations timeout too quickly. When the server times out, the client may receive incomplete information.
Solution
Increase timeout values in your server configuration.
In Tomcat’s server.xml
, adjust the Connector settings:
<Connector port="8080" protocol="HTTP/1.1"
connectionTimeout="20000"
redirectPort="8443" />
Here, we have a connection timeout of 20 seconds, allowing the server to maintain connections longer.
2. Overloading the Server
Pitfall: Too Many Open Connections
A downside of long polling is the potential for the server to become overloaded with open requests. Each client connection occupies a server thread, which could lead to resource constraints.
Solution
Consider implementing a limit on the number of simultaneous long polling requests. If your application scales, consider offloading long-poll requests to a dedicated microservice or utilizing a queuing system.
3. Response Delay and User Experience
Pitfall: Extended Wait Times
Clients may be left waiting without any feedback if the server holds their requests too long.
Solution
Implement a heartbeat mechanism. Instead of letting the client timeout without any feedback, send “heartbeat” messages to indicate that the server is still processing their request.
4. Error Handling
Pitfall: Lack of Error Management
If an error occurs during the long-polling process, simply throwing an exception will disrupt the process, likely leading to confusion for end users.
Solution
You can employ a strategy to catch exceptions and provide feedback to your clients when an error arises.
@GetMapping("/long-poll")
@ResponseBody
public String longPoll() {
try {
// Simulated processing
TimeUnit.SECONDS.sleep(10);
return "Message from server after delay";
} catch (InterruptedException e) {
// Handle error
return "An error occurred while processing your request";
}
}
5. Caching Issues
Pitfall: Stale Responses
With long polling, especially in browsers, caching can lead to serving stale responses to clients.
Solution
Modify your controller method to disable caching explicitly:
@GetMapping("/long-poll")
@ResponseBody
public ResponseEntity<String> longPoll(HttpServletResponse response) {
response.setHeader("Cache-Control", "no-cache, no-store, must-revalidate");
// Process long polling...
}
This will ensure that each request interacts with the server directly without being served a cached response.
The Bottom Line
Long polling remains an effective method for achieving near-real-time communication in web applications. However, pitfalls such as timeouts, server overload, user experience considerations, error management, and caching can pose significant challenges. By keeping these challenges and their solutions in mind, you can implement a robust long polling strategy in your Spring applications running on Tomcat.
For more insights into using Spring effectively, check out the Official Spring Documentation. If you're looking to optimize your web applications even further, consider exploring WebSockets as a more modern approach to achieve real-time communication.
By understanding long polling thoroughly and avoiding common pitfalls, you will be better equipped to deliver a seamless user experience that keeps your clients connected and informed.
Checkout our other articles