Unlocking the True Power of Java 9 Stream API: Pitfalls to Avoid
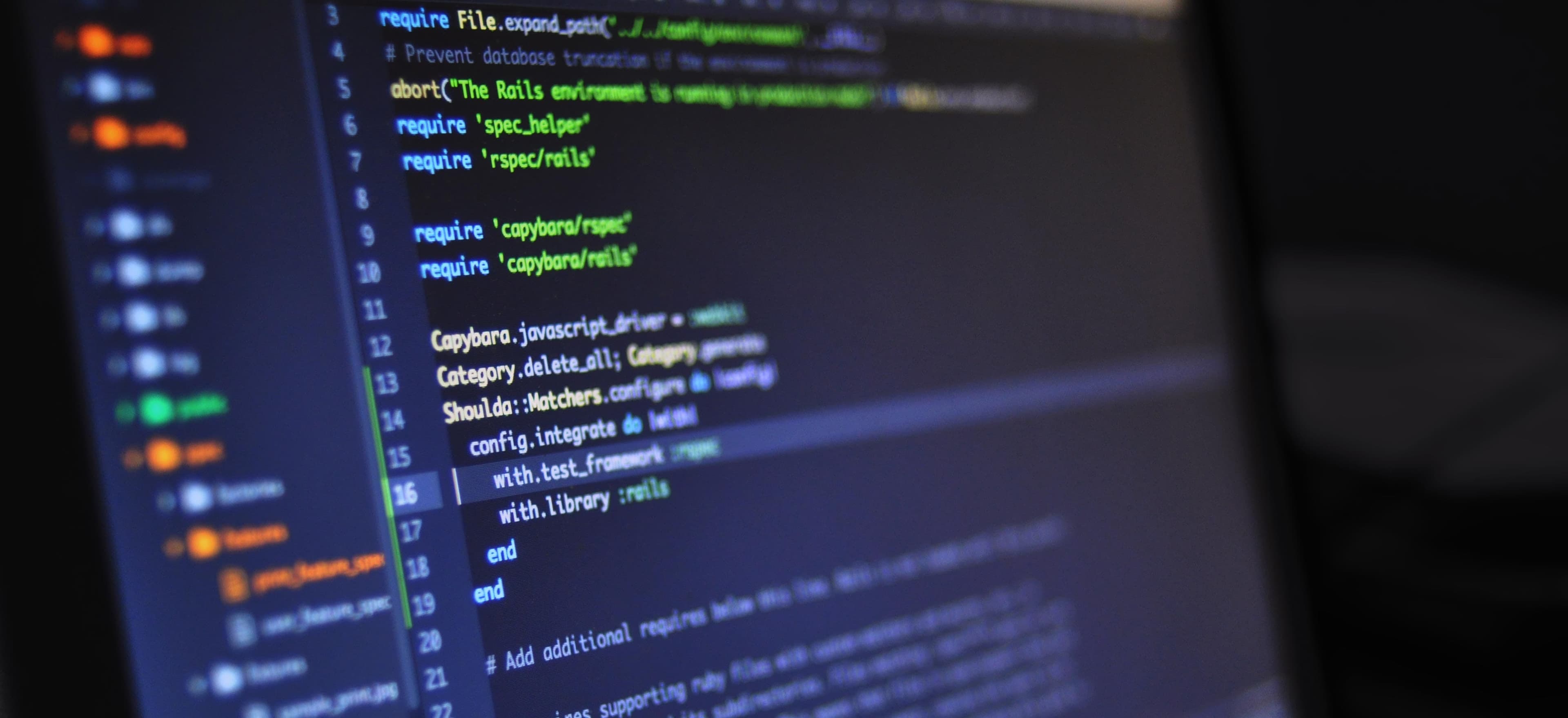
- Published on
Unlocking the True Power of Java 9 Stream API: Pitfalls to Avoid
Java, as one of the most popular programming languages in the world, continuously evolves with each new version. One of the most significant enhancements introduced in Java 8 was the Stream API, which revolutionized how developers handle collections of data. Though Java 9 did not introduce a completely new Stream API, it enhanced its capabilities and added new features that can make coding both easier and more efficient. However, as with any powerful tool, there are pitfalls to avoid to fully benefit from its capabilities. In this blog post, we will delve into the various aspects of the Java 9 Stream API, discussing its enhanced functionality while emphasizing common mistakes developers make.
Understanding Streams and Their Benefits
The Stream API allows you to process data from collections in a functional style. By embracing a declarative approach, you can write more readable and concise code. Here are some key benefits of using the Stream API:
- Parallel Processing: The Stream API allows you to perform operations in parallel with minimal effort.
- Lazy Evaluation: Streams are computed on demand, meaning they don't process data until necessary.
- Chaining Operations: You can chain multiple operations together for clean, expressive coding.
Let's consider a simple example that highlights these concepts:
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Using stream to filter and map values
List<Integer> squares = numbers.stream()
.filter(n -> n % 2 == 0)
.map(n -> n * n)
.toList();
squares.forEach(System.out::println); // Output: 4, 16
}
}
In this code snippet, we filter even numbers and then map them to their squares. Notice how concise and expressive it is, thanks to the Stream API.
Key Features Added in Java 9
Java 9 introduced some enhancements to the Stream API that are worth mentioning:
- TakeWhile and DropWhile Operations: These methods allow you to process elements conditionally, affecting both the beginning and end of the stream.
- OfNullable Method: This static factory method creates a stream containing a single element or an empty stream if the element is null.
Here’s a quick example on how to use takeWhile
:
import java.util.List;
public class TakeWhileExample {
public static void main(String[] args) {
List<Integer> numbers = List.of(1, 2, 3, 4, 5); // Java 9 immutable list
// Using takeWhile to continue iterating until a condition is met
List<Integer> result = numbers.stream()
.takeWhile(n -> n < 4)
.toList();
result.forEach(System.out::println); // Output: 1, 2, 3
}
}
This code showcases a neat way to filter elements based on a condition that involves the sequence and the data itself.
Pitfalls to Avoid in Java 9 Stream API
While the Stream API can be incredibly powerful, developers can run into several pitfalls. Recognizing and avoiding these pitfalls is essential for effective use of Streams.
1. Not Understanding Lazy Evaluation
The Stream API employs lazy evaluation, meaning that operations are not executed until a terminal operation is invoked. This can lead to unexpected behavior, especially if side effects are involved:
List<String> names = Arrays.asList("John", "Jane", "Doe");
names.stream()
.filter(name -> name.startsWith("J"))
.peek(System.out::println); // No output yet
// Terminal operation
long count = names.stream()
.filter(name -> name.startsWith("J"))
.count(); // Count is 2, no peek output
Why this varies: In the above code, the peek
operation doesn't produce output until a terminal operation is reached, as peek
is primarily meant for debugging.
2. Collecting Streams Incorrectly
Using incorrect collectors or failing to understand the collection's type can lead to runtime exceptions or undesired results. For instance, when collecting to a Set:
List<String> names = Arrays.asList("John", "Jane", "John");
Set<String> uniqueNames = names.stream()
.collect(Collectors.toSet());
System.out.println(uniqueNames); // Output: [Jane, John]
Why it fails: If you mistakenly use Collectors.toList()
when you actually need a Set, you might end up with duplicates in your collection.
3. Not Handling Nulls Properly
Nulls can lead to NullPointerException
if not handled correctly. The ofNullable
method is a great tool to avoid this issue:
String name = null;
Stream.ofNullable(name)
.map(String::toUpperCase)
.ifPresent(System.out::println); // No output, no exception thrown
Why it works: This way, the stream is safely created from a nullable source.
4. Overusing Parallel Streams
While parallel streams can improve performance, they are also not a silver bullet. Using them without understanding can lead to overhead and race conditions:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Using parallel stream
numbers.parallelStream()
.map(n -> {
// Potentially costly operation
return n * n;
})
.forEach(System.out::println);
Why caution is needed: The overhead of managing multiple threads may outweigh the benefits, particularly for smaller datasets. Always benchmark when using parallel streams.
5. Ignoring Performance
In certain situations, traditional loops may be more effective than streams. For example, if you need to perform multiple operations in a way that cannot fully leverage a stream, reconsider your approach.
The Last Word
The Stream API in Java 9 offers powerful tools for data manipulation. Understanding its strengths is essential to implement efficient and clean code. However, avoiding common pitfalls is equally crucial. Lazy evaluation, collecting streams properly, handling nulls, and using parallel streams judiciously can ensure that you harness the full potential of the Stream API.
By understanding both its capabilities and the risks involved, developers can write better, more efficient Java code. For further reading on Java’s evolution and its features, check out the Java SE 9 Documentation and explore programming paradigms with a deeper understanding of functional programming in Java.
With practice and understanding, the Java 9 Stream API can unlock new coding capabilities and lead to higher productivity. Happy coding!
Checkout our other articles