Mastering JPA QueryDSL Projections for Efficient Queries
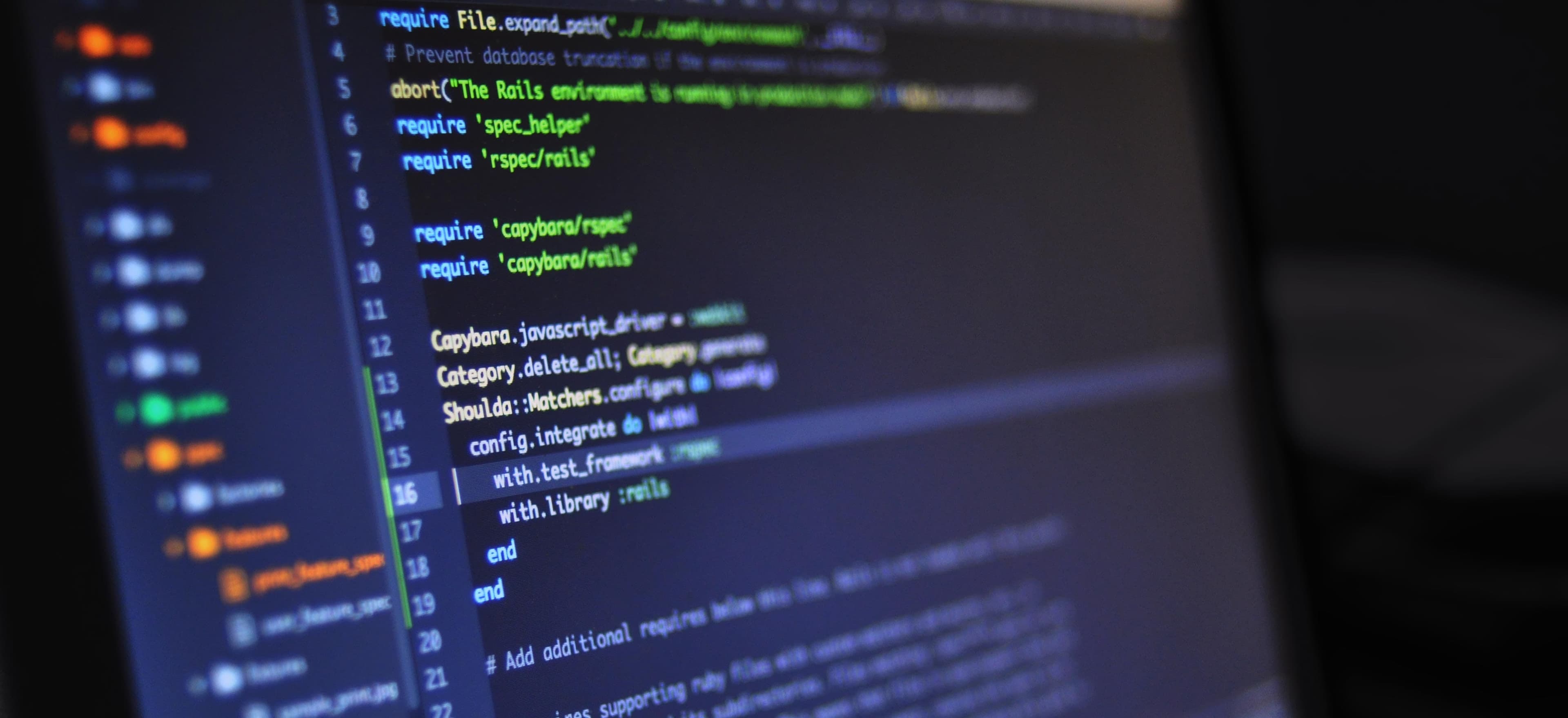
- Published on
Mastering JPA QueryDSL Projections for Efficient Queries
When developing database-driven applications in Java, efficient querying becomes paramount. Java Persistence API (JPA) provides a seamless way to interact with databases through an object-oriented approach, but writing queries can often become cumbersome. Enter QueryDSL, a powerful framework that enhances JPA querying capabilities—especially when working with projections. In this post, we'll explore how to leverage QueryDSL projections for more efficient and cleaner database queries.
What are Projections?
In the context of QueryDSL and JPA, projections allow you to retrieve a subset of a table's rows and columns. By specifying exactly what data to retrieve, you can reduce the amount of data transferred between your database and application, improving performance. Projections enable you to work with DTOs (Data Transfer Objects), allowing you to shape the data as required by your application logic.
Understanding QueryDSL Basics
Before diving into projections, it’s essential to grasp the basics of QueryDSL. QueryDSL provides a typesafe way to construct queries through generated classes. For example, consider a simple User
entity:
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// Getters and Setters
}
Once you have this entity, you need to generate QueryDSL Q-types. These types allow you to build queries without using string-based JPQL or SQL syntax. To generate Q
types, you can use the Maven plugin.
<plugin>
<groupId>com.mysema.maven</groupId>
<artifactId>apt-maven-plugin</artifactId>
<version>1.0.1</version>
<executions>
<execution>
<goals>
<goal>process</goal>
</goals>
</execution>
</executions>
</plugin>
Setting Up Projections with QueryDSL
Now let’s see how to set up projections using QueryDSL. Use a DTO for our projections:
public class UserDTO {
private Long id;
private String name;
public UserDTO(Long id, String name) {
this.id = id;
this.name = name;
}
// Getters and Setters
}
Writing a Projection Query
To write a projection query using QueryDSL, you can use the select
method along with the new
keyword in your query. Here's how you can fetch only the id
and name
fields:
QUser user = QUser.user; // Generated Q-type for User
List<UserDTO> userDTOs = queryFactory.select(
Projections.constructor(UserDTO.class, user.id, user.name))
.from(user)
.fetch();
Why Use Projections?
- Performance: By selecting only the necessary fields, you reduce the amount of data retrieved from the database.
- Clarity: DTOs help encapsulate and express your queries' output clearer, making your code more understandable.
- Maintainability: Changing database schemas becomes easier since the queries are defined in a more structured way.
Advanced Projections: Using Custom Projections
Sometimes you need more flexibility than what standard DTO projections provide. In such cases, you might want to create custom projections. Here's a simple example:
public interface UserProjection {
Long getId();
String getName();
}
Extending your projections to interfaces allows you to take advantage of dynamic projections from QueryDSL.
Fetching with Dynamic Projections
You can modify your fetch call by specifying the projection as follows:
List<UserProjection> projections = queryFactory.select(
Projections.fields(UserProjection.class, user.id, user.name))
.from(user)
.fetch();
This method offers simplicity and flexibility while allowing you to define the structure expected by your application.
Combining Projections with Query Filters
Now that we have an understanding of how to work with projections let's explore how to combine them with queries that filter data.
Example: Filtering Users
Suppose we only want to retrieve users who have "example.com" in their emails. Here’s how you can achieve that:
List<UserDTO> filteredUsers = queryFactory.select(
Projections.constructor(UserDTO.class, user.id, user.name))
.from(user)
.where(user.email.contains("example.com"))
.fetch();
Why Filter with Projections?
When working with larger datasets, filtering helps in reducing the number of records fetched, leading to better performance. Projections additionally ensure you’re only pulling the required data.
Including Joins in Projections
In real-world applications, entities are often related. QueryDSL makes it easy to include joins in your projections.
Example: User with Role
Suppose we have a Role
entity related to the User
entity. Here’s how you could implement a projection that fetches the user along with their roles.
@Entity
public class Role {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String roleName;
@ManyToOne
@JoinColumn(name = "user_id")
private User user;
// Getters and Setters
}
Assuming you have the Q
type for Role as QRole
, you can create a projection like this:
public class UserWithRolesDTO {
private Long userId;
private String userName;
private List<String> roles;
public UserWithRolesDTO(Long userId, String userName, List<String> roles) {
this.userId = userId;
this.userName = userName;
this.roles = roles;
}
// Getters and Setters
}
Writing the Join Query
Here’s how to project the user details along with their roles.
List<UserWithRolesDTO> results = queryFactory.select(
Projections.constructor(UserWithRolesDTO.class,
user.id,
user.name,
JPAExpressions.select(role.roleName)
.from(role)
.where(role.user.id.eq(user.id))
))
.from(user)
.fetch();
Why Use Joins in Projections?
Joins allow you to aggregate related data, minimizing multiple database calls. This is particularly advantageous when you expect to use related data in one go.
Summary
Mastering JPA QueryDSL projections can significantly enhance the efficiency and maintainability of your Java applications. By:
- Selecting specific fields: You reduce database load and bandwidth usage.
- Using DTOs and projections: You deliver clarity and structure to your query results.
- Filtering and joining: You optimize data retrieval, ensuring that your application performs well even as data grows.
To further explore QueryDSL, check the official documentation. With these tips, you're now well-equipped to leverage projections effectively in your Java applications. Start mastering your queries today!
Checkout our other articles