Mastering Java: Ace Your Spring REST Interview Today!
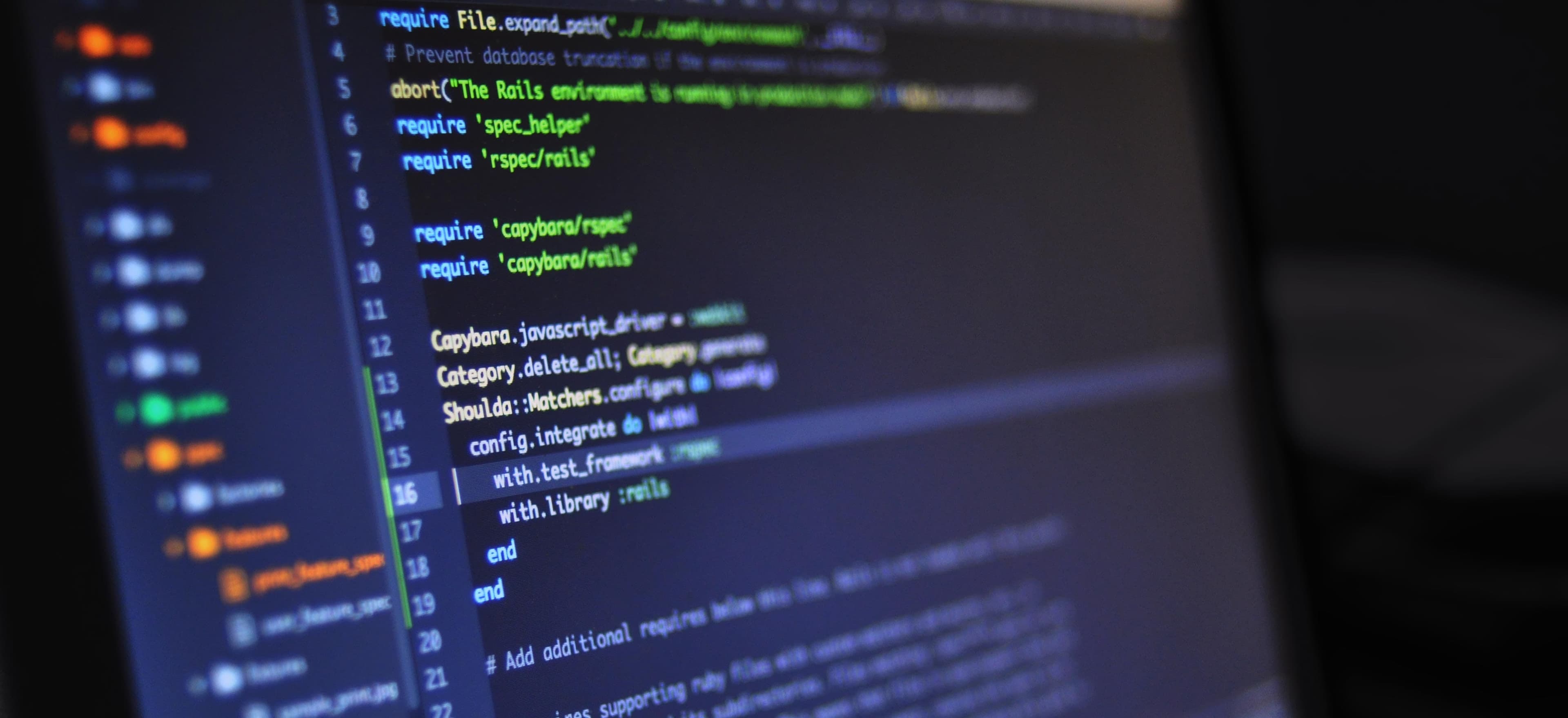
- Published on
Mastering Java: Ace Your Spring REST Interview Today!
In today’s fast-paced software development world, having a strong grasp of Java, particularly its Spring framework, is crucial for success in backend development. Rest APIs built using Spring offer developers a powerful toolkit for creating scalable and robust applications. If you're preparing for a Spring REST API interview, you're in the right place. This blog will cover fundamental concepts, practical code snippets, and tips that will help you shine in your next interview.
Table of Contents
- Understanding Spring and REST
- Building Your First Spring REST API
- Key Annotations in Spring REST
- Exception Handling in Spring
- Security in Spring REST APIs
- Testing Spring REST APIs
- Conclusion
1. Understanding Spring and REST
Before diving into coding, let's clarify what Spring and REST are.
Spring Framework is an extensive Java framework that provides comprehensive features for enterprise applications. It supports various features including Dependency Injection (DI), Aspect-Oriented Programming (AOP), and much more.
REST (Representational State Transfer) is an architectural style that defines a set of constraints for creating web services. It utilizes standard HTTP methods and is stateless. As developers leverage Spring to implement RESTful APIs, understanding how these concepts interplay is vital.
2. Building Your First Spring REST API
Let's set up a simple Spring REST API. Here’s a minimal implementation to get you started:
Maven Dependencies
First, ensure your pom.xml
has the following dependencies:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
Controller Class
Next, let's create a simple controller that handles HTTP requests:
@RestController
@RequestMapping("/api/v1/greeting")
public class GreetingController {
@GetMapping
public ResponseEntity<String> greet() {
return ResponseEntity.ok("Hello, World!");
}
}
Explanation:
- @RestController indicates that this class will handle RESTful web service requests.
- @RequestMapping defines the base URI for all API endpoints in the controller.
- @GetMapping maps HTTP GET requests onto your Java method.
This controller will respond to GET /api/v1/greeting
and return a simple greeting message.
3. Key Annotations in Spring REST
Understanding the key annotations helps streamline REST API development. Here are some of the most vital ones:
- @GetMapping, @PostMapping, @PutMapping, @DeleteMapping: These are specific variations of
@RequestMapping
for different HTTP methods. - @PathVariable: Extracts variables from the URI.
- @RequestParam: Binds HTTP request parameters to Java method parameters.
- @RequestBody: Maps the request body to a Java object.
Example of @RequestBody and @PathVariable
Here is an example where a user can send data to create a greeting:
@PostMapping
public ResponseEntity<String> createGreeting(@RequestBody Greeting greeting) {
return ResponseEntity.status(HttpStatus.CREATED).body("Greeting created: " + greeting.getMessage());
}
In this example:
- The
@RequestBody
annotation automatically deserializes the incoming JSON into aGreeting
object. - The method returns a 201 Created status with the message.
4. Exception Handling in Spring
Handling exceptions gracefully is crucial for a robust API. Here’s a way to centralize exception handling in Spring using @ControllerAdvice
:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<String> handleResourceNotFound(ResourceNotFoundException ex) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body(ex.getMessage());
}
}
Explanation:
- @ControllerAdvice marks the class as a global exception handler.
- @ExceptionHandler(ResourceNotFoundException.class) specifies that the method will handle
ResourceNotFoundException
. - When this exception is thrown, the API responds with a 404 status and an error message.
5. Security in Spring REST APIs
Security must be integrated into RESTful services. Spring Security can be easily set up:
Maven Dependency
Add this dependency to your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
Basic Configuration
Here’s a basic configuration to secure REST endpoints:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers("/api/v1/greeting").permitAll()
.anyRequest().authenticated()
.and()
.httpBasic();
}
}
Explanation:
- @EnableWebSecurity enables the security features.
- Users can access the
/api/v1/greeting
endpoint without authentication, while other endpoints require it.
6. Testing Spring REST APIs
Testing Spring REST applications is essential for ensuring software quality. You can use MockMvc
to test your REST endpoints.
Example Test
@RunWith(SpringRunner.class)
@WebMvcTest(GreetingController.class)
public class GreetingControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void testGreet() throws Exception {
mockMvc.perform(get("/api/v1/greeting"))
.andExpect(status().isOk())
.andExpect(content().string("Hello, World!"));
}
}
Explanation:
- Here we use
@WebMvcTest
to isolate the controller during tests. MockMvc
allows testing of HTTP requests and asserts the response status and content.
7. Conclusion
Mastering Spring REST APIs involves understanding the core concepts, key annotations, security measures, and testing practices. The examples provided illustrate the basics of building a RESTful service, but there’s much more to explore. Stay updated by referencing the Spring official documentation for deeper dives into advanced topics.
As you prepare for your upcoming Spring REST API interview, review these elements, experiment with coding exercises, and practice discussing these topics in-depth. Good luck with your interview, and happy coding!
Checkout our other articles